-
-#if ! defined(MICROS_PER_TICK)
-#define MICROS_PER_TICK 50L // must be with L to get 32 bit results if multiplied with rawbuf[] content.
-#endif
-
-#define MILLIS_IN_ONE_SECOND 1000L
-#define MICROS_IN_ONE_SECOND 1000000L
-#define MICROS_IN_ONE_MILLI 1000L
-
-
-#define CLKFUDGE 5 // fudge factor for clock interrupt overhead
-#define CLK 256 // max value for clock (timer 2)
-#define CLK_16BIT 65536 // max value for clock (timer 1)
-#define PRESCALE 8 // timer2 clock prescale
-#define SYSCLOCK 16000000 // main Arduino clock
-#define CLKSPERUSEC (SYSCLOCK/PRESCALE/1000000) // timer clocks per microsecond
-
-#define ERR 0
-#define DECODED 1
-
-// defines for setting and clearing register bits
-#ifndef cbi
-#define cbi(sfr, bit) (_SFR_BYTE(sfr) &= ~_BV(bit))
-#endif
-#ifndef sbi
-#define sbi(sfr, bit) (_SFR_BYTE(sfr) |= _BV(bit))
-#endif
-
-// clock timer reset value
-#define INIT_TIMER_COUNT (CLK - USECPERTICK*CLKSPERUSEC + CLKFUDGE)
-#define INIT_TIMER_16BIT_COUNT (CLK_16BIT - USECPERTICK*CLKSPERUSEC + CLKFUDGE)
-
-#if defined (__AVR__)
-#define RESET_TIMER1 TCNT1 = INIT_TIMER_16BIT_COUNT
-#define RESET_TIMER2 TCNT2 = INIT_TIMER_COUNT
-#define RESET_TIMER3 TCNT3 = INIT_TIMER_COUNT
-#define RESET_TIMER4 TCNT4 = INIT_TIMER_COUNT
-#elif defined (ESP32)
-#define LEDC_channel_IR 2
-#define LEDC_TIMER_8BIT 8
-#endif
-
-// pulse parameters in usec
-#define NEC_HDR_MARK 9000
-#define NEC_HDR_SPACE 4500
-#define NEC_BIT_MARK 560
-#define NEC_ONE_SPACE 1600
-#define NEC_ZERO_SPACE 560
-#define NEC_RPT_SPACE 2250
-
-#define SONY_HDR_MARK 2400
-#define SONY_HDR_SPACE 600
-#define SONY_ONE_MARK 1200
-#define SONY_ZERO_MARK 600
-#define SONY_RPT_LENGTH 45000
-
-#define RC5_T1 889
-#define RC5_RPT_LENGTH 46000
-
-#define RC6_HDR_MARK 2666
-#define RC6_HDR_SPACE 889
-#define RC6_T1 444
-#define RC6_RPT_LENGTH 46000
-
-#define TOLERANCE 50 // percent tolerance in measurements
-#define LTOL (1.0 - TOLERANCE/100.)
-#define UTOL (1.0 + TOLERANCE/100.)
-
-#define _GAP 5000 // Minimum map between transmissions
-#define GAP_TICKS (_GAP/USECPERTICK)
-
-#define TICKS_LOW(us) (int) (((us)*LTOL/USECPERTICK))
-#define TICKS_HIGH(us) (int) (((us)*UTOL/USECPERTICK + 1))
-
-#ifndef DEBUG
-#define MATCH(measured_ticks, desired_us) ((measured_ticks) >= TICKS_LOW(desired_us) && (measured_ticks) <= TICKS_HIGH(desired_us))
-#define MATCH_MARK(measured_ticks, desired_us) MATCH(measured_ticks, (desired_us))
-#define MATCH_SPACE(measured_ticks, desired_us) MATCH(measured_ticks, (desired_us))
-// Debugging versions are in IRremote.cpp
-#endif
-
-// receiver states
-#define STATE_IDLE 2
-#define STATE_MARK 3
-#define STATE_SPACE 4
-#define STATE_STOP 5
-
-// information for the interrupt handler
-typedef struct {
- uint8_t recvpin; // pin for IR data from detector
- uint8_t rcvstate; // state machine
- unsigned int timer; // state timer, counts 50uS ticks.
- unsigned int rawbuf[RAWBUF]; // raw data
- uint8_t rawlen; // counter of entries in rawbuf
-}
-irparams_t;
-
-// Defined in IRremote.cpp
-extern volatile irparams_t irparams;
-
-// IR detector output is active low
-#define MARK 0
-#define SPACE 1
-
-#define TOPBIT 0x80000000
-
-#define NEC_BITS 32
-#define SONY_BITS 12
-#define MIN_RC5_SAMPLES 11
-#define MIN_RC6_SAMPLES 1
-
-#endif
-
diff --git a/libraries/_07_m_Sensor_IR_Remote/LICENSE b/libraries/_07_m_Sensor_IR_Remote/LICENSE
new file mode 100644
index 00000000..184ff748
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/LICENSE
@@ -0,0 +1,25 @@
+MIT License
+
+(c) Copyright 2009 Ken Shirriff http://www.righto.com
+(c) Copyright 2016 Rafi Khan
+(c) Copyright 2020-2022 Armin Joachimsmeyer et al.
+
+ http://www.opensource.org/licenses/mit-license.php
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/libraries/_07_m_Sensor_IR_Remote/LICENSE.txt b/libraries/_07_m_Sensor_IR_Remote/LICENSE.txt
deleted file mode 100644
index 77cec6dd..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/LICENSE.txt
+++ /dev/null
@@ -1,458 +0,0 @@
-
- GNU LESSER GENERAL PUBLIC LICENSE
- Version 2.1, February 1999
-
- Copyright (C) 1991, 1999 Free Software Foundation, Inc.
- 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
- Everyone is permitted to copy and distribute verbatim copies
- of this license document, but changing it is not allowed.
-
-[This is the first released version of the Lesser GPL. It also counts
- as the successor of the GNU Library Public License, version 2, hence
- the version number 2.1.]
-
- Preamble
-
- The licenses for most software are designed to take away your
-freedom to share and change it. By contrast, the GNU General Public
-Licenses are intended to guarantee your freedom to share and change
-free software--to make sure the software is free for all its users.
-
- This license, the Lesser General Public License, applies to some
-specially designated software packages--typically libraries--of the
-Free Software Foundation and other authors who decide to use it. You
-can use it too, but we suggest you first think carefully about whether
-this license or the ordinary General Public License is the better
-strategy to use in any particular case, based on the explanations below.
-
- When we speak of free software, we are referring to freedom of use,
-not price. Our General Public Licenses are designed to make sure that
-you have the freedom to distribute copies of free software (and charge
-for this service if you wish); that you receive source code or can get
-it if you want it; that you can change the software and use pieces of
-it in new free programs; and that you are informed that you can do
-these things.
-
- To protect your rights, we need to make restrictions that forbid
-distributors to deny you these rights or to ask you to surrender these
-rights. These restrictions translate to certain responsibilities for
-you if you distribute copies of the library or if you modify it.
-
- For example, if you distribute copies of the library, whether gratis
-or for a fee, you must give the recipients all the rights that we gave
-you. You must make sure that they, too, receive or can get the source
-code. If you link other code with the library, you must provide
-complete object files to the recipients, so that they can relink them
-with the library after making changes to the library and recompiling
-it. And you must show them these terms so they know their rights.
-
- We protect your rights with a two-step method: (1) we copyright the
-library, and (2) we offer you this license, which gives you legal
-permission to copy, distribute and/or modify the library.
-
- To protect each distributor, we want to make it very clear that
-there is no warranty for the free library. Also, if the library is
-modified by someone else and passed on, the recipients should know
-that what they have is not the original version, so that the original
-author's reputation will not be affected by problems that might be
-introduced by others.
-
- Finally, software patents pose a constant threat to the existence of
-any free program. We wish to make sure that a company cannot
-effectively restrict the users of a free program by obtaining a
-restrictive license from a patent holder. Therefore, we insist that
-any patent license obtained for a version of the library must be
-consistent with the full freedom of use specified in this license.
-
- Most GNU software, including some libraries, is covered by the
-ordinary GNU General Public License. This license, the GNU Lesser
-General Public License, applies to certain designated libraries, and
-is quite different from the ordinary General Public License. We use
-this license for certain libraries in order to permit linking those
-libraries into non-free programs.
-
- When a program is linked with a library, whether statically or using
-a shared library, the combination of the two is legally speaking a
-combined work, a derivative of the original library. The ordinary
-General Public License therefore permits such linking only if the
-entire combination fits its criteria of freedom. The Lesser General
-Public License permits more lax criteria for linking other code with
-the library.
-
- We call this license the "Lesser" General Public License because it
-does Less to protect the user's freedom than the ordinary General
-Public License. It also provides other free software developers Less
-of an advantage over competing non-free programs. These disadvantages
-are the reason we use the ordinary General Public License for many
-libraries. However, the Lesser license provides advantages in certain
-special circumstances.
-
- For example, on rare occasions, there may be a special need to
-encourage the widest possible use of a certain library, so that it becomes
-a de-facto standard. To achieve this, non-free programs must be
-allowed to use the library. A more frequent case is that a free
-library does the same job as widely used non-free libraries. In this
-case, there is little to gain by limiting the free library to free
-software only, so we use the Lesser General Public License.
-
- In other cases, permission to use a particular library in non-free
-programs enables a greater number of people to use a large body of
-free software. For example, permission to use the GNU C Library in
-non-free programs enables many more people to use the whole GNU
-operating system, as well as its variant, the GNU/Linux operating
-system.
-
- Although the Lesser General Public License is Less protective of the
-users' freedom, it does ensure that the user of a program that is
-linked with the Library has the freedom and the wherewithal to run
-that program using a modified version of the Library.
-
- The precise terms and conditions for copying, distribution and
-modification follow. Pay close attention to the difference between a
-"work based on the library" and a "work that uses the library". The
-former contains code derived from the library, whereas the latter must
-be combined with the library in order to run.
-
- GNU LESSER GENERAL PUBLIC LICENSE
- TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION AND MODIFICATION
-
- 0. This License Agreement applies to any software library or other
-program which contains a notice placed by the copyright holder or
-other authorized party saying it may be distributed under the terms of
-this Lesser General Public License (also called "this License").
-Each licensee is addressed as "you".
-
- A "library" means a collection of software functions and/or data
-prepared so as to be conveniently linked with application programs
-(which use some of those functions and data) to form executables.
-
- The "Library", below, refers to any such software library or work
-which has been distributed under these terms. A "work based on the
-Library" means either the Library or any derivative work under
-copyright law: that is to say, a work containing the Library or a
-portion of it, either verbatim or with modifications and/or translated
-straightforwardly into another language. (Hereinafter, translation is
-included without limitation in the term "modification".)
-
- "Source code" for a work means the preferred form of the work for
-making modifications to it. For a library, complete source code means
-all the source code for all modules it contains, plus any associated
-interface definition files, plus the scripts used to control compilation
-and installation of the library.
-
- Activities other than copying, distribution and modification are not
-covered by this License; they are outside its scope. The act of
-running a program using the Library is not restricted, and output from
-such a program is covered only if its contents constitute a work based
-on the Library (independent of the use of the Library in a tool for
-writing it). Whether that is true depends on what the Library does
-and what the program that uses the Library does.
-
- 1. You may copy and distribute verbatim copies of the Library's
-complete source code as you receive it, in any medium, provided that
-you conspicuously and appropriately publish on each copy an
-appropriate copyright notice and disclaimer of warranty; keep intact
-all the notices that refer to this License and to the absence of any
-warranty; and distribute a copy of this License along with the
-Library.
-
- You may charge a fee for the physical act of transferring a copy,
-and you may at your option offer warranty protection in exchange for a
-fee.
-
- 2. You may modify your copy or copies of the Library or any portion
-of it, thus forming a work based on the Library, and copy and
-distribute such modifications or work under the terms of Section 1
-above, provided that you also meet all of these conditions:
-
- a) The modified work must itself be a software library.
-
- b) You must cause the files modified to carry prominent notices
- stating that you changed the files and the date of any change.
-
- c) You must cause the whole of the work to be licensed at no
- charge to all third parties under the terms of this License.
-
- d) If a facility in the modified Library refers to a function or a
- table of data to be supplied by an application program that uses
- the facility, other than as an argument passed when the facility
- is invoked, then you must make a good faith effort to ensure that,
- in the event an application does not supply such function or
- table, the facility still operates, and performs whatever part of
- its purpose remains meaningful.
-
- (For example, a function in a library to compute square roots has
- a purpose that is entirely well-defined independent of the
- application. Therefore, Subsection 2d requires that any
- application-supplied function or table used by this function must
- be optional: if the application does not supply it, the square
- root function must still compute square roots.)
-
-These requirements apply to the modified work as a whole. If
-identifiable sections of that work are not derived from the Library,
-and can be reasonably considered independent and separate works in
-themselves, then this License, and its terms, do not apply to those
-sections when you distribute them as separate works. But when you
-distribute the same sections as part of a whole which is a work based
-on the Library, the distribution of the whole must be on the terms of
-this License, whose permissions for other licensees extend to the
-entire whole, and thus to each and every part regardless of who wrote
-it.
-
-Thus, it is not the intent of this section to claim rights or contest
-your rights to work written entirely by you; rather, the intent is to
-exercise the right to control the distribution of derivative or
-collective works based on the Library.
-
-In addition, mere aggregation of another work not based on the Library
-with the Library (or with a work based on the Library) on a volume of
-a storage or distribution medium does not bring the other work under
-the scope of this License.
-
- 3. You may opt to apply the terms of the ordinary GNU General Public
-License instead of this License to a given copy of the Library. To do
-this, you must alter all the notices that refer to this License, so
-that they refer to the ordinary GNU General Public License, version 2,
-instead of to this License. (If a newer version than version 2 of the
-ordinary GNU General Public License has appeared, then you can specify
-that version instead if you wish.) Do not make any other change in
-these notices.
-
- Once this change is made in a given copy, it is irreversible for
-that copy, so the ordinary GNU General Public License applies to all
-subsequent copies and derivative works made from that copy.
-
- This option is useful when you wish to copy part of the code of
-the Library into a program that is not a library.
-
- 4. You may copy and distribute the Library (or a portion or
-derivative of it, under Section 2) in object code or executable form
-under the terms of Sections 1 and 2 above provided that you accompany
-it with the complete corresponding machine-readable source code, which
-must be distributed under the terms of Sections 1 and 2 above on a
-medium customarily used for software interchange.
-
- If distribution of object code is made by offering access to copy
-from a designated place, then offering equivalent access to copy the
-source code from the same place satisfies the requirement to
-distribute the source code, even though third parties are not
-compelled to copy the source along with the object code.
-
- 5. A program that contains no derivative of any portion of the
-Library, but is designed to work with the Library by being compiled or
-linked with it, is called a "work that uses the Library". Such a
-work, in isolation, is not a derivative work of the Library, and
-therefore falls outside the scope of this License.
-
- However, linking a "work that uses the Library" with the Library
-creates an executable that is a derivative of the Library (because it
-contains portions of the Library), rather than a "work that uses the
-library". The executable is therefore covered by this License.
-Section 6 states terms for distribution of such executables.
-
- When a "work that uses the Library" uses material from a header file
-that is part of the Library, the object code for the work may be a
-derivative work of the Library even though the source code is not.
-Whether this is true is especially significant if the work can be
-linked without the Library, or if the work is itself a library. The
-threshold for this to be true is not precisely defined by law.
-
- If such an object file uses only numerical parameters, data
-structure layouts and accessors, and small macros and small inline
-functions (ten lines or less in length), then the use of the object
-file is unrestricted, regardless of whether it is legally a derivative
-work. (Executables containing this object code plus portions of the
-Library will still fall under Section 6.)
-
- Otherwise, if the work is a derivative of the Library, you may
-distribute the object code for the work under the terms of Section 6.
-Any executables containing that work also fall under Section 6,
-whether or not they are linked directly with the Library itself.
-
- 6. As an exception to the Sections above, you may also combine or
-link a "work that uses the Library" with the Library to produce a
-work containing portions of the Library, and distribute that work
-under terms of your choice, provided that the terms permit
-modification of the work for the customer's own use and reverse
-engineering for debugging such modifications.
-
- You must give prominent notice with each copy of the work that the
-Library is used in it and that the Library and its use are covered by
-this License. You must supply a copy of this License. If the work
-during execution displays copyright notices, you must include the
-copyright notice for the Library among them, as well as a reference
-directing the user to the copy of this License. Also, you must do one
-of these things:
-
- a) Accompany the work with the complete corresponding
- machine-readable source code for the Library including whatever
- changes were used in the work (which must be distributed under
- Sections 1 and 2 above); and, if the work is an executable linked
- with the Library, with the complete machine-readable "work that
- uses the Library", as object code and/or source code, so that the
- user can modify the Library and then relink to produce a modified
- executable containing the modified Library. (It is understood
- that the user who changes the contents of definitions files in the
- Library will not necessarily be able to recompile the application
- to use the modified definitions.)
-
- b) Use a suitable shared library mechanism for linking with the
- Library. A suitable mechanism is one that (1) uses at run time a
- copy of the library already present on the user's computer system,
- rather than copying library functions into the executable, and (2)
- will operate properly with a modified version of the library, if
- the user installs one, as long as the modified version is
- interface-compatible with the version that the work was made with.
-
- c) Accompany the work with a written offer, valid for at
- least three years, to give the same user the materials
- specified in Subsection 6a, above, for a charge no more
- than the cost of performing this distribution.
-
- d) If distribution of the work is made by offering access to copy
- from a designated place, offer equivalent access to copy the above
- specified materials from the same place.
-
- e) Verify that the user has already received a copy of these
- materials or that you have already sent this user a copy.
-
- For an executable, the required form of the "work that uses the
-Library" must include any data and utility programs needed for
-reproducing the executable from it. However, as a special exception,
-the materials to be distributed need not include anything that is
-normally distributed (in either source or binary form) with the major
-components (compiler, kernel, and so on) of the operating system on
-which the executable runs, unless that component itself accompanies
-the executable.
-
- It may happen that this requirement contradicts the license
-restrictions of other proprietary libraries that do not normally
-accompany the operating system. Such a contradiction means you cannot
-use both them and the Library together in an executable that you
-distribute.
-
- 7. You may place library facilities that are a work based on the
-Library side-by-side in a single library together with other library
-facilities not covered by this License, and distribute such a combined
-library, provided that the separate distribution of the work based on
-the Library and of the other library facilities is otherwise
-permitted, and provided that you do these two things:
-
- a) Accompany the combined library with a copy of the same work
- based on the Library, uncombined with any other library
- facilities. This must be distributed under the terms of the
- Sections above.
-
- b) Give prominent notice with the combined library of the fact
- that part of it is a work based on the Library, and explaining
- where to find the accompanying uncombined form of the same work.
-
- 8. You may not copy, modify, sublicense, link with, or distribute
-the Library except as expressly provided under this License. Any
-attempt otherwise to copy, modify, sublicense, link with, or
-distribute the Library is void, and will automatically terminate your
-rights under this License. However, parties who have received copies,
-or rights, from you under this License will not have their licenses
-terminated so long as such parties remain in full compliance.
-
- 9. You are not required to accept this License, since you have not
-signed it. However, nothing else grants you permission to modify or
-distribute the Library or its derivative works. These actions are
-prohibited by law if you do not accept this License. Therefore, by
-modifying or distributing the Library (or any work based on the
-Library), you indicate your acceptance of this License to do so, and
-all its terms and conditions for copying, distributing or modifying
-the Library or works based on it.
-
- 10. Each time you redistribute the Library (or any work based on the
-Library), the recipient automatically receives a license from the
-original licensor to copy, distribute, link with or modify the Library
-subject to these terms and conditions. You may not impose any further
-restrictions on the recipients' exercise of the rights granted herein.
-You are not responsible for enforcing compliance by third parties with
-this License.
-
- 11. If, as a consequence of a court judgment or allegation of patent
-infringement or for any other reason (not limited to patent issues),
-conditions are imposed on you (whether by court order, agreement or
-otherwise) that contradict the conditions of this License, they do not
-excuse you from the conditions of this License. If you cannot
-distribute so as to satisfy simultaneously your obligations under this
-License and any other pertinent obligations, then as a consequence you
-may not distribute the Library at all. For example, if a patent
-license would not permit royalty-free redistribution of the Library by
-all those who receive copies directly or indirectly through you, then
-the only way you could satisfy both it and this License would be to
-refrain entirely from distribution of the Library.
-
-If any portion of this section is held invalid or unenforceable under any
-particular circumstance, the balance of the section is intended to apply,
-and the section as a whole is intended to apply in other circumstances.
-
-It is not the purpose of this section to induce you to infringe any
-patents or other property right claims or to contest validity of any
-such claims; this section has the sole purpose of protecting the
-integrity of the free software distribution system which is
-implemented by public license practices. Many people have made
-generous contributions to the wide range of software distributed
-through that system in reliance on consistent application of that
-system; it is up to the author/donor to decide if he or she is willing
-to distribute software through any other system and a licensee cannot
-impose that choice.
-
-This section is intended to make thoroughly clear what is believed to
-be a consequence of the rest of this License.
-
- 12. If the distribution and/or use of the Library is restricted in
-certain countries either by patents or by copyrighted interfaces, the
-original copyright holder who places the Library under this License may add
-an explicit geographical distribution limitation excluding those countries,
-so that distribution is permitted only in or among countries not thus
-excluded. In such case, this License incorporates the limitation as if
-written in the body of this License.
-
- 13. The Free Software Foundation may publish revised and/or new
-versions of the Lesser General Public License from time to time.
-Such new versions will be similar in spirit to the present version,
-but may differ in detail to address new problems or concerns.
-
-Each version is given a distinguishing version number. If the Library
-specifies a version number of this License which applies to it and
-"any later version", you have the option of following the terms and
-conditions either of that version or of any later version published by
-the Free Software Foundation. If the Library does not specify a
-license version number, you may choose any version ever published by
-the Free Software Foundation.
-
- 14. If you wish to incorporate parts of the Library into other free
-programs whose distribution conditions are incompatible with these,
-write to the author to ask for permission. For software which is
-copyrighted by the Free Software Foundation, write to the Free
-Software Foundation; we sometimes make exceptions for this. Our
-decision will be guided by the two goals of preserving the free status
-of all derivatives of our free software and of promoting the sharing
-and reuse of software generally.
-
- NO WARRANTY
-
- 15. BECAUSE THE LIBRARY IS LICENSED FREE OF CHARGE, THERE IS NO
-WARRANTY FOR THE LIBRARY, TO THE EXTENT PERMITTED BY APPLICABLE LAW.
-EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR
-OTHER PARTIES PROVIDE THE LIBRARY "AS IS" WITHOUT WARRANTY OF ANY
-KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE
-IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
-PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE
-LIBRARY IS WITH YOU. SHOULD THE LIBRARY PROVE DEFECTIVE, YOU ASSUME
-THE COST OF ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
-
- 16. IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN
-WRITING WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MAY MODIFY
-AND/OR REDISTRIBUTE THE LIBRARY AS PERMITTED ABOVE, BE LIABLE TO YOU
-FOR DAMAGES, INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL OR
-CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OR INABILITY TO USE THE
-LIBRARY (INCLUDING BUT NOT LIMITED TO LOSS OF DATA OR DATA BEING
-RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD PARTIES OR A
-FAILURE OF THE LIBRARY TO OPERATE WITH ANY OTHER SOFTWARE), EVEN IF
-SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH
-DAMAGES.
-
diff --git a/libraries/_07_m_Sensor_IR_Remote/README.md b/libraries/_07_m_Sensor_IR_Remote/README.md
new file mode 100644
index 00000000..6315c9ee
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/README.md
@@ -0,0 +1,952 @@
+
+
+# Arduino IRremote
+A library enabling the sending & receiving of infra-red signals.
+
+[](https://opensource.org/licenses/MIT)
+
+[](https://github.com/Arduino-IRremote/Arduino-IRremote/releases/latest)
+
+[](https://github.com/Arduino-IRremote/Arduino-IRremote/commits/master)
+
+[](https://github.com/Arduino-IRremote/Arduino-IRremote/actions)
+
+
+[](https://stand-with-ukraine.pp.ua)
+
+Available as [Arduino library "IRremote"](https://www.arduinolibraries.info/libraries/i-rremote).
+
+[](https://www.ardu-badge.com/IRremote)
+
+[](https://arduino-irremote.github.io/Arduino-IRremote/classIRrecv.html)
+
+[](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/changelog.md)
+
+[](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/Contributing.md)
+
+#### If you find this program useful, please give it a star.
+
+🌎 [Google Translate](https://translate.google.com/translate?sl=en&u=https://github.com/Arduino-IRremote/Arduino-IRremote)
+
+
+
+# Overview
+- [Supported IR Protocols](https://github.com/Arduino-IRremote/Arduino-IRremote#supported-ir-protocols)
+- [Features](https://github.com/Arduino-IRremote/Arduino-IRremote#features)
+ * [New features with version 4.x](https://github.com/Arduino-IRremote/Arduino-IRremote#new-features-with-version-4x)
+ * [New features with version 3.x](https://github.com/Arduino-IRremote/Arduino-IRremote#new-features-with-version-3x)
+- [Converting your 2.x program to the 4.x version](https://github.com/Arduino-IRremote/Arduino-IRremote#converting-your-2x-program-to-the-4x-version)
+ * [How to convert old MSB first 32 bit IR data codes to new LSB first 32 bit IR data codes](https://github.com/Arduino-IRremote/Arduino-IRremote#how-to-convert-old-msb-first-32-bit-ir-data-codes-to-new-lsb-first-32-bit-ir-data-codes)
+- [Errors with using the 3.x versions for old tutorials](https://github.com/Arduino-IRremote/Arduino-IRremote#errors-with-using-the-3x-versions-for-old-tutorials)
+ * [Staying on 2.x](https://github.com/Arduino-IRremote/Arduino-IRremote#staying-on-2x)
+- [Why *.hpp instead of *.cpp](https://github.com/Arduino-IRremote/Arduino-IRremote#why-hpp-instead-of-cpp)
+- [Using the new *.hpp files](https://github.com/Arduino-IRremote/Arduino-IRremote#using-the-new-hpp-files)
+- [Receiving IR codes](https://github.com/Arduino-IRremote/Arduino-IRremote#receiving-ir-codes)
+ * [decodedIRData structure](https://github.com/Arduino-IRremote/Arduino-IRremote#decodedirdata-structure)
+ * [Ambiguous protocols](https://github.com/Arduino-IRremote/Arduino-IRremote#ambiguous-protocols)
+ * [Unknown protocol](https://github.com/Arduino-IRremote/Arduino-IRremote#unknown-protocol)
+- [Sending IR codes](https://github.com/Arduino-IRremote/Arduino-IRremote#sending-ir-codes)
+ * [Send pin](https://github.com/Arduino-IRremote/Arduino-IRremote#send-pin)
+ + [List of public IR code databases](https://github.com/Arduino-IRremote/Arduino-IRremote#list-of-public-ir-code-databases)
+- [Tiny NEC receiver and sender](https://github.com/Arduino-IRremote/Arduino-IRremote#tiny-nec-receiver-and-sender)
+- [The FAST protocol](https://github.com/Arduino-IRremote/Arduino-IRremote#the-fast-protocol)
+- [FAQ and hints](https://github.com/Arduino-IRremote/Arduino-IRremote#faq-and-hints)
+ * [Problems with Neopixels, FastLed etc.](https://github.com/Arduino-IRremote/Arduino-IRremote#problems-with-neopixels-fastled-etc)
+ * [Does not work/compile with another library](https://github.com/Arduino-IRremote/Arduino-IRremote#does-not-workcompile-with-another-library)
+ * [Multiple IR receiver](https://github.com/Arduino-IRremote/Arduino-IRremote#multiple-ir-receiver)
+ * [Increase strength of sent output signal](https://github.com/Arduino-IRremote/Arduino-IRremote#increase-strength-of-sent-output-signal)
+ * [Minimal CPU clock frequency](https://github.com/Arduino-IRremote/Arduino-IRremote#minimal-cpu-clock-frequency)
+ * [Bang & Olufsen protocol](https://github.com/Arduino-IRremote/Arduino-IRremote#bang--olufsen-protocol)
+- [Handling unknown Protocols](https://github.com/Arduino-IRremote/Arduino-IRremote#handling-unknown-protocols)
+ * [Disclaimer](https://github.com/Arduino-IRremote/Arduino-IRremote#disclaimer)
+ * [Protocol=PULSE_DISTANCE](https://github.com/Arduino-IRremote/Arduino-IRremote#protocolpulse_distance)
+ * [Protocol=UNKNOWN](https://github.com/Arduino-IRremote/Arduino-IRremote#protocolunknown)
+ * [How to deal with protocols not supported by IRremote](https://github.com/Arduino-IRremote/Arduino-IRremote#how-to-deal-with-protocols-not-supported-by-irremote)
+- [Examples for this library](https://github.com/Arduino-IRremote/Arduino-IRremote#examples-for-this-library)
+- [WOKWI online examples](https://github.com/Arduino-IRremote/Arduino-IRremote#wokwi-online-examples)
+- [Issues and discussions](https://github.com/Arduino-IRremote/Arduino-IRremote#issues-and-discussions)
+- [Compile options / macros for this library](https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library)
+ + [Changing include (*.h) files with Arduino IDE](https://github.com/Arduino-IRremote/Arduino-IRremote#changing-include-h-files-with-arduino-ide)
+ + [Modifying compile options with Sloeber IDE](https://github.com/Arduino-IRremote/Arduino-IRremote#modifying-compile-options--macros-with-sloeber-ide)
+- [Supported Boards](https://github.com/Arduino-IRremote/Arduino-IRremote#supported-boards)
+- [Timer and pin usage](https://github.com/Arduino-IRremote/Arduino-IRremote#timer-and-pin-usage)
+ * [Incompatibilities to other libraries and Arduino commands like tone() and analogWrite()](https://github.com/Arduino-IRremote/Arduino-IRremote#incompatibilities-to-other-libraries-and-arduino-commands-like-tone-and-analogwrite)
+ * [Hardware-PWM signal generation for sending](https://github.com/Arduino-IRremote/Arduino-IRremote#hardware-pwm-signal-generation-for-sending)
+ * [Why do we use 30% duty cycle for sending](https://github.com/Arduino-IRremote/Arduino-IRremote#why-do-we-use-30-duty-cycle-for-sending)
+- [How we decode signals](https://github.com/Arduino-IRremote/Arduino-IRremote#how-we-decode-signals)
+- [NEC encoding diagrams](https://github.com/Arduino-IRremote/Arduino-IRremote#nec-encoding-diagrams)
+- [Quick comparison of 5 Arduino IR receiving libraries](https://github.com/Arduino-IRremote/Arduino-IRremote#quick-comparison-of-5-arduino-ir-receiving-libraries)
+- [Useful links](https://github.com/Arduino-IRremote/Arduino-IRremote#useful-links)
+- [Contributors](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/Contributors.md)
+- [License](https://github.com/Arduino-IRremote/Arduino-IRremote#license)
+- [Copyright](https://github.com/Arduino-IRremote/Arduino-IRremote#copyright)
+
+
+
+# Supported IR Protocols
+` NEC / Onkyo / Apple ` ` Denon / Sharp ` ` Panasonic / Kaseikyo `
+
+` JVC ` ` LG ` ` RC5 ` ` RC6 ` ` Samsung ` ` Sony `
+
+` Universal Pulse Distance ` ` Universal Pulse Width ` ` Hash ` ` Pronto `
+
+` BoseWave ` ` Bang & Olufsen ` ` Lego ` ` FAST ` ` Whynter ` ` MagiQuest `
+
+Protocols can be switched off and on by defining macros before the line `#include ` like [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SimpleReceiver/SimpleReceiver.ino#L33):
+
+```c++
+#define DECODE_NEC
+//#define DECODE_DENON
+#include
+```
+
+
+# Features
+- Lots of tutorials and examples.
+- Actively maintained.
+- Allows receiving and sending of **raw timing data**.
+
+## New features with version 4.x
+- New universal **Pulse Distance / Pulse Width decoder** added, which covers many previous unknown protocols.
+- Printout of code how to send received command by `IrReceiver.printIRSendUsage(&Serial)`.
+- RawData type is now 64 bit for 32 bit platforms and therefore `decodedIRData.decodedRawData` can contain complete frame information for more protocols than with 32 bit as before.
+- Callback after receiving a command - It calls your code as soon as a message was received.
+- Improved handling of `PULSE_DISTANCE` + `PULSE_WIDTH` protocols.
+- New FAST protocol.
+
+#### Converting your 3.x program to the 4.x version
+- You must replace `#define DECODE_DISTANCE` by `#define DECODE_DISTANCE_WIDTH` (only if you explicitly enabled this decoder).
+- The parameter `bool hasStopBit` is not longer required and removed e.g. for function `sendPulseDistanceWidth()`.
+
+## New features with version 3.x
+- **Any pin** can be used for sending -if `SEND_PWM_BY_TIMER` is not defined- and receiving.
+- Feedback LED can be activated for sending / receiving.
+- An 8/16 bit ****command** value as well as an 16 bit **address** and a protocol number is provided for decoding (instead of the old 32 bit value).
+- Protocol values comply to **protocol standards**.
+ NEC, Panasonic, Sony, Samsung and JVC decode & send LSB first.
+- Supports **Universal Distance protocol**, which covers a lot of previous unknown protocols.
+- Compatible with **tone()** library. See the [ReceiveDemo](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/21b5747a58e9d47c9e3f1beb056d58c875a92b47/examples/ReceiveDemo/ReceiveDemo.ino#L159-L169) example.
+- Simultaneous sending and receiving. See the [SendAndReceive](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SendAndReceive/SendAndReceive.ino#L167-L170) example.
+- Supports **more platforms**.
+- Allows for the generation of non PWM signal to just **simulate an active low receiver signal** for direct connect to existent receiving devices without using IR.
+- Easy protocol configuration, **directly in your [source code](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SimpleReceiver/SimpleReceiver.ino#L33-L57)**.
+ Reduces memory footprint and decreases decoding time.
+- Contains a [very small NEC only decoder](https://github.com/Arduino-IRremote/Arduino-IRremote#minimal-nec-receiver), which **does not require any timer resource**.
+
+[-> Feature comparison of 5 Arduino IR libraries](https://github.com/Arduino-IRremote/Arduino-IRremote#quick-comparison-of-5-arduino-ir-receiving-libraries).
+
+
+
+# Converting your 2.x program to the 4.x version
+Starting with the 3.1 version, **the generation of PWM for sending is done by software**, thus saving the hardware timer and **enabling arbitrary output pins for sending**.
+If you use an (old) Arduino core that does not use the `-flto` flag for compile, you can activate the line `#define SUPPRESS_ERROR_MESSAGE_FOR_BEGIN` in IRRemote.h, if you get false error messages regarding begin() during compilation.
+
+- **IRreceiver** and **IRsender** object have been added and can be used without defining them, like the well known Arduino **Serial** object.
+- Just remove the line `IRrecv IrReceiver(IR_RECEIVE_PIN);` and/or `IRsend IrSender;` in your program, and replace all occurrences of `IRrecv.` or `irrecv.` with `IrReceiver` and replace all `IRsend` or `irsend` with `IrSender`.
+- Since the decoded values are now in `IrReceiver.decodedIRData` and not in `results` any more, remove the line `decode_results results` or similar.
+- Like for the Serial object, call [`IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK)`](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/ReceiveDemo/ReceiveDemo.ino#L106)
+ or `IrReceiver.begin(IR_RECEIVE_PIN, DISABLE_LED_FEEDBACK)` instead of the `IrReceiver.enableIRIn()` or `irrecv.enableIRIn()` in setup().
+For sending, call `IrSender.begin();` or `IrSender.begin(DISABLE_LED_FEEDBACK);` in setup().
+If IR_SEND_PIN is not defined (before the line `#include `) you must use e.g. `IrSender.begin(3, ENABLE_LED_FEEDBACK, USE_DEFAULT_FEEDBACK_LED_PIN);`
+- Old `decode(decode_results *aResults)` function is replaced by simple `decode()`. So if you have a statement `if(irrecv.decode(&results))` replace it with `if (IrReceiver.decode())`.
+- The decoded result is now in in `IrReceiver.decodedIRData` and not in `results` any more, therefore replace any occurrences of `results.value` and `results.decode_type` (and similar) to
+ `IrReceiver.decodedIRData.decodedRawData` and `IrReceiver.decodedIRData.protocol`.
+- Overflow, Repeat and other flags are now in [`IrReceiver.receivedIRData.flags`](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRProtocol.h#L90-L101).
+- Seldom used: `results.rawbuf` and `results.rawlen` must be replaced by `IrReceiver.decodedIRData.rawDataPtr->rawbuf` and `IrReceiver.decodedIRData.rawDataPtr->rawlen`.
+
+- The 5 protocols **NEC, Panasonic, Sony, Samsung and JVC** have been converted to LSB first. Send functions for sending old MSB data for **NEC** and **JVC** were renamed to `sendNECMSB`, and `sendJVCMSB()`. The old `sendSAMSUNG()` and `sendSony()` MSB functions are still available. The old MSB version of `sendPanasonic()` function was deleted, since it had bugs nobody recognized.
+For converting MSB codes to LSB see [below](https://github.com/Arduino-IRremote/Arduino-IRremote#how-to-convert-old-msb-first-32-bit-ir-data-codes-to-new-lsb-first-32-bit-ir-data-codes).
+
+### Example
+#### Old 2.x program:
+
+```c++
+#include
+#define RECV_PIN 2
+
+IRrecv irrecv(RECV_PIN);
+decode_results results;
+
+void setup()
+{
+...
+ irrecv.enableIRIn(); // Start the receiver
+}
+
+void loop() {
+ if (irrecv.decode(&results)) {
+ Serial.println(results.value, HEX);
+ ...
+ irrecv.resume(); // Receive the next value
+ }
+ ...
+}
+```
+
+#### New 4.x program:
+
+```c++
+#include
+#define IR_RECEIVE_PIN 2
+
+void setup()
+{
+...
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK); // Start the receiver
+}
+
+void loop() {
+ if (IrReceiver.decode()) {
+ Serial.println(IrReceiver.decodedIRData.decodedRawData, HEX); // Print "old" raw data
+ // USE NEW 3.x FUNCTIONS
+ IrReceiver.printIRResultShort(&Serial); // Print complete received data in one line
+ IrReceiver.printIRSendUsage(&Serial); // Print the statement required to send this data
+ ...
+ IrReceiver.resume(); // Enable receiving of the next value
+ }
+ ...
+}
+```
+
+## How to convert old MSB first 32 bit IR data codes to new LSB first 32 bit IR data codes
+For the new decoders for **NEC, Panasonic, Sony, Samsung and JVC**, the result `IrReceiver.decodedIRData.decodedRawData` is now **LSB-first**, as the definition of these protocols suggests!
+
+To convert one into the other, you must reverse the byte/nibble positions and then reverse all bit positions of each byte/nibble or write it as one binary string and reverse/mirror it.
+Example:
+`0xCB 34 01 02`
+`0x20 10 43 BC` after nibble reverse
+`0x40 80 2C D3` after bit reverse of each nibble
+### Nibble reverse map:
+```
+ 0->0 1->8 2->4 3->C
+ 4->2 5->A 6->6 7->E
+ 8->1 9->9 A->5 B->D
+ C->3 D->B E->7 F->F
+```
+`0xCB340102` is binary `1100 1011 0011 0100 0000 0001 0000 0010`.
+`0x40802CD3` is binary `0100 0000 1000 0000 0010 1100 1101 0011`.
+If you **read the first binary sequence backwards** (right to left), you get the second sequence.
+You may use `bitreverseOneByte()` or `bitreverse32Bit()` for this.
+
+
+
+# Errors with using the 4.x versions for old tutorials
+If you suffer from errors with old tutorial code including `IRremote.h` instead of `IRremote.hpp`, just try to rollback to [Version 2.4.0](https://github.com/Arduino-IRremote/Arduino-IRremote/releases/tag/v2.4.0).
+Most likely your code will run and you will not miss the new features...
+
+
+
+## Staying on 2.x
+Consider using the [original 2.4 release form 2017](https://github.com/Arduino-IRremote/Arduino-IRremote/releases/tag/v2.4.0)
+or the last backwards compatible [2.8 version](https://github.com/Arduino-IRremote/Arduino-IRremote/releases/tag/2.8.0) for you project.
+It may be sufficient and deals flawlessly with 32 bit IR codes.
+If this doesn't fit your case, be assured that 3.x is at least trying to be backwards compatible, so your old examples should still work fine.
+
+### Drawbacks
+- Only the following decoders are available:
+ ` NEC ` ` Denon ` ` Panasonic ` ` JVC ` ` LG `
+ ` RC5 ` ` RC6 ` ` Samsung ` ` Sony `
+- The call of `irrecv.decode(&results)` uses the old MSB first decoders like in 2.x and sets the 32 bit codes in `results.value`.
+- The old functions `sendNEC()` and `sendJVC()` are renamed to `sendNECMSB()` and `sendJVCMSB()`.
+ Use them to send your **old MSB-first 32 bit IR data codes**.
+- No decoding by a (constant) 8/16 bit address and an 8 bit command.
+
+
+
+# Why *.hpp instead of *.cpp?
+**Every \*.cpp file is compiled separately** by a call of the compiler exclusively for this cpp file. These calls are managed by the IDE / make system.
+In the Arduino IDE the calls are executed when you click on *Verify* or *Upload*.
+
+And now our problem with Arduino is:
+**How to set [compile options](#compile-options--macros-for-this-library) for all *.cpp files, especially for libraries used?**
+IDE's like [Sloeber](https://github.com/ArminJo/ServoEasing#modifying-compile-options--macros-with-sloeber-ide) or [PlatformIO](https://github.com/ArminJo/ServoEasing#modifying-compile-options--macros-with-platformio) support this by allowing to specify a set of options per project.
+They add these options at each compiler call e.g. `-DTRACE`.
+
+But Arduino lacks this feature.
+So the **workaround** is not to compile all sources separately, but to concatenate them to one huge source file by including them in your source.
+This is done by e.g. `#include "IRremote.hpp"`.
+
+But why not `#include "IRremote.cpp"`?
+Try it and you will see tons of errors, because each function of the *.cpp file is now compiled twice,
+first by compiling the huge file and second by compiling the *.cpp file separately, like described above.
+So using the extension *cpp* is not longer possible, and one solution is to use *hpp* as extension, to show that it is an included *.cpp file.
+Every other extension e.g. *cinclude* would do, but *hpp* seems to be common sense.
+
+# Using the new *.hpp files
+In order to support [compile options](#compile-options--macros-for-this-library) more easily,
+you must use the statement `#include ` instead of `#include ` in your main program (aka *.ino file with setup() and loop()).
+
+In **all other files** you must use the following, to **prevent `multiple definitions` linker errors**:
+
+```c++
+#define USE_IRREMOTE_HPP_AS_PLAIN_INCLUDE
+#include
+```
+
+**Ensure that all macros in your main program are defined before any** `#include `.
+The following macros will definitely be overridden with default values otherwise:
+- `RAW_BUFFER_LENGTH`
+- `IR_SEND_PIN`
+- `SEND_PWM_BY_TIMER`
+
+
+
+# Receiving IR codes
+Check for a **completly received IR frame** with:
+`if (IrReceiver.decode()) {}`
+This also decodes the received data.
+After successful decoding, the IR data is contained in the IRData structure, available as `IrReceiver.decodedIRData`.
+
+## decodedIRData structure
+```c++
+struct IRData {
+ decode_type_t protocol; // UNKNOWN, NEC, SONY, RC5, PULSE_DISTANCE, ...
+ uint16_t address; // Decoded address
+ uint16_t command; // Decoded command
+ uint16_t extra; // Used for Kaseikyo unknown vendor ID. Ticks used for decoding Distance protocol.
+ uint16_t numberOfBits; // Number of bits received for data (address + command + parity) - to determine protocol length if different length are possible.
+ uint8_t flags; // IRDATA_FLAGS_IS_REPEAT, IRDATA_FLAGS_WAS_OVERFLOW etc. See IRDATA_FLAGS_* definitions
+ IRRawDataType decodedRawData; // Up to 32 (64 bit for 32 bit CPU architectures) bit decoded raw data, used for sendRaw functions.
+ uint32_t decodedRawDataArray[RAW_DATA_ARRAY_SIZE]; // 32 bit decoded raw data, to be used for send function.
+ irparams_struct *rawDataPtr; // Pointer of the raw timing data to be decoded. Mainly the data buffer filled by receiving ISR.
+};
+```
+#### Flags
+This is the [list of flags](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRProtocol.h#L88) contained in the flags field.
+Check it with e.g. `if(IrReceiver.decodedIRData.flags & IRDATA_FLAGS_IS_REPEAT)`.
+
+| Flag name | Description |
+|:---|----|
+| IRDATA_FLAGS_IS_REPEAT | The gap between the preceding frame is as smaller than the maximum gap expected for a repeat. !!!We do not check for changed command or address, because it is almost not possible to press 2 different buttons on the remote within around 100 ms!!!
+| IRDATA_FLAGS_IS_AUTO_REPEAT | The current repeat frame is a repeat, that is always sent after a regular frame and cannot be avoided. Only specified for protocols DENON, and LEGO. |
+| IRDATA_FLAGS_PARITY_FAILED | The current (autorepeat) frame violated parity check. |
+| IRDATA_FLAGS_TOGGLE_BIT | Is set if RC5 or RC6 toggle bit is set. |
+| IRDATA_FLAGS_EXTRA_INFO | There is extra info not contained in address and data (e.g. Kaseikyo unknown vendor ID, or in decodedRawDataArray). |
+| IRDATA_FLAGS_WAS_OVERFLOW | irparams.rawlen is set to 0 in this case to avoid endless OverflowFlag. |
+| IRDATA_FLAGS_IS_MSB_FIRST | This value is mainly determined by the (known) protocol. |
+
+#### To access the **RAW data**, use:
+```c++
+auto myRawdata= IrReceiver.decodedIRData.decodedRawData;
+```
+
+The definitions for the `IrReceiver.decodedIRData.flags` are described [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRremoteInt.h#L128-L140).
+
+#### Print all fields:
+```c++
+IrReceiver.printIRResultShort(&Serial);
+```
+
+#### Print the raw timing data received:
+```c++
+IrReceiver.printIRResultRawFormatted(&Serial, true);`
+```
+The raw data depends on the internal state of the Arduino timer in relation to the received signal and might therefore be slightly different each time. (resolution problem). The decoded values are the interpreted ones which are tolerant to such slight differences!
+
+#### Print how to send the received data:
+```c++
+IrReceiver.printIRSendUsage(&Serial);
+```
+
+## Ambiguous protocols
+### NEC, Extended NEC, ONKYO
+The **NEC protocol** is defined as 8 bit address and 8 bit command. But the physical address and data fields are each 16 bit wide.
+The additional 8 bits are used to send the inverted address or command for parity checking.
+The **extended NEC protocol** uses the additional 8 parity bit of address for a 16 bit address, thus disabling the parity check for address.
+The **ONKYO protocol** in turn uses the additional 8 parity bit of address and command for a 16 bit address and command.
+
+The decoder reduces the 16 bit values to 8 bit ones if the parity is correct.
+If the parity is not correct, it assumes no parity error, but takes the values as 16 bit values without parity assuming extended NEC or extended NEC protocol protocol.
+
+But now we have a problem when we want to receive e.g. the **16 bit** address 0x00FF or 0x32CD!
+The decoder interprets this as a NEC 8 bit address 0x00 / 0x32 with correct parity of 0xFF / 0xCD and reduces it to 0x00 / 0x32.
+
+One way to handle this, is to force the library to **always** use the ONKYO protocol interpretation by using `#define DECODE_ONKYO`.
+Another way is to check if `IrReceiver.decodedIRData.protocol` is NEC and not ONKYO and to revert the parity reducing manually.
+
+### NEC, NEC2
+On a long press, the **NEC protocol** does not repeat its frame, it sends a special short repeat frame.
+This enables an easy distinction between long presses and repeated presses and saves a bit of battery energy.
+This behavior is quite unique for NEC and its derived protocols like LG.
+
+So there are of course also remote control systems, which uses the NEC protocol but on a long press just repeat the first frame instead of sending the special short repeat frame. We named this the **NEC2** protocol and it is sent with `sendNEC2()`.
+But be careful, the NEC2 protocol can only be detected by the NEC library decoder **after** the first frame and if you do a long press!
+
+## Unknown protocol
+If your protocol seems not to be supported by this library, you may try the [IRMP library](https://github.com/IRMP-org/IRMP).
+
+
+
+# Sending IR codes
+If you have a device at hand which can generate the IR codes you want to work with (aka IR remote), **it is recommended** to receive the codes with the [ReceiveDemo example](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/ReceiveDemo/ReceiveDemo.ino), which will tell you on the serial output how to send them.
+
+```
+Protocol=LG Address=0x2 Command=0x3434 Raw-Data=0x23434E 28 bits MSB first
+Send with: IrSender.sendLG(0x2, 0x3434, );
+```
+You will discover that **the address is a constant** and the commands sometimes are sensibly grouped.
+If you are uncertain about the numbers of repeats to use for sending, **3** is a good starting point. If this works, you can check lower values afterwards.
+
+If you have enabled `DECODE_DISTANCE_WIDTH`, the code printed by `printIRSendUsage()` **differs between 8 and 32 bit platforms**, so it is best to run the receiving program on the same platform as the sending program.
+
+The codes found in the [irdb database](https://github.com/probonopd/irdb/tree/master/codes) specify a **device**, a **subdevice** and a **function**. Most of the times, *device* and *subdevice* can be taken as upper and lower byte of the **address parameter** and *function* is the **command parameter** for the **new structured functions** with address, command and repeat-count parameters like e.g. `IrSender.sendNEC((device << 8) | subdevice, 0x19, 2)`.
+An **exact mapping** can be found in the [IRP definition files for IR protocols](https://github.com/probonopd/MakeHex/tree/master/protocols). "D" and "S" denotes device and subdevice and "F" denotes the function.
+
+**All sending functions support the sending of repeats** if sensible.
+Repeat frames are sent at a fixed period determined by the protocol. e.g. 110 ms from start to start for NEC.
+Keep in mind, that **there is no delay after the last sent mark**.
+If you handle the sending of repeat frames by your own, you must insert sensible delays before the repeat frames to enable correct decoding.
+
+The old send*Raw() functions for sending like e.g. `IrSender.sendNECRaw(0xE61957A8,2)` are kept for backward compatibility to **(old)** tutorials and unsupported as well as error prone.
+
+## Send pin
+Any pin can be choosen as send pin, because the PWM signal is generated by default with software bit banging, since `SEND_PWM_BY_TIMER` is not active.
+If `IR_SEND_PIN` is specified (as c macro), it reduces program size and improves send timing for AVR. If you want to use a variable to specify send pin e.g. with `setSendPin(uint8_t aSendPinNumber)`, you must disable this `IR_SEND_PIN` macro. Then you can change send pin at any time before sending an IR frame. See also [Compile options / macros for this library](https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library).
+
+### List of public IR code databases
+http://www.harctoolbox.org/IR-resources.html
+
+## Flipper Zero
+[Flipper IRDB Database](https://github.com/Lucaslhm/Flipper-IRDB)
+
+| [Flipper decoding](https://github.com/flipperdevices/flipperzero-firmware/tree/release/lib/infrared/encoder_decoder) | [IRremote decoding](https://github.com/Arduino-IRremote/Arduino-IRremote/tree/master/src) |
+|-|-|
+| Samsung32 | Samsung |
+| NEC | NEC |
+| NECext | ONKYO |
+| [\\\\\\\\\](https://github.com/flipperdevices/flipperzero-firmware/blob/027ea9ea36da137144548295c016d99255af53c3/lib/infrared/encoder_decoder/kaseikyo/infrared_decoder_kaseikyo.c#L26)
and ID is MSB of address.
address: 8A 02 20 00
command: 56 03 00 00
-> **IRremote:**
Address 0x6A8, sendPanasonic (for 02 20) and Command 0x35 | \\\\\\\ |
+
+
+
+
+# Tiny NEC receiver and sender
+For applications only requiring NEC, NEC variants or FAST -see below- protocol, there is a special receiver / sender included,
+which has very **small code size of 500 bytes and does NOT require any timer**.
+
+Check out the [TinyReceiver](https://github.com/Arduino-IRremote/Arduino-IRremote#tinyreceiver--tinysender) and [IRDispatcherDemo](https://github.com/Arduino-IRremote/Arduino-IRremote#irdispatcherdemo) examples.
+Take care to include `TinyIRReceiver.hpp` or `TinyIRSender.hpp` instead of `IRremote.hpp`.
+
+### TinyIRReceiver usage
+```c++
+//#define USE_ONKYO_PROTOCOL // Like NEC, but take the 16 bit address and command each as one 16 bit value and not as 8 bit normal and 8 bit inverted value.
+//#define USE_FAST_PROTOCOL // Use FAST protocol instead of NEC / ONKYO
+#include "TinyIRReceiver.hpp"
+
+void setup() {
+ initPCIInterruptForTinyReceiver(); // Enables the interrupt generation on change of IR input signal
+}
+
+void loop() {
+ if (TinyIRReceiverData.justWritten) {
+ TinyIRReceiverData.justWritten = false;
+ printTinyReceiverResultMinimal(&Serial);
+ }
+}
+```
+
+### TinyIRSender usage
+```c++
+#include "TinyIRSender.hpp"
+
+void setup() {
+ sendNEC(3, 0, 11, 2); // Send address 0 and command 11 on pin 3 with 2 repeats.
+}
+
+void loop() {}
+```
+
+Another tiny receiver and sender **supporting more protocols** can be found [here](https://github.com/LuisMiCa/IRsmallDecoder).
+
+# The FAST protocol
+The FAST protocol is a proprietary modified JVC protocol **without address, with parity and with a shorter header**.
+It is meant to have a quick response to the event which sent the protocol frame on another board.
+FAST takes **21 ms for sending** and is sent at a **50 ms period**.
+It has full 8 bit parity for error detection.
+
+### FAST protocol characteristics:
+- Bit timing is like JVC
+- The header is shorter, 3156 µs vs. 12500 µs
+- No address and 16 bit data, interpreted as 8 bit command and 8 bit inverted command, leading to a fixed protocol length of (6 + (16 * 3) + 1) * 526 = 55 * 526 = 28930 microseconds or 29 ms.
+- Repeats are sent as complete frames but in a 50 ms period / with a 21 ms distance.
+
+### Sending FAST protocol with IRremote
+```c++
+#define IR_SEND_PIN 3
+#include
+
+void setup() {
+ sendFAST(11, 2); // Send command 11 on pin 3 with 2 repeats.
+}
+
+void loop() {}
+```
+
+### Sending FAST protocol with TinyIRSender
+```c++
+#define USE_FAST_PROTOCOL // Use FAST protocol. No address and 16 bit data, interpreted as 8 bit command and 8 bit inverted command
+#include "TinyIRSender.hpp"
+
+void setup() {
+ sendFAST(3, 11, 2); // Send command 11 on pin 3 with 2 repeats.
+}
+
+void loop() {}
+```
+
+
+The FAST protocol can be received by IRremote and TinyIRReceiver.
+
+# FAQ and hints
+
+## Problems with Neopixels, FastLed etc.
+IRremote will not work right when you use **Neopixels** (aka WS2811/WS2812/WS2812B) or other libraries blocking interrupts for a longer time (> 50 µs).
+Whether you use the Adafruit Neopixel lib, or FastLED, interrupts get disabled on many lower end CPUs like the basic Arduinos for longer than 50 µs.
+In turn, this stops the IR interrupt handler from running when it needs to. See also this [video](https://www.youtube.com/watch?v=62-nEJtm070).
+
+One **workaround** is to wait for the IR receiver to be idle before you send the Neopixel data with `if (IrReceiver.isIdle()) { strip.show();}`.
+This **prevents at least breaking a running IR transmission** and -depending of the update rate of the Neopixel- may work quite well.
+There are some other solutions to this on more powerful processors,
+[see this page from Marc MERLIN](http://marc.merlins.org/perso/arduino/post_2017-04-03_Arduino-328P-Uno-Teensy3_1-ESP8266-ESP32-IR-and-Neopixels.html)
+
+## Does not work/compile with another library
+**Another library is only working/compiling** if you deactivate the line `IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);`.
+This is often due to **timer resource conflicts** with the other library. Please see [below](https://github.com/Arduino-IRremote/Arduino-IRremote#timer-and-pin-usage).
+
+## Multiple IR receivers
+IRreceiver consists of one timer triggered function reading the digital IR signal value from one pin every 50 µs.
+So **multiple IR receivers** can only be used by connecting the output pins of several IR receivers together.
+The IR receivers use an NPN transistor as output device with just a 30k resistor to VCC.
+This is almost "open collector" and allows connecting of several output pins to one Arduino input pin.
+But keep in mind, that any weak / disturbed signal from one of the receivers will in turn also disturb a good signal from another one.
+
+## Increase strength of sent output signal
+**The best way to increase the IR power for free** is to use 2 or 3 IR diodes in series. One diode requires 1.2 volt at 20 mA or 1.5 volt at 100 mA so you can supply up to 3 diodes with a 5 volt output.
+To power **2 diodes** with 1.2 V and 20 mA and a 5 V supply, set the resistor to: (5 V - 2.4 V) -> 2.6 V / 20 mA = **130 Ω**.
+For **3 diodes** it requires 1.4 V / 20 mA = **70 Ω**.
+The actual current might be lower since of **loss at the AVR pin**. E.g. 0.3 V at 20 mA.
+If you do not require more current than 20 mA, there is no need to use an external transistor (at least for AVR chips).
+
+On my Arduino Nanos, I always use a 100 Ω series resistor and one IR LED :grinning:.
+
+## Minimal CPU clock frequency
+For receiving, the **minimal CPU clock frequency is 4 MHz**, since the 50 µs timer ISR (Interrupt Service Routine) takes around 12 µs on a 16 MHz ATmega.
+The TinyReceiver, which reqires no polling, runs with 1 MHz.
+For sending, the **default software generated PWM has problems on AVR running with 8 MHz**. The PWM frequency is around 30 instead of 38 kHz and RC6 is not reliable. You can switch to timer PWM generation by `#define SEND_PWM_BY_TIMER`.
+
+## Bang & Olufsen protocol
+The Bang & Olufsen protocol decoder is not enabled by default, i.e if no protocol is enabled explicitly by #define `DECODE_`. It must always be enabled explicitly by `#define DECODE_BEO`.
+This is because it has an **IR transmit frequency of 455 kHz** and therefore requires a different receiver hardware (TSOP7000).
+And because **generating a 455 kHz PWM signal is currently only implemented for `SEND_PWM_BY_TIMER`**, sending only works if `SEND_PWM_BY_TIMER` or `USE_NO_SEND_PWM` is defined.
+For more info, see [ir_BangOlufsen.hpp](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/ir_BangOlufsen.hpp#L44).
+
+# Handling unknown Protocols
+## Disclaimer
+**This library was designed to fit inside MCUs with relatively low levels of resources and was intended to work as a library together with other applications which also require some resources of the MCU to operate.**
+
+For **air conditioners** [see this fork](https://github.com/crankyoldgit/IRremoteESP8266), which supports an impressive set of protocols and a lot of air conditioners.
+
+For **long signals** see the blog entry: ["Recording long Infrared Remote control signals with Arduino"](https://www.analysir.com/blog/2014/03/19/air-conditioners-problems-recording-long-infrared-remote-control-signals-arduino).
+
+
+## Protocol=PULSE_DISTANCE
+If you get something like this:
+```
+PULSE_DISTANCE: HeaderMarkMicros=8900 HeaderSpaceMicros=4450 MarkMicros=550 OneSpaceMicros=1700 ZeroSpaceMicros=600 NumberOfBits=56 0x43D8613C 0x3BC3BC
+```
+then you have a code consisting of **56 bits**, which is probably from an air conditioner remote.
+You can send it with sendPulseDistance().
+```c++
+uint32_t tRawData[] = { 0xB02002, 0xA010 };
+IrSender.sendPulseDistance(38, 3450, 1700, 450, 1250, 450, 400, &tRawData[0], 48, false, 0, 0);
+```
+You can send it with calling sendPulseDistanceWidthData() twice, once for the first 32 bit and next for the remaining 24 bits.
+**The PulseDistance or PulseWidth decoders just decode a timing steam to a bit stream**.
+They can not put any semantics like address, command or checksum on this bitstream, since it is no known protocol.
+But the bitstream is way more readable, than a timing stream. This bitstream is read **LSB first by default**.
+If this does not suit you for further research, you can change it [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/ir_DistanceProtocol.hpp#L48).
+
+## Protocol=UNKNOWN
+If you see something like `Protocol=UNKNOWN Hash=0x13BD886C 35 bits received` as output of e.g. the ReceiveDemo example, you either have a problem with decoding a protocol, or an unsupported protocol.
+
+- If you have an **odd number of bits** received, your receiver circuit probably has problems. Maybe because the IR signal is too weak.
+- If you see timings like `+ 600,- 600 + 550,- 150 + 200,- 100 + 750,- 550` then one 450 µs space was split into two 150 and 100 µs spaces with a spike / error signal of 200 µs between. Maybe because of a defective receiver or a weak signal in conjunction with another light emitting source nearby.
+- If you see timings like `+ 500,- 550 + 450,- 550 + 500,- 500 + 500,-1550`, then marks are generally shorter than spaces and therefore `MARK_EXCESS_MICROS` (specified in your ino file) should be **negative** to compensate for this at decoding.
+- If you see `Protocol=UNKNOWN Hash=0x0 1 bits received` it may be that the space after the initial mark is longer than [`RECORD_GAP_MICROS`](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRremote.h#L124).
+ This was observed for some LG air conditioner protocols. Try again with a line e.g. `#define RECORD_GAP_MICROS 12000` before the line `#include ` in your .ino file.
+- To see more info supporting you to find the reason for your UNKNOWN protocol, you must enable the line `//#define DEBUG` in IRremoteInt.h.
+
+## How to deal with protocols not supported by IRremote
+If you do not know which protocol your IR transmitter uses, you have several choices.
+- Use the [IRreceiveDump example](examples/ReceiveDump) to dump out the IR timing.
+ You can then reproduce/send this timing with the [SendRawDemo example](examples/SendRawDemo).
+ For **long codes** with more than 48 bits like from air conditioners, you can **change the length of the input buffer** in [IRremote.h](src/IRremoteInt.h#L36).
+- The [IRMP AllProtocol example](https://github.com/IRMP-org/IRMP#allprotocol-example) prints the protocol and data for one of the **40 supported protocols**.
+ The same library can be used to send this codes.
+- If you have a bigger Arduino board at hand (> 100 kByte program memory) you can try the
+ [IRremoteDecode example](https://github.com/bengtmartensson/Arduino-DecodeIR/blob/master/examples/IRremoteDecode/IRremoteDecode.ino) of the Arduino library [DecodeIR](https://github.com/bengtmartensson/Arduino-DecodeIR).
+- Use [IrScrutinizer](http://www.harctoolbox.org/IrScrutinizer.html).
+ It can automatically generate a send sketch for your protocol by exporting as "Arduino Raw". It supports IRremote,
+ the old [IRLib](https://github.com/cyborg5/IRLib) and [Infrared4Arduino](https://github.com/bengtmartensson/Infrared4Arduino).
+
+
+
+# Examples for this library
+The examples are available at File > Examples > Examples from Custom Libraries / IRremote.
+ In order to fit the examples to the 8K flash of ATtiny85 and ATtiny88, the [Arduino library ATtinySerialOut](https://github.com/ArminJo/ATtinySerialOut) is required for this CPU's.
+
+#### SimpleReceiver + SimpleSender
+The **[SimpleReceiver](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SimpleReceiver/SimpleReceiver.ino)** and **[SimpleSender](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SimpleSender/SimpleSender.ino)** examples are a good starting point.
+A simple example can be tested online with [WOKWI](https://wokwi.com/projects/338611596994544210).
+
+#### TinyReceiver + TinySender
+If **code size** or **timer usage** matters, look at these examples.
+The **[TinyReceiver](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/TinyReceiver/TinyReceiver.ino)** example uses the **TinyIRReceiver** library
+which can **only receive NEC, Extended NEC, ONKYO and FAST protocols, but does not require any timer**.
+They use pin change interrupt for on the fly decoding, which is the reason for the restricted protocol choice.
+TinyReceiver can be tested online with [WOKWI](https://wokwi.com/arduino/projects/339264565653013075).
+
+The **[TinySender](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/TinySender/TinySender.ino)** example uses the **TinyIRSender** library which can **only send NEC, ONKYO and FAST protocols**.
+It sends NEC protocol codes in standard format with 8 bit address and 8 bit command as in SimpleSender example. It has options to send using Extended NEC, ONKYO and FAST protocols.
+Saves 780 bytes program memory and 26 bytes RAM compared to SimpleSender, which does the same, but uses the IRRemote library (and is therefore much more flexible).
+
+#### SmallReceiver
+If the protocol is not NEC and code size matters, look at this [example](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SmallReceiver/SmallReceiver.ino).
+
+#### ReceiveDemo + AllProtocolsOnLCD
+[ReceiveDemo](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/ReceiveDemo/ReceiveDemo.ino) receives all protocols and **generates a beep with the Arduino tone() function** on each packet received.
+Long press of one IR button (receiving of multiple repeats for one command) is detected.
+[AllProtocolsOnLCD](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/AllProtocolsOnLCD/AllProtocolsOnLCD.ino) additionally **displays the short result on a 1602 LCD**. The LCD can be connected parallel or serial (I2C).
+By connecting debug pin to ground, you can force printing of the raw values for each frame. The pin number of the debug pin is printed during setup, because it depends on board and LCD connection type.
+This example also serves as an **example how to use IRremote and tone() together**.
+
+#### ReceiveDump
+Receives all protocols and dumps the received signal in different flavors including Pronto format. Since the printing takes much time, repeat signals may be skipped or interpreted as UNKNOWN.
+
+#### SendDemo
+Sends all available protocols at least once.
+
+#### SendAndReceive
+Demonstrates **receiving while sending**.
+
+#### ReceiveAndSend
+Record and **play back last received IR signal** at button press. IR frames of known protocols are sent by the approriate protocol encoder. `UNKNOWN` protocol frames are stored as raw data and sent with `sendRaw()`.
+
+#### ReceiveAndSendDistanceWidth
+Try to decode each IR frame with the *universal* **DistanceWidth decoder**, store the data and send it on button press with `sendPulseDistanceWidthFromArray()`.
+Storing data for distance width protocol requires 17 bytes.
+The ReceiveAndSend example requires 16 bytes for known protocol data and 37 bytes for raw data of e.g.NEC protocol.
+
+#### ReceiveOneAndSendMultiple
+Serves as a IR **remote macro expander**. Receives Samsung32 protocol and on receiving a specified input frame, it sends multiple Samsung32 frames with appropriate delays in between.
+This serves as a **Netflix-key emulation** for my old Samsung H5273 TV.
+
+#### IRDispatcherDemo
+Framework for **calling different functions of your program** for different IR codes.
+
+#### IRrelay
+**Control a relay** (connected to an output pin) with your remote.
+
+#### IRremoteExtensionTest
+[Example](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/IRremoteExtensionTest/IRremoteExtensionTest.ino) for a user defined class, which itself uses the IRrecv class from IRremote.
+
+#### SendLGAirConditionerDemo
+[Example](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/SendLGAirConditionerDemo/SendLGAirConditionerDemo.ino) for sending LG air conditioner IR codes controlled by Serial input.
+By just using the function `bool Aircondition_LG::sendCommandAndParameter(char aCommand, int aParameter)` you can control the air conditioner by any other command source.
+The file *acLG.h* contains the command documentation of the LG air conditioner IR protocol. Based on reverse engineering of the LG AKB73315611 remote.
+
+IReceiverTimingAnalysis can be tested online with [WOKWI](https://wokwi.com/projects/299033930562011656)
+Click on the receiver while simulation is running to specify individual IR codes.
+
+#### ReceiverTimingAnalysis
+This [example](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/examples/ReceiverTimingAnalysis/ReceiverTimingAnalysis.ino) analyzes the signal delivered by your IR receiver module.
+Values can be used to determine the stability of the received signal as well as a hint for determining the protocol.
+It also computes the `MARK_EXCESS_MICROS` value, which is the extension of the mark (pulse) duration introduced by the IR receiver module.
+It can be tested online with [WOKWI](https://wokwi.com/arduino/projects/299033930562011656).
+Click on the receiver while simulation is running to specify individual NEC IR codes.
+
+#### UnitTest
+ReceiveDemo + SendDemo in one program. Demonstrates **receiving while sending**.
+Here you see the delay of the receiver output (blue) from the IR diode input (yellow).
+
+
+# WOKWI online examples
+- [Simple receiver](https://wokwi.com/projects/338611596994544210)
+- [Simple toggle by IR key 5](https://wokwi.com/projects/338611596994544210)
+- [TinyReceiver](https://wokwi.com/arduino/projects/339264565653013075)
+- [ReceiverTimingAnalysis](https://wokwi.com/projects/299033930562011656)
+- [Receiver with LCD output and switch statement](https://wokwi.com/projects/298934082074575369)
+
+
+
+# Issues and discussions
+- Do not open an issue without first testing some of the examples!
+- If you have a problem, please post the MCVE (Minimal Complete Verifiable Example) showing this problem. My experience is, that most of the times you will find the problem while creating this MCVE :smile:.
+- [Use code blocks](https://github.com/adam-p/markdown-here/wiki/Markdown-Cheatsheet#code); **it helps us help you when we can read your code!**
+
+
+
+# Compile options / macros for this library
+To customize the library to different requirements, there are some compile options / macros available.
+These macros must be defined in your program **before** the line `#include ` to take effect.
+Modify them by enabling / disabling them, or change the values if applicable.
+
+| Name | Default value | Description |
+|-|-:|-|
+| `RAW_BUFFER_LENGTH` | 100 | Buffer size of raw input buffer. Must be even! 100 is sufficient for *regular* protocols of up to 48 bits, but for most air conditioner protocols a value of up to 750 is required. Use the ReceiveDump example to find smallest value for your requirements. |
+| `EXCLUDE_UNIVERSAL_PROTOCOLS` | disabled | Excludes the universal decoder for pulse distance protocols and decodeHash (special decoder for all protocols) from `decode()`. Saves up to 1000 bytes program memory. |
+| `DECODE_` | all | Selection of individual protocol(s) to be decoded. You can specify multiple protocols. See [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRremote.hpp#L98-L121) |
+| `DECODE_STRICT_CHECKS` | disabled | Check for additional required characteristics of protocol timing like length of mark for a constant mark protocol, where space length determines the bit value. Requires up to 194 additional bytes of program memory. |
+| `IR_REMOTE_DISABLE_RECEIVE_COMPLETE_CALLBACK` | disabled | Saves up to 60 bytes of program memory and 2 bytes RAM. |
+| `MARK_EXCESS_MICROS` | 20 | MARK_EXCESS_MICROS is subtracted from all marks and added to all spaces before decoding, to compensate for the signal forming of different IR receiver modules. |
+| `RECORD_GAP_MICROS` | 5000 | Minimum gap between IR transmissions, to detect the end of a protocol.
Must be greater than any space of a protocol e.g. the NEC header space of 4500 µs.
Must be smaller than any gap between a command and a repeat; e.g. the retransmission gap for Sony is around 24 ms.
Keep in mind, that this is the delay between the end of the received command and the start of decoding. |
+| `IR_INPUT_IS_ACTIVE_HIGH` | disabled | Enable it if you use a RF receiver, which has an active HIGH output signal. |
+| `IR_SEND_PIN` | disabled | If specified, it reduces program size and improves send timing for AVR. If you want to use a variable to specify send pin e.g. with `setSendPin(uint8_t aSendPinNumber)`, you must not use / disable this macro in your source. |
+| `SEND_PWM_BY_TIMER` | disabled | Disables carrier PWM generation in software and use hardware PWM (by timer). Has the advantage of more exact PWM generation, especially the duty cycle (which is not very relevant for most IR receiver circuits), and the disadvantage of using a hardware timer, which in turn is not available for other libraries and to fix the send pin (but not the receive pin) at the [dedicated timer output pin(s)](https://github.com/Arduino-IRremote/Arduino-IRremote#timer-and-pin-usage). Is enabled for ESP32 and RP2040 in all examples, since they support PWM gereration for each pin without using a shared resource (timer). |
+| `USE_NO_SEND_PWM` | disabled | Uses no carrier PWM, just simulate an **active low** receiver signal. Used for transferring signal by cable instead of IR. Overrides `SEND_PWM_BY_TIMER` definition. |
+| `IR_SEND_DUTY_CYCLE_PERCENT` | 30 | Duty cycle of IR send signal. |
+| `USE_OPEN_DRAIN_OUTPUT_FOR_SEND_PIN` | disabled | Uses or simulates open drain output mode at send pin. **Attention, active state of open drain is LOW**, so connect the send LED between positive supply and send pin! |
+| `DISABLE_CODE_FOR_RECEIVER` | disabled | Saves up to 450 bytes program memory and 269 bytes RAM if receiving functionality is not required. |
+| `EXCLUDE_EXOTIC_PROTOCOLS` | disabled | Excludes BANG_OLUFSEN, BOSEWAVE, WHYNTER, FAST and LEGO_PF from `decode()` and from sending with `IrSender.write()`. Saves up to 650 bytes program memory. |
+| `FEEDBACK_LED_IS_ACTIVE_LOW` | disabled | Required on some boards (like my BluePill and my ESP8266 board), where the feedback LED is active low. |
+| `NO_LED_FEEDBACK_CODE` | disabled | Disables the LED feedback code for send and receive. Saves around 100 bytes program memory for receiving, around 500 bytes for sending and halving the receiver ISR (Interrupt Service Routine) processing time. |
+| `MICROS_PER_TICK` | 50 | Resolution of the raw input buffer data. Corresponds to 2 pulses of each 26.3 µs at 38 kHz. |
+| `TOLERANCE_FOR_DECODERS_MARK_OR_SPACE_MATCHING` | 25 | Relative tolerance (in percent) for matchTicks(), matchMark() and matchSpace() functions used for protocol decoding. |
+| `DEBUG` | disabled | Enables lots of lovely debug output. |
+| `IR_USE_AVR_TIMER*` | | Selection of timer to be used for generating IR receiving sample interval. |
+
+These next macros for **TinyIRReceiver** must be defined in your program before the line `#include ` to take effect.
+| Name | Default value | Description |
+|-|-:|-|
+| `IR_RECEIVE_PIN` | 2 | The pin number for TinyIRReceiver IR input, which gets compiled in. |
+| `IR_FEEDBACK_LED_PIN` | `LED_BUILTIN` | The pin number for TinyIRReceiver feedback LED, which gets compiled in. |
+| `NO_LED_FEEDBACK_CODE` | disabled | Disables the feedback LED function. Saves 14 bytes program memory. |
+| `DISABLE_PARITY_CHECKS` | disabled | Disables the addres and command parity checks. Saves 48 bytes program memory. |
+| `USE_EXTENDED_NEC_PROTOCOL` | disabled | Like NEC, but take the 16 bit address as one 16 bit value and not as 8 bit normal and 8 bit inverted value. |
+| `USE_ONKYO_PROTOCOL` | disabled | Like NEC, but take the 16 bit address and command each as one 16 bit value and not as 8 bit normal and 8 bit inverted value. |
+| `USE_FAST_PROTOCOL` | disabled | Use FAST protocol (no address and 16 bit data, interpreted as 8 bit command and 8 bit inverted command) instead of NEC. |
+| `ENABLE_NEC2_REPEATS` | disabled | Instead of sending / receiving the NEC special repeat code, send / receive the original frame for repeat. |
+| `USE_CALLBACK_FOR_TINY_RECEIVER` | disabled | Call the fixed function `void handleReceivedTinyIRData()` each time a frame or repeat is received. |
+
+The next macro for **IRCommandDispatcher** must be defined in your program before the line `#include ` to take effect.
+| `USE_TINY_IR_RECEIVER` | disabled | Use [TinyReceiver](https://github.com/Arduino-IRremote/Arduino-IRremote#tinyreceiver--tinysender) for receiving IR codes. |
+| `IR_COMMAND_HAS_MORE_THAN_8_BIT` | disabled | Enables mapping and dispatching of IR commands consisting of more than 8 bits. Saves up to 160 bytes program memory and 4 bytes RAM + 1 byte RAM per mapping entry. |
+| `BUZZER_PIN` | | If `USE_TINY_IR_RECEIVER` is enabled, the pin to be used for the optional 50 ms buzzer feedback before executing a command. Other IR libraries than Tiny are not compatible with tone() command. |
+
+### Changing include (*.h) files with Arduino IDE
+First, use *Sketch > Show Sketch Folder (Ctrl+K)*.
+If you have not yet saved the example as your own sketch, then you are instantly in the right library folder.
+Otherwise you have to navigate to the parallel `libraries` folder and select the library you want to access.
+In both cases the library source and include files are located in the libraries `src` directory.
+The modification must be renewed for each new library version!
+
+### Modifying compile options / macros with PlatformIO
+If you are using PlatformIO, you can define the macros in the *[platformio.ini](https://docs.platformio.org/en/latest/projectconf/section_env_build.html)* file with `build_flags = -D MACRO_NAME` or `build_flags = -D MACRO_NAME=macroValue`.
+
+### Modifying compile options / macros with Sloeber IDE
+If you are using [Sloeber](https://eclipse.baeyens.it) as your IDE, you can easily define global symbols with *Properties > Arduino > CompileOptions*.
+
+
+
+
+# Supported Boards
+**Issues and discussions with the content "Is it possible to use this library with the ATTinyXYZ? / board XYZ" without any reasonable explanations will be immediately closed without further notice.**
+
+Digispark boards are only tested with [ATTinyCore](https://github.com/SpenceKonde/ATTinyCore) using `New Style` pin mapping for the Digispark Pro board.
+ATtiny boards are only tested with [ATTinyCore](https://github.com/SpenceKonde/ATTinyCore#supported-devices) or [megaTinyCore](https://github.com/SpenceKonde/megaTinyCore).
+
+- Arduino Uno / Mega / Leonardo / Duemilanove / Diecimila / LilyPad / Mini / Fio / Nano etc.
+- Arduino Uno R4, but not yet tested, because of lack of a R4 board. **Sending does not work** on the `arduino:renesas_uno:unor4wifi`.
+- Teensy 1.0 / 1.0++ / 2.0 / 2++ / 3.0 / 3.1 / 3.2 / Teensy-LC - but [limited support](https://forum.pjrc.com/threads/65912-Enable-Continuous-Integration-with-arduino-cli-for-3-party-libraries); Credits: PaulStoffregen (Teensy Team)
+- Sanguino
+- ATmega8, 48, 88, 168, 328
+- ATmega8535, 16, 32, 164, 324, 644, 1284,
+- ATmega64, 128
+- ATmega4809 (Nano every)
+- ATtiny3217 (Tiny Core 32 Dev Board)
+- ATtiny84, 85, 167 (Digispark + Digispark Pro)
+- SAMD21 (Zero, MKR*, **but not SAMD51 and not DUE, the latter is SAM architecture**)
+- ESP8266
+- ESP32 (ESP32-C3 since board package 2.0.2 from Espressif) **not for ESP32 core version > 3.0.0**
+- Sparkfun Pro Micro
+- Nano Every, Uno WiFi Rev2, nRF5 BBC MicroBit, Nano33_BLE
+- BluePill with STM32
+- RP2040 based boards (Raspberry Pi Pico, Nano RP2040 Connect etc.)
+
+For ESP8266/ESP32, [this library](https://github.com/crankyoldgit/IRremoteESP8266) supports an [impressive set of protocols and a lot of air conditioners](https://github.com/crankyoldgit/IRremoteESP8266/blob/master/SupportedProtocols.md)
+
+We are open to suggestions for adding support to new boards, however we highly recommend you contact your supplier first and ask them to provide support from their side.
+If you can provide **examples of using a periodic timer for interrupts** for the new board, and the board name for selection in the Arduino IDE, then you have way better chances to get your board supported by IRremote.
+
+
+
+# Timer and pin usage
+The **receiver sample interval of 50 µs is generated by a timer**. On many boards this must be a hardware timer. On some boards where a software timer is available, the software timer is used.
+
+Every pin can be used for receiving.
+If software PWM is selected, which is default, every pin can also be used for sending. Sending with software PWM does not require a timer!
+
+The TinyReceiver example uses the **TinyReceiver** library, which can **only receive NEC codes, but does not require any timer** and runs even on a 1 MHz ATtiny85.
+
+The code for the timer and the **timer selection** is located in [private/IRTimer.hpp](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/private/IRTimer.hpp). The selected timer can be adjusted here.
+
+**Be aware that the hardware timer used for receiving should not be used for analogWrite()!**.
+
+| Board/CPU | Receive
& send PWM Timer
Default timer is **bold** | Hardware-Send-PWM Pin | analogWrite()
pins occupied by timer |
+|-|-|-|-|
+| [ATtiny84](https://github.com/SpenceKonde/ATTinyCore/blob/v2.0.0-devThis-is-the-head-submit-PRs-against-this/avr/extras/ATtiny_x4.md) | **1** | **6** | |
+| [ATtiny85 > 4 MHz](https://github.com/SpenceKonde/ATTinyCore/blob/v2.0.0-devThis-is-the-head-submit-PRs-against-this/avr/extras/ATtiny_x5.md) | **0**, 1 | **0**, 4 | **0**, 1 & 4 |
+| [ATtiny88 > 4 MHz](https://github.com/SpenceKonde/ATTinyCore/blob/v2.0.0-devThis-is-the-head-submit-PRs-against-this/avr/extras/ATtiny_x8.md) | **1** | **PB1 / 8** | **PB1 / 8 & PB2 / 9** |
+| [ATtiny167 > 4 MHz](https://github.com/SpenceKonde/ATTinyCore/blob/v2.0.0-devThis-is-the-head-submit-PRs-against-this/avr/extras/ATtiny_x7.md) | **1** | **9**, 8 - 15 | **8 - 15** |
+| [ATtiny1604](https://github.com/SpenceKonde/megaTinyCore/blob/master/megaavr/extras/ATtiny_x04.md) | **TCB0** | **PA05** |
+| [ATtiny1614, ATtiny816](https://github.com/SpenceKonde/megaTinyCore/blob/master/megaavr/extras/ATtiny_x14.md) | **TCA0** | **PA3** |
+| [ATtiny3217](https://github.com/SpenceKonde/megaTinyCore/blob/master/megaavr/extras/ATtiny_x17.md) | **TCA0**, TCD | % |
+| [ATmega8](https://github.com/MCUdude/MiniCore#supported-microcontrollers) | **1** | **9** |
+| [ATmega1284](https://github.com/MCUdude/MightyCore#supported-microcontrollers) | 1, **2**, 3 | 13, 14, 6 |
+| [ATmega164, ATmega324, ATmega644](https://github.com/MCUdude/MightyCore#supported-microcontrollers) | 1, **2** | 13, **14** |
+| [ATmega8535 ATmega16, ATmega32](https://github.com/MCUdude/MightyCore#supported-microcontrollers) | **1** | **13** |
+| [ATmega64, ATmega128, ATmega1281, ATmega2561](https://github.com/MCUdude/MegaCore#supported-microcontrollers) | **1** | **13** |
+| [ATmega8515, ATmega162](https://github.com/MCUdude/MajorCore#pinout ) | **1** | **13** |
+| ATmega168, **ATmega328** | 1, **2** | 9, **3** | 9 & 10, **3 & 11** |
+| ATmega1280, **ATmega2560** | 1, **2**, 3, 4, 5 | 5, 6, **9**, 11, 46 | 5, 6, **9**, 11, 46 |
+| ATmega4809 | **TCB0** | **A4** | |
+| Leonardo (Atmega32u4) | 1, 3, **4_HS** | 5, **9**, 13 | 5, **9**, 13 |
+| Zero (SAMD) | **TC3** | \*, **9** | |
+| [ESP32](http://esp32.net/) | **Ledc chan. 0** | All pins | |
+| [Sparkfun Pro Micro](https://www.sparkfun.com/products/12640) | 1, **3** | **5**, 9 | |
+| [Teensy 1.0](https://www.pjrc.com/teensy/pinout.html) | **1** | **17** | 15, 18 |
+| [Teensy 2.0](https://www.pjrc.com/teensy/pinout.html) | 1, 3, **4_HS** | 9, **10**, 14 | 12 |
+| [Teensy++ 1.0 / 2.0](https://www.pjrc.com/teensy/pinout.html) | 1, **2**, 3 | **1**, 16, 25 | 0 |
+| [Teensy-LC](https://www.pjrc.com/teensy/pinout.html) | **TPM1** | **16** | 17 |
+| [Teensy 3.0 - 3.6](https://www.pjrc.com/teensy/pinout.html) | **CMT** | **5** | |
+| [Teensy 4.0 - 4.1](https://www.pjrc.com/teensy/pinout.html) | **FlexPWM1.3** | **8** | 7, 25 |
+| [BluePill / STM32F103C8T6](https://github.com/stm32duino/Arduino_Core_STM32) | **3** | % | **PA6 & PA7 & PB0 & PB1** |
+| [BluePill / STM32F103C8T6](https://stm32-base.org/boards/STM32F103C8T6-Blue-Pill) | **TIM4** | % | **PB6 & PB7 & PB8 & PB9** |
+| [RP2040 / Pi Pico](https://github.com/earlephilhower/arduino-pico) | [default alarm pool](https://raspberrypi.github.io/pico-sdk-doxygen/group__repeating__timer.html) | All pins | No pin |
+| [RP2040 / Mbed based](https://github.com/arduino/ArduinoCore-mbed) | Mbed Ticker | All pins | No pin |
+
+### No timer required for sending
+The **send PWM signal** is by default generated by software. **Therefore every pin can be used for sending**.
+The PWM pulse length is guaranteed to be constant by using `delayMicroseconds()`.
+Take care not to generate interrupts during sending with software generated PWM, otherwise you will get jitter in the generated PWM.
+E.g. wait for a former `Serial.print()` statement to be finished by `Serial.flush()`.
+Since the Arduino `micros()` function has a resolution of 4 µs at 16 MHz, we always see a small jitter in the signal, which seems to be OK for the receivers.
+
+| Software generated PWM showing small jitter because of the limited resolution of 4 µs of the Arduino core `micros()` function for an ATmega328 | Detail (ATmega328 generated) showing 30% duty cycle |
+|-|-|
+|  |  |
+
+## Incompatibilities to other libraries and Arduino commands like tone() and analogWrite()
+If you use a library which requires the same timer as IRremote, you have a problem, since **the timer resource cannot be shared simultaneously** by both libraries.
+
+### Change timer
+The best approach is to change the timer used for IRremote, which can be accomplished by specifying the timer before `#include `.
+The timer specifications available for your board can be found in [private/IRTimer.hpp](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/private/IRTimer.hpp).
+
+```c++
+// Arduino Mega
+#elif defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
+# if !defined(IR_USE_AVR_TIMER1) && !defined(IR_USE_AVR_TIMER2) && !defined(IR_USE_AVR_TIMER3) && !defined(IR_USE_AVR_TIMER4) && !defined(IR_USE_AVR_TIMER5)
+//#define IR_USE_AVR_TIMER1 // send pin = pin 11
+#define IR_USE_AVR_TIMER2 // send pin = pin 9
+//#define IR_USE_AVR_TIMER3 // send pin = pin 5
+//#define IR_USE_AVR_TIMER4 // send pin = pin 6
+//#define IR_USE_AVR_TIMER5 // send pin = pin 46
+# endif
+```
+Here you see the Arduino Mega board and the available specifications are `IR_USE_AVR_TIMER[1,2,3,4,5]`.
+You **just have to include a line** e.g. `#define IR_USE_AVR_TIMER3` before `#include ` to enable timer 3.
+
+But be aware that the new timer in turn might be incompatible with other libraries or commands.
+For other boards/platforms you must look for the appropriate section guarded by e.g. `#elif defined(ESP32)`.
+
+### Stop and start timer
+Another approach can be to share the timer **sequentially** if their functionality is used only for a short period of time like for the **Arduino tone() command**.
+An example can be seen [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/21b5747a58e9d47c9e3f1beb056d58c875a92b47/examples/ReceiveDemo/ReceiveDemo.ino#L159-L169), where the timer settings for IR receive are restored after the tone has stopped.
+For this we must call `IrReceiver.start()` or better `IrReceiver.start(microsecondsOfToneDuration)`.
+This only works since each call to` tone()` completely initializes the timer 2 used by the `tone()` command.
+
+## Hardware-PWM signal generation for sending
+If you define `SEND_PWM_BY_TIMER`, the send PWM signal is forced to be generated by a hardware timer on most platforms.
+By default, the same timer as for the receiver is used.
+Since each hardware timer has its dedicated output pin(s), you must change timer or timer sub-specifications to change PWM output pin. See [private/IRTimer.hpp](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/private/IRTimer.hpp)
+**Exeptions** are currently [ESP32, ARDUINO_ARCH_RP2040, PARTICLE and ARDUINO_ARCH_MBED](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/39bdf8d7bf5b90dc221f8ae9fb3efed9f0a8a1db/examples/SimpleSender/PinDefinitionsAndMore.h#L273), where **PWM generation does not require a timer**.
+
+## Why do we use 30% duty cycle for sending
+We [do it](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRSend.hpp#L1192) according to the statement in the [Vishay datasheet](https://www.vishay.com/docs/80069/circuit.pdf):
+- Carrier duty cycle 50 %, peak current of emitter IF = 200 mA, the resulting transmission distance is 25 m.
+- Carrier duty cycle 10 %, peak current of emitter IF = 800 mA, the resulting transmission distance is 29 m. - Factor 1.16
+The reason is, that it is not the pure energy of the fundamental which is responsible for the receiver to detect a signal.
+Due to automatic gain control and other bias effects, high intensity of the 38 kHz pulse counts more than medium intensity (e.g. 50% duty cycle) at the same total energy.
+
+
+
+# How we decode signals
+The IR signal is sampled at a **50 µs interval**. For a constant 525 µs pulse or pause we therefore get 10 or 11 samples, each with 50% probability.
+And believe me, if you send a 525 µs signal, your receiver will output something between around 400 and 700 µs!
+Therefore **we decode by default with a +/- 25% margin** using the formulas [here](https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/src/IRremoteInt.h#L376-L399).
+E.g. for the NEC protocol with its 560 µs unit length, we have TICKS_LOW = 8.358 and TICKS_HIGH = 15.0. This means, we accept any value between 8 ticks / 400 µs and 15 ticks / 750 µs (inclusive) as a mark or as a zero space. For a one space we have TICKS_LOW = 25.07 and TICKS_HIGH = 45.0.
+And since the receivers generated marks are longer or shorter than the spaces,
+we have introduced the [`MARK_EXCESS_MICROS`](https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library) macro
+to compensate for this receiver (and signal strength as well as ambient light dependent :disappointed: ) specific deviation.
+Welcome to the world of **real world signal processing**.
+
+
+
+# NEC encoding diagrams
+Created with sigrok PulseView with IR_NEC decoder by DjordjeMandic.
+8 bit address NEC code
+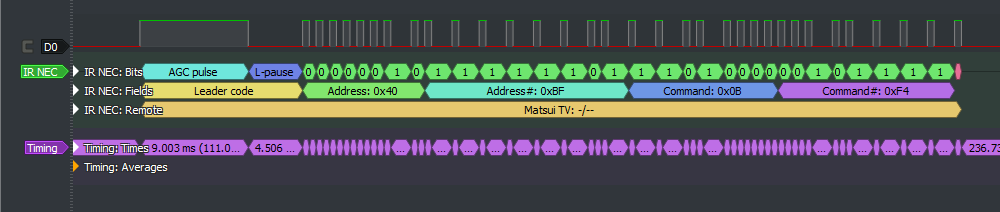
+16 bit address NEC code
+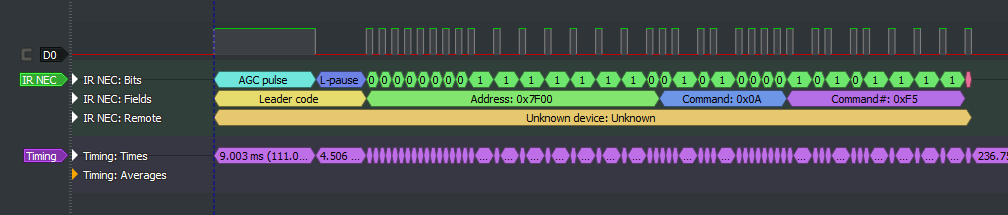
+
+
+
+# Quick comparison of 5 Arduino IR receiving libraries
+**This is a short comparison and may not be complete or correct.**
+
+I created this comparison matrix for [myself](https://github.com/ArminJo) in order to choose a small IR lib for my project and to have a quick overview, when to choose which library.
+It is dated from **24.06.2022** and updated 10/2023. If you have complains about the data or request for extensions, please send a PM or open a discussion.
+
+[Here](https://github.com/crankyoldgit/IRremoteESP8266) you find an **ESP8266/ESP32** version of IRremote with an **[impressive list of supported protocols](https://github.com/crankyoldgit/IRremoteESP8266/blob/master/SupportedProtocols.md)**.
+
+| Subject | [IRMP](https://github.com/IRMP-org/IRMP) | [IRLremote](https://github.com/NicoHood/IRLremote) | [IRLib2](https://github.com/cyborg5/IRLib2)
**mostly unmaintained** | [IRremote](https://github.com/Arduino-IRremote/Arduino-IRremote) | [TinyIR](https://github.com/Arduino-IRremote/Arduino-IRremote/tree/master/examples/TinyReceiver/TinyReceiver.ino) | [IRsmallDecoder](https://github.com/LuisMiCa/IRsmallDecoder)
+|-|-|-|-|-|-|-|
+| Number of protocols | **50** | Nec + Panasonic + Hash \* | 12 + Hash \* | 17 + PulseDistance + Hash \* | NEC + FAST | NEC + RC5 + Sony + Samsung |
+| Timing method receive | Timer2 or interrupt for pin 2 or 3 | **Interrupt** | Timer2 or interrupt for pin 2 or 3 | Timer2 | **Interrupt** | **Interrupt** |
+| Timing method send | PWM and timing with Timer2 interrupts | Timer2 interrupts | Timer2 and blocking wait | PWM with Timer2 and/or blocking wait with delay
Microseconds() | blocking wait with delay
Microseconds() | % |
+| Send pins| All | All | All ? | Timer dependent | All | % |
+| Decode method | OnTheFly | OnTheFly | RAM | RAM | OnTheFly | OnTheFly |
+| Encode method | OnTheFly | OnTheFly | OnTheFly | OnTheFly or RAM | OnTheFly | % |
+| Callback support | x | % | % | x | x | % |
+| Repeat handling | Receive + Send (partially) | % | ? | Receive + Send | Receive + Send | Receive |
+| LED feedback | x | % | x | x | Receive | % |
+| FLASH usage (simple NEC example with 5 prints) | 1820
(4300 for 15 main / 8000 for all 40 protocols)
(+200 for callback)
(+80 for interrupt at pin 2+3)| 1270
(1400 for pin 2+3) | 4830 | 1770 | **900** | ?1100? |
+| RAM usage | 52
(73 / 100 for 15 (main) / 40 protocols) | 62 | 334 | 227 | **19** | 29 |
+| Supported platforms | **avr, megaavr, attiny, Digispark (Pro), esp8266, ESP32, STM32, SAMD 21, Apollo3
(plus arm and pic for non Arduino IDE)** | avr, esp8266 | avr, SAMD 21, SAMD 51 | avr, attiny, [esp8266](https://github.com/crankyoldgit/IRremoteESP8266), esp32, SAM, SAMD | **All platforms with attach
Interrupt()** | **All platforms with attach
Interrupt()** |
+| Last library update | 5/2023 | 4/2018 | 11/2022 | 9/2023 | 5/2023 | 2/2022 |
+| Remarks | Decodes 40 protocols concurrently.
39 Protocols to send.
Work in progress. | Only one protocol at a time. | Consists of 5 libraries. **Project containing bugs - 63 issues, 10 pull requests.* | Universal decoder and encoder.
Supports **Pronto** codes and sending of raw timing values. | Requires no timer. | Requires no timer. |
+
+\* The Hash protocol gives you a hash as code, which may be sufficient to distinguish your keys on the remote, but may not work with some protocols like Mitsubishi
+
+
+
+# Useful links
+- [List of public IR code databases](http://www.harctoolbox.org/IR-resources.html)
+- [LIRC database](http://lirc-remotes.sourceforge.net/remotes-table.html)
+- [IRMP list of IR protocols](https://www.mikrocontroller.net/articles/IRMP_-_english#IR_Protocols)
+- [IRDB database for IR codes](https://github.com/probonopd/irdb/tree/master/codes)
+- [IRP definition files for IR protocols](https://github.com/probonopd/MakeHex/tree/master/protocols)
+- [IR Remote Control Theory and some protocols (upper right hamburger icon)](https://www.sbprojects.net/knowledge/ir/)
+- [Interpreting Decoded IR Signals (v2.45)](http://www.hifi-remote.com/johnsfine/DecodeIR.html)
+- ["Recording long Infrared Remote control signals with Arduino"](https://www.analysir.com/blog/2014/03/19/air-conditioners-problems-recording-long-infrared-remote-control-signals-arduino)
+- The original blog post of Ken Shirriff [A Multi-Protocol Infrared Remote Library for the Arduino](http://www.arcfn.com/2009/08/multi-protocol-infrared-remote-library.html)
+- [Vishay datasheet](https://www.vishay.com/docs/80069/circuit.pdf)
+
+# License
+Up to the version 2.7.0, the License is GPLv2.
+From the version 2.8.0, the license is the MIT license.
+
+# Copyright
+Initially coded 2009 Ken Shirriff http://www.righto.com
+Copyright (c) 2016-2017 Rafi Khan
+Copyright (c) 2020-2023 [Armin Joachimsmeyer](https://github.com/ArminJo)
diff --git a/libraries/_07_m_Sensor_IR_Remote/changelog.md b/libraries/_07_m_Sensor_IR_Remote/changelog.md
new file mode 100644
index 00000000..8f3cec43
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/changelog.md
@@ -0,0 +1,368 @@
+# Changelog
+The latest version may not be released!
+See also the commit log at github: https://github.com/Arduino-IRremote/Arduino-IRremote/commits/master
+
+# 4.2.2
+- Added convenience function isIRReceiverAttachedForTinyReceiver().
+- Added Extended NEC Protocol macro to TinyIR by Buzzerb.
+
+# 4.2.1
+- Fix wrong type of tEnableLEDFeedback in IRSend.hpp and IRReceive.hpp.
+- TinyReceiver 2.0
+ - New TinyIRReceiverData which is filled with address, command and flags.
+ - Removed parameters address, command and flags from callback handleReceivedTinyIRData() and printTinyReceiverResultMinimal().
+ - Callback function now only enabled if USE_CALLBACK_FOR_TINY_RECEIVER is activated.
+- Fix changing IR_SEND_PIN dynamically for ESP32.
+- Fix wrong type of tEnableLEDFeedback.
+- Support for ESP32-C3.
+
+# 4.2.0
+- The old decode function is renamed to decode_old(decode_results *aResults). decode (decode_results *aResults) is only available in IRremote.h and prints a message.
+- Added DECODE_ONKYO, to force 16 bit command and data decoding.
+- Enable Bang&Olufsen 455 kHz if SEND_PWM_BY_TIMER is defined.
+- Fixed bug: TinyReceiver throwing ISR not in IRAM on ESP8266.
+- Usage of ATTinyCore pin numbering scheme e.g. PIN_PB2.
+- Added ARDUINO_ARCH_NRF52 to support Seeed XIAO nRF52840 Sense.
+- First untested support of Uno R4.
+- Extraced version macros to IRVersion.h.
+
+## 4.1.2
+- Workaround for ESP32 RTOS delay() timing bug influencing the mark() function.
+
+## 4.1.1
+- SAMD51 use timer3 if timer5 not available.
+- Disabled #define LOCAL_DEBUG in IRReceive.hpp, which was accidently enabled at 4.1.0.
+
+## 4.1.0
+- Fixed bug in printing durations > 64535 in printIRResultRawFormatted().
+- Narrowed constraints for RC5 RC6 number of bits.
+- Changed the first parameter of printTinyReceiverResultMinimal() to &Serial.
+- Removed 3 Serial prints for deprecation warnings to fix #1094.
+- Version 1.2.0 of TinyIR. Now FAST protocol with 40 ms period and shorter header space.
+- Removed field "bool hasStopBit" and parameter "bool aSendStopBit" from PulseDistanceWidthProtocolConstants structure and related functions.
+- Changed a lot of "unsigned int" types to "uint16_t" types.
+- Improved overflow handling.
+- Improved software PWM generation.
+- Added FAST protocol.
+- Improved handling of PULSE_DISTANCE + PULSE_WIDTH protocols.
+- New example ReceiveAndSendDistanceWidth.
+- Removed the automatic restarting of the receiver timer after sending with SEND_PWM_BY_TIMER enabled.
+- Split ISR into ISR and function IRPinChangeInterruptHandler().
+- Added functions addTicksToInternalTickCounter() and addMicrosToInternalTickCounter().
+
+## 4.0.0
+- Added decoding of PulseDistanceWidth protocols and therefore changed function decodeDistance() to decodeDistanceWidth() and filename ir_DistanceProtocol.hpp to ir_DistanceWidthProtocol.hpp.
+- Removed static function printIRSendUsage(), but kept class function printIRSendUsage().
+- Changed type of decodedRawData and decodedRawDataArray which is now 64 bit for 32 bit platforms.
+- Added receiver callback functionality and registerReceiveCompleteCallback() function.
+- Introduced common structure PulseDistanceWidthProtocolConstants.
+- Where possible, changed all send and decode functions to use PulseDistanceWidthProtocolConstants.
+- Improved MSB/LSB handling
+- New convenience fuctions bitreverse32Bit() and bitreverseOneByte().
+- Improved Magiquest protocol.
+- Fix for #1028 - Prevent long delay caused by overflow when frame duration < repeat period - Thanks to Stephen Humphries!
+- Support for ATtiny816 - Thanks to elockman.
+- Added Bang&Olufsen protocol. #1030.
+- Third parameter of function "void begin(uint_fast8_t aSendPin, bool aEnableLEDFeedback, uint_fast8_t aFeedbackLEDPin)" is not optional anymore and this function is now only available if IR_SEND_PIN is not defined. #1033.
+- Fixed bug in sendSony() for command parameter > 0x7F;
+- Fixed bug with swapped LG2 header mark and space.
+- Disabled strict checks while decoding. They can be enabled by defining DECODE_STRICT_CHECKS.
+- Merged the 2 decode pulse width and distance functions.
+- Changed macro names _REPEAT_SPACE to _REPEAT_DISTANCE.
+- Improved TinyIRReceiver,added FAST protocol for it and added TinyIRSender.hpp and TinySender example, renamed TinyReceiver.h to TinyIR.h.
+- Added DISABLE_CODE_FOR_RECEIVER to save program memory and RAM if receiving functionality is not required.
+- Extracted protocol functions used by receive and send to IRProtocol.hpp.
+- Analyzed Denon code table and therefore changed Denon from MSB to LSB first.
+- Renamed sendRC6(aRawData...) to sendRC6Raw( aRawData...).
+- Support for seeduino which lacks the print(unsigned long long...) method. Thanks to sklott https://stackoverflow.com/users/11680056/sklott
+- Added support for attiny1614 by Joe Ostrander.
+- Fixed SEND_PWM_BY_TIMER for ATtiny167 thanks to freskpe.
+- Improved SHARP repeat decoding.
+- Replaced macros TIMER_EN/DISABLE_RECEIVE_INTR and EN/DISABLE_SEND_PWM_BY_TIMER by functions.
+- Added SAMSUNG48 protocol and sendSamsung48() function.
+
+## 3.9.0
+- Improved documentation with the help of [ElectronicsArchiver}(https://github.com/ElectronicsArchiver).
+- Added NEC2 protocol.
+- Improved Magiquest protocol.
+- Renamed sendSamsungRepeat() to sendSamsungLGRepeat().
+- Added function sendPulseDistanceWidth().
+- Improved repeat detection for some protocols.
+
+## 3.8.0
+- Changed Samsung repeat handling. Old handling is available as SamsungLG.
+- Added function printIRSendUsage().
+- Reduced output size and improved format of printIRResultRawFormatted() to fasten up output (and getting repeats properly decoded).
+- Fixed Bug in sendDenonRaw() and improved decodeDenon().
+- Fixed potential bug in SendBiphase data for 1 bit.
+- Fixed bug in send for RP4020.
+- Fixed pin mapping problems especially for Teensy 2.0.
+- Added support for decoding of "special" NEC repeats.
+- Added SAMD51 support.
+- Improved pin mapping for TinyReceiver.
+
+## 3.7.1
+- SendRaw now supports bufferlenght > 255.
+- Improved DistanceProtocol decoder output.
+- Fixed ESP32 send bug for 2.x ESP32 cores.
+
+## 3.7.0
+- Changed TOLERANCE to TOLERANCE_FOR_DECODERS_MARK_OR_SPACE_MATCHING and documented it.
+- Changed last uint8_t to uint_fast8_t and uint16_t to unsigned integer.
+- Improved MagiQuest protocol.
+- Improved prints and documentation.
+- Added IrReceiver.restartAfterSend() and inserted it in every send(). Closes #989
+- Use IRAM_ATTR instead of deprecated ICACHE_RAM_ATTR for ESP8266.
+- Removed pulse width decoding from ir_DistanceProtocol.
+
+## 3.6.1
+- Switched Bose internal protocol timing for 0 and 1 -> old 1 timing is now 0 and vice versa.
+
+## 3.6.0
+- Separated enable flag of send and receive feedback LED. Inspired by PR#970 from luvaihassanali.
+- RP2040 support added.
+- Refactored IRTimer.hpp.
+- Refactored IR_SEND_PIN and IrSender.sendPin handling.
+- Renamed IR_SEND_DUTY_CYCLE to IR_SEND_DUTY_CYCLE_PERCENT.
+- Fixed bugs for SEND_PWM_BY_TIMER active.
+
+## 3.5.2
+- Improved support for Teensy boards by Paul Stoffregen.
+
+## 3.5.1
+- Renamed INFO_PRINT to IR_INFO_PRINT as well as for DEBUG and TRACE.
+- Fixed error with DEBUG in TinyIRReceiver.hpp.
+- Support for ATmega88 see issue #923. Thanks to Dolmant.
+- NO_LED_FEEDBACK_CODE replaces and extends DISABLE_LED_FEEDBACK_FOR_RECEIVE.
+- Removed NO_LEGACY_COMPATIBILITY macro, it was useless now.
+- Fix ESP32 send bug see issue #927.
+
+## 3.5.0
+- Improved ir_DistanceProtocol.
+- Tone for ESP32.
+- last phase renamed *.cpp.h to .hpp.
+- No deprecation print for ATtinies.
+- Renamed ac_LG.cpp to ac_LG.hpp.
+- Maintained MagiQuest by E. Stuart Hicks.
+- Improved print Pronto by Asuki Kono.
+- Added printActiveIRProtocols() function.
+- Used IR_SEND_PIN to reduce code size and improved send timing for AVR.
+
+## 3.4.0
+- Added LG2 protocol.
+- Added ATtiny167 (Digispark Pro) support.
+- Renamed *.cpp.h to .hpp.
+- organized carrier frequencies.
+- Compiler switch USE_OPEN_DRAIN_OUTPUT_FOR_SEND_PIN added.
+- Moved blink13() back to IRrecv class.
+- Added Kaseikyo convenience functions like sendKaseikyo_Denon().
+- Improved / adjusted LG protocol and added class Aircondition_LG based on real hardware supplied by makerspace 201 (https://wiki.hackerspaces.org/ZwoNullEins) from Cologne.
+- Improved universal decoder for pulse distance protocols to support more than 32 bits.
+- Added mbed support.
+
+## 3.3.0
+- Fix errors if LED_BUILTIN is not defined.
+- Fixed error for AVR timer1. Thanks to alexbarcelo.
+- New example IRremoteExtensionTest.
+- Enabled megaAVR 0-series devices.
+- Added universal decoder for pulse distance protocols.
+
+## 3.2.0
+- Fix for ESP32 send Error, removed `USE_SOFT_SEND_PWM` macro.
+- Added Onkyo protocol.
+- Support for old 2.x code by backwards compatible `decode(decode_results *aResults)` function.
+- Removed USE_OLD_DECODE macro and added NO_LEGACY_COMPATIBILITY macro.
+- Added ATtiny1604 support.
+- New SendAndReceive example.
+- Added ESP8266 support.
+- Extended DEBUG output.
+
+## 3.1.0
+- Generation of PWM by software is active by default.
+- Removed decode_results results.
+- Renamed most irparams_struct values.
+- Fixed LG send bug and added unit test.
+- Replaced `#define DECODE_NEC 1/0` by defining/not defining.
+- Use LED_BUILTIN instead of FEEDBACK_LED if FeedbackLEDPin is 0.
+- Use F_CPU instead of SYSCLOCK.
+- Removed SENDPIN_ON and SENDPIN_OFF macros.
+
+- Refactored board specific code for timer and feedback LED.
+- Extracted common LED feedback functions and implemented feedback for send.
+- MATCH_MARK() etc. now available as matchMark().
+- Added STM32F1 by (by Roger Clark) support.
+- Added stm32 (by ST) support. Thanks to Paolo Malaspina.
+- Added ATtiny88 support.
+
+## 3.0.2
+- Bug fix for USE_OLD_DECODE.
+- Increase RECORD_GAP_MICROS to 11000.
+- Fix overflow message. (#793).
+- Improved handling for HASH decoder.
+- Tested for ATtiny85.
+- Added `printIRResultMinimal()`.
+- Added missing IRAM_ATTR for ESP32.
+- Adapted to TinyCore 0.0.7.
+- Fixed decodeSony 20 bit bug #811.
+- Replaced delayMicroseconds with customDelayMicroseconds and removed NoInterrupt() for send functions, removed SPIN_WAIT macro, sleepMicros() and sleepUntilMicros().
+- Fixed LG checksum error.
+- Fixed JVC repeat error.
+
+## 3.0.0 + 3.0.1 2021/02
+- New LSB first decoders are default.
+- Added SendRaw with byte data.
+- Fixed resume bug if irparams.rawlen >= RAW_BUFFER_LENGTH. Thanks to Iosif Peterfi
+- Added `dumpPronto(String *aString, unsigned int frequency)` with String object as argument. Thanks to Iosif Peterfi
+- Removed Test2 example.
+- Fixed swapped cases in `getProtocolString()`. Thanks to Jim-2249
+- Added compile option `IR_INPUT_IS_ACTIVE_HIGH`. Thanks to Jim-2249
+- Corrected template. Thanks to Jim-2249
+- Introduced standard decode and send functions.
+- Added compatibility with tone for AVR's.
+- New TinyIRreceiver does not require a timer.
+- New MinimalReceiver and IRDispatcherDemo examples.
+- Added TinyCore 32 / ATtiny3217 support.
+- Added Apple protocol.
+
+## 2.8.1 2020/10
+- Fixed bug in Sony decode introduced in 2.8.0.
+
+## 2.8.0 2020/10
+- Changed License to MIT see https://github.com/Arduino-IRremote/Arduino-IRremote/issues/397.
+- Added ATtiny timer 1 support.
+- Changed wrong return code signature of decodePulseDistanceData() and its handling.
+- Removed Mitsubishi protocol, since the implementation is in contradiction with all documentation I found and therefore supposed to be wrong.
+- Removed AIWA implementation, since it is only for 1 device and at least the sending was implemented wrong.
+- Added Lego_PF decode.
+- Changed internal usage of custom_delay_usec.
+- Moved dump/print functions from example to irReceiver.
+- irPronto.cpp: Using Print instead of Stream saves 1020 bytes program memory. Changed from & to * parameter type to be more transparent and consistent with other code of IRremote.
+
+## 2.7.0 2020/09
+- Added ATmega328PB support.
+- Renamed hardware specific macro and function names.
+- Renamed `USE_SOFT_CARRIER`, `USE_NO_CARRIER`, `DUTY_CYCLE` macros to `USE_SOFT_SEND_PWM`, `USE_NO_SEND_PWM`, `IR_SEND_DUTY_CYCLE`.
+- Removed blocking wait for ATmega32U4 Serial in examples.
+- Deactivated default debug output.
+- Optimized types in sendRC5ext and sendSharpAlt.
+- Added `DECODE_NEC_STANDARD` and `SEND_NEC_STANDARD`.
+- Renamed all IRrecv* examples to IRreceive*.
+- Added functions `printIRResultShort(&Serial)` and `getProtocolString(decode_type_t aDecodeType)`.
+- Added flag `decodedIRData.isRepeat`.
+- Updated examples.
+
+## 2.6.1 2020/08
+- Adjusted JVC and LG timing.
+- Fixed 4809 bug.
+
+## 2.6.0 2020/08
+- Added support for MagiQuest IR wands.
+- Corrected Samsung timing.
+- NEC repeat implementation.
+- Formatting and changing `TIMER_CONFIG_KHZ` and `TIMER_CONFIG_NORMAL` macros to static functions.
+- Added `IRAM_ATTR` for ESP32 ISR.
+- Removed `#define HAS_AVR_INTERRUPT_H`.
+- Changed Receiver States. Now starting with 0.
+- Changed switch to if / else if in IRRemote.cpp because of ESP32 compiler bug.
+- Changed `DEBUG` handling since compiler warns about empty "IF" or "ELSE" statements in IRRemote.cpp.
+
+## 2.5.0 2020/06
+- Corrected keywords.txt.
+- BoseWave protocol added PR #690.
+- Formatting comply to the new stylesheet.
+- Renamed "boarddefs.h" [ISSUE #375](https://github.com/Arduino-IRremote/Arduino-IRremote/issues/375).
+- Renamed `SEND_PIN` to `IR_SEND_PIN`.
+- Renamed state macros.
+- Enabled `DUTY_CYCLE` for send signal.
+- Added sending for ESP32.
+- Changed rawlen from uint8_t to unsigned int allowing bigger receive buffer and renamed `RAWBUF` to `RAW_BUFFER_LENGTH`.
+- Introduced `USE_NO_CARRIER` for simulating an IR receiver.
+Changes from #283 by bengtmartensson
+- Added function sendRaw_P() for sending data from flash.
+Changes from #268 by adamlhumphreys
+- Optimized by reducing floating point operations as suggested by madmalkav (#193).
+- Optimized with macros when using default `MICROS_PER_TICK` and `TOLERANCE`.
+- Made decodeHash as a settable protocol defined by `DECODE_HASH`.
+- Added Philips Extended RC-5 protocol support [PR #522] (https://github.com/Arduino-IRremote/Arduino-IRremote/pull/522)
+
+## 2.4.0 - 2017/08/10
+ - Cleanup of hardware dependencies. Merge in SAM support [PR #437](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/437)
+
+## 2.3.3 - 2017/03/31
+- Added ESP32 IR receive support [PR #427](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/425)
+
+## 2.2.3 - 2017/03/27
+- Fix calculation of pause length in LEGO PF protocol [PR #427](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/427)
+
+## 2.2.2 - 2017/01/20
+- Fixed naming bug [PR #398](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/398)
+
+## 2.2.1 - 2016/07/27
+- Added tests for Lego Power Functions Protocol [PR #336](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/336)
+
+## 2.2.0 - 2016/06/28
+- Added support for ATmega8535
+- Added support for ATmega16
+- Added support for ATmega32
+- Added support for ATmega164
+- Added support for ATmega324
+- Added support for ATmega644
+- Added support for ATmega1284
+- Added support for ATmega64
+- Added support for ATmega128
+
+[PR](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/324)
+
+## 2.1.1 - 2016/05/04
+- Added Lego Power Functions Protocol [PR #309](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/309)
+
+## 2.1.0 - 2016/02/20
+- Improved Debugging [PR #258](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/258)
+- Display TIME instead of TICKS [PR #258](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/258)
+
+## 2.0.4 - 2016/02/20
+- Add Panasonic and JVC to IRrecord example [PR](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/54)
+
+## 2.0.3 - 2016/02/20
+- Change IRSend Raw parameter to const [PR](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/227)
+
+## 2.0.2 - 2015/12/02
+- Added IRremoteInfo Sketch - [PR](https://github.com/Arduino-IRremote/Arduino-IRremote/pull/241)
+- Enforcing changelog.md
+
+## 2.0.1 - 2015/07/26 - [Release](https://github.com/shirriff/Arduino-IRremote/releases/tag/BETA)
+### Changes
+- Updated README
+- Updated Contributors
+- Fixed #110 Mess
+- Created Gitter Room
+- Added Gitter Badge
+- Standardised Code Base
+- Clean Debug Output
+- Optimized Send Loops
+- Modularized Design
+- Optimized and Updated Examples
+- Improved Documentation
+- Fixed and Improved many coding errors
+- Fixed Aiwa RC-T501 Decoding
+- Fixed Interrupt on ATmega8
+- Switched to Stable Release of PlatformIO
+
+### Additions
+- Added Aiwa RC-T501 Protocol
+- Added Denon Protocol
+- Added Pronto Support
+- Added compile options
+- Added Template For New Protocols
+- Added this changelog
+- Added Teensy LC Support
+- Added ATtiny84 Support
+- Added ATtiny85 Support
+- Added isIdle method
+
+### Deletions
+- Removed (Fixed) #110
+- Broke Teensy 3 / 3.1 Support
+
+### Not Working
+- Teensy 3 / 3.1 Support is in Development
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.h b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.h
new file mode 100644
index 00000000..ce283c2f
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.h
@@ -0,0 +1,227 @@
+/*
+ * ADCUtils.h
+ *
+ * Copyright (C) 2016-2022 Armin Joachimsmeyer
+ * Email: armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of Arduino-Utils https://github.com/ArminJo/Arduino-Utils.
+ *
+ * ArduinoUtils is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+#ifndef _ADC_UTILS_H
+#define _ADC_UTILS_H
+
+#include
+
+#if defined(__AVR__) && defined(ADCSRA) && defined(ADATE) && (!defined(__AVR_ATmega4809__))
+#define ADC_UTILS_ARE_AVAILABLE
+
+// PRESCALE4 => 13 * 4 = 52 microseconds per ADC conversion at 1 MHz Clock => 19,2 kHz
+#define ADC_PRESCALE2 1 // 26 microseconds per ADC conversion at 1 MHz
+#define ADC_PRESCALE4 2 // 52 microseconds per ADC conversion at 1 MHz
+// PRESCALE8 => 13 * 8 = 104 microseconds per ADC sample at 1 MHz Clock => 9,6 kHz
+#define ADC_PRESCALE8 3 // 104 microseconds per ADC conversion at 1 MHz
+#define ADC_PRESCALE16 4 // 13/208 microseconds per ADC conversion at 16/1 MHz - degradations in linearity at 16 MHz
+#define ADC_PRESCALE32 5 // 26/416 microseconds per ADC conversion at 16/1 MHz - very good linearity at 16 MHz
+#define ADC_PRESCALE64 6 // 52 microseconds per ADC conversion at 16 MHz
+#define ADC_PRESCALE128 7 // 104 microseconds per ADC conversion at 16 MHz --- Arduino default
+
+// definitions for 0.1 ms conversion time
+#if (F_CPU == 1000000)
+#define ADC_PRESCALE ADC_PRESCALE8
+#elif (F_CPU == 8000000)
+#define ADC_PRESCALE ADC_PRESCALE64
+#elif (F_CPU == 16000000)
+#define ADC_PRESCALE ADC_PRESCALE128
+#endif
+
+/*
+ * Reference shift values are complicated for ATtinyX5 since we have the extra register bit REFS2
+ * in ATTinyCore, this bit is handled programmatical and therefore the defines are different.
+ * To keep my library small, I use the changed defines.
+ * After including this file you can not call the ATTinyCore readAnalog functions reliable, if you specify references other than default!
+ */
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__)
+// defines are for ADCUtils.cpp, they can be used WITHOUT bit reordering
+#undef DEFAULT
+#undef EXTERNAL
+#undef INTERNAL1V1
+#undef INTERNAL
+#undef INTERNAL2V56
+#undef INTERNAL2V56_EXTCAP
+
+#define DEFAULT 0
+#define EXTERNAL 4
+#define INTERNAL1V1 8
+#define INTERNAL INTERNAL1V1
+#define INTERNAL2V56 9
+#define INTERNAL2V56_EXTCAP 13
+
+#define SHIFT_VALUE_FOR_REFERENCE REFS2
+#define MASK_FOR_ADC_REFERENCE (_BV(REFS0) | _BV(REFS1) | _BV(REFS2))
+#define MASK_FOR_ADC_CHANNELS (_BV(MUX0) | _BV(MUX1) | _BV(MUX2) | _BV(MUX3))
+#else // AVR_ATtiny85
+
+#define SHIFT_VALUE_FOR_REFERENCE REFS0
+#define MASK_FOR_ADC_REFERENCE (_BV(REFS0) | _BV(REFS1))
+#define MASK_FOR_ADC_CHANNELS (_BV(MUX0) | _BV(MUX1) | _BV(MUX2) | _BV(MUX3))
+#endif
+
+// Temperature channel definitions - 1 LSB / 1 degree Celsius
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__)
+#define ADC_TEMPERATURE_CHANNEL_MUX 15
+#define ADC_1_1_VOLT_CHANNEL_MUX 12
+#define ADC_GND_CHANNEL_MUX 13
+#define ADC_CHANNEL_MUX_MASK 0x0F
+
+#elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__)
+#define ADC_ISCR_CHANNEL_MUX 3
+#define ADC_TEMPERATURE_CHANNEL_MUX 11
+#define ADC_1_1_VOLT_CHANNEL_MUX 12
+#define ADC_GND_CHANNEL_MUX 14
+#define ADC_VCC_4TH_CHANNEL_MUX 13
+#define ADC_CHANNEL_MUX_MASK 0x1F
+
+#elif defined(__AVR_ATmega328P__)
+#define ADC_TEMPERATURE_CHANNEL_MUX 8
+#define ADC_1_1_VOLT_CHANNEL_MUX 14
+#define ADC_GND_CHANNEL_MUX 15
+#define ADC_CHANNEL_MUX_MASK 0x0F
+
+#elif defined(__AVR_ATmega32U4__)
+#define ADC_TEMPERATURE_CHANNEL_MUX 0x27
+#define ADC_1_1_VOLT_CHANNEL_MUX 0x1E
+#define ADC_GND_CHANNEL_MUX 0x1F
+#define ADC_CHANNEL_MUX_MASK 0x3F
+
+#elif defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__) || defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) || defined(__AVR_ATmega644__) || defined(__AVR_ATmega644A__) || defined(__AVR_ATmega644P__) || defined(__AVR_ATmega644PA__)
+#define ADC_1_1_VOLT_CHANNEL_MUX 0x1E
+#define ADC_GND_CHANNEL_MUX 0x1F
+#define ADC_CHANNEL_MUX_MASK 0x1F
+
+#define INTERNAL INTERNAL1V1
+
+#else
+#error "No temperature channel definitions specified for this AVR CPU"
+#endif
+
+/*
+ * Thresholds for OVER and UNDER voltage and detection of kind of power supply (USB or Li-ion)
+ *
+ * Default values are suitable for Li-ion batteries.
+ * We normally have voltage drop at the connectors, so the battery voltage is assumed slightly higher, than the Arduino VCC.
+ * But keep in mind that the ultrasonic distance module HC-SR04 may not work reliable below 3.7 volt.
+ */
+#if !defined(LI_ION_VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define LI_ION_VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT 3400 // Do not stress your battery and we require some power for standby
+#endif
+#if !defined(LI_ION_VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define LI_ION_VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT 3000 // Many Li-ions are specified down to 3.0 volt
+#endif
+
+#if !defined(VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT LI_ION_VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT
+#endif
+#if !defined(VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT LI_ION_VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT
+#endif
+#if !defined(VCC_OVERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define VCC_OVERVOLTAGE_THRESHOLD_MILLIVOLT 5250 // + 5 % operation voltage
+#endif
+#if !defined(VCC_EMERGENCY_OVERVOLTAGE_THRESHOLD_MILLIVOLT)
+#define VCC_EMERGENCY_OVERVOLTAGE_THRESHOLD_MILLIVOLT 5500 // +10 %. Max recommended operation voltage
+#endif
+#if !defined(VCC_CHECK_PERIOD_MILLIS)
+#define VCC_CHECK_PERIOD_MILLIS 10000L // 10 seconds period of VCC checks
+#endif
+#if !defined(VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP)
+#define VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP 6 // Shutdown after 6 times (60 seconds) VCC below VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT or 1 time below VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT
+#endif
+
+#if !defined(VOLTAGE_USB_POWERED_LOWER_THRESHOLD_MILLIVOLT)
+#define VOLTAGE_USB_POWERED_LOWER_THRESHOLD_MILLIVOLT 4300 // Assume USB powered above this voltage
+#endif
+
+#if !defined(VOLTAGE_USB_POWERED_UPPER_THRESHOLD_MILLIVOLT)
+#define VOLTAGE_USB_POWERED_UPPER_THRESHOLD_MILLIVOLT 4950 // Assume USB powered below this voltage, because of the loss in USB cable. If we have > 4950, we assume to be powered by VIN.
+// In contrast to e.g. powered by VIN, which results in almost perfect 5 volt supply
+#endif
+
+extern long sLastVCCCheckMillis;
+extern uint8_t sVCCTooLowCounter;
+
+uint16_t readADCChannel(uint8_t aADCChannelNumber);
+uint16_t readADCChannelWithReference(uint8_t aADCChannelNumber, uint8_t aReference);
+uint16_t waitAndReadADCChannelWithReference(uint8_t aADCChannelNumber, uint8_t aReference);
+uint16_t waitAndReadADCChannelWithReferenceAndRestoreADMUXAndReference(uint8_t aADCChannelNumber, uint8_t aReference);
+uint16_t readADCChannelWithOversample(uint8_t aADCChannelNumber, uint8_t aOversampleExponent);
+void setADCChannelAndReferenceForNextConversion(uint8_t aADCChannelNumber, uint8_t aReference);
+uint16_t readADCChannelWithReferenceOversampleFast(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aOversampleExponent);
+uint32_t readADCChannelMultiSamples(uint8_t aPrescale, uint16_t aNumberOfSamples);
+uint16_t readADCChannelMultiSamplesWithReference(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aNumberOfSamples);
+uint32_t readADCChannelMultiSamplesWithReferenceAndPrescaler(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aPrescale,
+ uint16_t aNumberOfSamples);
+uint16_t readADCChannelWithReferenceMax(uint8_t aADCChannelNumber, uint8_t aReference, uint16_t aNumberOfSamples);
+uint16_t readADCChannelWithReferenceMaxMicros(uint8_t aADCChannelNumber, uint8_t aReference, uint16_t aMicrosecondsToAquire);
+uint16_t readUntil4ConsecutiveValuesAreEqual(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aDelay,
+ uint8_t aAllowedDifference, uint8_t aMaxRetries);
+
+uint8_t checkAndWaitForReferenceAndChannelToSwitch(uint8_t aADCChannelNumber, uint8_t aReference);
+
+/*
+ * readVCC*() functions store the result in sVCCVoltageMillivolt or sVCCVoltage
+ */
+float getVCCVoltageSimple(void);
+void readVCCVoltageSimple(void);
+void readVCCVoltageMillivoltSimple(void);
+void readVCCVoltage(void);
+uint16_t getVCCVoltageMillivolt(void);
+void readVCCVoltageMillivolt(void);
+uint16_t getVCCVoltageReadingFor1_1VoltReference(void);
+uint16_t printVCCVoltageMillivolt(Print *aSerial);
+void readAndPrintVCCVoltageMillivolt(Print *aSerial);
+
+uint16_t getVoltageMillivolt(uint16_t aVCCVoltageMillivolt, uint8_t aADCChannelForVoltageMeasurement);
+uint16_t getVoltageMillivolt(uint8_t aADCChannelForVoltageMeasurement);
+uint16_t getVoltageMillivoltWith_1_1VoltReference(uint8_t aADCChannelForVoltageMeasurement);
+float getCPUTemperatureSimple(void);
+float getCPUTemperature(void);
+float getTemperature(void) __attribute__ ((deprecated ("Renamed to getCPUTemperature()"))); // deprecated
+
+bool isVCCUSBPowered();
+bool isVCCUSBPowered(Print *aSerial);
+bool isVCCUndervoltageMultipleTimes();
+void resetCounterForVCCUndervoltageMultipleTimes();
+bool isVCCUndervoltage();
+bool isVCCEmergencyUndervoltage();
+bool isVCCOvervoltage();
+bool isVCCOvervoltageSimple();
+
+#endif // defined(__AVR__) ...
+
+/*
+ * Variables and functions defined as dummies to allow for seamless compiling on non AVR platforms
+ */
+extern float sVCCVoltage;
+extern uint16_t sVCCVoltageMillivolt;
+
+uint16_t readADCChannelWithReferenceOversample(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aOversampleExponent);
+
+uint16_t getVCCVoltageMillivoltSimple(void);
+float getVCCVoltage(void);
+float getCPUTemperature(void);
+
+#endif // _ADC_UTILS_H
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.hpp b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.hpp
new file mode 100644
index 00000000..0cff66eb
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/ADCUtils.hpp
@@ -0,0 +1,799 @@
+/*
+ * ADCUtils.hpp
+ *
+ * ADC utility functions. Conversion time is defined as 0.104 milliseconds for 16 MHz Arduinos in ADCUtils.h.
+ *
+ * Copyright (C) 2016-2023 Armin Joachimsmeyer
+ * Email: armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of Arduino-Utils https://github.com/ArminJo/Arduino-Utils.
+ *
+ * ArduinoUtils is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#ifndef _ADC_UTILS_HPP
+#define _ADC_UTILS_HPP
+
+#include "ADCUtils.h"
+#if defined(ADC_UTILS_ARE_AVAILABLE) // set in ADCUtils.h, if supported architecture was detected
+
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
+
+/*
+ * By replacing this value with the voltage you measured a the AREF pin after a conversion
+ * with INTERNAL you can calibrate your ADC readout. For my Nanos I measured e.g. 1060 mV and 1093 mV.
+ */
+#if !defined(ADC_INTERNAL_REFERENCE_MILLIVOLT)
+#define ADC_INTERNAL_REFERENCE_MILLIVOLT 1100L // Change to value measured at the AREF pin. If value > real AREF voltage, measured values are > real values
+#endif
+
+// Union to speed up the combination of low and high bytes to a word
+// it is not optimal since the compiler still generates 2 unnecessary moves
+// but using -- value = (high << 8) | low -- gives 5 unnecessary instructions
+union WordUnionForADCUtils {
+ struct {
+ uint8_t LowByte;
+ uint8_t HighByte;
+ } UByte;
+ uint16_t UWord;
+ int16_t Word;
+ uint8_t *BytePointer;
+};
+
+/*
+ * Enable this to see information on each call.
+ * Since there should be no library which uses Serial, it should only be enabled for development purposes.
+ */
+#if defined(DEBUG) && !defined(LOCAL_DEBUG)
+#define LOCAL_DEBUG
+#else
+//#define LOCAL_DEBUG // This enables debug output only for this file
+#endif
+
+/*
+ * Persistent storage for VCC value
+ */
+float sVCCVoltage;
+uint16_t sVCCVoltageMillivolt;
+
+// for isVCCTooLowMultipleTimes()
+long sLastVCCCheckMillis;
+uint8_t sVCCTooLowCounter = 0;
+
+/*
+ * Conversion time is defined as 0.104 milliseconds by ADC_PRESCALE in ADCUtils.h.
+ */
+uint16_t readADCChannel(uint8_t aADCChannelNumber) {
+ WordUnionForADCUtils tUValue;
+ ADMUX = aADCChannelNumber | (DEFAULT << SHIFT_VALUE_FOR_REFERENCE);
+
+ // ADCSRB = 0; // Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADIF-Reset Interrupt Flag - NOT free running mode
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADIF) | ADC_PRESCALE);
+
+ // wait for single conversion to finish
+ loop_until_bit_is_clear(ADCSRA, ADSC);
+
+ // Get value
+ tUValue.UByte.LowByte = ADCL;
+ tUValue.UByte.HighByte = ADCH;
+ return tUValue.UWord;
+ // return ADCL | (ADCH <<8); // needs 4 bytes more
+}
+
+/*
+ * Conversion time is defined as 0.104 milliseconds by ADC_PRESCALE in ADCUtils.h.
+ */
+uint16_t readADCChannelWithReference(uint8_t aADCChannelNumber, uint8_t aReference) {
+ WordUnionForADCUtils tUValue;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ // ADCSRB = 0; // Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADIF-Reset Interrupt Flag - NOT free running mode
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADIF) | ADC_PRESCALE);
+
+ // wait for single conversion to finish
+ loop_until_bit_is_clear(ADCSRA, ADSC);
+
+ // Get value
+ tUValue.UByte.LowByte = ADCL;
+ tUValue.UByte.HighByte = ADCH;
+ return tUValue.UWord;
+}
+
+/*
+ * Conversion time is defined as 0.104 milliseconds by ADC_PRESCALE in ADCUtils.h.
+ * Does NOT restore ADMUX after reading
+ */
+uint16_t waitAndReadADCChannelWithReference(uint8_t aADCChannelNumber, uint8_t aReference) {
+ checkAndWaitForReferenceAndChannelToSwitch(aADCChannelNumber, aReference);
+ return readADCChannelWithReference(aADCChannelNumber, aReference);
+}
+
+/*
+ * Conversion time is defined as 0.104 milliseconds by ADC_PRESCALE in ADCUtils.h.
+ * Restores ADMUX after reading
+ */
+uint16_t waitAndReadADCChannelWithReferenceAndRestoreADMUXAndReference(uint8_t aADCChannelNumber, uint8_t aReference) {
+ uint8_t tOldADMUX = checkAndWaitForReferenceAndChannelToSwitch(aADCChannelNumber, aReference);
+ uint16_t tResult = readADCChannelWithReference(aADCChannelNumber, aReference);
+ checkAndWaitForReferenceAndChannelToSwitch(tOldADMUX & MASK_FOR_ADC_CHANNELS, tOldADMUX >> SHIFT_VALUE_FOR_REFERENCE);
+ return tResult;
+}
+
+/*
+ * To prepare reference and ADMUX for next measurement
+ */
+void setADCChannelAndReferenceForNextConversion(uint8_t aADCChannelNumber, uint8_t aReference) {
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+}
+
+/*
+ * @return original ADMUX register content for optional later restoring values
+ * All experimental values are acquired by using the ADCSwitchingTest example from this library
+ */
+uint8_t checkAndWaitForReferenceAndChannelToSwitch(uint8_t aADCChannelNumber, uint8_t aReference) {
+ uint8_t tOldADMUX = ADMUX;
+ /*
+ * Must wait >= 7 us if reference has to be switched from 1.1 volt/INTERNAL to VCC/DEFAULT (seen on oscilloscope)
+ * This is done after the 2 ADC clock cycles required for Sample & Hold :-)
+ *
+ * Must wait >= 7600 us for Nano board >= 6200 for Uno board if reference has to be switched from VCC/DEFAULT to 1.1 volt/INTERNAL
+ * Must wait >= 200 us if channel has to be switched to 1.1 volt internal channel if S&H was at 5 Volt
+ */
+ uint8_t tNewReference = (aReference << SHIFT_VALUE_FOR_REFERENCE);
+ ADMUX = aADCChannelNumber | tNewReference;
+#if defined(INTERNAL2V56)
+ if ((tOldADMUX & MASK_FOR_ADC_REFERENCE) != tNewReference && (aReference == INTERNAL || aReference == INTERNAL2V56)) {
+#else
+ if ((tOldADMUX & MASK_FOR_ADC_REFERENCE) != tNewReference && aReference == INTERNAL) {
+#endif
+#if defined(LOCAL_DEBUG)
+ Serial.println(F("Switch from DEFAULT to INTERNAL"));
+#endif
+ /*
+ * Switch reference from DEFAULT to INTERNAL
+ */
+ delayMicroseconds(8000); // experimental value is >= 7600 us for Nano board and 6200 for Uno board
+ } else if ((tOldADMUX & ADC_CHANNEL_MUX_MASK) != aADCChannelNumber) {
+ if (aADCChannelNumber == ADC_1_1_VOLT_CHANNEL_MUX) {
+ /*
+ * Internal 1.1 Volt channel requires <= 200 us for Nano board
+ */
+ delayMicroseconds(350); // 350 was ok and 300 was too less for UltimateBatteryTester - result was 226 instead of 225
+ } else {
+ /*
+ * 100 kOhm requires < 100 us, 1 MOhm requires 120 us S&H switching time
+ */
+ delayMicroseconds(120); // experimental value is <= 1100 us for Nano board
+ }
+ }
+ return tOldADMUX;
+}
+
+/*
+ * Oversample and multiple samples only makes sense if you expect a noisy input signal
+ * It does NOT increase the precision of the measurement, since the ADC has insignificant noise
+ */
+uint16_t readADCChannelWithOversample(uint8_t aADCChannelNumber, uint8_t aOversampleExponent) {
+ return readADCChannelWithReferenceOversample(aADCChannelNumber, DEFAULT, aOversampleExponent);
+}
+
+/*
+ * Conversion time is defined as 0.104 milliseconds by ADC_PRESCALE in ADCUtils.h.
+ */
+uint16_t readADCChannelWithReferenceOversample(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aOversampleExponent) {
+ uint16_t tSumValue = 0;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | ADC_PRESCALE);
+
+ uint8_t tCount = _BV(aOversampleExponent);
+ for (uint8_t i = 0; i < tCount; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // Add value
+ tSumValue += ADCL | (ADCH << 8); // using WordUnionForADCUtils does not save space here
+ // tSumValue += (ADCH << 8) | ADCL; // this does NOT work!
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ // return rounded value
+ return ((tSumValue + (tCount >> 1)) >> aOversampleExponent);
+}
+
+/*
+ * Use ADC_PRESCALE32 which gives 26 us conversion time and good linearity for 16 MHz Arduino
+ */
+uint16_t readADCChannelWithReferenceOversampleFast(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aOversampleExponent) {
+ uint16_t tSumValue = 0;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | ADC_PRESCALE32);
+
+ uint8_t tCount = _BV(aOversampleExponent);
+ for (uint8_t i = 0; i < tCount; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // Add value
+ tSumValue += ADCL | (ADCH << 8); // using WordUnionForADCUtils does not save space here
+ // tSumValue += (ADCH << 8) | ADCL; // this does NOT work!
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ return ((tSumValue + (tCount >> 1)) >> aOversampleExponent);
+}
+
+/*
+ * Returns sum of all sample values
+ * Conversion time is defined as 0.104 milliseconds for 16 MHz Arduino by ADC_PRESCALE (=ADC_PRESCALE128) in ADCUtils.h.
+ * @ param aNumberOfSamples If > 64 an overflow may occur.
+ */
+uint16_t readADCChannelMultiSamplesWithReference(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aNumberOfSamples) {
+ uint16_t tSumValue = 0;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | ADC_PRESCALE);
+
+ for (uint8_t i = 0; i < aNumberOfSamples; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // Add value
+ tSumValue += ADCL | (ADCH << 8); // using WordUnionForADCUtils does not save space here
+ // tSumValue += (ADCH << 8) | ADCL; // this does NOT work!
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ return tSumValue;
+}
+
+/*
+ * Returns sum of all sample values
+ * Conversion time is defined as 0.104 milliseconds for 16 MHz Arduino for ADC_PRESCALE128 in ADCUtils.h.
+ * @ param aPrescale can be one of ADC_PRESCALE2, ADC_PRESCALE4, 8, 16, 32, 64, 128.
+ * ADC_PRESCALE32 is recommended for excellent linearity and fast readout of 26 microseconds
+ * @ param aNumberOfSamples If > 16k an overflow may occur.
+ */
+uint32_t readADCChannelMultiSamplesWithReferenceAndPrescaler(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aPrescale,
+ uint16_t aNumberOfSamples) {
+ uint32_t tSumValue = 0;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | aPrescale);
+
+ for (uint16_t i = 0; i < aNumberOfSamples; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // Add value
+ tSumValue += ADCL | (ADCH << 8); // using WordUnionForADCUtils does not save space here
+ // tSumValue += (ADCH << 8) | ADCL; // this does NOT work!
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ return tSumValue;
+}
+
+/*
+ * Returns sum of all sample values
+ * Assumes, that channel and reference are still set to the right values
+ * @ param aNumberOfSamples If > 16k an overflow may occur.
+ */
+uint32_t readADCChannelMultiSamples(uint8_t aPrescale, uint16_t aNumberOfSamples) {
+ uint32_t tSumValue = 0;
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | aPrescale);
+
+ for (uint16_t i = 0; i < aNumberOfSamples; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // Add value
+ tSumValue += ADCL | (ADCH << 8); // using WordUnionForADCUtils does not save space here
+ // tSumValue += (ADCH << 8) | ADCL; // this does NOT work!
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ return tSumValue;
+}
+/*
+ * use ADC_PRESCALE32 which gives 26 us conversion time and good linearity
+ * @return the maximum value of aNumberOfSamples samples.
+ */
+uint16_t readADCChannelWithReferenceMax(uint8_t aADCChannelNumber, uint8_t aReference, uint16_t aNumberOfSamples) {
+ uint16_t tADCValue = 0;
+ uint16_t tMaximum = 0;
+ ADMUX = aADCChannelNumber | (aReference << SHIFT_VALUE_FOR_REFERENCE);
+
+ ADCSRB = 0; // Free running mode. Only active if ADATE is set to 1.
+ // ADSC-StartConversion ADATE-AutoTriggerEnable ADIF-Reset Interrupt Flag
+ ADCSRA = (_BV(ADEN) | _BV(ADSC) | _BV(ADATE) | _BV(ADIF) | ADC_PRESCALE32);
+
+ for (uint16_t i = 0; i < aNumberOfSamples; i++) {
+ /*
+ * wait for free running conversion to finish.
+ * Do not wait for ADSC here, since ADSC is only low for 1 ADC Clock cycle on free running conversion.
+ */
+ loop_until_bit_is_set(ADCSRA, ADIF);
+
+ ADCSRA |= _BV(ADIF); // clear bit to enable recognizing next conversion has finished
+ // check value
+ tADCValue = ADCL | (ADCH << 8);
+ if (tADCValue > tMaximum) {
+ tMaximum = tADCValue;
+ }
+ }
+ ADCSRA &= ~_BV(ADATE); // Disable auto-triggering (free running mode)
+ return tMaximum;
+}
+
+/*
+ * use ADC_PRESCALE32 which gives 26 us conversion time and good linearity
+ * @return the maximum value during aMicrosecondsToAquire measurement.
+ */
+uint16_t readADCChannelWithReferenceMaxMicros(uint8_t aADCChannelNumber, uint8_t aReference, uint16_t aMicrosecondsToAquire) {
+ uint16_t tNumberOfSamples = aMicrosecondsToAquire / 26;
+ return readADCChannelWithReferenceMax(aADCChannelNumber, aReference, tNumberOfSamples);
+}
+
+/*
+ * aMaxRetries = 255 -> try forever
+ * @return (tMax + tMin) / 2
+ */
+uint16_t readUntil4ConsecutiveValuesAreEqual(uint8_t aADCChannelNumber, uint8_t aReference, uint8_t aDelay,
+ uint8_t aAllowedDifference, uint8_t aMaxRetries) {
+ int tValues[4]; // last value is in tValues[3]
+ int tMin;
+ int tMax;
+
+ /*
+ * Initialize first 4 values before checking
+ */
+ tValues[0] = readADCChannelWithReference(aADCChannelNumber, aReference);
+ for (int i = 1; i < 4; ++i) {
+ if (aDelay != 0) {
+ delay(aDelay); // Minimum is only 3 delays!
+ }
+ tValues[i] = readADCChannelWithReference(aADCChannelNumber, aReference);
+ }
+
+ do {
+ /*
+ * Get min and max of the last 4 values
+ */
+ tMin = 1024;
+ tMax = 0;
+ for (uint_fast8_t i = 0; i < 4; ++i) {
+ if (tValues[i] < tMin) {
+ tMin = tValues[i];
+ }
+ if (tValues[i] > tMax) {
+ tMax = tValues[i];
+ }
+ }
+ /*
+ * check for terminating condition
+ */
+ if ((tMax - tMin) <= aAllowedDifference) {
+ break;
+ } else {
+ /*
+ * Get next value
+ */
+// Serial.print("Difference=");
+// Serial.println(tMax - tMin);
+ // Move values to front
+ for (int i = 0; i < 3; ++i) {
+ tValues[i] = tValues[i + 1];
+ }
+ // and wait before getting next value
+ if (aDelay != 0) {
+ delay(aDelay);
+ }
+ tValues[3] = readADCChannelWithReference(aADCChannelNumber, aReference);
+ }
+ if (aMaxRetries != 255) {
+ aMaxRetries--;
+ }
+ } while (aMaxRetries > 0);
+
+#if defined(LOCAL_DEBUG)
+ if(aMaxRetries == 0) {
+ Serial.print(F("No 4 equal values for difference "));
+ Serial.print(aAllowedDifference);
+ Serial.print(F(" found "));
+ Serial.print(tValues[0]);
+ Serial.print(' ');
+ Serial.print(tValues[1]);
+ Serial.print(' ');
+ Serial.print(tValues[2]);
+ Serial.print(' ');
+ Serial.println(tValues[3]);
+ } else {
+ Serial.print(aMaxRetries);
+ Serial.println(F(" retries left"));
+ }
+#endif
+
+ return (tMax + tMin) / 2;
+}
+
+/*
+ * !!! Function without handling of switched reference and channel.!!!
+ * Use it ONLY if you only call getVCCVoltageSimple() or getVCCVoltageMillivoltSimple() in your program.
+ * !!! Resolution is only 20 millivolt !!!
+ * Raw reading of 1.1 V is 225 at 5 V.
+ * Raw reading of 1.1 V is 221 at 5.1 V.
+ * Raw reading of 1.1 V is 214 at 5.25 V (+5 %).
+ * Raw reading of 1.1 V is 204 at 5.5 V (+10 %).
+ */
+float getVCCVoltageSimple(void) {
+ // use AVCC with (optional) external capacitor at AREF pin as reference
+ float tVCC = readADCChannelMultiSamplesWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT, 4);
+ return ((1023 * 1.1 * 4) / tVCC);
+}
+
+/*
+ * !!! Function without handling of switched reference and channel.!!!
+ * Use it ONLY if you only call getVCCVoltageSimple() or getVCCVoltageMillivoltSimple() in your program.
+ * !!! Resolution is only 20 millivolt !!!
+ */
+uint16_t getVCCVoltageMillivoltSimple(void) {
+ // use AVCC with external capacitor at AREF pin as reference
+ uint16_t tVCC = readADCChannelMultiSamplesWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT, 4);
+ return ((1023L * ADC_INTERNAL_REFERENCE_MILLIVOLT * 4) / tVCC);
+}
+
+/*
+ * Gets the hypothetical 14 bit reading of VCC using 1.1 volt reference
+ * Similar to getVCCVoltageMillivolt() * 1023 / 1100
+ */
+uint16_t getVCCVoltageReadingFor1_1VoltReference(void) {
+ uint16_t tVCC = waitAndReadADCChannelWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT);
+ /*
+ * Do not switch back ADMUX to enable checkAndWaitForReferenceAndChannelToSwitch() to work correctly for the next measurement
+ */
+ return ((1023L * 1023L) / tVCC);
+}
+
+/*
+ * !!! Resolution is only 20 millivolt !!!
+ */
+float getVCCVoltage(void) {
+ return (getVCCVoltageMillivolt() / 1000.0);
+}
+
+/*
+ * Read value of 1.1 volt internal channel using VCC (DEFAULT) as reference.
+ * Handles reference and channel switching by introducing the appropriate delays.
+ * !!! Resolution is only 20 millivolt !!!
+ * Raw reading of 1.1 V is 225 at 5 V.
+ * Raw reading of 1.1 V is 221 at 5.1 V.
+ * Raw reading of 1.1 V is 214 at 5.25 V (+5 %).
+ * Raw reading of 1.1 V is 204 at 5.5 V (+10 %).
+ */
+uint16_t getVCCVoltageMillivolt(void) {
+ uint16_t tVCC = waitAndReadADCChannelWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT);
+ /*
+ * Do not switch back ADMUX to enable checkAndWaitForReferenceAndChannelToSwitch() to work correctly for the next measurement
+ */
+ return ((1023L * ADC_INTERNAL_REFERENCE_MILLIVOLT) / tVCC);
+}
+
+/*
+ * Does not set sVCCVoltageMillivolt
+ */
+uint16_t printVCCVoltageMillivolt(Print *aSerial) {
+ aSerial->print(F("VCC="));
+ uint16_t tVCCVoltageMillivolt = getVCCVoltageMillivolt();
+ aSerial->print(tVCCVoltageMillivolt);
+ aSerial->println(" mV");
+ return tVCCVoltageMillivolt;
+}
+
+void readAndPrintVCCVoltageMillivolt(Print *aSerial) {
+ aSerial->print(F("VCC="));
+ sVCCVoltageMillivolt = getVCCVoltageMillivolt();
+ aSerial->print(sVCCVoltageMillivolt);
+ aSerial->println(" mV");
+}
+/*
+ * !!! Function without handling of switched reference and channel.!!!
+ * Use it ONLY if you only call getVCCVoltageSimple() or getVCCVoltageMillivoltSimple() in your program.
+ * !!! Resolution is only 20 millivolt !!!
+ */
+void readVCCVoltageSimple(void) {
+ // use AVCC with (optional) external capacitor at AREF pin as reference
+ float tVCC = readADCChannelMultiSamplesWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT, 4);
+ sVCCVoltage = (1023 * (((float) ADC_INTERNAL_REFERENCE_MILLIVOLT) / 1000) * 4) / tVCC;
+}
+
+/*
+ * !!! Function without handling of switched reference and channel.!!!
+ * Use it ONLY if you only call getVCCVoltageSimple() or getVCCVoltageMillivoltSimple() in your program.
+ * !!! Resolution is only 20 millivolt !!!
+ */
+void readVCCVoltageMillivoltSimple(void) {
+ // use AVCC with external capacitor at AREF pin as reference
+ uint16_t tVCCVoltageMillivoltRaw = readADCChannelMultiSamplesWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT, 4);
+ sVCCVoltageMillivolt = (1023L * ADC_INTERNAL_REFERENCE_MILLIVOLT * 4) / tVCCVoltageMillivoltRaw;
+}
+
+/*
+ * !!! Resolution is only 20 millivolt !!!
+ */
+void readVCCVoltage(void) {
+ sVCCVoltage = getVCCVoltageMillivolt() / 1000.0;
+}
+
+/*
+ * Read value of 1.1 volt internal channel using VCC (DEFAULT) as reference.
+ * Handles reference and channel switching by introducing the appropriate delays.
+ * !!! Resolution is only 20 millivolt !!!
+ * Sets also the sVCCVoltageMillivolt variable.
+ */
+void readVCCVoltageMillivolt(void) {
+ uint16_t tVCCVoltageMillivoltRaw = waitAndReadADCChannelWithReference(ADC_1_1_VOLT_CHANNEL_MUX, DEFAULT);
+ /*
+ * Do not switch back ADMUX to enable checkAndWaitForReferenceAndChannelToSwitch() to work correctly for the next measurement
+ */
+ sVCCVoltageMillivolt = (1023L * ADC_INTERNAL_REFERENCE_MILLIVOLT) / tVCCVoltageMillivoltRaw;
+}
+
+/*
+ * Get voltage at ADC channel aADCChannelForVoltageMeasurement
+ * aVCCVoltageMillivolt is assumed as reference voltage
+ */
+uint16_t getVoltageMillivolt(uint16_t aVCCVoltageMillivolt, uint8_t aADCChannelForVoltageMeasurement) {
+ uint16_t tInputVoltageRaw = waitAndReadADCChannelWithReference(aADCChannelForVoltageMeasurement, DEFAULT);
+ return (aVCCVoltageMillivolt * (uint32_t) tInputVoltageRaw) / 1023;
+}
+
+/*
+ * Get voltage at ADC channel aADCChannelForVoltageMeasurement
+ * Reference voltage VCC is determined just before
+ */
+uint16_t getVoltageMillivolt(uint8_t aADCChannelForVoltageMeasurement) {
+ uint16_t tInputVoltageRaw = waitAndReadADCChannelWithReference(aADCChannelForVoltageMeasurement, DEFAULT);
+ return (getVCCVoltageMillivolt() * (uint32_t) tInputVoltageRaw) / 1023;
+}
+
+uint16_t getVoltageMillivoltWith_1_1VoltReference(uint8_t aADCChannelForVoltageMeasurement) {
+ uint16_t tInputVoltageRaw = waitAndReadADCChannelWithReference(aADCChannelForVoltageMeasurement, INTERNAL);
+ return (ADC_INTERNAL_REFERENCE_MILLIVOLT * (uint32_t) tInputVoltageRaw) / 1023;
+}
+
+/*
+ * Return true if sVCCVoltageMillivolt is > 4.3 V and < 4.95 V
+ */
+bool isVCCUSBPowered() {
+ readVCCVoltageMillivolt();
+ return (VOLTAGE_USB_POWERED_LOWER_THRESHOLD_MILLIVOLT < sVCCVoltageMillivolt
+ && sVCCVoltageMillivolt < VOLTAGE_USB_POWERED_UPPER_THRESHOLD_MILLIVOLT);
+}
+
+/*
+ * Return true if sVCCVoltageMillivolt is > 4.3 V and < 4.95 V
+ */
+bool isVCCUSBPowered(Print *aSerial) {
+ readVCCVoltageMillivolt();
+ aSerial->print(F("USB powered is "));
+ bool tReturnValue;
+ if (VOLTAGE_USB_POWERED_LOWER_THRESHOLD_MILLIVOLT
+ < sVCCVoltageMillivolt&& sVCCVoltageMillivolt < VOLTAGE_USB_POWERED_UPPER_THRESHOLD_MILLIVOLT) {
+ tReturnValue = true;
+ aSerial->print(F("true "));
+ } else {
+ tReturnValue = false;
+ aSerial->print(F("false "));
+ }
+ printVCCVoltageMillivolt(aSerial);
+ return tReturnValue;
+}
+
+/*
+ * @ return true only once, when VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP (6) times voltage too low -> shutdown
+ */
+bool isVCCUndervoltageMultipleTimes() {
+ /*
+ * Check VCC every VCC_CHECK_PERIOD_MILLIS (10) seconds
+ */
+
+ if (millis() - sLastVCCCheckMillis >= VCC_CHECK_PERIOD_MILLIS) {
+ sLastVCCCheckMillis = millis();
+
+# if defined(INFO)
+ readAndPrintVCCVoltageMillivolt(&Serial);
+# else
+ readVCCVoltageMillivolt();
+# endif
+
+ if (sVCCTooLowCounter < VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP) {
+ /*
+ * Do not check again if shutdown has happened
+ */
+ if (sVCCVoltageMillivolt > VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT) {
+ sVCCTooLowCounter = 0; // reset counter
+ } else {
+ /*
+ * Voltage too low, wait VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP (6) times and then shut down.
+ */
+ if (sVCCVoltageMillivolt < VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT) {
+ // emergency shutdown
+ sVCCTooLowCounter = VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP;
+# if defined(INFO)
+ Serial.println(
+ F(
+ "Voltage < " STR(VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT) " mV detected -> emergency shutdown"));
+# endif
+ } else {
+ sVCCTooLowCounter++;
+# if defined(INFO)
+ Serial.print(F("Voltage < " STR(VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT) " mV detected: "));
+ Serial.print(VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP - sVCCTooLowCounter);
+ Serial.println(F(" tries left"));
+# endif
+ }
+ if (sVCCTooLowCounter == VCC_UNDERVOLTAGE_CHECKS_BEFORE_STOP) {
+ /*
+ * 6 times voltage too low -> return signal for shutdown etc.
+ */
+ return true;
+ }
+ }
+ }
+ }
+ return false;
+}
+
+/*
+ * Return true if VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT (3 V) reached
+ */
+bool isVCCUndervoltage() {
+ readVCCVoltageMillivolt();
+ return (sVCCVoltageMillivolt < VCC_UNDERVOLTAGE_THRESHOLD_MILLIVOLT);
+}
+
+/*
+ * Return true if VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT (3 V) reached
+ */
+bool isVCCEmergencyUndervoltage() {
+ readVCCVoltageMillivolt();
+ return (sVCCVoltageMillivolt < VCC_EMERGENCY_UNDERVOLTAGE_THRESHOLD_MILLIVOLT);
+}
+
+void resetCounterForVCCUndervoltageMultipleTimes() {
+ sVCCTooLowCounter = 0;
+}
+
+/*
+ * Recommended VCC is 1.8 V to 5.5 V, absolute maximum VCC is 6.0 V.
+ * Check for 5.25 V, because such overvoltage is quite unlikely to happen during regular operation.
+ * Raw reading of 1.1 V is 225 at 5 V.
+ * Raw reading of 1.1 V is 221 at 5.1 V.
+ * Raw reading of 1.1 V is 214 at 5.25 V (+5 %).
+ * Raw reading of 1.1 V is 204 at 5.5 V (+10 %).
+ * @return true if 5 % overvoltage reached
+ */
+bool isVCCOvervoltage() {
+ readVCCVoltageMillivolt();
+ return (sVCCVoltageMillivolt > VCC_OVERVOLTAGE_THRESHOLD_MILLIVOLT);
+}
+bool isVCCOvervoltageSimple() {
+ readVCCVoltageMillivoltSimple();
+ return (sVCCVoltageMillivolt > VCC_OVERVOLTAGE_THRESHOLD_MILLIVOLT);
+}
+
+/*
+ * Temperature sensor is enabled by selecting the appropriate channel.
+ * Different formula for 328P and 328PB!
+ * !!! Function without handling of switched reference and channel.!!!
+ * Use it ONLY if you only use INTERNAL reference (e.g. only call getTemperatureSimple()) in your program.
+ */
+float getCPUTemperatureSimple(void) {
+#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
+ return 0.0;
+#else
+ // use internal 1.1 volt as reference. 4 times oversample. Assume the signal has noise, but never verified :-(
+ uint16_t tTempRaw = readADCChannelWithReferenceOversample(ADC_TEMPERATURE_CHANNEL_MUX, INTERNAL, 2);
+#if defined(LOCAL_DEBUG)
+ Serial.print(F("TempRaw="));
+ Serial.println(tTempRaw);
+#endif
+
+#if defined(__AVR_ATmega328PB__)
+ tTempRaw -= 245;
+ return (float)tTempRaw;
+#else
+ tTempRaw -= 317;
+ return (float) tTempRaw / 1.22;
+#endif
+#endif
+}
+
+/*
+ * Handles usage of 1.1 V reference and channel switching by introducing the appropriate delays.
+ */
+float getTemperature(void) {
+ return getCPUTemperature();
+}
+float getCPUTemperature(void) {
+#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
+ return 0.0;
+#else
+ // use internal 1.1 volt as reference
+ checkAndWaitForReferenceAndChannelToSwitch(ADC_TEMPERATURE_CHANNEL_MUX, INTERNAL);
+ return getCPUTemperatureSimple();
+#endif
+}
+
+#else // defined(ADC_UTILS_ARE_AVAILABLE)
+// Dummy definition of functions defined in ADCUtils to compile examples for non AVR platforms without errors
+/*
+ * Persistent storage for VCC value
+ */
+float sVCCVoltage;
+uint16_t sVCCVoltageMillivolt;
+
+uint16_t getVCCVoltageMillivoltSimple(void){
+ return 3300;
+}
+
+uint16_t readADCChannelWithReferenceOversample(uint8_t aChannelNumber __attribute__((unused)),
+ uint8_t aReference __attribute__((unused)), uint8_t aOversampleExponent __attribute__((unused))) {
+ return 0;
+}
+float getCPUTemperature() {
+ return 20.0;
+}
+float getVCCVoltage() {
+ return 3.3;
+}
+#endif // defined(ADC_UTILS_ARE_AVAILABLE)
+
+#if defined(LOCAL_DEBUG)
+#undef LOCAL_DEBUG
+#endif
+#endif // _ADC_UTILS_HPP
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/AllProtocolsOnLCD.ino b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/AllProtocolsOnLCD.ino
new file mode 100644
index 00000000..6daddec6
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/AllProtocolsOnLCD.ino
@@ -0,0 +1,454 @@
+/*
+ * AllProtocolsOnLCD.cpp
+ *
+ * Modified ReceiveDemo.cpp with additional 1602 LCD output.
+ * If debug button is pressed (pin connected to ground) a long output is generated.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2022-2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 180 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# elif RAMEND <= 0x8FF || RAMSIZE < 0x8FF
+#define RAW_BUFFER_LENGTH 600 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# else
+#define RAW_BUFFER_LENGTH 750 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# endif
+#endif
+
+//#define NO_LED_FEEDBACK_CODE // saves 92 bytes program memory
+#if FLASHEND <= 0x1FFF // For 8k flash or less, like ATtiny85. Exclude exotic protocols.
+#define EXCLUDE_EXOTIC_PROTOCOLS
+# if !defined(DIGISTUMPCORE) // ATTinyCore is bigger than Digispark core
+#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+# endif
+#endif
+//#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+//#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 650 bytes program memory if all other protocols are active
+
+// MARK_EXCESS_MICROS is subtracted from all marks and added to all spaces before decoding,
+// to compensate for the signal forming of different IR receiver modules. See also IRremote.hpp line 142.
+#define MARK_EXCESS_MICROS 20 // Adapt it to your IR receiver module. 20 is recommended for the cheap VS1838 modules.
+
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols.
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+//#define DECODE_NEC // Includes Apple and Onkyo
+
+#include
+
+/*
+ * Activate the type of LCD you use
+ * Default is parallel LCD with 2 rows of 16 characters (1602).
+ * Serial LCD has the disadvantage, that the first repeat is not detected,
+ * because of the long lasting serial communication.
+ */
+//#define USE_NO_LCD
+//#define USE_SERIAL_LCD
+// Definitions for the 1602 LCD
+#define LCD_COLUMNS 16
+#define LCD_ROWS 2
+
+#if defined(USE_SERIAL_LCD)
+#include "LiquidCrystal_I2C.h" // Use an up to date library version, which has the init method
+LiquidCrystal_I2C myLCD(0x27, LCD_COLUMNS, LCD_ROWS); // set the LCD address to 0x27 for a 16 chars and 2 line display
+#elif !defined(USE_NO_LCD)
+#define USE_PARALLEL_LCD
+#include "LiquidCrystal.h"
+//LiquidCrystal myLCD(4, 5, 6, 7, 8, 9);
+LiquidCrystal myLCD(7, 8, 3, 4, 5, 6);
+#endif
+
+#if defined(USE_PARALLEL_LCD)
+#define DEBUG_BUTTON_PIN 11 // If low, print timing for each received data set
+#undef TONE_PIN
+#define TONE_PIN 9 // Pin 4 is used by LCD
+#else
+#define DEBUG_BUTTON_PIN 6
+#endif
+#if defined(__AVR_ATmega328P__)
+#define AUXILIARY_DEBUG_BUTTON_PIN 12 // Is set to low to enable using of a simple connector for enabling debug with pin 11
+#endif
+
+#define MILLIS_BETWEEN_ATTENTION_BEEP 60000 // 60 sec
+uint32_t sMillisOfLastReceivedIRFrame = 0;
+
+#if defined(USE_SERIAL_LCD) || defined(USE_PARALLEL_LCD)
+#define USE_LCD
+# if defined(__AVR__) && defined(ADCSRA) && defined(ADATE)
+// For cyclically display of VCC and isVCCUSBPowered()
+#define VOLTAGE_USB_POWERED_LOWER_THRESHOLD_MILLIVOLT 4250
+#include "ADCUtils.hpp"
+#define MILLIS_BETWEEN_VOLTAGE_PRINT 5000
+#define LCD_VOLTAGE_START_INDEX 11
+uint32_t volatile sMillisOfLastVoltagePrint = 0;
+bool ProtocolStringOverwritesVoltage = false;
+# endif
+#define LCD_IR_COMMAND_START_INDEX 9
+
+void printsVCCVoltageMillivoltOnLCD();
+void printIRResultOnLCD();
+size_t printByteHexOnLCD(uint16_t aHexByteValue);
+void printSpacesOnLCD(uint_fast8_t aNumberOfSpacesToPrint);
+
+#endif // defined(USE_SERIAL_LCD) || defined(USE_PARALLEL_LCD)
+
+void setup() {
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ pinMode(DEBUG_BUTTON_PIN, INPUT_PULLUP);
+# if defined(AUXILIARY_DEBUG_BUTTON_PIN)
+ pinMode(AUXILIARY_DEBUG_BUTTON_PIN, OUTPUT);
+ digitalWrite(AUXILIARY_DEBUG_BUTTON_PIN, LOW); // To use a simple connector to enable debug
+# endif
+#endif
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+// Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ tone(TONE_PIN, 2200);
+ delay(200);
+ noTone(TONE_PIN);
+
+// In case the interrupt driver crashes on setup, give a clue
+// to the user what's going on.
+ Serial.println(F("Enabling IRin..."));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+
+#if defined(USE_SERIAL_LCD)
+ Serial.println(
+ F("With serial LCD connection, the first repeat is not detected, because of the long lasting serial communication!"));
+#endif
+
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ Serial.println();
+ Serial.print(F("If you connect debug pin "));
+# if defined(APPLICATION_PIN_STRING)
+ Serial.print(APPLICATION_PIN_STRING);
+# else
+ Serial.print(DEBUG_BUTTON_PIN);
+# endif
+ Serial.print(F(" to ground"));
+# if defined(AUXILIARY_DEBUG_BUTTON_PIN)
+ Serial.print(F(" or to pin "));
+ Serial.print(AUXILIARY_DEBUG_BUTTON_PIN);
+#endif
+ Serial.println(F(", raw data is always printed"));
+
+ // infos for receive
+ Serial.print(RECORD_GAP_MICROS);
+ Serial.println(F(" us is the (minimum) gap, after which the start of a new IR packet is assumed"));
+ Serial.print(MARK_EXCESS_MICROS);
+ Serial.println(F(" us are subtracted from all marks and added to all spaces for decoding"));
+#endif
+
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ readVCCVoltageMillivolt();
+#endif
+
+#if defined(USE_SERIAL_LCD)
+ myLCD.init();
+ myLCD.clear();
+ myLCD.backlight(); // Switch backlight LED on
+#endif
+#if defined(USE_PARALLEL_LCD)
+ myLCD.begin(LCD_COLUMNS, LCD_ROWS); // This also clears display
+#endif
+
+#if defined(USE_LCD)
+ myLCD.setCursor(0, 0);
+ myLCD.print(F("IRRemote v" VERSION_IRREMOTE));
+ myLCD.setCursor(0, 1);
+ myLCD.print(F(__DATE__));
+#endif
+
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ readVCCVoltageMillivolt();
+#endif
+}
+
+void loop() {
+ /*
+ * Check if received data is available and if yes, try to decode it.
+ * Decoded result is in the IrReceiver.decodedIRData structure.
+ *
+ * E.g. command is in IrReceiver.decodedIRData.command
+ * address is in command is in IrReceiver.decodedIRData.address
+ * and up to 32 bit raw data in IrReceiver.decodedIRData.decodedRawData
+ */
+ if (IrReceiver.decode()) {
+ Serial.println();
+ // Print a short summary of received data
+ IrReceiver.printIRResultShort(&Serial);
+
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_WAS_OVERFLOW) {
+ Serial.println(F("Try to increase the \"RAW_BUFFER_LENGTH\" value of " STR(RAW_BUFFER_LENGTH) " in " __FILE__));
+#if defined(USE_LCD)
+ myLCD.setCursor(0, 0);
+ myLCD.print(F("Overflow "));
+#endif
+
+ // see also https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library
+
+ } else {
+ // play tone
+ auto tStartMillis = millis();
+ IrReceiver.stop();
+ tone(TONE_PIN, 2200);
+
+ if ((IrReceiver.decodedIRData.protocol == UNKNOWN || digitalRead(DEBUG_BUTTON_PIN) == LOW)
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ || isVCCUSBPowered()
+#endif
+ ) {
+ // Print more info, but only if we are connected to USB, i.e. VCC is > 4300 mV, because this may take to long to detect some fast repeats
+ IrReceiver.printIRSendUsage(&Serial);
+ IrReceiver.printIRResultRawFormatted(&Serial, false); // print ticks, this is faster :-)
+ }
+
+ // Guarantee at least 5 millis for tone. decode starts 5 millis (RECORD_GAP_MICROS) after end of frame
+ // so here we are 10 millis after end of frame. Sony20 has only a 12 ms repeat gap.
+ while ((millis() - tStartMillis) < 5)
+ ;
+ noTone(TONE_PIN);
+
+ // Restore IR timer. millis() - tStartMillis to compensate for stop of receiver. This enables a correct gap measurement.
+ IrReceiver.startWithTicksToAdd((millis() - tStartMillis) * (MICROS_IN_ONE_MILLI / MICROS_PER_TICK));
+
+#if defined(USE_LCD)
+ printIRResultOnLCD();
+#endif
+ }
+
+ /*
+ * !!!Important!!! Enable receiving of the next value,
+ * since receiving has stopped after the end of the current received data packet.
+ */
+ IrReceiver.resume();
+ } // if (IrReceiver.decode())
+
+ /*
+ * Check if generating attention beep every minute, after the current measurement was finished
+ */
+ if ((millis() - sMillisOfLastReceivedIRFrame) >= MILLIS_BETWEEN_ATTENTION_BEEP
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ && !isVCCUSBPowered()
+#endif
+ ) {
+ sMillisOfLastReceivedIRFrame = millis();
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ printsVCCVoltageMillivoltOnLCD();
+#endif
+ IrReceiver.stop();
+ tone(TONE_PIN, 2200);
+ delay(50);
+ noTone(TONE_PIN);
+ IrReceiver.startWithTicksToAdd(50 * (MICROS_IN_ONE_MILLI / MICROS_PER_TICK));
+ }
+
+#if defined(USE_LCD) && defined(ADC_UTILS_ARE_AVAILABLE)
+ //Periodically print VCC
+ if (!ProtocolStringOverwritesVoltage && millis() - sMillisOfLastVoltagePrint > MILLIS_BETWEEN_VOLTAGE_PRINT) {
+ /*
+ * Periodically print VCC
+ */
+ sMillisOfLastVoltagePrint = millis();
+ readVCCVoltageMillivolt();
+ printsVCCVoltageMillivoltOnLCD();
+ }
+#endif
+
+}
+
+#if defined(USE_LCD)
+void printsVCCVoltageMillivoltOnLCD() {
+# if defined(ADC_UTILS_ARE_AVAILABLE)
+ char tVoltageString[5];
+ dtostrf(sVCCVoltageMillivolt / 1000.0, 4, 2, tVoltageString);
+ myLCD.setCursor(LCD_VOLTAGE_START_INDEX - 1, 0);
+ myLCD.print(' ');
+ myLCD.print(tVoltageString);
+ myLCD.print('V');
+# endif
+}
+
+/*
+ * LCD output for 1602 LCDs
+ * 40 - 55 Milliseconds per initial output
+ * The expander runs at 100 kHz :-(
+ * 8 milliseconds for 8 bit; 10 ms for 16 bit code output
+ * 3 milliseconds for repeat output
+ *
+ */
+void printIRResultOnLCD() {
+ static uint16_t sLastProtocolIndex = 4711;
+ static uint16_t sLastProtocolAddress = 4711;
+ static uint16_t sLastCommand = 0;
+ static uint8_t sLastCommandPrintPosition;
+
+ /*
+ * Print only if protocol has changed
+ */
+ if (sLastProtocolIndex != IrReceiver.decodedIRData.protocol) {
+ sLastProtocolIndex = IrReceiver.decodedIRData.protocol;
+ /*
+ * Show protocol name and handle overwrite over voltage display
+ */
+ myLCD.setCursor(0, 0);
+ uint_fast8_t tProtocolStringLength = myLCD.print(getProtocolString(IrReceiver.decodedIRData.protocol));
+# if defined(__AVR__) && defined(ADCSRA) && defined(ADATE)
+ if (tProtocolStringLength > LCD_VOLTAGE_START_INDEX) {
+ // we overwrite the voltage -> clear rest of line and inhibit new printing of voltage
+ ProtocolStringOverwritesVoltage = true;
+ if (tProtocolStringLength < LCD_COLUMNS) {
+ printSpacesOnLCD(LCD_COLUMNS - tProtocolStringLength);
+ }
+ } else {
+ // Trigger printing of VCC in main loop
+ sMillisOfLastVoltagePrint = 0;
+ ProtocolStringOverwritesVoltage = false;
+ printSpacesOnLCD(LCD_VOLTAGE_START_INDEX - tProtocolStringLength);
+ }
+# else
+ printSpacesOnLCD(LCD_COLUMNS - tProtocolStringLength);
+# endif
+ }
+
+ if (IrReceiver.decodedIRData.protocol == UNKNOWN) {
+ /*
+ * Print number of bits received and microseconds of signal
+ */
+ myLCD.setCursor(0, 1);
+ uint8_t tNumberOfBits = (IrReceiver.decodedIRData.rawDataPtr->rawlen + 1) / 2;
+ if (tNumberOfBits < 10) {
+ myLCD.print(' '); // padding space
+ }
+ myLCD.print(tNumberOfBits);
+ myLCD.print(F(" bit "));
+ uint_fast8_t tDurationStringLength = myLCD.print(IrReceiver.getTotalDurationOfRawData());
+ myLCD.print(F(" \xE4s")); // \xE4 is micro symbol
+ printSpacesOnLCD(7 - tDurationStringLength);
+ sLastProtocolAddress = 4711;
+ sLastCommand = 44711;
+
+ } else {
+ /*
+ * Print only if address has changed
+ */
+ if (sLastProtocolAddress != IrReceiver.decodedIRData.address) {
+ sLastProtocolAddress = IrReceiver.decodedIRData.address;
+
+ myLCD.setCursor(0, 1);
+ /*
+ * Show address
+ */
+# if defined(DECODE_DISTANCE_WIDTH)
+ if (IrReceiver.decodedIRData.protocol == PULSE_DISTANCE || IrReceiver.decodedIRData.protocol == PULSE_WIDTH) {
+ myLCD.print(F("[0]=0x"));
+ uint_fast8_t tAddressStringLength = myLCD.print(IrReceiver.decodedIRData.decodedRawDataArray[0], HEX);
+ printSpacesOnLCD(LCD_COLUMNS - tAddressStringLength);
+ sLastCommand = 0; // to trigger restoration of "C=" string
+ return; // no command here
+ } else {
+# endif
+ myLCD.print(F("A="));
+ uint_fast8_t tAddressStringLength = printByteHexOnLCD(IrReceiver.decodedIRData.address);
+ printSpacesOnLCD((LCD_IR_COMMAND_START_INDEX - 2) - tAddressStringLength);
+# if defined(DECODE_DISTANCE_WIDTH)
+ }
+# endif
+ }
+
+ /*
+ * Print command always
+ */
+ uint16_t tCommand = IrReceiver.decodedIRData.command;
+
+// Check if prefix position must change
+ if (sLastCommand == 0 || (sLastCommand >= 0x100 && tCommand < 0x100) || (sLastCommand < 0x100 && tCommand >= 0x100)) {
+ sLastCommand = tCommand;
+ /*
+ * Print prefix for 8/16 bit commands
+ */
+ if (tCommand >= 0x100) {
+ // Do not print "C=" here to have 2 additional characters for command
+ sLastCommandPrintPosition = 9;
+ } else {
+ myLCD.setCursor(LCD_IR_COMMAND_START_INDEX, 1);
+ myLCD.print(F("C="));
+ sLastCommandPrintPosition = 11;
+ }
+ }
+
+ /*
+ * Command data
+ */
+ myLCD.setCursor(sLastCommandPrintPosition, 1);
+ printByteHexOnLCD(tCommand);
+
+ /*
+ * Show or clear repetition flag
+ */
+ if (IrReceiver.decodedIRData.flags & (IRDATA_FLAGS_IS_REPEAT)) {
+ myLCD.print('R');
+ return; // Since it is a repetition, printed data has not changed
+ } else {
+ myLCD.print(' ');
+ }
+ } // IrReceiver.decodedIRData.protocol == UNKNOWN
+}
+
+size_t printByteHexOnLCD(uint16_t aHexByteValue) {
+ myLCD.print(F("0x"));
+ size_t tPrintSize = 2;
+ if (aHexByteValue < 0x10 || (aHexByteValue > 0x100 && aHexByteValue < 0x1000)) {
+ myLCD.print('0'); // leading 0
+ tPrintSize++;
+ }
+ return myLCD.print(aHexByteValue, HEX) + tPrintSize;
+}
+
+void printSpacesOnLCD(uint_fast8_t aNumberOfSpacesToPrint) {
+ for (uint_fast8_t i = 0; i < aNumberOfSpacesToPrint; ++i) {
+ myLCD.print(' ');
+ }
+}
+#endif // defined(USE_LCD)
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.cpp b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.cpp
new file mode 100644
index 00000000..5dcef083
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.cpp
@@ -0,0 +1,326 @@
+#include "LiquidCrystal.h"
+
+#include
+#include
+#include
+#include "Arduino.h"
+
+// When the display powers up, it is configured as follows:
+//
+// 1. Display clear
+// 2. Function set:
+// DL = 1; 8-bit interface data
+// N = 0; 1-line display
+// F = 0; 5x8 dot character font
+// 3. Display on/off control:
+// D = 0; Display off
+// C = 0; Cursor off
+// B = 0; Blinking off
+// 4. Entry mode set:
+// I/D = 1; Increment by 1
+// S = 0; No shift
+//
+// Note, however, that resetting the Arduino doesn't reset the LCD, so we
+// can't assume that its in that state when a sketch starts (and the
+// LiquidCrystal constructor is called).
+
+LiquidCrystal::LiquidCrystal(uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7)
+{
+ init(0, rs, rw, enable, d0, d1, d2, d3, d4, d5, d6, d7);
+}
+
+LiquidCrystal::LiquidCrystal(uint8_t rs, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7)
+{
+ init(0, rs, 255, enable, d0, d1, d2, d3, d4, d5, d6, d7);
+}
+
+LiquidCrystal::LiquidCrystal(uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3)
+{
+ init(1, rs, rw, enable, d0, d1, d2, d3, 0, 0, 0, 0);
+}
+
+LiquidCrystal::LiquidCrystal(uint8_t rs, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3)
+{
+ init(1, rs, 255, enable, d0, d1, d2, d3, 0, 0, 0, 0);
+}
+
+void LiquidCrystal::init(uint8_t fourbitmode, uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7)
+{
+ _rs_pin = rs;
+ _rw_pin = rw;
+ _enable_pin = enable;
+
+ _data_pins[0] = d0;
+ _data_pins[1] = d1;
+ _data_pins[2] = d2;
+ _data_pins[3] = d3;
+ _data_pins[4] = d4;
+ _data_pins[5] = d5;
+ _data_pins[6] = d6;
+ _data_pins[7] = d7;
+
+ if (fourbitmode)
+ _displayfunction = LCD_4BITMODE | LCD_1LINE | LCD_5x8DOTS;
+ else
+ _displayfunction = LCD_8BITMODE | LCD_1LINE | LCD_5x8DOTS;
+
+ begin(16, 1);
+}
+
+void LiquidCrystal::begin(uint8_t cols, uint8_t lines, uint8_t dotsize) {
+ if (lines > 1) {
+ _displayfunction |= LCD_2LINE;
+ }
+ _numlines = lines;
+
+ setRowOffsets(0x00, 0x40, 0x00 + cols, 0x40 + cols);
+
+ // for some 1 line displays you can select a 10 pixel high font
+ if ((dotsize != LCD_5x8DOTS) && (lines == 1)) {
+ _displayfunction |= LCD_5x10DOTS;
+ }
+
+ pinMode(_rs_pin, OUTPUT);
+ // we can save 1 pin by not using RW. Indicate by passing 255 instead of pin#
+ if (_rw_pin != 255) {
+ pinMode(_rw_pin, OUTPUT);
+ }
+ pinMode(_enable_pin, OUTPUT);
+
+ // Do these once, instead of every time a character is drawn for speed reasons.
+ for (int i=0; i<((_displayfunction & LCD_8BITMODE) ? 8 : 4); ++i)
+ {
+ pinMode(_data_pins[i], OUTPUT);
+ }
+
+ // SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION!
+ // according to datasheet, we need at least 40ms after power rises above 2.7V
+ // before sending commands. Arduino can turn on way before 4.5V so we'll wait 50
+ delayMicroseconds(50000);
+ // Now we pull both RS and R/W low to begin commands
+ digitalWrite(_rs_pin, LOW);
+ digitalWrite(_enable_pin, LOW);
+ if (_rw_pin != 255) {
+ digitalWrite(_rw_pin, LOW);
+ }
+
+ //put the LCD into 4 bit or 8 bit mode
+ if (! (_displayfunction & LCD_8BITMODE)) {
+ // this is according to the hitachi HD44780 datasheet
+ // figure 24, pg 46
+
+ // we start in 8bit mode, try to set 4 bit mode
+ write4bits(0x03);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // second try
+ write4bits(0x03);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // third go!
+ write4bits(0x03);
+ delayMicroseconds(150);
+
+ // finally, set to 4-bit interface
+ write4bits(0x02);
+ } else {
+ // this is according to the hitachi HD44780 datasheet
+ // page 45 figure 23
+
+ // Send function set command sequence
+ command(LCD_FUNCTIONSET | _displayfunction);
+ delayMicroseconds(4500); // wait more than 4.1ms
+
+ // second try
+ command(LCD_FUNCTIONSET | _displayfunction);
+ delayMicroseconds(150);
+
+ // third go
+ command(LCD_FUNCTIONSET | _displayfunction);
+ }
+
+ // finally, set # lines, font size, etc.
+ command(LCD_FUNCTIONSET | _displayfunction);
+
+ // turn the display on with no cursor or blinking default
+ _displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF;
+ display();
+
+ // clear it off
+ clear();
+
+ // Initialize to default text direction (for romance languages)
+ _displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT;
+ // set the entry mode
+ command(LCD_ENTRYMODESET | _displaymode);
+
+}
+
+void LiquidCrystal::setRowOffsets(int row0, int row1, int row2, int row3)
+{
+ _row_offsets[0] = row0;
+ _row_offsets[1] = row1;
+ _row_offsets[2] = row2;
+ _row_offsets[3] = row3;
+}
+
+/********** high level commands, for the user! */
+void LiquidCrystal::clear()
+{
+ command(LCD_CLEARDISPLAY); // clear display, set cursor position to zero
+ delayMicroseconds(2000); // this command takes a long time!
+}
+
+void LiquidCrystal::home()
+{
+ command(LCD_RETURNHOME); // set cursor position to zero
+ delayMicroseconds(2000); // this command takes a long time!
+}
+
+void LiquidCrystal::setCursor(uint8_t col, uint8_t row)
+{
+ const size_t max_lines = sizeof(_row_offsets) / sizeof(*_row_offsets);
+ if ( row >= max_lines ) {
+ row = max_lines - 1; // we count rows starting w/0
+ }
+ if ( row >= _numlines ) {
+ row = _numlines - 1; // we count rows starting w/0
+ }
+
+ command(LCD_SETDDRAMADDR | (col + _row_offsets[row]));
+}
+
+// Turn the display on/off (quickly)
+void LiquidCrystal::noDisplay() {
+ _displaycontrol &= ~LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal::display() {
+ _displaycontrol |= LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turns the underline cursor on/off
+void LiquidCrystal::noCursor() {
+ _displaycontrol &= ~LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal::cursor() {
+ _displaycontrol |= LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turn on and off the blinking cursor
+void LiquidCrystal::noBlink() {
+ _displaycontrol &= ~LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal::blink() {
+ _displaycontrol |= LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// These commands scroll the display without changing the RAM
+void LiquidCrystal::scrollDisplayLeft(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVELEFT);
+}
+void LiquidCrystal::scrollDisplayRight(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVERIGHT);
+}
+
+// This is for text that flows Left to Right
+void LiquidCrystal::leftToRight(void) {
+ _displaymode |= LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This is for text that flows Right to Left
+void LiquidCrystal::rightToLeft(void) {
+ _displaymode &= ~LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'right justify' text from the cursor
+void LiquidCrystal::autoscroll(void) {
+ _displaymode |= LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'left justify' text from the cursor
+void LiquidCrystal::noAutoscroll(void) {
+ _displaymode &= ~LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// Allows us to fill the first 8 CGRAM locations
+// with custom characters
+void LiquidCrystal::createChar(uint8_t location, uint8_t charmap[]) {
+ location &= 0x7; // we only have 8 locations 0-7
+ command(LCD_SETCGRAMADDR | (location << 3));
+ for (int i=0; i<8; i++) {
+ write(charmap[i]);
+ }
+}
+
+/*********** mid level commands, for sending data/cmds */
+
+inline void LiquidCrystal::command(uint8_t value) {
+ send(value, LOW);
+}
+
+inline size_t LiquidCrystal::write(uint8_t value) {
+ send(value, HIGH);
+ return 1; // assume sucess
+}
+
+/************ low level data pushing commands **********/
+
+// write either command or data, with automatic 4/8-bit selection
+void LiquidCrystal::send(uint8_t value, uint8_t mode) {
+ digitalWrite(_rs_pin, mode);
+
+ // if there is a RW pin indicated, set it low to Write
+ if (_rw_pin != 255) {
+ digitalWrite(_rw_pin, LOW);
+ }
+
+ if (_displayfunction & LCD_8BITMODE) {
+ write8bits(value);
+ } else {
+ write4bits(value>>4);
+ write4bits(value);
+ }
+}
+
+void LiquidCrystal::pulseEnable(void) {
+ digitalWrite(_enable_pin, LOW);
+ delayMicroseconds(1);
+ digitalWrite(_enable_pin, HIGH);
+ delayMicroseconds(1); // enable pulse must be >450ns
+ digitalWrite(_enable_pin, LOW);
+ delayMicroseconds(100); // commands need > 37us to settle
+}
+
+void LiquidCrystal::write4bits(uint8_t value) {
+ for (int i = 0; i < 4; i++) {
+ digitalWrite(_data_pins[i], (value >> i) & 0x01);
+ }
+
+ pulseEnable();
+}
+
+void LiquidCrystal::write8bits(uint8_t value) {
+ for (int i = 0; i < 8; i++) {
+ digitalWrite(_data_pins[i], (value >> i) & 0x01);
+ }
+
+ pulseEnable();
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.h b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.h
new file mode 100644
index 00000000..07206242
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal.h
@@ -0,0 +1,108 @@
+#ifndef LiquidCrystal_h
+#define LiquidCrystal_h
+
+#include
+#include "Print.h"
+
+// commands
+#define LCD_CLEARDISPLAY 0x01
+#define LCD_RETURNHOME 0x02
+#define LCD_ENTRYMODESET 0x04
+#define LCD_DISPLAYCONTROL 0x08
+#define LCD_CURSORSHIFT 0x10
+#define LCD_FUNCTIONSET 0x20
+#define LCD_SETCGRAMADDR 0x40
+#define LCD_SETDDRAMADDR 0x80
+
+// flags for display entry mode
+#define LCD_ENTRYRIGHT 0x00
+#define LCD_ENTRYLEFT 0x02
+#define LCD_ENTRYSHIFTINCREMENT 0x01
+#define LCD_ENTRYSHIFTDECREMENT 0x00
+
+// flags for display on/off control
+#define LCD_DISPLAYON 0x04
+#define LCD_DISPLAYOFF 0x00
+#define LCD_CURSORON 0x02
+#define LCD_CURSOROFF 0x00
+#define LCD_BLINKON 0x01
+#define LCD_BLINKOFF 0x00
+
+// flags for display/cursor shift
+#define LCD_DISPLAYMOVE 0x08
+#define LCD_CURSORMOVE 0x00
+#define LCD_MOVERIGHT 0x04
+#define LCD_MOVELEFT 0x00
+
+// flags for function set
+#define LCD_8BITMODE 0x10
+#define LCD_4BITMODE 0x00
+#define LCD_2LINE 0x08
+#define LCD_1LINE 0x00
+#define LCD_5x10DOTS 0x04
+#define LCD_5x8DOTS 0x00
+
+class LiquidCrystal : public Print {
+public:
+ LiquidCrystal(uint8_t rs, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7);
+ LiquidCrystal(uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7);
+ LiquidCrystal(uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3);
+ LiquidCrystal(uint8_t rs, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3);
+
+ void init(uint8_t fourbitmode, uint8_t rs, uint8_t rw, uint8_t enable,
+ uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
+ uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7);
+
+ void begin(uint8_t cols, uint8_t rows, uint8_t charsize = LCD_5x8DOTS);
+
+ void clear();
+ void home();
+
+ void noDisplay();
+ void display();
+ void noBlink();
+ void blink();
+ void noCursor();
+ void cursor();
+ void scrollDisplayLeft();
+ void scrollDisplayRight();
+ void leftToRight();
+ void rightToLeft();
+ void autoscroll();
+ void noAutoscroll();
+
+ void setRowOffsets(int row1, int row2, int row3, int row4);
+ void createChar(uint8_t, uint8_t[]);
+ void setCursor(uint8_t, uint8_t);
+ virtual size_t write(uint8_t);
+ void command(uint8_t);
+
+ using Print::write;
+private:
+ void send(uint8_t, uint8_t);
+ void write4bits(uint8_t);
+ void write8bits(uint8_t);
+ void pulseEnable();
+
+ uint8_t _rs_pin; // LOW: command. HIGH: character.
+ uint8_t _rw_pin; // LOW: write to LCD. HIGH: read from LCD.
+ uint8_t _enable_pin; // activated by a HIGH pulse.
+ uint8_t _data_pins[8];
+
+ uint8_t _displayfunction;
+ uint8_t _displaycontrol;
+ uint8_t _displaymode;
+
+ uint8_t _initialized;
+
+ uint8_t _numlines;
+ uint8_t _row_offsets[4];
+};
+
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.cpp b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.cpp
new file mode 100644
index 00000000..2659ef41
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.cpp
@@ -0,0 +1,344 @@
+// Based on the work by DFRobot
+
+#include "Arduino.h"
+
+#if defined(__AVR__) && !defined(USE_SOFT_I2C_MASTER) && __has_include("SoftI2CMasterConfig.h")
+#define USE_SOFT_I2C_MASTER // must be before #include "LiquidCrystal_I2C.h"
+#endif
+
+#include "LiquidCrystal_I2C.h"
+#include
+
+inline size_t LiquidCrystal_I2C::write(uint8_t value) {
+ send(value, Rs);
+ return 1;
+}
+
+#if defined(USE_SOFT_I2C_MASTER)
+//#define USE_SOFT_I2C_MASTER_H_AS_PLAIN_INCLUDE
+#include "SoftI2CMasterConfig.h" // Include configuration for sources
+#include "SoftI2CMaster.h" // include sources
+#elif defined(USE_SOFT_WIRE)
+#define USE_SOFTWIRE_H_AS_PLAIN_INCLUDE
+#include "SoftWire.h"
+#endif
+
+// When the display powers up, it is configured as follows:
+//
+// 1. Display clear
+// 2. Function set:
+// DL = 1; 8-bit interface data
+// N = 0; 1-line display
+// F = 0; 5x8 dot character font
+// 3. Display on/off control:
+// D = 0; Display off
+// C = 0; Cursor off
+// B = 0; Blinking off
+// 4. Entry mode set:
+// I/D = 1; Increment by 1
+// S = 0; No shift
+//
+// Note, however, that resetting the Arduino doesn't reset the LCD, so we
+// can't assume that its in that state when a sketch starts (and the
+// LiquidCrystal constructor is called).
+
+LiquidCrystal_I2C::LiquidCrystal_I2C(uint8_t lcd_Addr, uint8_t lcd_cols, uint8_t lcd_rows) {
+ _Addr = lcd_Addr;
+ _cols = lcd_cols;
+ _rows = lcd_rows;
+ _backlightval = LCD_NOBACKLIGHT;
+ _oled = false;
+}
+
+void LiquidCrystal_I2C::oled_init() {
+ _oled = true;
+ init_priv();
+}
+
+void LiquidCrystal_I2C::init() {
+ init_priv();
+}
+
+void LiquidCrystal_I2C::init_priv() {
+#if defined(USE_SOFT_I2C_MASTER)
+ i2c_init();
+#else
+ Wire.begin();
+#endif
+ _displayfunction = LCD_4BITMODE | LCD_1LINE | LCD_5x8DOTS;
+ begin(_cols, _rows);
+}
+
+void LiquidCrystal_I2C::begin(uint8_t cols __attribute__((unused)), uint8_t lines, uint8_t dotsize) {
+ if (lines > 1) {
+ _displayfunction |= LCD_2LINE;
+ }
+ _numlines = lines;
+
+ // for some 1 line displays you can select a 10 pixel high font
+ if ((dotsize != 0) && (lines == 1)) {
+ _displayfunction |= LCD_5x10DOTS;
+ }
+
+ // SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION!
+ // according to datasheet, we need at least 40ms after power rises above 2.7V
+ // before sending commands. Arduino can turn on way before 4.5V so we'll wait 50
+ delay(50);
+
+ // Now we pull both RS and R/W low to begin commands
+ expanderWrite(_backlightval); // reset expander and turn backlight off (Bit 8 =1)
+ delay(1000);
+
+ //put the LCD into 4 bit mode
+ // this is according to the hitachi HD44780 datasheet
+ // figure 24, pg 46
+
+ // we start in 8bit mode, try to set 4 bit mode
+ write4bits(0x03 << 4);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // second try
+ write4bits(0x03 << 4);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // third go!
+ write4bits(0x03 << 4);
+ delayMicroseconds(150);
+
+ // finally, set to 4-bit interface
+ write4bits(0x02 << 4);
+
+ // set # lines, font size, etc.
+ command(LCD_FUNCTIONSET | _displayfunction);
+
+ // turn the display on with no cursor or blinking default
+ _displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF;
+ display();
+
+ // clear it off
+ clear();
+
+ // Initialize to default text direction (for roman languages)
+ _displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT;
+
+ // set the entry mode
+ command(LCD_ENTRYMODESET | _displaymode);
+
+ home();
+
+}
+
+/********** high level commands, for the user! */
+void LiquidCrystal_I2C::clear() {
+ command(LCD_CLEARDISPLAY); // clear display, set cursor position to zero
+ delayMicroseconds(1500); // this command takes a long time! // AJ 20.9.23 1200 is too short for my 2004 LCD's, 1400 is OK
+ if (_oled)
+ setCursor(0, 0);
+}
+
+void LiquidCrystal_I2C::home() {
+ command(LCD_RETURNHOME); // set cursor position to zero
+ delayMicroseconds(2000); // this command takes a long time!
+}
+
+void LiquidCrystal_I2C::setCursor(uint8_t col, uint8_t row) {
+ int row_offsets[] = { 0x00, 0x40, 0x14, 0x54 };
+ if (row > _numlines) {
+ row = _numlines - 1; // we count rows starting w/0
+ }
+ command(LCD_SETDDRAMADDR | (col + row_offsets[row]));
+}
+
+// Turn the display on/off (quickly)
+void LiquidCrystal_I2C::noDisplay() {
+ _displaycontrol &= ~LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::display() {
+ _displaycontrol |= LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turns the underline cursor on/off
+void LiquidCrystal_I2C::noCursor() {
+ _displaycontrol &= ~LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::cursor() {
+ _displaycontrol |= LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turn on and off the blinking cursor
+void LiquidCrystal_I2C::noBlink() {
+ _displaycontrol &= ~LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::blink() {
+ _displaycontrol |= LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// These commands scroll the display without changing the RAM
+void LiquidCrystal_I2C::scrollDisplayLeft(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVELEFT);
+}
+void LiquidCrystal_I2C::scrollDisplayRight(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVERIGHT);
+}
+
+// This is for text that flows Left to Right
+void LiquidCrystal_I2C::leftToRight(void) {
+ _displaymode |= LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This is for text that flows Right to Left
+void LiquidCrystal_I2C::rightToLeft(void) {
+ _displaymode &= ~LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'right justify' text from the cursor
+void LiquidCrystal_I2C::autoscroll(void) {
+ _displaymode |= LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'left justify' text from the cursor
+void LiquidCrystal_I2C::noAutoscroll(void) {
+ _displaymode &= ~LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// Allows us to fill the first 8 CGRAM locations
+// with custom characters
+void LiquidCrystal_I2C::createChar(uint8_t location, uint8_t charmap[]) {
+ location &= 0x7; // we only have 8 locations 0-7
+ command(LCD_SETCGRAMADDR | (location << 3));
+ for (int i = 0; i < 8; i++) {
+ write(charmap[i]);
+ }
+}
+
+//createChar with PROGMEM input
+void LiquidCrystal_I2C::createChar(uint8_t location, const char *charmap) {
+ location &= 0x7; // we only have 8 locations 0-7
+ command(LCD_SETCGRAMADDR | (location << 3));
+ for (int i = 0; i < 8; i++) {
+ write(pgm_read_byte_near(charmap++));
+ }
+}
+
+// Turn the (optional) backlight off/on
+void LiquidCrystal_I2C::noBacklight(void) {
+ _backlightval = LCD_NOBACKLIGHT;
+ expanderWrite(0);
+}
+
+void LiquidCrystal_I2C::backlight(void) {
+ _backlightval = LCD_BACKLIGHT;
+ expanderWrite(0);
+}
+
+/*********** mid level commands, for sending data/cmds */
+
+inline void LiquidCrystal_I2C::command(uint8_t value) {
+ send(value, 0);
+}
+
+/************ low level data pushing commands **********/
+
+// write either command or data
+void LiquidCrystal_I2C::send(uint8_t value, uint8_t mode) {
+ uint8_t highnib = value & 0xf0;
+ uint8_t lownib = (value << 4) & 0xf0;
+ write4bits((highnib) | mode);
+ write4bits((lownib) | mode);
+}
+
+void LiquidCrystal_I2C::write4bits(uint8_t value) {
+ expanderWrite(value);
+ pulseEnable(value);
+}
+
+void LiquidCrystal_I2C::expanderWrite(uint8_t _data) {
+#if defined(USE_SOFT_I2C_MASTER)
+ i2c_write_byte(_Addr << 1, _data | _backlightval);
+#else
+ Wire.beginTransmission(_Addr);
+ Wire.write((int )(_data) | _backlightval);
+ Wire.endTransmission();
+#endif
+}
+
+void LiquidCrystal_I2C::pulseEnable(uint8_t _data) {
+ expanderWrite(_data | En); // En high
+// delayMicroseconds(1); // enable pulse must be >450ns // AJ 20.9.23 not required for my LCD's
+
+ expanderWrite(_data & ~En); // En low
+// delayMicroseconds(50); // commands need > 37us to settle // AJ 20.9.23 not required for my LCD's
+}
+
+// Alias functions
+
+void LiquidCrystal_I2C::cursor_on() {
+ cursor();
+}
+
+void LiquidCrystal_I2C::cursor_off() {
+ noCursor();
+}
+
+void LiquidCrystal_I2C::blink_on() {
+ blink();
+}
+
+void LiquidCrystal_I2C::blink_off() {
+ noBlink();
+}
+
+void LiquidCrystal_I2C::load_custom_character(uint8_t char_num, uint8_t *rows) {
+ createChar(char_num, rows);
+}
+
+void LiquidCrystal_I2C::setBacklight(uint8_t new_val) {
+ if (new_val) {
+ backlight(); // turn backlight on
+ } else {
+ noBacklight(); // turn backlight off
+ }
+}
+
+void LiquidCrystal_I2C::printstr(const char c[]) {
+ //This function is not identical to the function used for "real" I2C displays
+ //it's here so the user sketch doesn't have to be changed
+ print(c);
+}
+
+// unsupported API functions
+#pragma GCC diagnostic push
+#pragma GCC diagnostic ignored "-Wunused-parameter"
+void LiquidCrystal_I2C::off() {
+}
+void LiquidCrystal_I2C::on() {
+}
+void LiquidCrystal_I2C::setDelay(int cmdDelay, int charDelay) {
+}
+uint8_t LiquidCrystal_I2C::status() {
+ return 0;
+}
+uint8_t LiquidCrystal_I2C::keypad() {
+ return 0;
+}
+uint8_t LiquidCrystal_I2C::init_bargraph(uint8_t graphtype) {
+ return 0;
+}
+void LiquidCrystal_I2C::draw_horizontal_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end) {
+}
+void LiquidCrystal_I2C::draw_vertical_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_row_end) {
+}
+void LiquidCrystal_I2C::setContrast(uint8_t new_val) {
+}
+#pragma GCC diagnostic pop
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.h b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.h
new file mode 100644
index 00000000..420214a4
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/LiquidCrystal_I2C.h
@@ -0,0 +1,129 @@
+//YWROBOT
+#ifndef LiquidCrystal_I2C_h
+#define LiquidCrystal_I2C_h
+
+#include
+#include "Print.h"
+#if !defined(USE_SOFT_I2C_MASTER) && !defined(USE_SOFT_WIRE)
+#include
+#endif
+
+// commands
+#define LCD_CLEARDISPLAY 0x01
+#define LCD_RETURNHOME 0x02
+#define LCD_ENTRYMODESET 0x04
+#define LCD_DISPLAYCONTROL 0x08
+#define LCD_CURSORSHIFT 0x10
+#define LCD_FUNCTIONSET 0x20
+#define LCD_SETCGRAMADDR 0x40
+#define LCD_SETDDRAMADDR 0x80
+
+// flags for display entry mode
+#define LCD_ENTRYRIGHT 0x00
+#define LCD_ENTRYLEFT 0x02
+#define LCD_ENTRYSHIFTINCREMENT 0x01
+#define LCD_ENTRYSHIFTDECREMENT 0x00
+
+// flags for display on/off control
+#define LCD_DISPLAYON 0x04
+#define LCD_DISPLAYOFF 0x00
+#define LCD_CURSORON 0x02
+#define LCD_CURSOROFF 0x00
+#define LCD_BLINKON 0x01
+#define LCD_BLINKOFF 0x00
+
+// flags for display/cursor shift
+#define LCD_DISPLAYMOVE 0x08
+#define LCD_CURSORMOVE 0x00
+#define LCD_MOVERIGHT 0x04
+#define LCD_MOVELEFT 0x00
+
+// flags for function set
+#define LCD_8BITMODE 0x10
+#define LCD_4BITMODE 0x00
+#define LCD_2LINE 0x08
+#define LCD_1LINE 0x00
+#define LCD_5x10DOTS 0x04
+#define LCD_5x8DOTS 0x00
+
+// flags for backlight control
+#define LCD_BACKLIGHT 0x08
+#define LCD_NOBACKLIGHT 0x00
+
+#define En 0b00000100 // Enable bit
+#define Rw 0b00000010 // Read/Write bit
+#define Rs 0b00000001 // Register select bit
+
+class LiquidCrystal_I2C : public Print {
+public:
+ LiquidCrystal_I2C(uint8_t lcd_Addr,uint8_t lcd_cols,uint8_t lcd_rows);
+ void begin(uint8_t cols, uint8_t rows, uint8_t charsize = LCD_5x8DOTS );
+ void clear();
+ void home();
+ void noDisplay();
+ void display();
+ void noBlink();
+ void blink();
+ void noCursor();
+ void cursor();
+ void scrollDisplayLeft();
+ void scrollDisplayRight();
+ void printLeft();
+ void printRight();
+ void leftToRight();
+ void rightToLeft();
+ void shiftIncrement();
+ void shiftDecrement();
+ void noBacklight();
+ void backlight();
+ void autoscroll();
+ void noAutoscroll();
+ void createChar(uint8_t, uint8_t[]);
+ void createChar(uint8_t location, const char *charmap);
+ // Example: const char bell[8] PROGMEM = {B00100,B01110,B01110,B01110,B11111,B00000,B00100,B00000};
+
+ void setCursor(uint8_t, uint8_t);
+ size_t write(uint8_t);
+ void command(uint8_t);
+ void init();
+ void oled_init();
+
+////compatibility API function aliases
+void blink_on(); // alias for blink()
+void blink_off(); // alias for noBlink()
+void cursor_on(); // alias for cursor()
+void cursor_off(); // alias for noCursor()
+void setBacklight(uint8_t new_val); // alias for backlight() and nobacklight()
+void load_custom_character(uint8_t char_num, uint8_t *rows); // alias for createChar()
+void printstr(const char[]);
+
+////Unsupported API functions (not implemented in this library)
+uint8_t status();
+void setContrast(uint8_t new_val);
+uint8_t keypad();
+void setDelay(int,int);
+void on();
+void off();
+uint8_t init_bargraph(uint8_t graphtype);
+void draw_horizontal_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end);
+void draw_vertical_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end);
+
+
+private:
+ void init_priv();
+ void send(uint8_t, uint8_t);
+ void write4bits(uint8_t);
+ void expanderWrite(uint8_t);
+ void pulseEnable(uint8_t);
+ uint8_t _Addr;
+ uint8_t _displayfunction;
+ uint8_t _displaycontrol;
+ uint8_t _displaymode;
+ uint8_t _numlines;
+ bool _oled;
+ uint8_t _cols;
+ uint8_t _rows;
+ uint8_t _backlightval;
+};
+
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/AllProtocolsOnLCD/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/ControlRelay.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/ControlRelay.ino
new file mode 100644
index 00000000..1460872f
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/ControlRelay.ino
@@ -0,0 +1,106 @@
+/*
+ * ControlRelay.cpp
+ *
+ * Toggles an output pin at each command received
+ * An IR detector/demodulator must be connected to the input RECV_PIN.
+ * Initially coded 2009 Ken Shirriff http://www.righto.com
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2009-2021 Ken Shirriff, Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#if FLASHEND <= 0x1FFF || (RAMEND <= 0x4FF || RAMSIZE < 0x4FF) // For 8k flash or 512 bytes RAM or less, like ATtiny85, ATtiny167
+#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+#define EXCLUDE_EXOTIC_PROTOCOLS
+#endif
+
+#include
+
+#if defined(APPLICATION_PIN)
+#define RELAY_PIN APPLICATION_PIN
+#else
+#define RELAY_PIN 5
+#endif
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+ pinMode(RELAY_PIN, OUTPUT);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+}
+
+int on = 0;
+unsigned long last = millis();
+
+void loop() {
+ if (IrReceiver.decode()) {
+ // If it's been at least 1/4 second since the last
+ // IR received, toggle the relay
+ if (millis() - last > 250) {
+ on = !on;
+ Serial.print(F("Switch relay "));
+ if (on) {
+ digitalWrite(RELAY_PIN, HIGH);
+ Serial.println(F("on"));
+ } else {
+ digitalWrite(RELAY_PIN, LOW);
+ Serial.println(F("off"));
+ }
+
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604
+ IrReceiver.printIRResultShort(&Serial);
+ IrReceiver.printIRSendUsage(&Serial);
+ Serial.println();
+ if (IrReceiver.decodedIRData.protocol == UNKNOWN) {
+ // We have an unknown protocol, print more info
+ Serial.println(F("Received noise or an unknown (or not yet enabled) protocol"));
+ IrReceiver.printIRResultRawFormatted(&Serial, true);
+ }
+#else
+ // Print a minimal summary of received data
+ IrReceiver.printIRResultMinimal(&Serial);
+ Serial.println();
+#endif // FLASHEND
+ }
+ last = millis();
+ IrReceiver.resume(); // Enable receiving of the next value
+ }
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ControlRelay/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/DemoIRCommandMapping.h b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/DemoIRCommandMapping.h
new file mode 100644
index 00000000..26110da2
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/DemoIRCommandMapping.h
@@ -0,0 +1,193 @@
+/*
+ * DemoIRCommandMapping.h
+ *
+ * IR remote button codes, strings, and functions to call
+ *
+ * Copyright (C) 2019-2022 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ */
+
+#ifndef _IR_COMMAND_MAPPING_H
+#define _IR_COMMAND_MAPPING_H
+
+#include
+//#include "Commands.h" // includes all the commands used in the mapping arrays below
+
+/*
+ * !!! Choose your remote !!!
+ */
+//#define USE_KEYES_REMOTE_CLONE With number pad and direction control switched, will be taken as default
+//#define USE_KEYES_REMOTE
+#if !defined(USE_KEYES_REMOTE) && !defined(USE_KEYES_REMOTE_CLONE)
+#define USE_KEYES_REMOTE_CLONE // the one you can buy at aliexpress
+#endif
+
+#if (defined(USE_KEYES_REMOTE) && defined(USE_KEYES_REMOTE_CLONE))
+#error "Please choose only one remote for compile"
+#endif
+
+#if defined(USE_KEYES_REMOTE_CLONE)
+#define IR_REMOTE_NAME "KEYES_CLONE"
+// Codes for the KEYES CLONE remote control with 17 keys with number pad above direction control
+#if defined(USE_IRMP_LIBRARY)
+#define IR_ADDRESS 0xFF00 // IRMP interprets NEC addresses always as 16 bit
+#else
+#define IR_ADDRESS 0x00
+#endif
+
+#define IR_UP 0x18
+#define IR_DOWN 0x52
+#define IR_RIGHT 0x5A
+#define IR_LEFT 0x08
+#define IR_OK 0x1C
+
+#define IR_1 0x45
+#define IR_2 0x46
+#define IR_3 0x47
+#define IR_4 0x44
+#define IR_5 0x40
+#define IR_6 0x43
+#define IR_7 0x07
+#define IR_8 0x15
+#define IR_9 0x09
+#define IR_0 0x19
+
+#define IR_STAR 0x16
+#define IR_HASH 0x0D
+/*
+ * SECOND:
+ * IR button to command mapping for better reading. IR buttons should only referenced here.
+ */
+#define COMMAND_ON IR_UP
+#define COMMAND_OFF IR_DOWN
+#define COMMAND_INCREASE_BLINK IR_RIGHT
+#define COMMAND_DECREASE_BLINK IR_LEFT
+
+#define COMMAND_START IR_OK
+#define COMMAND_STOP IR_HASH
+#define COMMAND_RESET IR_STAR
+#define COMMAND_BLINK IR_0
+#define COMMAND_TONE1 IR_1
+
+#define COMMAND_TONE2 IR_2
+#define COMMAND_TONE3 IR_3
+//#define IR_4
+//#define IR_5
+//#define IR_6
+//#define IR_7
+//#define IR_8
+//#define IR_9
+
+#endif
+
+#if defined(USE_KEYES_REMOTE)
+#define IR_REMOTE_NAME "KEYES"
+/*
+ * FIRST:
+ * IR code to button mapping for better reading. IR codes should only referenced here.
+ */
+// Codes for the KEYES remote control with 17 keys and direction control above number pad
+#if defined(USE_IRMP_LIBRARY)
+#define IR_ADDRESS 0xFF00 // IRMP interprets NEC addresses always as 16 bit
+#else
+#define IR_ADDRESS 0x00
+#endif
+
+#define IR_UP 0x46
+#define IR_DOWN 0x15
+#define IR_RIGHT 0x43
+#define IR_LEFT 0x44
+#define IR_OK 0x40
+
+#define IR_1 0x16
+#define IR_2 0x19
+#define IR_3 0x0D
+#define IR_4 0x0C
+#define IR_5 0x18
+#define IR_6 0x5E
+#define IR_7 0x08
+#define IR_8 0x1C
+#define IR_9 0x5A
+#define IR_0 0x52
+
+#define IR_STAR 0x42
+#define IR_HASH 0x4A
+
+/*
+ * SECOND:
+ * IR button to command mapping for better reading. IR buttons should only referenced here.
+ */
+#define COMMAND_ON IR_UP
+#define COMMAND_OFF IR_DOWN
+#define COMMAND_INCREASE_BLINK IR_RIGHT
+#define COMMAND_DECREASE_BLINK IR_LEFT
+
+#define COMMAND_RESET IR_OK
+#define COMMAND_STOP IR_HASH
+#define COMMAND_STOP IR_STAR
+#define COMMAND_BLINK IR_0
+#define COMMAND_TONE2 IR_1
+
+#define COMMAND_TONE1 IR_2
+#define COMMAND_TONE2 IR_3
+#define COMMAND_TONE2 IR_4
+#define COMMAND_TONE2 IR_5
+#define COMMAND_TONE2 IR_6
+#define COMMAND_TONE2 IR_7
+#define COMMAND_TONE2 IR_8
+#define COMMAND_TONE2 IR_9
+#endif
+
+/*
+ * THIRD:
+ * Main mapping of commands to C functions
+ */
+
+// IR strings of functions for output
+static const char LEDon[] PROGMEM ="LED on";
+static const char LEDoff[] PROGMEM ="LED off";
+
+static const char blink20times[] PROGMEM ="blink 20 times";
+static const char blinkStart[] PROGMEM ="blink start";
+
+static const char increaseBlink[] PROGMEM ="increase blink frequency";
+static const char decreaseBlink[] PROGMEM ="decrease blink frequency";
+
+static const char tone2200[] PROGMEM ="tone 2200";
+static const char tone1800[] PROGMEM ="tone 1800";
+static const char printMenu[] PROGMEM ="printMenu";
+
+static const char reset[] PROGMEM ="reset";
+static const char stop[] PROGMEM ="stop";
+
+// not used yet
+static const char test[] PROGMEM ="test";
+static const char pattern[] PROGMEM ="pattern";
+static const char unknown[] PROGMEM ="unknown";
+
+/*
+ * Main mapping array of commands to C functions and command strings
+ */
+const struct IRToCommandMappingStruct IRMapping[] = { /**/
+{ COMMAND_BLINK, IR_COMMAND_FLAG_BLOCKING, &doLedBlink20times, blink20times }, /**/
+{ COMMAND_STOP, IR_COMMAND_FLAG_BLOCKING, &doStop, stop },
+
+/*
+ * Short commands, which can be executed always
+ */
+{ COMMAND_TONE1, IR_COMMAND_FLAG_BLOCKING, &doTone1800, tone1800 }, /**/
+{ COMMAND_TONE3, IR_COMMAND_FLAG_BLOCKING, &doPrintMenu, printMenu }, /**/
+{ COMMAND_ON, IR_COMMAND_FLAG_NON_BLOCKING, &doLedOn, LEDon }, /**/
+{ COMMAND_OFF, IR_COMMAND_FLAG_NON_BLOCKING, &doLedOff, LEDoff }, /**/
+{ COMMAND_START, IR_COMMAND_FLAG_NON_BLOCKING, &doLedBlinkStart, blinkStart }, /**/
+{ COMMAND_RESET, IR_COMMAND_FLAG_NON_BLOCKING, &doResetBlinkFrequency, reset },
+
+/*
+ * Repeatable short commands
+ */
+{ COMMAND_TONE2, IR_COMMAND_FLAG_REPEATABLE_NON_BLOCKING, &doTone2200, tone2200 }, /**/
+{ COMMAND_INCREASE_BLINK, IR_COMMAND_FLAG_REPEATABLE_NON_BLOCKING, &doIncreaseBlinkFrequency, increaseBlink }, /**/
+{ COMMAND_DECREASE_BLINK, IR_COMMAND_FLAG_REPEATABLE_NON_BLOCKING, &doDecreaseBlinkFrequency, decreaseBlink } };
+
+#endif // _IR_COMMAND_MAPPING_H
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.h b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.h
new file mode 100644
index 00000000..83a98b27
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.h
@@ -0,0 +1,138 @@
+/*
+ * IRCommandDispatcher.h
+ *
+ * Library to process IR commands by calling functions specified in a mapping array.
+ *
+ * To run this example you need to install the "IRremote" or "IRMP" library under "Tools -> Manage Libraries..." or "Ctrl+Shift+I"
+ *
+ * Copyright (C) 2019-2024 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of ServoEasing https://github.com/ArminJo/ServoEasing.
+ * This file is part of IRMP https://github.com/IRMP-org/IRMP.
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * IRCommandDispatcher is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#ifndef _IR_COMMAND_DISPATCHER_H
+#define _IR_COMMAND_DISPATCHER_H
+
+#include
+
+/*
+ * For command mapping file
+ */
+#define IR_COMMAND_FLAG_BLOCKING 0x00 // default - blocking command, repeat not accepted, only one command at a time. Stops an already running command.
+#define IR_COMMAND_FLAG_REPEATABLE 0x01 // repeat accepted
+#define IR_COMMAND_FLAG_NON_BLOCKING 0x02 // Non blocking (short) command that can be processed any time and may interrupt other IR commands - used for stop, set direction etc.
+#define IR_COMMAND_FLAG_REPEATABLE_NON_BLOCKING (IR_COMMAND_FLAG_REPEATABLE | IR_COMMAND_FLAG_NON_BLOCKING)
+#define IR_COMMAND_FLAG_BEEP 0x04 // Do a single short beep before executing command. May not be useful for short or repeating commands.
+#define IR_COMMAND_FLAG_BLOCKING_BEEP (IR_COMMAND_FLAG_BLOCKING | IR_COMMAND_FLAG_BEEP)
+
+
+#if !defined(IS_STOP_REQUESTED)
+#define IS_STOP_REQUESTED IRDispatcher.requestToStopReceived
+#endif
+#if !defined(RETURN_IF_STOP)
+#define RETURN_IF_STOP if (IRDispatcher.requestToStopReceived) return
+#endif
+#if !defined(BREAK_IF_STOP)
+#define BREAK_IF_STOP if (IRDispatcher.requestToStopReceived) break
+#endif
+#if !defined(DELAY_AND_RETURN_IF_STOP)
+#define DELAY_AND_RETURN_IF_STOP(aDurationMillis) if (IRDispatcher.delayAndCheckForStop(aDurationMillis)) return
+#endif
+
+// Basic mapping structure
+struct IRToCommandMappingStruct {
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+ uint16_t IRCode;
+#else
+ uint8_t IRCode;
+#endif
+ uint8_t Flags;
+ void (*CommandToCall)();
+ const char *CommandString;
+};
+
+struct IRDataForCommandDispatcherStruct {
+ uint16_t address; // to distinguish between multiple senders
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+ uint16_t command;
+#else
+ uint8_t command;
+#endif
+ bool isRepeat;
+ uint32_t MillisOfLastCode; // millis() of last IR command -including repeats!- received - for timeouts etc.
+ volatile bool isAvailable; // flag for a polling interpreting function, that a new command has arrived. Is set true by library and set false by main loop.
+};
+
+/*
+ * Special codes (hopefully) not sent by the remote - otherwise please redefine it here
+ */
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+#define COMMAND_EMPTY 0xFFFF // code no command
+#else
+#define COMMAND_EMPTY 0xFF // code no command
+#endif
+
+class IRCommandDispatcher {
+public:
+ void init();
+ void printIRInfo(Print *aSerial);
+
+ bool checkAndRunNonBlockingCommands();
+ bool checkAndRunSuspendedBlockingCommands();
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+ void setNextBlockingCommand(uint16_t aBlockingCommandToRunNext);
+#else
+ void setNextBlockingCommand(uint8_t aBlockingCommandToRunNext);
+#endif
+ bool delayAndCheckForStop(uint16_t aDelayMillis);
+
+ // The main dispatcher function
+ void checkAndCallCommand(bool aCallBlockingCommandImmediately);
+
+ void printIRCommandString(Print *aSerial);
+ void setRequestToStopReceived(bool aRequestToStopReceived = true);
+
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+ uint16_t currentBlockingCommandCalled = COMMAND_EMPTY; // The code for the current called command
+ uint16_t lastBlockingCommandCalled = COMMAND_EMPTY; // The code for the last called command. Can be evaluated by main loop
+ uint16_t BlockingCommandToRunNext = COMMAND_EMPTY; // Storage for command currently suspended to allow the current command to end, before it is called by main loop
+#else
+ uint8_t currentBlockingCommandCalled = COMMAND_EMPTY; // The code for the current called command
+ uint8_t lastBlockingCommandCalled = COMMAND_EMPTY; // The code for the last called command. Can be evaluated by main loop
+ uint8_t BlockingCommandToRunNext = COMMAND_EMPTY; // Storage for command currently suspended to allow the current command to end, before it is called by main loop
+#endif
+ bool justCalledBlockingCommand = false; // Flag that a blocking command was received and called - is set before call of command
+ /*
+ * Flag for running blocking commands to terminate. To check, you can use "if (IRDispatcher.requestToStopReceived) return;" (available as macro RETURN_IF_STOP).
+ * It is set if a blocking IR command received, which cannot be executed directly. Can be reset by main loop, if command has stopped.
+ * It is reset before executing a blocking command.
+ */
+ volatile bool requestToStopReceived;
+ /*
+ * This flag must be true, if we have a function, which want to interpret the IR codes by itself e.g. the calibrate function of QuadrupedControl
+ */
+ bool doNotUseDispatcher = false;
+
+ struct IRDataForCommandDispatcherStruct IRReceivedData;
+
+};
+
+extern IRCommandDispatcher IRDispatcher;
+
+#endif // _IR_COMMAND_DISPATCHER_H
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.hpp b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.hpp
new file mode 100644
index 00000000..701103a1
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRCommandDispatcher.hpp
@@ -0,0 +1,366 @@
+/*
+ * IRCommandDispatcher.hpp
+ *
+ * Library to process IR commands by calling functions specified in a mapping array.
+ * Commands can be tagged as blocking or non blocking.
+ *
+ * To run this example you need to install the "IRremote" or "IRMP" library.
+ * Install it under "Tools -> Manage Libraries..." or "Ctrl+Shift+I"
+ *
+ * The IR library calls a callback function, which executes a non blocking command directly in ISR (Interrupt Service Routine) context!
+ * A blocking command is stored and sets a stop flag for an already running blocking function to terminate.
+ * The blocking command can in turn be executed by main loop by calling IRDispatcher.checkAndRunSuspendedBlockingCommands().
+ *
+ * Copyright (C) 2019-2024 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of ServoEasing https://github.com/ArminJo/ServoEasing.
+ * This file is part of IRMP https://github.com/IRMP-org/IRMP.
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * IRCommandDispatcher is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+/*
+ * Program behavior is modified by the following macros
+ * USE_TINY_IR_RECEIVER
+ * USE_IRMP_LIBRARY
+ * IR_COMMAND_HAS_MORE_THAN_8_BIT
+ */
+
+#ifndef _IR_COMMAND_DISPATCHER_HPP
+#define _IR_COMMAND_DISPATCHER_HPP
+
+#include
+
+#include "IRCommandDispatcher.h"
+
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
+/*
+ * Enable this to see information on each call.
+ * Since there should be no library which uses Serial, it should only be enabled for development purposes.
+ */
+#if defined(INFO) && !defined(LOCAL_INFO)
+#define LOCAL_INFO
+#else
+//#define LOCAL_INFO // This enables info output only for this file
+#endif
+#if defined(DEBUG) && !defined(LOCAL_DEBUG)
+#define LOCAL_DEBUG
+// Propagate debug level
+#define LOCAL_INFO
+#else
+//#define LOCAL_DEBUG // This enables debug output only for this file
+#endif
+
+IRCommandDispatcher IRDispatcher;
+
+#if defined(LOCAL_INFO)
+#define CD_INFO_PRINT(...) Serial.print(__VA_ARGS__);
+#define CD_INFO_PRINTLN(...) Serial.println(__VA_ARGS__);
+#else
+#define CD_INFO_PRINT(...) void();
+#define CD_INFO_PRINTLN(...) void();
+#endif
+
+#if defined(USE_TINY_IR_RECEIVER)
+#define USE_CALLBACK_FOR_TINY_RECEIVER // Call the fixed function "void handleReceivedTinyIRData()" each time a frame or repeat is received.
+#include "TinyIRReceiver.hpp" // included in "IRremote" library
+
+void IRCommandDispatcher::init() {
+ initPCIInterruptForTinyReceiver();
+}
+
+/*
+ * @return true, if IR Receiver is attached
+ */
+void IRCommandDispatcher::printIRInfo(Print *aSerial) {
+ aSerial->println();
+ // For available IR commands see IRCommandMapping.h https://github.com/ArminJo/PWMMotorControl/blob/master/examples/SmartCarFollower/IRCommandMapping.h
+ aSerial->print(F("Listening to IR remote of type "));
+ aSerial->print(IR_REMOTE_NAME);
+ aSerial->println(F(" at pin " STR(IR_RECEIVE_PIN)));
+}
+
+/*
+ * This is the TinyReceiver callback function, which is called if a complete command was received
+ * It checks for right address and then call the dispatcher
+ */
+# if defined(ESP8266) || defined(ESP32)
+IRAM_ATTR
+# endif
+void handleReceivedTinyIRData() {
+ IRDispatcher.IRReceivedData.address = TinyIRReceiverData.Address;
+ IRDispatcher.IRReceivedData.command = TinyIRReceiverData.Command;
+ IRDispatcher.IRReceivedData.isRepeat = TinyIRReceiverData.Flags & IRDATA_FLAGS_IS_REPEAT;
+ IRDispatcher.IRReceivedData.MillisOfLastCode = millis();
+
+# if defined(LOCAL_INFO)
+ printTinyReceiverResultMinimal(&Serial);
+# endif
+
+ if (TinyIRReceiverData.Address == IR_ADDRESS) { // IR_ADDRESS is defined in *IRCommandMapping.h
+ IRDispatcher.IRReceivedData.isAvailable = true;
+ if(!IRDispatcher.doNotUseDispatcher) {
+ /*
+ * Only short (non blocking) commands are executed directly in ISR (Interrupt Service Routine) context,
+ * others are stored for main loop which calls checkAndRunSuspendedBlockingCommands()
+ */
+ IRDispatcher.checkAndCallCommand(false);
+ }
+
+ } else {
+ CD_INFO_PRINT(F("Wrong address. Expected 0x"));
+ CD_INFO_PRINTLN(IR_ADDRESS, HEX);
+ }
+}
+
+#elif defined(USE_IRMP_LIBRARY)
+# if !defined(IRMP_USE_COMPLETE_CALLBACK)
+# error IRMP_USE_COMPLETE_CALLBACK must be activated for IRMP library
+# endif
+
+void IRCommandDispatcher::init() {
+ irmp_init();
+}
+
+/*
+ * This is the callback function, which is called if a complete command was received
+ */
+#if defined(ESP8266) || defined(ESP32)
+IRAM_ATTR
+#endif
+void handleReceivedIRData() {
+ IRMP_DATA tTeporaryData;
+ irmp_get_data(&tTeporaryData);
+ IRDispatcher.IRReceivedData.address = tTeporaryData.address;
+ IRDispatcher.IRReceivedData.command = tTeporaryData.command;
+ IRDispatcher.IRReceivedData.isRepeat = tTeporaryData.flags & IRMP_FLAG_REPETITION;
+ IRDispatcher.IRReceivedData.MillisOfLastCode = millis();
+
+ CD_INFO_PRINT(F("A=0x"));
+ CD_INFO_PRINT(IRDispatcher.IRReceivedData.address, HEX);
+ CD_INFO_PRINT(F(" C=0x"));
+ CD_INFO_PRINT(IRDispatcher.IRReceivedData.command, HEX);
+ if (IRDispatcher.IRReceivedData.isRepeat) {
+ CD_INFO_PRINT(F("R"));
+ }
+ CD_INFO_PRINTLN();
+
+ // To enable delay() for commands
+# if !defined(ARDUINO_ARCH_MBED)
+ interrupts(); // be careful with always executable commands which lasts longer than the IR repeat duration.
+# endif
+
+ if (IRDispatcher.IRReceivedData.address == IR_ADDRESS) {
+ IRDispatcher.checkAndCallCommand(true);
+ } else {
+ CD_INFO_PRINT(F("Wrong address. Expected 0x"));
+ CD_INFO_PRINTLN(IR_ADDRESS, HEX);
+ }
+}
+#endif // elif defined(USE_IRMP_LIBRARY)
+
+/*
+ * The main dispatcher function called by IR-ISR, main loop and checkAndRunSuspendedBlockingCommands()
+ * Non blocking commands are executed directly, blocking commands are executed if enabled by parameter and no other command is just running.
+ * Otherwise request to stop (requestToStopReceived) is set and command is stored for main loop to be later execute by checkAndRunSuspendedBlockingCommands().
+ * Sets flags justCalledRegularIRCommand, executingBlockingCommand, requestToStopReceived
+ * @param aCallBlockingCommandImmediately Run blocking command directly, if no other command is just running. Should be false if called by ISR in order not to block ISR.
+ */
+void IRCommandDispatcher::checkAndCallCommand(bool aCallBlockingCommandImmediately) {
+ if (IRReceivedData.command == COMMAND_EMPTY) {
+ return;
+ }
+
+ /*
+ * Search for command in Array of IRToCommandMappingStruct
+ */
+ for (uint_fast8_t i = 0; i < sizeof(IRMapping) / sizeof(struct IRToCommandMappingStruct); ++i) {
+ if (IRReceivedData.command == IRMapping[i].IRCode) {
+ /*
+ * Command found
+ */
+#if defined(LOCAL_INFO)
+ const __FlashStringHelper *tCommandName = reinterpret_cast(IRMapping[i].CommandString);
+#endif
+ /*
+ * Check for repeat and if repeat is allowed for the current command
+ */
+ if (IRReceivedData.isRepeat && !(IRMapping[i].Flags & IR_COMMAND_FLAG_REPEATABLE)) {
+#if defined(LOCAL_DEBUG)
+ Serial.print(F("Repeats of command \""));
+ Serial.print(tCommandName);
+ Serial.println("\" not accepted");
+#endif
+ return;
+ }
+
+ /*
+ * Do not accept recursive call of the same command
+ */
+ if (currentBlockingCommandCalled == IRReceivedData.command) {
+#if defined(LOCAL_DEBUG)
+ Serial.print(F("Recursive command \""));
+ Serial.print(tCommandName);
+ Serial.println("\" not accepted");
+#endif
+ return;
+ }
+
+ /*
+ * Execute commands
+ */
+ bool tIsNonBlockingCommand = (IRMapping[i].Flags & IR_COMMAND_FLAG_NON_BLOCKING);
+ if (tIsNonBlockingCommand) {
+ // short command here, just call
+ CD_INFO_PRINT(F("Run non blocking command: "));
+ CD_INFO_PRINTLN(tCommandName);
+#if defined(BUZZER_PIN) && defined(USE_TINY_IR_RECEIVER)
+ /*
+ * Do (non blocking) buzzer feedback before command is executed
+ */
+ if(IRMapping[i].Flags & IR_COMMAND_FLAG_BEEP) {
+ tone(BUZZER_PIN, 2200, 50);
+ }
+#endif
+ IRMapping[i].CommandToCall();
+ } else {
+ /*
+ * Blocking command here
+ */
+ if (aCallBlockingCommandImmediately && currentBlockingCommandCalled == COMMAND_EMPTY) {
+ /*
+ * Here no blocking command was running and we are called from main loop
+ */
+ requestToStopReceived = false; // Do not stop the command executed now
+ justCalledBlockingCommand = true;
+ currentBlockingCommandCalled = IRReceivedData.command; // set lock for recursive calls
+ lastBlockingCommandCalled = IRReceivedData.command; // set history, can be evaluated by main loop
+ /*
+ * This call is blocking!!!
+ */
+ CD_INFO_PRINT(F("Run blocking command: "));
+ CD_INFO_PRINTLN(tCommandName);
+
+#if defined(BUZZER_PIN) && defined(USE_TINY_IR_RECEIVER)
+ /*
+ * Do (non blocking) buzzer feedback before command is executed
+ */
+ if(IRMapping[i].Flags & IR_COMMAND_FLAG_BEEP) {
+ tone(BUZZER_PIN, 2200, 50);
+ }
+#endif
+
+ IRMapping[i].CommandToCall();
+#if defined(TRACE)
+ Serial.println(F("End of blocking command"));
+#endif
+ currentBlockingCommandCalled = COMMAND_EMPTY;
+ } else {
+ /*
+ * Called by ISR or another command still running.
+ * Do not run command directly, but set request to stop to true and store command
+ * for main loop to execute by checkAndRunSuspendedBlockingCommands()
+ */
+ BlockingCommandToRunNext = IRReceivedData.command;
+ requestToStopReceived = true; // to stop running command
+ CD_INFO_PRINT(F("Requested stop and stored blocking command "));
+ CD_INFO_PRINT(tCommandName);
+ CD_INFO_PRINTLN(F(" as next command to run."));
+ }
+ }
+ break; // command found
+ } // if (IRReceivedData.command == IRMapping[i].IRCode)
+ } // for loop
+ return;
+}
+
+/*
+ * Intended to be called from main loop
+ * @return true, if command was called
+ */
+bool IRCommandDispatcher::checkAndRunSuspendedBlockingCommands() {
+ /*
+ * Take last rejected command and call associated function
+ */
+ if (BlockingCommandToRunNext != COMMAND_EMPTY) {
+
+ CD_INFO_PRINT(F("Run stored command=0x"));
+ CD_INFO_PRINTLN(BlockingCommandToRunNext, HEX);
+
+ IRReceivedData.command = BlockingCommandToRunNext;
+ BlockingCommandToRunNext = COMMAND_EMPTY;
+ IRReceivedData.isRepeat = false;
+ requestToStopReceived = false; // Do not stop the command executed now
+ checkAndCallCommand(true);
+ return true;
+ }
+ return false;
+}
+
+/*
+ * Not used internally
+ */
+#if defined(IR_COMMAND_HAS_MORE_THAN_8_BIT)
+void IRCommandDispatcher::setNextBlockingCommand(uint16_t aBlockingCommandToRunNext)
+#else
+void IRCommandDispatcher::setNextBlockingCommand(uint8_t aBlockingCommandToRunNext)
+#endif
+ {
+ CD_INFO_PRINT(F("Set next command to run to 0x"));
+ CD_INFO_PRINTLN(aBlockingCommandToRunNext, HEX);
+ BlockingCommandToRunNext = aBlockingCommandToRunNext;
+ requestToStopReceived = true;
+}
+
+/*
+ * Special delay function for the IRCommandDispatcher. Returns prematurely if requestToStopReceived is set.
+ * To be used in blocking functions as delay
+ * @return true - as soon as stop received
+ */
+bool IRCommandDispatcher::delayAndCheckForStop(uint16_t aDelayMillis) {
+ uint32_t tStartMillis = millis();
+ do {
+ if (requestToStopReceived) {
+ return true;
+ }
+ } while (millis() - tStartMillis < aDelayMillis);
+ return false;
+}
+
+void IRCommandDispatcher::printIRCommandString(Print *aSerial) {
+ for (uint_fast8_t i = 0; i < sizeof(IRMapping) / sizeof(struct IRToCommandMappingStruct); ++i) {
+ if (IRReceivedData.command == IRMapping[i].IRCode) {
+ aSerial->println(reinterpret_cast(IRMapping[i].CommandString));
+ return;
+ }
+ }
+ aSerial->println(reinterpret_cast(unknown));
+}
+
+void IRCommandDispatcher::setRequestToStopReceived(bool aRequestToStopReceived) {
+ requestToStopReceived = aRequestToStopReceived;
+}
+
+#if defined(LOCAL_DEBUG)
+#undef LOCAL_DEBUG
+#endif
+#if defined(LOCAL_INFO)
+#undef LOCAL_INFO
+#endif
+#endif // _IR_COMMAND_DISPATCHER_HPP
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRDispatcherDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRDispatcherDemo.ino
new file mode 100644
index 00000000..88f72024
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/IRDispatcherDemo.ino
@@ -0,0 +1,280 @@
+/*
+ * IRDispatcherDemo.cpp
+ *
+ * Receives NEC IR commands and maps them to different actions by means of a mapping array.
+ *
+ * Copyright (C) 2020-2021 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRMP https://github.com/IRMP-org/IRMP.
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * IRMP is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+#include
+
+/*
+ * Choose the library to be used for IR receiving
+ */
+#define USE_TINY_IR_RECEIVER // Recommended, but only for NEC protocol!!! If disabled and IRMP_INPUT_PIN is defined, the IRMP library is used for decoding
+//#define TINY_RECEIVER_USE_ARDUINO_ATTACH_INTERRUPT // Requires additional 112 bytes program memory + 4 bytes RAM
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+// Some kind of auto detect library if USE_TINY_IR_RECEIVER is deactivated
+#if !defined(USE_TINY_IR_RECEIVER)
+# if defined(IR_RECEIVE_PIN)
+#define USE_TINY_IR_RECEIVER
+# elif !defined(USE_IRMP_LIBRARY) && defined(IRMP_INPUT_PIN)
+#define USE_IRMP_LIBRARY
+# else
+#error No IR library selected
+# endif
+#endif
+
+//#define NO_LED_FEEDBACK_CODE // You can set it here, before the include of IRCommandDispatcher below
+
+#if defined(USE_TINY_IR_RECEIVER)
+//#define NO_LED_FEEDBACK_CODE // Activate this if you want to suppress LED feedback or if you do not have a LED. This saves 14 bytes code and 2 clock cycles per interrupt.
+
+#elif defined(USE_IRMP_LIBRARY)
+/*
+ * IRMP version
+ */
+#define IR_RECEIVE_PIN 2
+#define IRMP_USE_COMPLETE_CALLBACK 1 // Enable callback functionality. It is required if IRMP library is used.
+#if defined(ALTERNATIVE_IR_FEEDBACK_LED_PIN)
+#define FEEDBACK_LED_PIN ALTERNATIVE_IR_FEEDBACK_LED_PIN
+#endif
+
+//#define IRMP_ENABLE_PIN_CHANGE_INTERRUPT // Enable interrupt functionality (not for all protocols) - requires around 376 additional bytes of program memory
+
+#define IRMP_PROTOCOL_NAMES 1 // Enable protocol number mapping to protocol strings - requires some program memory. Must before #include
+
+#define IRMP_SUPPORT_NEC_PROTOCOL 1 // this enables only one protocol
+//#define IRMP_SUPPORT_KASEIKYO_PROTOCOL 1
+
+# if defined(ALTERNATIVE_IR_FEEDBACK_LED_PIN)
+#define IRMP_FEEDBACK_LED_PIN ALTERNATIVE_IR_FEEDBACK_LED_PIN
+# endif
+/*
+ * After setting the definitions we can include the code and compile it.
+ */
+#include
+void handleReceivedIRData();
+void irmp_tone(uint8_t _pin, unsigned int frequency, unsigned long duration);
+#endif // #if defined(USE_IRMP_LIBRARY)
+
+bool doBlink = false;
+uint16_t sBlinkDelay = 200;
+
+void doPrintMenu();
+void doLedOn();
+void doLedOff();
+void doIncreaseBlinkFrequency();
+void doDecreaseBlinkFrequency();
+void doStop();
+void doResetBlinkFrequency();
+void doLedBlinkStart();
+void doLedBlink20times();
+void doTone1800();
+void doTone2200();
+
+/*
+ * Set definitions and include IRCommandDispatcher library after the declaration of all commands to map
+ */
+#define INFO // to see some informative output
+#include "IRCommandDispatcher.h" // Only for required declarations, the library itself is included below after the definitions of the commands
+#include "DemoIRCommandMapping.h" // must be included before IRCommandDispatcher.hpp to define IR_ADDRESS and IRMapping and string "unknown".
+#include "IRCommandDispatcher.hpp"
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+#if defined(ESP8266)
+ Serial.println(); // to separate it from the internal boot output
+#endif
+
+ // Just to know which program is running on my Arduino
+#if defined(USE_TINY_IR_RECEIVER)
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing TinyIRReceiver"));
+#elif defined(USE_IRREMOTE_LIBRARY)
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing IRremote library version " VERSION_IRREMOTE));
+#elif defined(USE_IRMP_LIBRARY)
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing IRMP library version " VERSION_IRMP));
+#endif
+
+#if !defined(ESP8266) && !defined(NRF5)
+ // play feedback tone before setup, since it kills the IR timer settings
+ tone(TONE_PIN, 1000, 50);
+ delay(50);
+#endif
+
+ IRDispatcher.init(); // This just calls irmp_init()
+#if defined(USE_TINY_IR_RECEIVER)
+ Serial.println(F("Ready to receive NEC IR signals at pin " STR(IR_RECEIVE_PIN)));
+#else
+ irmp_register_complete_callback_function(&handleReceivedIRData); // fixed function in IRCommandDispatcher.hpp
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ irmp_print_active_protocols(&Serial);
+ Serial.println(F("at pin " STR(IRMP_INPUT_PIN)));
+
+# if defined(ALTERNATIVE_IR_FEEDBACK_LED_PIN)
+ irmp_irsnd_LEDFeedback(true); // Enable receive signal feedback at ALTERNATIVE_IR_FEEDBACK_LED_PIN
+ Serial.println(F("IR feedback pin is " STR(ALTERNATIVE_IR_FEEDBACK_LED_PIN)));
+# endif
+#endif
+
+ Serial.print(F("Listening to commands of IR remote of type "));
+ Serial.println(IR_REMOTE_NAME);
+ doPrintMenu();
+}
+
+void loop() {
+
+ IRDispatcher.checkAndRunSuspendedBlockingCommands();
+
+ if (doBlink) {
+ digitalWrite(LED_BUILTIN, HIGH);
+ DELAY_AND_RETURN_IF_STOP(sBlinkDelay);
+ digitalWrite(LED_BUILTIN, LOW);
+ DELAY_AND_RETURN_IF_STOP(sBlinkDelay);
+ }
+
+ if (millis() - IRDispatcher.IRReceivedData.MillisOfLastCode > 120000)
+ {
+ //Short beep as remainder, if we did not receive any command in the last 2 minutes
+ IRDispatcher.IRReceivedData.MillisOfLastCode += 120000;
+ doTone1800();
+ }
+
+// delay(10);
+}
+
+void doPrintMenu(){
+ Serial.println();
+ Serial.println(F("Press 1 for tone 1800 Hz"));
+ Serial.println(F("Press 2 for tone 2200 Hz"));
+ Serial.println(F("Press 3 for this Menu"));
+ Serial.println(F("Press 0 for LED blink 20 times"));
+ Serial.println(F("Press UP for LED on"));
+ Serial.println(F("Press DOWN for LED off"));
+ Serial.println(F("Press OK for LED blink start"));
+ Serial.println(F("Press RIGHT for LED increase blink frequency"));
+ Serial.println(F("Press LEFT for LED decrease blink frequency"));
+ Serial.println(F("Press STAR for reset blink frequency"));
+ Serial.println(F("Press HASH for stop"));
+ Serial.println();
+}
+/*
+ * Here the actions that are matched to IR keys
+ */
+void doLedOn() {
+ digitalWrite(LED_BUILTIN, HIGH);
+ doBlink = false;
+}
+void doLedOff() {
+ digitalWrite(LED_BUILTIN, LOW);
+ doBlink = false;
+}
+void doIncreaseBlinkFrequency() {
+ doBlink = true;
+ if (sBlinkDelay > 5) {
+ sBlinkDelay -= sBlinkDelay / 4;
+ }
+}
+void doDecreaseBlinkFrequency() {
+ doBlink = true;
+ sBlinkDelay += sBlinkDelay / 4;
+}
+void doStop() {
+ doBlink = false;
+}
+void doResetBlinkFrequency() {
+ sBlinkDelay = 200;
+ digitalWrite(LED_BUILTIN, LOW);
+}
+void doLedBlinkStart() {
+ doBlink = true;
+}
+/*
+ * This is a blocking function and checks periodically for stop
+ */
+void doLedBlink20times() {
+ for (int i = 0; i < 20; ++i) {
+ digitalWrite(LED_BUILTIN, HIGH);
+ DELAY_AND_RETURN_IF_STOP(200);
+ digitalWrite(LED_BUILTIN, LOW);
+ DELAY_AND_RETURN_IF_STOP(200);
+ }
+}
+
+
+void doTone1800() {
+#if defined(USE_IRMP_LIBRARY) && !defined(IRMP_ENABLE_PIN_CHANGE_INTERRUPT)
+ irmp_tone(TONE_PIN, 1800, 200);
+#else
+# if !defined(ESP8266) && !defined(NRF5) // tone() stops timer 1 for ESP8266
+ tone(TONE_PIN, 1800, 200);
+# endif
+#endif
+}
+
+void doTone2200() {
+#if defined(USE_IRMP_LIBRARY) && !defined(IRMP_ENABLE_PIN_CHANGE_INTERRUPT)
+ // use IRMP compatible function for tone()
+ irmp_tone(TONE_PIN, 2200, 50);
+#else
+# if !defined(ESP8266) && !defined(NRF5) // tone() stops timer 1 for ESP8266
+ tone(TONE_PIN, 2200, 50);
+# endif
+#endif
+}
+
+#if defined(USE_IRMP_LIBRARY)
+/*
+ * Convenience IRMP compatible wrapper function for Arduino tone() if IRMP_ENABLE_PIN_CHANGE_INTERRUPT is NOT activated
+ * It currently disables the receiving of repeats
+ */
+void irmp_tone(uint8_t _pin, unsigned int frequency, unsigned long duration) {
+# if defined(__AVR__) && !defined(IRMP_ENABLE_PIN_CHANGE_INTERRUPT)
+ storeIRTimer();
+ tone(_pin, frequency, 0);
+ if (duration == 0) {
+ duration = 100;
+ }
+ delay(duration);
+ noTone(_pin);
+ restoreIRTimer();
+#elif defined(ESP8266)
+ // tone() stops timer 1
+ (void) _pin;
+ (void) frequency;
+ (void) duration;
+#else
+ tone(_pin, frequency, duration);
+#endif
+}
+#endif // #if defined(USE_IRMP_LIBRARY)
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRDispatcherDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecord/IRrecord.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRrecord/IRrecord.ino
deleted file mode 100644
index e9e03436..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecord/IRrecord.ino
+++ /dev/null
@@ -1,178 +0,0 @@
-/*
- * IRrecord: record and play back IR signals as a minimal
- * An IR detector/demodulator must be connected to the input RECV_PIN.
- * An IR LED must be connected to Arduino PWM pin 3.
- * Microduino Core:D3
- * Microduino Core+:D8
- * Microduino CoreUSB:D6
- * Microduino CoreRF:D5
- * A button must be connected to the input BUTTON_PIN; this is the
- * send button.
- * A visible LED can be connected to STATUS_PIN to provide status.
- *
- * The logic is:
- * If the button is pressed, send the IR code.
- * If an IR code is received, record it.
- *
- * Version 0.11 September, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- */
-
-#include
-
-int RECV_PIN = 4;
-int BUTTON_PIN = 12;
-int STATUS_PIN = 13;
-
-IRrecv irrecv(RECV_PIN);
-IRsend irsend;
-
-decode_results results;
-
-void setup()
-{
- Serial.begin(9600);
- irrecv.enableIRIn(); // Start the receiver
- pinMode(BUTTON_PIN, INPUT);
- pinMode(STATUS_PIN, OUTPUT);
-}
-
-// Storage for the recorded code
-int codeType = -1; // The type of code
-unsigned long codeValue; // The code value if not raw
-unsigned int rawCodes[RAWBUF]; // The durations if raw
-int codeLen; // The length of the code
-int toggle = 0; // The RC5/6 toggle state
-
-// Stores the code for later playback
-// Most of this code is just logging
-void storeCode(decode_results *results) {
- codeType = results->decode_type;
- int count = results->rawlen;
- if (codeType == UNKNOWN) {
- Serial.println("Received unknown code, saving as raw");
- codeLen = results->rawlen - 1;
- // To store raw codes:
- // Drop first value (gap)
- // Convert from ticks to microseconds
- // Tweak marks shorter, and spaces longer to cancel out IR receiver distortion
- for (int i = 1; i <= codeLen; i++) {
- if (i % 2) {
- // Mark
- rawCodes[i - 1] = results->rawbuf[i]*USECPERTICK - MARK_EXCESS;
- Serial.print(" m");
- }
- else {
- // Space
- rawCodes[i - 1] = results->rawbuf[i]*USECPERTICK + MARK_EXCESS;
- Serial.print(" s");
- }
- Serial.print(rawCodes[i - 1], DEC);
- }
- Serial.println("");
- }
- else {
- if (codeType == NEC) {
- Serial.print("Received NEC: ");
- if (results->value == REPEAT) {
- // Don't record a NEC repeat value as that's useless.
- Serial.println("repeat; ignoring.");
- return;
- }
- }
- else if (codeType == SONY) {
- Serial.print("Received SONY: ");
- }
- else if (codeType == RC5) {
- Serial.print("Received RC5: ");
- }
- else if (codeType == RC6) {
- Serial.print("Received RC6: ");
- }
- else {
- Serial.print("Unexpected codeType ");
- Serial.print(codeType, DEC);
- Serial.println("");
- }
- Serial.println(results->value, HEX);
- codeValue = results->value;
- codeLen = results->bits;
- }
-}
-
-void sendCode(int repeat) {
- if (codeType == NEC) {
- if (repeat) {
- irsend.sendNEC(REPEAT, codeLen);
- Serial.println("Sent NEC repeat");
- }
- else {
- irsend.sendNEC(codeValue, codeLen);
- Serial.print("Sent NEC ");
- Serial.println(codeValue, HEX);
- }
- }
- else if (codeType == SONY) {
- irsend.sendSony(codeValue, codeLen);
- Serial.print("Sent Sony ");
- Serial.println(codeValue, HEX);
- }
- else if (codeType == RC5 || codeType == RC6) {
- if (!repeat) {
- // Flip the toggle bit for a new button press
- toggle = 1 - toggle;
- }
- // Put the toggle bit into the code to send
- codeValue = codeValue & ~(1 << (codeLen - 1));
- codeValue = codeValue | (toggle << (codeLen - 1));
- if (codeType == RC5) {
- Serial.print("Sent RC5 ");
- Serial.println(codeValue, HEX);
- irsend.sendRC5(codeValue, codeLen);
- }
- else {
- irsend.sendRC6(codeValue, codeLen);
- Serial.print("Sent RC6 ");
- Serial.println(codeValue, HEX);
- }
- }
- else if (codeType == UNKNOWN /* i.e. raw */) {
- // Assume 38 KHz
- irsend.sendRaw(rawCodes, codeLen, 38);
- Serial.println("Sent raw");
- }
-}
-
-int lastButtonState;
-
-void loop() {
- // If button pressed, send the code.
- int buttonState = digitalRead(BUTTON_PIN);
- if (lastButtonState == HIGH && buttonState == LOW) {
- Serial.println("Released");
- irrecv.enableIRIn(); // Re-enable receiver
- }
-
- if (buttonState) {
- Serial.println("Pressed, sending");
- digitalWrite(STATUS_PIN, HIGH);
- sendCode(lastButtonState == buttonState);
- digitalWrite(STATUS_PIN, LOW);
- delay(50); // Wait a bit between retransmissions
- }
- else if (irrecv.decode(&results)) {
- digitalWrite(STATUS_PIN, HIGH);
- storeCode(&results);
- irrecv.resume(); // resume receiver
- digitalWrite(STATUS_PIN, LOW);
- }
- lastButtonState = buttonState;
-}
-
-
-
-
-
-
-
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDemo/IRrecvDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDemo/IRrecvDemo.ino
deleted file mode 100644
index 9c31cef5..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDemo/IRrecvDemo.ino
+++ /dev/null
@@ -1,28 +0,0 @@
-/*
- * IRremote: IRrecvDemo - demonstrates receiving IR codes with IRrecv
- * An IR detector/demodulator must be connected to the input RECV_PIN.
- * Version 0.1 July, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- */
-
-#include
-
-int RECV_PIN = 4;
-
-IRrecv irrecv(RECV_PIN);
-
-decode_results results;
-
-void setup()
-{
- Serial.begin(9600);
- irrecv.enableIRIn(); // Start the receiver
-}
-
-void loop() {
- if (irrecv.decode(&results)) {
- Serial.println(results.value, HEX);
- irrecv.resume(); // Receive the next value
- }
-}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDump/.test.skip b/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDump/.test.skip
deleted file mode 100644
index e69de29b..00000000
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDump/IRrecvDump.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDump/IRrecvDump.ino
deleted file mode 100644
index ce448468..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRrecvDump/IRrecvDump.ino
+++ /dev/null
@@ -1,74 +0,0 @@
-/*
- * IRremote: IRrecvDump - dump details of IR codes with IRrecv
- * An IR detector/demodulator must be connected to the input RECV_PIN.
- * Version 0.1 July, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- */
-
-#include
-
-int RECV_PIN = 4;
-
-IRrecv irrecv(RECV_PIN);
-
-decode_results results;
-
-void setup()
-{
- Serial.begin(9600);
- irrecv.enableIRIn(); // Start the receiver
-}
-
-// Dumps out the decode_results structure.
-// Call this after IRrecv::decode()
-// void * to work around compiler issue
-//void dump(void *v) {
-// decode_results *results = (decode_results *)v
-void dump(decode_results *results) {
- int count = results->rawlen;
- if (results->decode_type == UNKNOWN) {
- Serial.println("Could not decode message");
- }
- else {
- if (results->decode_type == NEC) {
- Serial.print("Decoded NEC: ");
- }
- else if (results->decode_type == SONY) {
- Serial.print("Decoded SONY: ");
- }
- else if (results->decode_type == RC5) {
- Serial.print("Decoded RC5: ");
- }
- else if (results->decode_type == RC6) {
- Serial.print("Decoded RC6: ");
- }
- Serial.print(results->value, HEX);
- Serial.print(" (");
- Serial.print(results->bits, DEC);
- Serial.println(" bits)");
- }
- Serial.print("Raw (");
- Serial.print(count, DEC);
- Serial.print("): ");
-
- for (int i = 0; i < count; i++) {
- if ((i % 2) == 1) {
- Serial.print(results->rawbuf[i]*USECPERTICK, DEC);
- }
- else {
- Serial.print(-(int)results->rawbuf[i]*USECPERTICK, DEC);
- }
- Serial.print(" ");
- }
- Serial.println("");
-}
-
-
-void loop() {
- if (irrecv.decode(&results)) {
- Serial.println(results.value, HEX);
- dump(&results);
- irrecv.resume(); // Receive the next value
- }
-}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrelay/.test.skip b/libraries/_07_m_Sensor_IR_Remote/examples/IRrelay/.test.skip
deleted file mode 100644
index e69de29b..00000000
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRrelay/IRrelay.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRrelay/IRrelay.ino
deleted file mode 100644
index 8e0a9a4b..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRrelay/IRrelay.ino
+++ /dev/null
@@ -1,85 +0,0 @@
-/*
- * IRremote: IRrecvDemo - demonstrates receiving IR codes with IRrecv
- * An IR detector/demodulator must be connected to the input RECV_PIN.
- * Version 0.1 July, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- */
-
-#include
-
-int RECV_PIN = 4;
-int RELAY_PIN = 6;
-
-IRrecv irrecv(RECV_PIN);
-decode_results results;
-
-// Dumps out the decode_results structure.
-// Call this after IRrecv::decode()
-// void * to work around compiler issue
-//void dump(void *v) {
-// decode_results *results = (decode_results *)v
-void dump(decode_results *results) {
- int count = results->rawlen;
- if (results->decode_type == UNKNOWN) {
- Serial.println("Could not decode message");
- }
- else {
- if (results->decode_type == NEC) {
- Serial.print("Decoded NEC: ");
- }
- else if (results->decode_type == SONY) {
- Serial.print("Decoded SONY: ");
- }
- else if (results->decode_type == RC5) {
- Serial.print("Decoded RC5: ");
- }
- else if (results->decode_type == RC6) {
- Serial.print("Decoded RC6: ");
- }
- Serial.print(results->value, HEX);
- Serial.print(" (");
- Serial.print(results->bits, DEC);
- Serial.println(" bits)");
- }
- Serial.print("Raw (");
- Serial.print(count, DEC);
- Serial.print("): ");
-
- for (int i = 0; i < count; i++) {
- if ((i % 2) == 1) {
- Serial.print(results->rawbuf[i]*USECPERTICK, DEC);
- }
- else {
- Serial.print(-(int)results->rawbuf[i]*USECPERTICK, DEC);
- }
- Serial.print(" ");
- }
- Serial.println("");
-}
-
-void setup()
-{
- pinMode(RELAY_PIN, OUTPUT);
- pinMode(13, OUTPUT);
- Serial.begin(9600);
- irrecv.enableIRIn(); // Start the receiver
-}
-
-int on = 0;
-unsigned long last = millis();
-
-void loop() {
- if (irrecv.decode(&results)) {
- // If it's been at least 1/4 second since the last
- // IR received, toggle the relay
- if (millis() - last > 250) {
- on = !on;
- digitalWrite(RELAY_PIN, on ? HIGH : LOW);
- digitalWrite(13, on ? HIGH : LOW);
- dump(&results);
- }
- last = millis();
- irrecv.resume(); // Receive the next value
- }
-}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.cpp b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.cpp
new file mode 100644
index 00000000..3ec6be4c
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.cpp
@@ -0,0 +1,47 @@
+/*
+ * IRremoteExtensionClass.cpp
+ *
+ * Example for a class which itself uses the IRrecv class from IRremote
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2021 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "IRremoteExtensionClass.h"
+
+IRExtensionClass::IRExtensionClass(IRrecv *aIrReceiver) {
+ MyIrReceiver = aIrReceiver;
+}
+void IRExtensionClass::decode() {
+ Serial.println(F("Call decode()"));
+ MyIrReceiver->decode();
+}
+
+void IRExtensionClass::resume() {
+ Serial.println(F("Call resume()"));
+ MyIrReceiver->resume();
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.h b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.h
new file mode 100644
index 00000000..700ad0ee
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionClass.h
@@ -0,0 +1,45 @@
+/*
+ * IRremoteExtensionClass.h
+ *
+ * Example for a class which itself uses the IRrecv class from IRremote
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2021 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#define USE_IRREMOTE_HPP_AS_PLAIN_INCLUDE
+#include
+
+class IRExtensionClass
+{
+public:
+ IRrecv * MyIrReceiver;
+ IRExtensionClass(IRrecv * aIrReceiver);
+ void decode();
+ void resume();
+};
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionTest.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionTest.ino
new file mode 100644
index 00000000..65b42c03
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/IRremoteExtensionTest.ino
@@ -0,0 +1,79 @@
+/*
+ * IRremoteExtensionTest.cpp
+ * Simple test using the IRremoteExtensionClass.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 180 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# elif RAMEND <= 0x8FF || RAMSIZE < 0x8FF
+#define RAW_BUFFER_LENGTH 500 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# else
+#define RAW_BUFFER_LENGTH 750 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# endif
+#endif
+
+#include
+
+#include "IRremoteExtensionClass.h"
+
+/*
+ * Create the class, which itself uses the IRrecv class from IRremote
+ */
+IRExtensionClass IRExtension(&IrReceiver);
+
+void setup() {
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+// Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+
+}
+
+void loop() {
+ if (IrReceiver.decode()) {
+ IrReceiver.printIRResultShort(&Serial);
+ IrReceiver.printIRSendUsage(&Serial);
+ IRExtension.resume(); // Use the extended function provided by IRExtension class
+ }
+ delay(100);
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteExtensionTest/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteInfo/IRremoteInfo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteInfo/IRremoteInfo.ino
new file mode 100644
index 00000000..22ae75fb
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/IRremoteInfo/IRremoteInfo.ino
@@ -0,0 +1,362 @@
+/*
+ * IRremote: IRremoteInfo - prints relevant config info & settings for IRremote over serial
+ * Intended to help identify & troubleshoot the various settings of IRremote
+ * For example, sometimes users are unsure of which pin is used for Tx or the RAW_BUFFER_LENGTH value
+ * This example can be used to assist the user directly or with support.
+ * Intended to help identify & troubleshoot the various settings of IRremote
+ * Hopefully this utility will be a useful tool for support & troubleshooting for IRremote
+ * Check out the blog post describing the sketch via http://www.analysir.com/blog/2015/11/28/helper-utility-for-troubleshooting-irremote/
+ * Version 1.0 November 2015
+ * Original Author: AnalysIR - IR software & modules for Makers & Pros, visit http://www.AnalysIR.com
+ */
+#include
+
+//#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 240 bytes program memory if IrSender.write is used
+//#define SEND_PWM_BY_TIMER
+//#define USE_NO_SEND_PWM
+//#define NO_LED_FEEDBACK_CODE // saves 566 bytes program memory
+
+#include
+
+// Function declarations for non Arduino IDE's
+void dumpHeader();
+void dumpRAW_BUFFER_LENGTH();
+void dumpTIMER();
+void dumpTimerPin();
+void dumpClock();
+void dumpPlatform();
+void dumpPulseParams();
+void dumpSignalParams();
+void dumpArduinoIDE();
+void dumpDebugMode();
+void dumpProtocols();
+void dumpFooter();
+
+void setup() {
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ //Runs only once per restart of the Arduino.
+ dumpHeader();
+ dumpRAW_BUFFER_LENGTH();
+ dumpTIMER();
+ dumpTimerPin();
+ dumpClock();
+ dumpPlatform();
+ dumpPulseParams();
+ dumpSignalParams();
+ dumpArduinoIDE();
+ dumpDebugMode();
+ dumpProtocols();
+ dumpFooter();
+}
+
+void loop() {
+ //nothing to do!
+}
+
+void dumpRAW_BUFFER_LENGTH() {
+ Serial.print(F("RAW_BUFFER_LENGTH: "));
+ Serial.println(RAW_BUFFER_LENGTH);
+}
+
+void dumpTIMER() {
+ bool flag = false;
+#if defined(IR_USE_TIMER1)
+ Serial.print(F("Timer defined for use: "));
+ Serial.println(F("Timer1"));
+ flag = true;
+#endif
+#if defined(IR_USE_TIMER2)
+ Serial.print(F("Timer defined for use: "));
+ Serial.println(F("Timer2"));
+ flag = true;
+#endif
+#if defined(IR_USE_TIMER3)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer3")); flag = true;
+#endif
+#if defined(IR_USE_TIMER4)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer4")); flag = true;
+#endif
+#if defined(IR_USE_TIMER5)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer5")); flag = true;
+#endif
+#if defined(IR_USE_TIMER4_HS)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer4_HS")); flag = true;
+#endif
+#if defined(IR_USE_TIMER_CMT)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer_CMT")); flag = true;
+#endif
+#if defined(IR_USE_TIMER_TPM1)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer_TPM1")); flag = true;
+#endif
+#if defined(IR_USE_TIMER_TINY0)
+ Serial.print(F("Timer defined for use: ")); Serial.println(F("Timer_TINY0")); flag = true;
+#endif
+
+ if (!flag) {
+ Serial.print(F("Timer Error: "));
+ Serial.println(F("not defined"));
+ }
+}
+
+void dumpTimerPin() {
+ Serial.print(F("IR Send Pin: "));
+#if defined(IR_SEND_PIN)
+ Serial.println(IR_SEND_PIN);
+#else
+ Serial.println(IrSender.sendPin);
+#endif
+}
+
+void dumpClock() {
+#if defined(F_CPU)
+ Serial.print(F("MCU Clock: "));
+ Serial.println(F_CPU);
+#endif
+}
+
+void dumpPlatform() {
+ Serial.print(F("MCU Platform: "));
+
+#if defined(__AVR_ATmega8__)
+ Serial.println(F("Atmega8"));
+#elif defined(__AVR_ATmega16__)
+ Serial.println(F("ATmega16"));
+#elif defined(__AVR_ATmega32__)
+ Serial.println(F("ATmega32"));
+#elif defined(__AVR_ATmega32U4__)
+ Serial.println(F("Arduino Leonardo / Yun / Teensy 1.0 / ATmega32U4"));
+#elif defined(__AVR_ATmega48__) || defined(__AVR_ATmega48P__)
+ Serial.println(F("ATmega48"));
+#elif defined(__AVR_ATmega64__)
+ Serial.println(F("ATmega64"));
+#elif defined(__AVR_ATmega88__) || defined(__AVR_ATmega88P__)
+ Serial.println(F("ATmega88"));
+#elif defined(__AVR_ATmega162__)
+ Serial.println(F("ATmega162"));
+#elif defined(__AVR_ATmega164A__) || defined(__AVR_ATmega164P__)
+ Serial.println(F("ATmega164"));
+#elif defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) || defined(__AVR_ATmega324PA__)
+ Serial.println(F("ATmega324"));
+
+#elif defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__)
+ Serial.println(F("ATmega644"));
+#elif defined(__AVR_ATmega1280__)
+ Serial.println(F("Arduino Mega1280"));
+#elif defined(__AVR_ATmega1281__)
+ Serial.println(F("ATmega1281"));
+#elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__)
+ Serial.println(F("ATmega1284"));
+#elif defined(__AVR_ATmega2560__)
+ Serial.println(F("Arduino Mega2560"));
+#elif defined(__AVR_ATmega2561__)
+ Serial.println(F("ATmega2561"));
+
+#elif defined(__AVR_ATmega8515__)
+ Serial.println(F("ATmega8515"));
+#elif defined(__AVR_ATmega8535__)
+ Serial.println(F("ATmega8535"));
+
+#elif defined(__AVR_AT90USB162__)
+ Serial.println(F("Teensy 1.0 / AT90USB162"));
+ // Teensy 2.0
+#elif defined(__MK20DX128__) || defined(__MK20DX256__)
+ Serial.println(F("Teensy 3.0 / Teensy 3.1 / MK20DX128 / MK20DX256"));
+#elif defined(__MKL26Z64__)
+ Serial.println(F("Teensy-LC / MKL26Z64"));
+#elif defined(__AVR_AT90USB646__)
+ Serial.println(F("Teensy++ 1.0 / AT90USB646"));
+#elif defined(__AVR_AT90USB1286__)
+ Serial.println(F("Teensy++ 2.0 / AT90USB1286"));
+
+#elif defined(__AVR_ATtiny84__)
+ Serial.println(F("ATtiny84"));
+#elif defined(__AVR_ATtiny85__)
+ Serial.println(F("ATtiny85"));
+#else
+ Serial.println(F("ATmega328(P) / (Duemilanove, Diecimila, LilyPad, Mini, Micro, Fio, Nano, etc)"));
+#endif
+}
+
+void dumpPulseParams() {
+ Serial.print(F("Mark Excess: "));
+ Serial.print(MARK_EXCESS_MICROS);
+ ;
+ Serial.println(F(" uSecs"));
+ Serial.print(F("Microseconds per tick: "));
+ Serial.print(MICROS_PER_TICK);
+ ;
+ Serial.println(F(" uSecs"));
+ Serial.print(F("Measurement tolerance: "));
+ Serial.print(TOLERANCE_FOR_DECODERS_MARK_OR_SPACE_MATCHING);
+ Serial.println(F("%"));
+}
+
+void dumpSignalParams() {
+ Serial.print(F("Minimum Gap between IR Signals: "));
+ Serial.print(RECORD_GAP_MICROS);
+ Serial.println(F(" uSecs"));
+}
+
+void dumpDebugMode() {
+ Serial.print(F("Debug Mode: "));
+#if DEBUG
+ Serial.println(F("ON"));
+#else
+ Serial.println(F("OFF (Normal)"));
+#endif
+
+}
+
+void dumpArduinoIDE() {
+ Serial.print(F("Arduino IDE version: "));
+ Serial.print(ARDUINO / 10000);
+ Serial.write('.');
+ Serial.print((ARDUINO % 10000) / 100);
+ Serial.write('.');
+ Serial.println(ARDUINO % 100);
+}
+
+void dumpProtocols() {
+
+ Serial.println();
+ Serial.print(F("IR PROTOCOLS "));
+ Serial.print(F("SEND "));
+ Serial.println(F("DECODE"));
+ Serial.print(F("============= "));
+ Serial.print(F("======== "));
+ Serial.println(F("========"));
+ Serial.print(F("RC5: "));
+#if defined(DECODE_RC5)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("RC6: "));
+#if defined(DECODE_RC6)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("NEC: "));
+#if defined(DECODE_NEC)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("SONY: "));
+#if defined(DECODE_SONY)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("PANASONIC: "));
+#if defined(DECODE_PANASONIC)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("JVC: "));
+#if defined(DECODE_JVC)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("SAMSUNG: "));
+#if defined(DECODE_SAMSUNG)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("LG: "));
+#if defined(DECODE_LG)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("DENON: "));
+#if defined(DECODE_DENON)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+#if !defined(EXCLUDE_EXOTIC_PROTOCOLS) // saves around 2000 bytes program memory
+
+ Serial.print(F("BANG_OLUFSEN: "));
+#if defined(DECODE_BEO)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("BOSEWAVE: "));
+#if defined(DECODE_BOSEWAVE)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("WHYNTER: "));
+#if defined(DECODE_WHYNTER)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+
+ Serial.print(F("FAST: "));
+#if defined(DECODE_FAST)
+ Serial.println(F("Enabled"));
+#else
+ Serial.println(F("Disabled"));
+#endif
+#endif
+}
+
+void printDecodeEnabled(int flag) {
+ if (flag) {
+ Serial.println(F("Enabled"));
+ } else {
+ Serial.println(F("Disabled"));
+ }
+}
+
+void dumpHeader() {
+ Serial.println(F("IRremoteInfo - by AnalysIR (http://www.AnalysIR.com/)"));
+ Serial.println(
+ F(
+ "- A helper sketch to assist in troubleshooting issues with the library by reviewing the settings within the IRremote library"));
+ Serial.println(
+ F(
+ "- Prints out the important settings within the library, which can be configured to suit the many supported platforms"));
+ Serial.println(F("- When seeking on-line support, please post or upload the output of this sketch, where appropriate"));
+ Serial.println();
+ Serial.println(F("IRremote Library Settings"));
+ Serial.println(F("========================="));
+}
+
+void dumpFooter() {
+ Serial.println();
+ Serial.println(F("Notes: "));
+ Serial.println(F("- Most of the settings above can be configured in the following files included as part of the library"));
+ Serial.println(F("- IRremoteInt.h"));
+ Serial.println(F("- IRremote.h"));
+ Serial.println(
+ F("- You can save SRAM by disabling the Decode or Send features for any protocol (Near the top of IRremoteInt.h)"));
+ Serial.println(
+ F(
+ "- Some Timer conflicts, with other libraries, can be easily resolved by configuring a different Timer for your platform"));
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRsendDemo/IRsendDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRsendDemo/IRsendDemo.ino
deleted file mode 100644
index 5fd80d18..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRsendDemo/IRsendDemo.ino
+++ /dev/null
@@ -1,30 +0,0 @@
-/*
- * IRremote: IRsendDemo - demonstrates sending IR codes with IRsend
- * An IR LED must be connected to Arduino PWM pin 3.
- * Microduino Core:D3
- * Microduino Core+:D8
- * Microduino CoreUSB:D6
- * Microduino CoreRF:D5
- * Version 0.1 July, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- */
-
-#include
-
-IRsend irsend;
-
-void setup()
-{
- Serial.begin(9600);
-}
-
-void loop() {
- if (Serial.read() != -1) {
- for (int i = 0; i < 3; i++) {
- irsend.sendSony(0xa90, 12); // Sony TV power code
- delay(100);
- }
- }
-}
-
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/IRtest/IRtest.ino b/libraries/_07_m_Sensor_IR_Remote/examples/IRtest/IRtest.ino
deleted file mode 100644
index 7a2dfa2e..00000000
--- a/libraries/_07_m_Sensor_IR_Remote/examples/IRtest/IRtest.ino
+++ /dev/null
@@ -1,195 +0,0 @@
-/*
- * IRremote: IRtest unittest
- * Version 0.1 July, 2009
- * Copyright 2009 Ken Shirriff
- * http://arcfn.com
- *
- * An IR LED must be connected to Arduino PWM pin 3.
- * Microduino Core:D3
- * Microduino Core+:D8
- * Microduino CoreUSB:D6
- * Microduino CoreRF:D5
- * Note: to run these tests, edit IRremote/IRremote.h to add "#define TEST"
- * You must then recompile the library by removing IRremote.o and restarting
- * the arduino IDE.
- */
-
-#include
-#include
-
-// Dumps out the decode_results structure.
-// Call this after IRrecv::decode()
-// void * to work around compiler issue
-//void dump(void *v) {
-// decode_results *results = (decode_results *)v
-void dump(decode_results *results) {
- int count = results->rawlen;
- if (results->decode_type == UNKNOWN) {
- Serial.println("Could not decode message");
- }
- else {
- if (results->decode_type == NEC) {
- Serial.print("Decoded NEC: ");
- }
- else if (results->decode_type == SONY) {
- Serial.print("Decoded SONY: ");
- }
- else if (results->decode_type == RC5) {
- Serial.print("Decoded RC5: ");
- }
- else if (results->decode_type == RC6) {
- Serial.print("Decoded RC6: ");
- }
- Serial.print(results->value, HEX);
- Serial.print(" (");
- Serial.print(results->bits, DEC);
- Serial.println(" bits)");
- }
- Serial.print("Raw (");
- Serial.print(count, DEC);
- Serial.print("): ");
-
- for (int i = 0; i < count; i++) {
- if ((i % 2) == 1) {
- Serial.print(results->rawbuf[i]*USECPERTICK, DEC);
- }
- else {
- Serial.print(-(int)results->rawbuf[i]*USECPERTICK, DEC);
- }
- Serial.print(" ");
- }
- Serial.println("");
-}
-
-IRrecv irrecv(0);
-decode_results results;
-
-class IRsendDummy :
-public IRsend
-{
-public:
- // For testing, just log the marks/spaces
-#define SENDLOG_LEN 128
- int sendlog[SENDLOG_LEN];
- int sendlogcnt;
- IRsendDummy() :
- IRsend() {
- }
- void reset() {
- sendlogcnt = 0;
- }
- void mark(int time) {
- sendlog[sendlogcnt] = time;
- if (sendlogcnt < SENDLOG_LEN) sendlogcnt++;
- }
- void space(int time) {
- sendlog[sendlogcnt] = -time;
- if (sendlogcnt < SENDLOG_LEN) sendlogcnt++;
- }
- // Copies the dummy buf into the interrupt buf
- void useDummyBuf() {
- int last = SPACE;
- irparams.rcvstate = STATE_STOP;
- irparams.rawlen = 1; // Skip the gap
- for (int i = 0 ; i < sendlogcnt; i++) {
- if (sendlog[i] < 0) {
- if (last == MARK) {
- // New space
- irparams.rawbuf[irparams.rawlen++] = (-sendlog[i] - MARK_EXCESS) / USECPERTICK;
- last = SPACE;
- }
- else {
- // More space
- irparams.rawbuf[irparams.rawlen - 1] += -sendlog[i] / USECPERTICK;
- }
- }
- else if (sendlog[i] > 0) {
- if (last == SPACE) {
- // New mark
- irparams.rawbuf[irparams.rawlen++] = (sendlog[i] + MARK_EXCESS) / USECPERTICK;
- last = MARK;
- }
- else {
- // More mark
- irparams.rawbuf[irparams.rawlen - 1] += sendlog[i] / USECPERTICK;
- }
- }
- }
- if (irparams.rawlen % 2) {
- irparams.rawlen--; // Remove trailing space
- }
- }
-};
-
-IRsendDummy irsenddummy;
-
-void verify(unsigned long val, int bits, int type) {
- irsenddummy.useDummyBuf();
- irrecv.decode(&results);
- Serial.print("Testing ");
- Serial.print(val, HEX);
- if (results.value == val && results.bits == bits && results.decode_type == type) {
- Serial.println(": OK");
- }
- else {
- Serial.println(": Error");
- dump(&results);
- }
-}
-
-void testNEC(unsigned long val, int bits) {
- irsenddummy.reset();
- irsenddummy.sendNEC(val, bits);
- verify(val, bits, NEC);
-}
-void testSony(unsigned long val, int bits) {
- irsenddummy.reset();
- irsenddummy.sendSony(val, bits);
- verify(val, bits, SONY);
-}
-void testRC5(unsigned long val, int bits) {
- irsenddummy.reset();
- irsenddummy.sendRC5(val, bits);
- verify(val, bits, RC5);
-}
-void testRC6(unsigned long val, int bits) {
- irsenddummy.reset();
- irsenddummy.sendRC6(val, bits);
- verify(val, bits, RC6);
-}
-
-void test() {
- Serial.println("NEC tests");
- testNEC(0x00000000, 32);
- testNEC(0xffffffff, 32);
- testNEC(0xaaaaaaaa, 32);
- testNEC(0x55555555, 32);
- testNEC(0x12345678, 32);
- Serial.println("Sony tests");
- testSony(0xfff, 12);
- testSony(0x000, 12);
- testSony(0xaaa, 12);
- testSony(0x555, 12);
- testSony(0x123, 12);
- Serial.println("RC5 tests");
- testRC5(0xfff, 12);
- testRC5(0x000, 12);
- testRC5(0xaaa, 12);
- testRC5(0x555, 12);
- testRC5(0x123, 12);
- Serial.println("RC6 tests");
- testRC6(0xfffff, 20);
- testRC6(0x00000, 20);
- testRC6(0xaaaaa, 20);
- testRC6(0x55555, 20);
- testRC6(0x12345, 20);
-}
-
-void setup()
-{
- Serial.begin(9600);
- test();
-}
-
-void loop() {
-}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/MicroGirs.ino b/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/MicroGirs.ino
new file mode 100644
index 00000000..a840bf24
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/MicroGirs.ino
@@ -0,0 +1,398 @@
+/**
+ * @file MicroGirs.ino
+ *
+ * @brief This is a minimalistic Girs server.
+ * It only depends on (a subset of) IRremote. It can be used with
+ * IrScrutinizer
+ * (select Sending/Capturing hw = Girs Client) as well as
+ * Lirc
+ * version 0.9.4 and later, using the driver Girs).
+ * (Authors of similar software are encourage to implement support.)
+ *
+ * It runs on all hardware on which IRemote runs.
+ *
+ * It consists of an interactive IR server, taking one-line commands from
+ * the "user" (which is typically not a person but another program), and
+ * responds with a one-line response. In the language of the Girs
+ * specifications, the modules "base", receive, and transmit are
+ * implemented. (The two latter can be disabled by not defining two
+ * corresponding CPP symbols.)
+ *
+ * It understands the following commands:
+ *
+ * The "version" command returns the program name and version,
+ * The "modules" command returns the modules implemented, normally base, receive and transmit.
+ * The "receive" command reads an IR signal using the used, demodulating IR sensor.
+ * The "send" commands transmits a supplied raw signal the requested number of times.
+ *
+ * Only the first character of the command is evaluated in this implementation.
+ *
+ * The "receive" command returns the received IR sequence as a sequence
+ * of durations, including a (dummy) trailing silence. On-periods
+ * ("marks", "flashes") are prefixed by "+", while off-periods ("spaces",
+ * "gaps") are prefixed by "-". The present version never times out.
+ *
+ * The \c send command takes the following parameters:
+ *
+ * send noSends frequencyHz introLength repeatLength endingLength durations...
+
+ * where
+ *
+ * * frequencyHz denotes the modulation frequency in Hz
+ * (\e not khz, as is normally used in IRremote)
+ * * introLength denotes the length of the intro sequence, must be even,
+ * * repeatLength denotes the length of the repeat sequence, must be even,
+ * * endingLength denotes the length of the ending sequence (normally 0), must be even.
+ * * duration... denotes the microsecond durations to send,
+ * starting with the first on-period, ending with a (possibly dummy) trailing silence
+ *
+ * Semantics: first the intro sequence will be sent once (i.e., the first
+ * repeatLength durations) (if non-empty). Then the repeat sequence will
+ * be sent (noSends-1) times, unless the intro sequence was empty, in
+ * which case it will be send noSends times. Finally, the ending
+ * sequence will be send once (if non-empty).
+ *
+ * Weaknesses of the IRremote implementation:
+ * * Reception never times out if no IR is seen.
+ * * The IRrecv class does its own decoding which is irrelevant for us.
+ * * The timeout at the end on a signal reception is not configurable.
+ * For example, a NEC1 type signal will cut after the intro sequence,
+ * and the repeats will be considered independent signals.
+ * In IrSqrutinizer, recognition of repeating signals will therefore not work.
+ * The size of the data is platform dependent ("unsigned int", which is 16 bit on AVR boards, 32 bits on 32 bit boards).
+ *
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 180 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# elif RAMEND <= 0x8FF || RAMSIZE < 0x8FF
+#define RAW_BUFFER_LENGTH 500 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# else
+#define RAW_BUFFER_LENGTH 750 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# endif
+#endif
+
+/**
+ * Baud rate for the serial/USB connection.
+ * (115200 is the default for IrScrutinizer and Lirc.)
+ */
+#define BAUDRATE 115200
+#define NO_DECODER
+
+#include "IRremote.hpp"
+#include
+
+/**
+ * Define to support reception of IR.
+ */
+#define RECEIVE
+
+/**
+ * Define to support transmission of IR signals.
+ */
+#define TRANSMIT
+
+// (The sending pin is in general not configurable, see the documentation of IRremote.)
+
+/**
+ * Character that ends the command lines. Do not change unless you known what
+ * you are doing. IrScrutinizer and Lirc expects \r.
+ */
+#define EOLCHAR '\r'
+
+////// End of user configurable variables ////////////////////
+
+/**
+ * The modules supported, as given by the "modules" command.
+ */
+#if defined(TRANSMIT)
+#if defined(RECEIVE)
+#define modulesSupported "base transmit receive"
+#else // ! RECEIVE
+#define modulesSupported "base transmit"
+#endif
+#else // !TRANSMIT
+#if defined(RECEIVE)
+#define modulesSupported "base receive"
+#else // ! RECETVE
+#error At lease one of TRANSMIT and RECEIVE must be defined
+#endif
+#endif
+
+/**
+ * Name of program, as reported by the "version" command.
+ */
+#define PROGNAME "MicroGirs"
+
+/**
+ * Version of program, as reported by the "version" command.
+ */
+#define VERSION "2020-07-05"
+
+#define okString "OK"
+#define errorString "ERROR"
+#define timeoutString "."
+
+// For compatibility with IRremote, we deliberately use
+// the platform dependent types.
+// (Although it is a questionable idea ;-) )
+/**
+ * Type used for modulation frequency in Hz (\e not kHz).
+ */
+typedef unsigned frequency_t; // max 65535, unless 32-bit
+
+/**
+ * Type used for durations in micro seconds.
+ */
+typedef uint16_t microseconds_t; // max 65535
+
+static const microseconds_t DUMMYENDING = 40000U;
+static const frequency_t FREQUENCY_T_MAX = UINT16_MAX;
+static const frequency_t MICROSECONDS_T_MAX = UINT16_MAX;
+
+/**
+ * Our own tokenizer class. Breaks the command line into tokens.
+ * Usage outside of this package is discouraged.
+ */
+class Tokenizer {
+private:
+ static const int invalidIndex = -1;
+
+ int index; // signed since invalidIndex is possible
+ const String &payload;
+ void trim();
+
+public:
+ Tokenizer(const String &str);
+
+ String getToken();
+ String getRest();
+ String getLine();
+ long getInt();
+ microseconds_t getMicroseconds();
+ frequency_t getFrequency();
+
+ static const int invalid = INT_MAX;
+};
+
+Tokenizer::Tokenizer(const String &str) :
+ index(0), payload(str) {
+}
+
+String Tokenizer::getRest() {
+ String result = index == invalidIndex ? String("") : payload.substring(index);
+ index = invalidIndex;
+ return result;
+}
+
+String Tokenizer::getLine() {
+ if (index == invalidIndex)
+ return String("");
+
+ int i = payload.indexOf('\n', index);
+ String s = (i > 0) ? payload.substring(index, i) : payload.substring(index);
+ index = (i > 0) ? i + 1 : invalidIndex;
+ return s;
+}
+
+String Tokenizer::getToken() {
+ if (index < 0)
+ return String("");
+
+ int i = payload.indexOf(' ', index);
+ String s = (i > 0) ? payload.substring(index, i) : payload.substring(index);
+ index = (i > 0) ? i : invalidIndex;
+ if (index != invalidIndex)
+ if (index != invalidIndex)
+ while (payload.charAt(index) == ' ')
+ index++;
+ return s;
+}
+
+long Tokenizer::getInt() {
+ String token = getToken();
+ return token == "" ? (long) invalid : token.toInt();
+}
+
+microseconds_t Tokenizer::getMicroseconds() {
+ long t = getToken().toInt();
+ return (microseconds_t) ((t < MICROSECONDS_T_MAX) ? t : MICROSECONDS_T_MAX);
+}
+
+frequency_t Tokenizer::getFrequency() {
+ long t = getToken().toInt();
+ return (frequency_t) ((t < FREQUENCY_T_MAX) ? t : FREQUENCY_T_MAX);
+}
+///////////////// end Tokenizer /////////////////////////////////
+
+#if defined(TRANSMIT)
+static inline unsigned hz2khz(frequency_t hz) {
+ return (hz + 500) / 1000;
+}
+
+/**
+ * Transmits the IR signal given as argument.
+ *
+ * The intro sequence (possibly empty) is first sent. Then the repeat signal
+ * (also possibly empty) is sent, "times" times, except for the case of
+ * the intro signal being empty, in which case it is sent "times" times.
+ * Finally the ending sequence (possibly empty) is sent.
+ *
+ * @param intro Sequence to be sent exactly once at the start.
+ * @param lengthIntro Number of durations in intro sequence, must be even.
+ * @param repeat Sequence top possibly be sent multiple times
+ * @param lengthRepeat Number of durations in repeat sequence.
+ * @param ending Sequence to be sent at the end, possibly empty
+ * @param lengthEnding Number of durations in ending sequence
+ * @param frequency Modulation frequency, in Hz (not kHz as normally in IRremote)
+ * @param times Number of times to send the signal, in the sense above.
+ */
+static void sendRaw(const microseconds_t intro[], unsigned lengthIntro, const microseconds_t repeat[], unsigned lengthRepeat,
+ const microseconds_t ending[], unsigned lengthEnding, frequency_t frequency, unsigned times) {
+ if (lengthIntro > 0U)
+ IrSender.sendRaw(intro, lengthIntro, hz2khz(frequency));
+ if (lengthRepeat > 0U)
+ for (unsigned i = 0U; i < times - (lengthIntro > 0U); i++)
+ IrSender.sendRaw(repeat, lengthRepeat, hz2khz(frequency));
+ if (lengthEnding > 0U)
+ IrSender.sendRaw(ending, lengthEnding, hz2khz(frequency));
+}
+#endif // TRANSMIT
+
+#if defined(RECEIVE)
+
+static void dump(Stream &stream) {
+ unsigned int count = IrReceiver.decodedIRData.rawDataPtr->rawlen;
+ // If buffer gets full, count = RAW_BUFFER_LENGTH, which is odd,
+ // and IrScrutinizer does not like that.
+ count &= ~1;
+ for (unsigned int i = 1; i < count; i++) {
+ stream.write(i & 1 ? '+' : '-');
+ stream.print(IrReceiver.decodedIRData.rawDataPtr->rawbuf[i] * MICROS_PER_TICK, DEC);
+ stream.print(" ");
+ }
+ stream.print('-');
+ stream.println(DUMMYENDING);
+}
+
+/**
+ * Reads a command from the stream given as argument.
+ * @param stream Stream to read from, typically Serial.
+ */
+static void receive(Stream &stream) {
+ IrReceiver.start();
+
+ while (!IrReceiver.decode()) {
+ }
+ IrReceiver.stop();
+
+ dump(stream);
+}
+
+#endif // RECEIVE
+
+/**
+ * Initialization.
+ */
+void setup() {
+ Serial.begin(BAUDRATE);
+ while (!Serial)
+ ; // wait for serial port to connect.
+
+ Serial.println(F(PROGNAME " " VERSION));
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+#if defined(RECEIVE)
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.print(F("at pin "));
+#endif
+
+#if defined(IR_SEND_PIN)
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+#else
+ IrSender.begin(3, ENABLE_LED_FEEDBACK, USE_DEFAULT_FEEDBACK_LED_PIN); // Specify send pin and enable feedback LED at default feedback LED pin
+#endif
+
+}
+
+static String readCommand(Stream &stream) {
+ while (stream.available() == 0) {
+ }
+
+ String line = stream.readStringUntil(EOLCHAR);
+ line.trim();
+ return line;
+}
+
+static void processCommand(const String &line, Stream &stream) {
+ Tokenizer tokenizer(line);
+ String cmd = tokenizer.getToken();
+
+ // Decode the command in cmd
+ if (cmd.length() == 0) {
+ // empty command, do nothing
+ stream.println(F(okString));
+ return;
+ }
+
+ switch (cmd[0]) {
+ case 'm':
+ stream.println(F(modulesSupported));
+ break;
+
+#if defined(RECEIVE)
+ case 'r': // receive
+ //case 'a':
+ //case 'c':
+ receive(stream);
+ break;
+#endif // RECEIVE
+
+#if defined(TRANSMIT)
+ case 's': // send
+ {
+ // TODO: handle unparsable data gracefully
+ unsigned noSends = (unsigned) tokenizer.getInt();
+ frequency_t frequency = tokenizer.getFrequency();
+ unsigned introLength = (unsigned) tokenizer.getInt();
+ unsigned repeatLength = (unsigned) tokenizer.getInt();
+ unsigned endingLength = (unsigned) tokenizer.getInt();
+ microseconds_t intro[introLength];
+ microseconds_t repeat[repeatLength];
+ microseconds_t ending[endingLength];
+ for (unsigned i = 0; i < introLength; i++)
+ intro[i] = tokenizer.getMicroseconds();
+ for (unsigned i = 0; i < repeatLength; i++)
+ repeat[i] = tokenizer.getMicroseconds();
+ for (unsigned i = 0; i < endingLength; i++)
+ ending[i] = tokenizer.getMicroseconds();
+ sendRaw(intro, introLength, repeat, repeatLength, ending, endingLength, frequency, noSends);
+ stream.println(F(okString));
+ }
+ break;
+#endif // TRANSMIT
+
+ case 'v': // version
+ stream.println(F(PROGNAME " " VERSION));
+ break;
+ default:
+ stream.println(F(errorString));
+ }
+}
+
+/**
+ * Reads a command from the serial line and executes it-
+ */
+void loop() {
+ String line = readCommand(Serial);
+ processCommand(line, Serial);
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/MicroGirs/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/ReceiveAndSend.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/ReceiveAndSend.ino
new file mode 100644
index 00000000..fdd4eaf3
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSend/ReceiveAndSend.ino
@@ -0,0 +1,242 @@
+/*
+ * ReceiveAndSend.cpp
+ *
+ * Record and play back last received IR signal at button press.
+ * The logic is:
+ * If the button is pressed, send the IR code.
+ * If an IR code is received, record it.
+ * If the protocol is unknown or not enabled, store it as raw data for later sending.
+ *
+ * An example for simultaneous receiving and sending is in the UnitTest example.
+ *
+ * An IR detector/demodulator must be connected to the input IR_RECEIVE_PIN.
+ *
+ * A button must be connected between the input SEND_BUTTON_PIN and ground.
+ * A visible LED can be connected to STATUS_PIN to provide status.
+ *
+ *
+ * Initially coded 2009 Ken Shirriff http://www.righto.com
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2009-2023 Ken Shirriff, Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+/*
+ * Specify which protocol(s) should be used for decoding.
+ * If no protocol is defined, all protocols (except Bang&Olufsen) are active.
+ * This must be done before the #include
+ */
+//#define DECODE_DENON // Includes Sharp
+//#define DECODE_JVC
+//#define DECODE_KASEIKYO
+//#define DECODE_PANASONIC // alias for DECODE_KASEIKYO
+//#define DECODE_LG
+#define DECODE_NEC // Includes Apple and Onkyo
+//#define DECODE_SAMSUNG
+//#define DECODE_SONY
+//#define DECODE_RC5
+//#define DECODE_RC6
+
+//#define DECODE_BOSEWAVE
+//#define DECODE_LEGO_PF
+//#define DECODE_MAGIQUEST
+//#define DECODE_WHYNTER
+//#define DECODE_FAST
+//
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 120
+# elif RAMEND <= 0xAFF || RAMSIZE < 0xAFF // 0xAFF for LEONARDO
+#define RAW_BUFFER_LENGTH 400 // 600 is too much here, because we have additional uint8_t rawCode[RAW_BUFFER_LENGTH];
+# else
+#define RAW_BUFFER_LENGTH 750
+# endif
+#endif
+
+//#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+//#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 650 bytes program memory if all other protocols are active
+//#define NO_LED_FEEDBACK_CODE // saves 92 bytes program memory
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols
+//#define SEND_PWM_BY_TIMER // Disable carrier PWM generation in software and use (restricted) hardware PWM.
+//#define USE_NO_SEND_PWM // Use no carrier PWM, just simulate an active low receiver signal. Overrides SEND_PWM_BY_TIMER definition
+
+// MARK_EXCESS_MICROS is subtracted from all marks and added to all spaces before decoding,
+// to compensate for the signal forming of different IR receiver modules. See also IRremote.hpp line 142.
+#define MARK_EXCESS_MICROS 20 // Adapt it to your IR receiver module. 20 is recommended for the cheap VS1838 modules.
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+#include
+
+int SEND_BUTTON_PIN = APPLICATION_PIN;
+
+int DELAY_BETWEEN_REPEAT = 50;
+
+// Storage for the recorded code
+struct storedIRDataStruct {
+ IRData receivedIRData;
+ // extensions for sendRaw
+ uint8_t rawCode[RAW_BUFFER_LENGTH]; // The durations if raw
+ uint8_t rawCodeLength; // The length of the code
+} sStoredIRData;
+
+bool sSendButtonWasActive;
+
+void storeCode();
+void sendCode(storedIRDataStruct *aIRDataToSend);
+
+void setup() {
+ pinMode(SEND_BUTTON_PIN, INPUT_PULLUP);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+ Serial.print(F("Ready to send IR signals at pin " STR(IR_SEND_PIN) " on press of button at pin "));
+ Serial.println(SEND_BUTTON_PIN);
+}
+
+void loop() {
+
+ // If button pressed, send the code.
+ bool tSendButtonIsActive = (digitalRead(SEND_BUTTON_PIN) == LOW); // Button pin is active LOW
+
+ /*
+ * Check for current button state
+ */
+ if (tSendButtonIsActive) {
+ if (!sSendButtonWasActive) {
+ Serial.println(F("Stop receiving"));
+ IrReceiver.stop();
+ }
+ /*
+ * Button pressed -> send stored data
+ */
+ Serial.print(F("Button pressed, now sending "));
+ if (sSendButtonWasActive == tSendButtonIsActive) {
+ Serial.print(F("repeat "));
+ sStoredIRData.receivedIRData.flags = IRDATA_FLAGS_IS_REPEAT;
+ } else {
+ sStoredIRData.receivedIRData.flags = IRDATA_FLAGS_EMPTY;
+ }
+ Serial.flush(); // To avoid disturbing the software PWM generation by serial output interrupts
+ sendCode(&sStoredIRData);
+ delay(DELAY_BETWEEN_REPEAT); // Wait a bit between retransmissions
+
+ } else if (sSendButtonWasActive) {
+ /*
+ * Button is just released -> activate receiving
+ */
+ // Restart receiver
+ Serial.println(F("Button released -> start receiving"));
+ IrReceiver.start();
+
+ } else if (IrReceiver.decode()) {
+ /*
+ * Button is not pressed and data available -> store received data and resume
+ */
+ storeCode();
+ IrReceiver.resume(); // resume receiver
+ }
+
+ sSendButtonWasActive = tSendButtonIsActive;
+ delay(100);
+}
+
+// Stores the code for later playback in sStoredIRData
+// Most of this code is just logging
+void storeCode() {
+ if (IrReceiver.decodedIRData.rawDataPtr->rawlen < 4) {
+ Serial.print(F("Ignore data with rawlen="));
+ Serial.println(IrReceiver.decodedIRData.rawDataPtr->rawlen);
+ return;
+ }
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_IS_REPEAT) {
+ Serial.println(F("Ignore repeat"));
+ return;
+ }
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_IS_AUTO_REPEAT) {
+ Serial.println(F("Ignore autorepeat"));
+ return;
+ }
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_PARITY_FAILED) {
+ Serial.println(F("Ignore parity error"));
+ return;
+ }
+ /*
+ * Copy decoded data
+ */
+ sStoredIRData.receivedIRData = IrReceiver.decodedIRData;
+
+ if (sStoredIRData.receivedIRData.protocol == UNKNOWN) {
+ Serial.print(F("Received unknown code and store "));
+ Serial.print(IrReceiver.decodedIRData.rawDataPtr->rawlen - 1);
+ Serial.println(F(" timing entries as raw "));
+ IrReceiver.printIRResultRawFormatted(&Serial, true); // Output the results in RAW format
+ sStoredIRData.rawCodeLength = IrReceiver.decodedIRData.rawDataPtr->rawlen - 1;
+ /*
+ * Store the current raw data in a dedicated array for later usage
+ */
+ IrReceiver.compensateAndStoreIRResultInArray(sStoredIRData.rawCode);
+ } else {
+ IrReceiver.printIRResultShort(&Serial);
+ IrReceiver.printIRSendUsage(&Serial);
+ sStoredIRData.receivedIRData.flags = 0; // clear flags -esp. repeat- for later sending
+ Serial.println();
+ }
+}
+
+void sendCode(storedIRDataStruct *aIRDataToSend) {
+ if (aIRDataToSend->receivedIRData.protocol == UNKNOWN /* i.e. raw */) {
+ // Assume 38 KHz
+ IrSender.sendRaw(aIRDataToSend->rawCode, aIRDataToSend->rawCodeLength, 38);
+
+ Serial.print(F("raw "));
+ Serial.print(aIRDataToSend->rawCodeLength);
+ Serial.println(F(" marks or spaces"));
+ } else {
+
+ /*
+ * Use the write function, which does the switch for different protocols
+ */
+ IrSender.write(&aIRDataToSend->receivedIRData);
+ printIRResultShort(&Serial, &aIRDataToSend->receivedIRData, false);
+ }
+}
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/ReceiveAndSendDistanceWidth.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/ReceiveAndSendDistanceWidth.ino
new file mode 100644
index 00000000..5c2e7355
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveAndSendDistanceWidth/ReceiveAndSendDistanceWidth.ino
@@ -0,0 +1,184 @@
+/*
+ * ReceiveAndSendDistanceWidth.cpp
+ *
+ * Record and play back last received distance width IR signal at button press.
+ * Using DistanceWidthProtocol covers a lot of known and unknown IR protocols,
+ * and requires less memory than raw protocol.
+ *
+ * The logic is:
+ * If the button is pressed, send the IR code.
+ * If an IR code is received, record it.
+ *
+ * An example for simultaneous receiving and sending is in the UnitTest example.
+ *
+ * An IR detector/demodulator must be connected to the input IR_RECEIVE_PIN.
+ *
+ * A button must be connected between the input SEND_BUTTON_PIN and ground.
+ * A visible LED can be connected to STATUS_PIN to provide status.
+ *
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#if !defined(IR_SEND_PIN)
+#define IR_SEND_PIN 3
+#endif
+
+/*
+ * Specify DistanceWidthProtocol for decoding. This must be done before the #include
+ */
+#define DECODE_DISTANCE_WIDTH // Universal decoder for pulse distance width protocols
+//
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 120
+# elif RAMEND <= 0xAFF || RAMSIZE < 0xAFF // 0xAFF for LEONARDO
+#define RAW_BUFFER_LENGTH 400 // 600 is too much here, because we have additional uint8_t rawCode[RAW_BUFFER_LENGTH];
+# else
+#define RAW_BUFFER_LENGTH 750
+# endif
+#endif
+
+//#define NO_LED_FEEDBACK_CODE // saves 92 bytes program memory
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols
+//#define SEND_PWM_BY_TIMER // Disable carrier PWM generation in software and use (restricted) hardware PWM.
+//#define USE_NO_SEND_PWM // Use no carrier PWM, just simulate an active low receiver signal. Overrides SEND_PWM_BY_TIMER definition
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+#include
+
+#define SEND_BUTTON_PIN APPLICATION_PIN
+
+#define DELAY_BETWEEN_REPEATS_MILLIS 70
+
+// Storage for the recorded code, pre-filled with NEC data
+IRRawDataType sDecodedRawDataArray[RAW_DATA_ARRAY_SIZE] = { 0x7B34ED12 }; // address 0x12 command 0x34
+DistanceWidthTimingInfoStruct sDistanceWidthTimingInfo = { 9000, 4500, 560, 1690, 560, 560 }; // NEC timing
+uint8_t sNumberOfBits = 32;
+
+bool sSendButtonWasActive;
+
+void setup() {
+ pinMode(SEND_BUTTON_PIN, INPUT_PULLUP);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+ Serial.println(F("Ready to receive pulse distance/width coded IR signals at pin " STR(IR_RECEIVE_PIN)));
+
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+ Serial.print(F("Ready to send IR signals at pin " STR(IR_SEND_PIN) " on press of button at pin "));
+ Serial.println(SEND_BUTTON_PIN);
+}
+
+void loop() {
+
+ // If button pressed, send the code.
+ bool tSendButtonIsActive = (digitalRead(SEND_BUTTON_PIN) == LOW); // Button pin is active LOW
+
+ /*
+ * Check for current button state
+ */
+ if (tSendButtonIsActive) {
+ if (!sSendButtonWasActive) {
+ Serial.println(F("Stop receiving"));
+ IrReceiver.stop();
+ }
+ /*
+ * Button pressed -> send stored data
+ */
+ Serial.print(F("Button pressed, now sending "));
+ Serial.print(sNumberOfBits);
+ Serial.print(F(" bits 0x"));
+ Serial.print(sDecodedRawDataArray[0], HEX);
+ Serial.print(F(" with sendPulseDistanceWidthFromArray timing="));
+ IrReceiver.printDistanceWidthTimingInfo(&Serial, &sDistanceWidthTimingInfo);
+ Serial.println();
+ Serial.flush(); // To avoid disturbing the software PWM generation by serial output interrupts
+
+ IrSender.sendPulseDistanceWidthFromArray(38, &sDistanceWidthTimingInfo, &sDecodedRawDataArray[0], sNumberOfBits,
+#if defined(USE_MSB_DECODING_FOR_DISTANCE_DECODER)
+ PROTOCOL_IS_MSB_FIRST
+#else
+ PROTOCOL_IS_LSB_FIRST
+#endif
+ , 100, 0);
+
+ delay(DELAY_BETWEEN_REPEATS_MILLIS); // Wait a bit between retransmissions
+
+ } else if (sSendButtonWasActive) {
+ /*
+ * Button is just released -> activate receiving
+ */
+ // Restart receiver
+ Serial.println(F("Button released -> start receiving"));
+ IrReceiver.start();
+
+ } else if (IrReceiver.decode()) {
+ /*
+ * Button is not pressed and data available -> store received data and resume
+ * DistanceWidthTimingInfo and sNumberOfBits should be constant for all keys of the same IR remote / protocol
+ */
+ IrReceiver.printIRResultShort(&Serial);
+ if (IrReceiver.decodedIRData.protocol != UNKNOWN) {
+ IrReceiver.printIRSendUsage(&Serial);
+
+ if (memcmp(&sDistanceWidthTimingInfo, &IrReceiver.decodedIRData.DistanceWidthTimingInfo,
+ sizeof(sDistanceWidthTimingInfo)) != 0) {
+ Serial.print(F("Store new timing info data="));
+ IrReceiver.printDistanceWidthTimingInfo(&Serial, &IrReceiver.decodedIRData.DistanceWidthTimingInfo);
+ Serial.println();
+ sDistanceWidthTimingInfo = IrReceiver.decodedIRData.DistanceWidthTimingInfo; // copy content here
+ } else {
+ Serial.print(F("Timing did not change, so we can reuse already stored timing info."));
+ }
+ if (sNumberOfBits != IrReceiver.decodedIRData.numberOfBits) {
+ Serial.print(F("Store new numberOfBits="));
+ sNumberOfBits = IrReceiver.decodedIRData.numberOfBits;
+ Serial.println(IrReceiver.decodedIRData.numberOfBits);
+ }
+ if (sDecodedRawDataArray[0] != IrReceiver.decodedIRData.decodedRawDataArray[0]) {
+ *sDecodedRawDataArray = *IrReceiver.decodedIRData.decodedRawDataArray; // copy content here
+ Serial.print(F("Store new sDecodedRawDataArray[0]=0x"));
+ Serial.println(IrReceiver.decodedIRData.decodedRawDataArray[0], HEX);
+ }
+ }
+ IrReceiver.resume(); // resume receiver
+ }
+
+ sSendButtonWasActive = tSendButtonIsActive;
+ delay(100);
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/IRremote_SendDemo_ReceiveDemo.log b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/IRremote_SendDemo_ReceiveDemo.log
new file mode 100644
index 00000000..d56a9481
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/IRremote_SendDemo_ReceiveDemo.log
@@ -0,0 +1,178 @@
+START ../src/ReceiveDemo.cpp from Feb 24 2023
+Using library version 4.1.0
+Enabling IRin...
+Ready to receive IR signals of protocols: NEC/NEC2/Onkyo/Apple, Panasonic/Kaseikyo, Denon/Sharp, Sony, RC5, RC6, LG, JVC, Samsung, FAST, Whynter, Lego Power Functions, Bosewave , MagiQuest, Universal Pulse Distance Width, Hash at pin 2
+
+If you connect debug pin 5 to ground, raw data is always printed
+5000 us is the (minimum) gap, after which the start of a new IR packet is assumed
+20 us are subtracted from all marks and added to all spaces for decoding
+
+Protocol=NEC Address=0x2 Command=0x34 Raw-Data=0xCB34FD02 32 bits LSB first
+Send with: IrSender.sendNEC(0x2, 0x34, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=NEC Address=0x80 Command=0x45 Raw-Data=0xBA457F80 32 bits LSB first
+Send with: IrSender.sendNEC(0x80, 0x45, );
+
+Protocol=NEC Address=0x4 Command=0x8 Raw-Data=0xF708FB04 32 bits LSB first
+Send with: IrSender.sendNEC(0x4, 0x8, );
+
+Protocol=Onkyo Address=0x102 Command=0x304 Raw-Data=0x3040102 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x102, 0x304, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=PulseDistance Raw-Data=0x5A 72 bits LSB first
+Send with:
+ uint32_t tRawData[]={0x87654321, 0xAFEDCBA9, 0x5A};
+ IrSender.sendPulseDistanceWidthFromArray(38, 8850, 4400, 550, 1700, 550, 600, &tRawData[0], 72, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=PulseWidth Raw-Data=0xDCBA9 52 bits LSB first
+Send with:
+ uint32_t tRawData[]={0x87654321, 0xDCBA9};
+ IrSender.sendPulseDistanceWidthFromArray(38, 300, 600, 600, 300, 350, 600, &tRawData[0], 52, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=PulseWidth Raw-Data=0x87654321 32 bits LSB first
+Send with: IrSender.sendPulseDistanceWidth(38, 1000, 500, 600, 300, 350, 300, 0x87654321, 32, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=Onkyo Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x102, 0x5634, );
+
+Protocol=Apple Address=0x2 Command=0x34 Raw-Data=0x23487EE 32 bits LSB first
+Send with: IrSender.sendApple(0x2, 0x34, );
+
+Protocol=Panasonic Address=0x102 Command=0x34 Raw-Data=0x4341020 48 bits LSB first
+Send with: IrSender.sendPanasonic(0x102, 0x34, );
+
+Protocol=Kaseikyo Address=0x102 Command=0x34 Extra=0x4711 Raw-Data=0x7341023 48 bits LSB first
+Send with: IrSender.sendKaseikyo(0x102, 0x34, , 0x4711);
+
+Protocol=Kaseikyo_Denon Address=0x102 Command=0x34 Raw-Data=0x4341020 48 bits LSB first
+Send with: IrSender.sendKaseikyo_Denon(0x102, 0x34, );
+
+Protocol=Denon Address=0x2 Command=0x34 Raw-Data=0x682 15 bits LSB first
+Send with: IrSender.sendDenon(0x2, 0x34, );
+
+Protocol=Denon Address=0x2 Command=0x34 Auto-Repeat gap=45650us Raw-Data=0x7962 15 bits LSB first
+
+Protocol=Sharp Address=0x2 Command=0x34 Raw-Data=0x4682 15 bits LSB first
+Send with: IrSender.sendSharp(0x2, 0x34, );
+
+Protocol=Sharp Address=0x2 Command=0x34 Auto-Repeat gap=46400us Raw-Data=0x3962 15 bits LSB first
+
+Protocol=Sony Address=0x2 Command=0x34 Raw-Data=0x134 12 bits LSB first
+Send with: IrSender.sendSony(0x2, 0x34, 2, 12);
+
+Protocol=Sony Address=0x2 Command=0x34 Raw-Data=0x134 15 bits LSB first
+Send with: IrSender.sendSony(0x2, 0x34, 2, 15);
+
+Protocol=Sony Address=0x102 Command=0x34 Raw-Data=0x8134 20 bits LSB first
+Send with: IrSender.sendSony(0x102, 0x34, 2, 20);
+
+Protocol=Samsung Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x5634, );
+
+Protocol=Samsung48 Address=0x102 Command=0x5634 Raw-Data=0xA956 48 bits LSB first
+Send with: IrSender.sendSamsung48(0x102, 0x5634, );
+
+Protocol=RC5 Address=0x2 Command=0x34 Raw-Data=0x10B4 13 bits MSB first
+Send with: IrSender.sendRC5(0x2, 0x34, );
+
+Protocol=RC5 Address=0x2 Command=0x74 Toggle=1 Raw-Data=0x8B4 13 bits MSB first
+Send with: IrSender.sendRC5(0x2, 0x74, );
+
+Protocol=RC6 Address=0x2 Command=0x34 Raw-Data=0x234 20 bits MSB first
+Send with: IrSender.sendRC6(0x2, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x34, );
+
+Protocol=JVC Address=0x2 Command=0x34 Raw-Data=0x3402 16 bits LSB first
+Send with: IrSender.sendJVC(0x2, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x5634, );
+
+Protocol=LG Address=0x2 Command=0x5634 Raw-Data=0x256342 28 bits MSB first
+Send with: IrSender.sendLG(0x2, 0x5634, );
+
+Protocol=MagiQuest Address=0x102 Command=0x34 Raw-Data=0x6BCD0102 56 bits MSB first
+Send with: IrSender.sendMagiQuest(0x6BCD0102, 0x34, );
+
+Protocol=BoseWave Address=0x0 Command=0x34 Raw-Data=0xCB34 16 bits LSB first
+Send with: IrSender.sendBoseWave(0x0, 0x34, );
+
+Protocol=FAST Address=0x0 Command=0x34 Raw-Data=0xCB34 16 bits LSB first
+Send with: IrSender.sendFAST(0x0, 0x34, );
+
+Protocol=Lego Address=0x2 Command=0x14 Raw-Data=0x2148 16 bits MSB first
+Send with: IrSender.sendLego(0x2, 0x14, );
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=180450us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179350us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179200us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179150us Raw-Data=0x2148 16 bits MSB first
+
+Overflow detected
+Try to increase the "RAW_BUFFER_LENGTH" value of 600 in ../src/ReceiveDemo.cpp
+
+Protocol=NEC Address=0x3 Command=0x45 Raw-Data=0xBA45FC03 32 bits LSB first
+Send with: IrSender.sendNEC(0x3, 0x45, );
+
+Protocol=NEC Address=0x3 Command=0x45 Repeat gap=43250us
+
+Protocol=NEC Address=0x203 Command=0x45 Raw-Data=0xBA450203 32 bits LSB first
+Send with: IrSender.sendNEC(0x203, 0x45, );
+
+Protocol=NEC Address=0x203 Command=0x45 Repeat gap=47550us
+
+Protocol=NEC Address=0x203 Command=0x45 Raw-Data=0xBA450203 32 bits LSB first
+Send with: IrSender.sendNEC(0x203, 0x45, );
+
+Protocol=NEC2 Address=0x203 Command=0x45 Repeat gap=46500us Raw-Data=0xBA450203 32 bits LSB first
+
+Protocol=Onkyo Address=0x203 Command=0x6745 Raw-Data=0x67450203 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x203, 0x6745, );
+
+Protocol=Onkyo Address=0x203 Command=0x6745 Repeat gap=46550us
+
+Protocol=Apple Address=0x3 Command=0x45 Raw-Data=0x34587EE 32 bits LSB first
+Send with: IrSender.sendApple(0x3, 0x45, );
+
+Protocol=Apple Address=0x3 Command=0x45 Repeat gap=31550us
+
+Protocol=Panasonic Address=0x203 Command=0x45 Raw-Data=0x55452030 48 bits LSB first
+Send with: IrSender.sendPanasonic(0x203, 0x45, );
+
+Protocol=Panasonic Address=0x203 Command=0x45 Repeat gap=72550us Raw-Data=0x55452030 48 bits LSB first
+
+Protocol=Kaseikyo Address=0x203 Command=0x45 Extra=0x4711 Raw-Data=0x56452033 48 bits LSB first
+Send with: IrSender.sendKaseikyo(0x203, 0x45, , 0x4711);
+
+Protocol=Kaseikyo Address=0x203 Command=0x45 Extra=0x4711 Repeat gap=66750us Raw-Data=0x56452033 48 bits LSB first
+
+Protocol=Kaseikyo_Denon Address=0x203 Command=0x45 Raw-Data=0x55452030 48 bits LSB first
+Send with: IrSender.sendKaseikyo_Denon(0x203, 0x45, );
+
+Protocol=Kaseikyo_Denon Address=0x203 Command=0x45 Repeat gap=68300us Raw-Data=0x55452030 48 bits LSB first
\ No newline at end of file
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/ReceiveDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/ReceiveDemo.ino
new file mode 100644
index 00000000..be18a649
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDemo/ReceiveDemo.ino
@@ -0,0 +1,314 @@
+/*
+ * ReceiveDemo.cpp
+ *
+ * Demonstrates receiving IR codes with the IRremote library and the use of the Arduino tone() function with this library.
+ * Long press of one IR button (receiving of multiple repeats for one command) is detected.
+ * If debug button is pressed (pin connected to ground) a long output is generated.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+//#define LOCAL_DEBUG // If defined, print timing for each received data set (the same as if DEBUG_BUTTON_PIN was connected to low)
+
+/*
+ * Specify which protocol(s) should be used for decoding.
+ * If no protocol is defined, all protocols (except Bang&Olufsen) are active.
+ * This must be done before the #include
+ */
+//#define DECODE_DENON // Includes Sharp
+//#define DECODE_JVC
+//#define DECODE_KASEIKYO
+//#define DECODE_PANASONIC // alias for DECODE_KASEIKYO
+//#define DECODE_LG
+//#define DECODE_ONKYO // Decodes only Onkyo and not NEC or Apple
+//#define DECODE_NEC // Includes Apple and Onkyo
+//#define DECODE_SAMSUNG
+//#define DECODE_SONY
+//#define DECODE_RC5
+//#define DECODE_RC6
+
+//#define DECODE_BOSEWAVE
+//#define DECODE_LEGO_PF
+//#define DECODE_MAGIQUEST
+//#define DECODE_WHYNTER
+//#define DECODE_FAST
+
+//#define DECODE_DISTANCE_WIDTH // Universal decoder for pulse distance width protocols
+//#define DECODE_HASH // special decoder for all protocols
+
+//#define DECODE_BEO // This protocol must always be enabled manually, i.e. it is NOT enabled if no protocol is defined. It prevents decoding of SONY!
+
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+// !!! Enabling B&O disables detection of Sony, because the repeat gap for SONY is smaller than the B&O frame gap :-( !!!
+//#define DECODE_BEO // Bang & Olufsen protocol always must be enabled explicitly. It has an IR transmit frequency of 455 kHz! It prevents decoding of SONY!
+#endif
+#if defined(DECODE_BEO)
+#define RECORD_GAP_MICROS 16000 // always get the complete frame in the receive buffer, but this prevents decoding of SONY!
+#endif
+// etc. see IRremote.hpp
+//
+
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 130 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 650 bytes program memory if all other protocols are active
+#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+# elif RAMEND <= 0x8FF || RAMSIZE < 0x8FF
+#define RAW_BUFFER_LENGTH 600 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# else
+#define RAW_BUFFER_LENGTH 750 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# endif
+#endif
+
+//#define NO_LED_FEEDBACK_CODE // saves 92 bytes program memory
+//#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+//#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 650 bytes program memory if all other protocols are active
+
+// MARK_EXCESS_MICROS is subtracted from all marks and added to all spaces before decoding,
+// to compensate for the signal forming of different IR receiver modules. See also IRremote.hpp line 142.
+//#define MARK_EXCESS_MICROS 20 // Adapt it to your IR receiver module. 40 is taken for the cheap VS1838 module her, since we have high intensity.
+
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+#include
+
+#if defined(APPLICATION_PIN)
+#define DEBUG_BUTTON_PIN APPLICATION_PIN // if low, print timing for each received data set
+#else
+#define DEBUG_BUTTON_PIN 6
+#endif
+
+bool detectLongPress(uint16_t aLongPressDurationMillis);
+
+void setup() {
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ pinMode(DEBUG_BUTTON_PIN, INPUT_PULLUP);
+#endif
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+// Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+// In case the interrupt driver crashes on setup, give a clue
+// to the user what's going on.
+ Serial.println(F("Enabling IRin..."));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+#if defined(IR_RECEIVE_PIN_STRING)
+ Serial.println(F("at pin " IR_RECEIVE_PIN_STRING));
+#else
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+#endif
+
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ Serial.println();
+ Serial.print(F("If you connect debug pin "));
+# if defined(APPLICATION_PIN_STRING)
+ Serial.print(APPLICATION_PIN_STRING);
+# else
+ Serial.print(DEBUG_BUTTON_PIN);
+# endif
+ Serial.println(F(" to ground, raw data is always printed"));
+
+ // infos for receive
+ Serial.print(RECORD_GAP_MICROS);
+ Serial.println(F(" us is the (minimum) gap, after which the start of a new IR packet is assumed"));
+ Serial.print(MARK_EXCESS_MICROS);
+ Serial.println(F(" us are subtracted from all marks and added to all spaces for decoding"));
+#endif
+
+}
+
+void loop() {
+ /*
+ * Check if received data is available and if yes, try to decode it.
+ * Decoded result is in the IrReceiver.decodedIRData structure.
+ *
+ * E.g. command is in IrReceiver.decodedIRData.command
+ * address is in command is in IrReceiver.decodedIRData.address
+ * and up to 32 bit raw data in IrReceiver.decodedIRData.decodedRawData
+ *
+ * At 115200 baud, printing takes 40 ms for NEC protocol and 10 ms for NEC repeat
+ */
+ if (IrReceiver.decode()) {
+ Serial.println();
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_WAS_OVERFLOW) {
+ Serial.println(F("Overflow detected"));
+ Serial.println(F("Try to increase the \"RAW_BUFFER_LENGTH\" value of " STR(RAW_BUFFER_LENGTH) " in " __FILE__));
+ // see also https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library
+# if !defined(ESP8266) && !defined(NRF5)
+ /*
+ * do double beep
+ */
+# if !defined(ESP32)
+ IrReceiver.stop(); // ESP32 uses another timer for tone()
+# endif
+ tone(TONE_PIN, 1100, 10);
+ delay(50);
+ tone(TONE_PIN, 1100, 10);
+ delay(50);
+# if !defined(ESP32)
+ IrReceiver.start(100000); // to compensate for 100 ms stop of receiver. This enables a correct gap measurement.
+# endif
+# endif
+
+ } else {
+ auto tStartMillis = millis();
+# if !defined(ESP32)
+ IrReceiver.stop(); // ESP32 uses another timer for tone()
+# endif
+ tone(TONE_PIN, 2200);
+
+ // No overflow, print a short summary of received data
+# if defined(LOCAL_DEBUG)
+ IrReceiver.printIRResultShort(&Serial, true);
+# else
+ IrReceiver.printIRResultShort(&Serial, true, digitalRead(DEBUG_BUTTON_PIN) == LOW);
+# endif
+ // Guarantee at least 5 millis for tone. decode starts 5 millis (RECORD_GAP_MICROS) after end of frame
+ // so here we are 10 millis after end of frame. Sony20 has only a 12 ms repeat gap.
+ while ((millis() - tStartMillis) < 5)
+ ;
+ noTone(TONE_PIN);
+
+# if !defined(ESP32)
+ // Restore IR timer. millis() - tStartMillis to compensate for stop of receiver. This enables a correct gap measurement.
+ IrReceiver.startWithTicksToAdd((millis() - tStartMillis) * (MICROS_IN_ONE_MILLI / MICROS_PER_TICK));
+# endif
+
+ IrReceiver.printIRSendUsage(&Serial);
+# if defined(LOCAL_DEBUG)
+ IrReceiver.printIRResultRawFormatted(&Serial, true);
+# else
+ if (IrReceiver.decodedIRData.protocol == UNKNOWN || digitalRead(DEBUG_BUTTON_PIN) == LOW) {
+ // We have an unknown protocol, print more info
+ if (IrReceiver.decodedIRData.protocol == UNKNOWN) {
+ Serial.println(F("Received noise or an unknown (or not yet enabled) protocol"));
+ }
+ IrReceiver.printIRResultRawFormatted(&Serial, true);
+ }
+# endif
+ }
+
+ // tone on esp8266 works once, then it disables the successful IrReceiver.start() / timerConfigForReceive().
+# if !defined(ESP8266) && !defined(NRF5) && !defined(LOCAL_DEBUG)
+ if ((IrReceiver.decodedIRData.protocol != SONY) && (IrReceiver.decodedIRData.protocol != PULSE_WIDTH)
+ && (IrReceiver.decodedIRData.protocol != PULSE_DISTANCE) && (IrReceiver.decodedIRData.protocol != UNKNOWN)
+ && digitalRead(DEBUG_BUTTON_PIN) != LOW) {
+ /*
+ * If no debug mode or a valid protocol was received, play tone, wait and restore IR timer.
+ * For SONY the tone prevents the detection of a repeat
+ * Otherwise do not play a tone to get exact gap time between transmissions and not running into repeat frames while wait for tone to end.
+ * This will give the next CheckForRecordGapsMicros() call a chance to eventually propose a change of the current RECORD_GAP_MICROS value.
+ */
+# if !defined(ESP32)
+ IrReceiver.stop(); // ESP32 uses another timer for tone()
+# endif
+ tone(TONE_PIN, 2200, 8);
+# if !defined(ESP32)
+ delay(8);
+ IrReceiver.start(8000); // Restore IR timer. 8000 to compensate for 8 ms stop of receiver. This enables a correct gap measurement.
+# endif
+ }
+# endif
+#else // #if FLASHEND >= 0x3FFF
+ // Print a minimal summary of received data
+ IrReceiver.printIRResultMinimal(&Serial);
+#endif // #if FLASHEND >= 0x3FFF
+
+ /*
+ * !!!Important!!! Enable receiving of the next value,
+ * since receiving has stopped after the end of the current received data packet.
+ */
+ IrReceiver.resume();
+
+ /*
+ * Finally check the received data and perform actions according to the received address and commands
+ */
+ if (IrReceiver.decodedIRData.address == 0) {
+ if (IrReceiver.decodedIRData.command == 0x10) {
+ // do something
+ } else if (IrReceiver.decodedIRData.command == 0x11) {
+ // do something else
+ }
+ }
+
+ // Check if repeats of the IR command was sent for more than 1000 ms
+ if (detectLongPress(1000)) {
+ Serial.print(F("Command 0x"));
+ Serial.print(IrReceiver.decodedIRData.command, HEX);
+ Serial.println(F(" was repeated for more than 2 seconds"));
+ }
+ } // if (IrReceiver.decode())
+
+ /*
+ * Your code here
+ * For all users of the FastLed library, use this code for strip.show() to improve receiving performance (which is still not 100%):
+ * if (IrReceiver.isIdle()) {
+ * strip.show();
+ * }
+ */
+
+}
+
+unsigned long sMillisOfFirstReceive;
+bool sLongPressJustDetected;
+/**
+ * True once we received the consecutive repeats for more than aLongPressDurationMillis milliseconds.
+ * The first frame, which is no repeat, is NOT counted for the duration!
+ * @return true once after the repeated IR command was received for longer than aLongPressDurationMillis milliseconds, false otherwise.
+ */
+bool detectLongPress(uint16_t aLongPressDurationMillis) {
+ if (!sLongPressJustDetected && (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_IS_REPEAT)) {
+ /*
+ * Here the repeat flag is set (which implies, that command is the same as the previous one)
+ */
+ if (millis() - aLongPressDurationMillis > sMillisOfFirstReceive) {
+ sLongPressJustDetected = true; // Long press here
+ }
+ } else {
+ // No repeat here
+ sMillisOfFirstReceive = millis();
+ sLongPressJustDetected = false;
+ }
+ return sLongPressJustDetected; // No long press here
+}
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.ino
new file mode 100644
index 00000000..4accf67e
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.ino
@@ -0,0 +1,152 @@
+/*
+ * ReceiveDump.cpp
+ *
+ * Dumps the received signal in different flavors.
+ * Since the printing takes so much time (200 ms @115200 for NEC protocol, 70ms for NEC repeat),
+ * repeat signals may be skipped or interpreted as UNKNOWN.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#if !defined(RAW_BUFFER_LENGTH)
+# if RAMEND <= 0x4FF || RAMSIZE < 0x4FF
+#define RAW_BUFFER_LENGTH 180 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# elif RAMEND <= 0x8FF || RAMSIZE < 0x8FF
+#define RAW_BUFFER_LENGTH 600 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# else
+#define RAW_BUFFER_LENGTH 750 // 750 (600 if we have only 2k RAM) is the value for air condition remotes. Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+# endif
+#endif
+
+/*
+ * MARK_EXCESS_MICROS is subtracted from all marks and added to all spaces before decoding,
+ * to compensate for the signal forming of different IR receiver modules. See also IRremote.hpp line 142.
+ *
+ * You can change this value accordingly to the receiver module you use.
+ * The required value can be derived from the timings printed here.
+ * Keep in mind that the timings may change with the distance
+ * between sender and receiver as well as with the ambient light intensity.
+ */
+#define MARK_EXCESS_MICROS 20 // Adapt it to your IR receiver module. 20 is recommended for the cheap VS1838 modules.
+
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+#include
+
+//+=============================================================================
+// Configure the Arduino
+//
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+
+ Serial.begin(115200); // Status message will be sent to PC at 9600 baud
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+
+ // infos for receive
+ Serial.print(RECORD_GAP_MICROS);
+ Serial.println(F(" us is the (minimum) gap, after which the start of a new IR packet is assumed"));
+ Serial.print(MARK_EXCESS_MICROS);
+ Serial.println();
+ Serial.println(F("Because of the verbose output (>200 ms at 115200 baud), repeats are not dumped correctly!"));
+ Serial.println();
+}
+
+//+=============================================================================
+// The repeating section of the code
+//
+void loop() {
+ if (IrReceiver.decode()) { // Grab an IR code
+ // At 115200 baud, printing takes 200 ms for NEC protocol and 70 ms for NEC repeat
+ Serial.println(); // blank line between entries
+ Serial.println(); // 2 blank lines between entries
+ IrReceiver.printIRResultShort(&Serial);
+ // Check if the buffer overflowed
+ if (IrReceiver.decodedIRData.flags & IRDATA_FLAGS_WAS_OVERFLOW) {
+ Serial.println(F("Try to increase the \"RAW_BUFFER_LENGTH\" value of " STR(RAW_BUFFER_LENGTH) " in " __FILE__));
+ // see also https://github.com/Arduino-IRremote/Arduino-IRremote#compile-options--macros-for-this-library
+ } else {
+ if (IrReceiver.decodedIRData.protocol == UNKNOWN) {
+ Serial.println(F("Received noise or an unknown (or not yet enabled) protocol"));
+ }
+ Serial.println();
+ IrReceiver.printIRSendUsage(&Serial);
+ Serial.println();
+ Serial.println(F("Raw result in internal ticks (50 us) - with leading gap"));
+ IrReceiver.printIRResultRawFormatted(&Serial, false); // Output the results in RAW format
+ Serial.println(F("Raw result in microseconds - with leading gap"));
+ IrReceiver.printIRResultRawFormatted(&Serial, true); // Output the results in RAW format
+ Serial.println(); // blank line between entries
+ Serial.print(F("Result as internal 8bit ticks (50 us) array - compensated with MARK_EXCESS_MICROS="));
+ Serial.println(MARK_EXCESS_MICROS);
+ IrReceiver.compensateAndPrintIRResultAsCArray(&Serial, false); // Output the results as uint8_t source code array of ticks
+ Serial.print(F("Result as microseconds array - compensated with MARK_EXCESS_MICROS="));
+ Serial.println(MARK_EXCESS_MICROS);
+ IrReceiver.compensateAndPrintIRResultAsCArray(&Serial, true); // Output the results as uint16_t source code array of micros
+ IrReceiver.printIRResultAsCVariables(&Serial); // Output address and data as source code variables
+ Serial.println(); // blank line between entries
+ IrReceiver.printIRSendUsage(&Serial);
+ Serial.println(); // blank line between entries
+
+ IrReceiver.compensateAndPrintIRResultAsPronto(&Serial);
+
+ /*
+ * Example for using the compensateAndStorePronto() function.
+ * Creating this String requires 2210 bytes program memory and 10 bytes RAM for the String class.
+ * The String object itself requires additional 440 bytes RAM from the heap.
+ * This values are for an Arduino Uno.
+ */
+// Serial.println(); // blank line between entries
+// String ProntoHEX = F("Pronto HEX contains: "); // Assign string to ProtoHex string object
+// if (int size = IrReceiver.compensateAndStorePronto(&ProntoHEX)) { // Dump the content of the IReceiver Pronto HEX to the String object
+// // Append compensateAndStorePronto() size information to the String object (requires 50 bytes heap)
+// ProntoHEX += F("\r\nProntoHEX is "); // Add codes size information to the String object
+// ProntoHEX += size;
+// ProntoHEX += F(" characters long and contains "); // Add codes count information to the String object
+// ProntoHEX += size / 5;
+// ProntoHEX += F(" codes");
+// Serial.println(ProntoHEX.c_str()); // Print to the serial console the whole String object
+// Serial.println(); // blank line between entries
+// }
+ }
+ IrReceiver.resume(); // Prepare for the next value
+ }
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.log b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.log
new file mode 100644
index 00000000..ae16f33e
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveDump/ReceiveDump.log
@@ -0,0 +1,52 @@
+START ../src/ReceiveDump.cpp from Nov 12 2022
+Using library version 4.0.0
+Ready to receive IR signals of protocols: NEC/NEC2/Onkyo/Apple, Panasonic/Kaseikyo, Denon/Sharp, Sony, RC5, RC6, LG, JVC, Samsung, Whynter, Lego Power Functions, Bosewave , MagiQuest, Universal Pulse Distance Width, Hash at pin 2
+5000 us is the (minimum) gap, after which the start of a new IR packet is assumed
+20 us are subtracted from all marks and added to all spaces for decoding
+
+
+Protocol=Samsung Address=0x707 Command=0x4 Raw-Data=0xFB040707 32 bits LSB first
+
+Send with: IrSender.sendSamsung(0x707, 0x4, );
+
+Raw result in internal ticks (50 us) - with leading gap
+rawData[68]:
+ -27948
+ +90,-84
+ +12,-32 +12,-32 +12,-32 +12,-11
+ +11,-11 +11,-11 +11,-11 +11,-11
+ +12,-32 +12,-32 +12,-32 +12,-10
+ +12,-10 +12,-10 +12,-10 +12,-11
+ +11,-11 +11,-11 +11,-33 +11,-11
+ +11,-11 +11,-11 +11,-11 +11,-11
+ +12,-32 +12,-32 +12,-10 +12,-32
+ +12,-32 +12,-32 +12,-32 +12,-32
+ +12
+Sum: 1200
+Raw result in microseconds - with leading gap
+rawData[68]:
+ -1397400
+ +4500,-4200
+ + 600,-1600 + 600,-1600 + 600,-1600 + 600,- 550
+ + 550,- 550 + 550,- 550 + 550,- 550 + 550,- 550
+ + 600,-1600 + 600,-1600 + 600,-1600 + 600,- 500
+ + 600,- 500 + 600,- 500 + 600,- 500 + 600,- 550
+ + 550,- 550 + 550,- 550 + 550,-1650 + 550,- 550
+ + 550,- 550 + 550,- 550 + 550,- 550 + 550,- 550
+ + 600,-1600 + 600,-1600 + 600,- 500 + 600,-1600
+ + 600,-1600 + 600,-1600 + 600,-1600 + 600,-1600
+ + 600
+Sum: 60000
+
+Result as internal ticks (50 us) array - compensated with MARK_EXCESS_MICROS=20
+uint8_t rawTicks[67] = {90,84, 12,32, 12,32, 12,32, 12,11, 11,11, 11,11, 11,11, 11,11, 12,32, 12,32, 12,32, 12,10, 12,10, 12,10, 12,10, 12,11, 11,11, 11,11, 11,33, 11,11, 11,11, 11,11, 11,11, 11,11, 12,32, 12,32, 12,10, 12,32, 12,32, 12,32, 12,32, 12,32, 12}; // Protocol=Samsung Address=0x707 Command=0x4 Raw-Data=0xFB040707 32 bits LSB first
+
+Result as microseconds array - compensated with MARK_EXCESS_MICROS=20
+uint16_t rawData[67] = {4480,4220, 580,1620, 580,1620, 580,1620, 580,570, 530,570, 530,570, 530,570, 530,570, 580,1620, 580,1620, 580,1620, 580,520, 580,520, 580,520, 580,520, 580,570, 530,570, 530,570, 530,1670, 530,570, 530,570, 530,570, 530,570, 530,570, 580,1620, 580,1620, 580,520, 580,1620, 580,1620, 580,1620, 580,1620, 580,1620, 580}; // Protocol=Samsung Address=0x707 Command=0x4 Raw-Data=0xFB040707 32 bits LSB first
+
+uint16_t address = 0x707;
+uint16_t command = 0x4;
+uint32_t data = 0xFB040707;
+
+Pronto Hex as string
+char prontoData[] = "0000 006D 0022 0000 00AE 00A1 0018 003D 0018 003D 0018 003D 0018 0014 0016 0014 0016 0014 0016 0014 0016 0014 0018 003D 0018 003D 0018 003D 0018 0012 0018 0012 0018 0012 0018 0012 0018 0014 0016 0014 0016 0014 0016 003F 0016 0014 0016 0014 0016 0014 0016 0014 0016 0014 0018 003D 0018 003D 0018 0012 0018 003D 0018 003D 0018 003D 0018 003D 0018 003D 0018 06C3 ";
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/ReceiveOneAndSendMultiple.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/ReceiveOneAndSendMultiple.ino
new file mode 100644
index 00000000..34baa144
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiveOneAndSendMultiple/ReceiveOneAndSendMultiple.ino
@@ -0,0 +1,257 @@
+/*
+ * ReceiveOneAndSendMultiple.cpp
+ *
+ * Serves as a IR remote macro expander
+ * Receives Samsung32 protocol and on receiving a specified input frame,
+ * it sends multiple Samsung32 frames with appropriate delays in between.
+ * This serves as a Netflix-key emulation for my old Samsung H5273 TV.
+ *
+ * Tested on a digispark ATTiny85 board using AttinyCore https://github.com/SpenceKonde/ATTinyCore
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+// Digispark ATMEL ATTINY85
+// Piezo speaker must have a 270 ohm resistor in series for USB programming and running at the Samsung TV.
+// IR LED has a 270 ohm resistor in series.
+// +-\/-+
+// !RESET (5) PB5 1| |8 Vcc
+// USB+ 3.6V Z-Diode, 1.5kOhm to VCC Piezo (3) PB3 2| |7 PB2 (2) TX Debug output
+// USB- 3.6V Z-Diode IR Output (4) PB4 3| |6 PB1 (1) Feedback LED
+// GND 4| |5 PB0 (0) IR Input
+// +----+
+/* SAUMSUMG REMOTE CODES (Model: BN59-01180A)
+ * Power Button - 0x2
+ * Power Off - 0x98
+ * 1 - 0x4
+ * 2 - 0x5
+ * 3 - 0x6
+ * 4 - 0x8
+ * 5 - 0x9
+ * 6 - 0xa
+ * 7 - 0xc
+ * 8 - 0xd
+ * 9 - 0xe
+ * CH List - 0x6b
+ * Vol + - 0x7
+ * Vol - - 0xb
+ * Mute - 0xf
+ * Source - 0x1
+ * Ch + - 0x12
+ * Ch - - 0x10
+ * Menu - 0x1a
+ * Home - 0x79
+ * MagicInfo Player - 0x30
+ * Tools - 0x4b
+ * Info - 0x1f
+ * Up arrow - 0x60
+ * Left arrow - 0x65
+ * Right arrow - 0x62
+ * Down arrow - 0x61
+ * Return - 0x58
+ * Exit - 0x2d
+ * A - 0x6c
+ * B - 0x14
+ * C - 0x15
+ * D - 0x16
+ * Set - 0xab
+ * Unset - 0xac
+ * Lock - 0x77
+ * Stop (square) - 0x46
+ * Rewind (arrows) - 0x45
+ * Play (triangle) - 0x47
+ * Pause (bars) - 0x4a
+ * Fast Forward (arrows) - 0x48
+ */
+
+#include
+
+// select only Samsung protocol for sending and receiving
+#define DECODE_SAMSUNG
+#define ADDRESS_OF_SAMSUNG_REMOTE 0x0707 // The value you see as address in printIRResultShort()
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+void sendSamsungSmartHubMacro(bool aDoSelect);
+void IRSendWithDelay(uint8_t aCommand, uint16_t aDelayMillis);
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // tone before IR setup, since it kills the IR timer settings
+ tone(TONE_PIN, 2200, 400);
+ digitalWrite(LED_BUILTIN, HIGH);
+ delay(400);
+ digitalWrite(LED_BUILTIN, LOW);
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+ Serial.println(F("Ready to send IR signals at pin " STR(IR_SEND_PIN)));
+}
+
+void loop() {
+ /*
+ * Check if new data available and get them
+ */
+ if (IrReceiver.decode()) {
+ // Print a short summary of received data
+ IrReceiver.printIRResultShort(&Serial);
+ IrReceiver.printIRSendUsage(&Serial);
+ Serial.println();
+
+ /*
+ * Here data is available -> evaluate IR command
+ */
+ switch (IrReceiver.decodedIRData.command) {
+ case 0x47: // The play key on the bottom of my Samsung remote
+ Serial.println(F("Play key detected, open Netflix"));
+ sendSamsungSmartHubMacro(true);
+ break;
+
+ case 0x4A: // The pause key on the bottom of my Samsung remote
+ Serial.println(F("Pause key detected, open SmartHub"));
+ sendSamsungSmartHubMacro(false);
+ break;
+
+ default:
+ break;
+ }
+
+ /*
+ * !!!Important!!! Enable receiving of the next value,
+ * since receiving has stopped after the end of the current received data packet.
+ */
+ IrReceiver.restartAfterSend(); // Is a NOP if sending does not require a timer.
+ IrReceiver.resume(); // Enable receiving of the next value
+ }
+}
+
+void IRSendWithDelay(uint8_t aCommand, uint16_t aDelayMillis) {
+ IrSender.sendSamsung(ADDRESS_OF_SAMSUNG_REMOTE, aCommand, 1); // send with one repeat
+ Serial.print(F("Send Samsung command 0x"));
+ Serial.println(aCommand);
+ delay(aDelayMillis);
+}
+
+bool sMacroWasCalledBefore = false;
+#define INITIAL_WAIT_TIME_APPS_READY_MILLIS 70000 // Time to let the TV load all software before Netflix can be started without an error
+#define INITIAL_WAIT_TIME_SMARTHUB_READY_MILLIS 20000 // Time to let the TV load all software before SmartHub manu can be displayed
+
+/*
+ * This macro calls the last SmartHub application you selected manually
+ *
+ * @param aDoSelect - if true select the current app (needs longer initial wait time) else show smarthub menu
+ *
+ */
+void sendSamsungSmartHubMacro(bool aDoSelect) {
+ uint32_t tWaitTimeAfterBoot;
+ if (aDoSelect) {
+ tWaitTimeAfterBoot = INITIAL_WAIT_TIME_APPS_READY_MILLIS;
+ } else {
+ tWaitTimeAfterBoot = INITIAL_WAIT_TIME_SMARTHUB_READY_MILLIS;
+ }
+
+# if !defined(ESP32)
+ IrReceiver.stop(); // ESP32 uses another timer for tone()
+# endif
+ if (millis() < tWaitTimeAfterBoot) {
+ // division by 1000 and printing requires much (8%) program memory
+ Serial.print(F("It is "));
+ Serial.print(millis() / 1000);
+ Serial.print(F(" seconds after boot, Samsung H5273 TV requires "));
+ Serial.print(tWaitTimeAfterBoot / 1000);
+ Serial.println(F(" seconds after boot to be ready for the command"));
+
+ tone(TONE_PIN, 2200, 100);
+ delay(200);
+ tone(TONE_PIN, 2200, 100);
+ delay(100);
+
+ if (millis() < tWaitTimeAfterBoot) {
+ Serial.print(F("Now do a blocking wait for "));
+ Serial.print(tWaitTimeAfterBoot - millis());
+ Serial.println(F(" milliseconds"));
+ delay(tWaitTimeAfterBoot - millis());
+ }
+ }
+
+ // Do beep feedback for special key to be received
+ tone(TONE_PIN, 2200, 200);
+ delay(200);
+
+# if !defined(ESP32)
+ IrReceiver.start(200000); // to compensate for 200 ms stop of receiver. This enables a correct gap measurement.
+# endif
+
+ Serial.println(F("Wait for \"not supported\" to disappear"));
+ delay(2000);
+
+ Serial.println(F("Start sending of Samsung IR macro"));
+
+ IRSendWithDelay(0x1A, 2000); // Menu and wait for the Menu to pop up
+
+ Serial.println(F("Wait for the menu to pop up"));
+ if (!sMacroWasCalledBefore) {
+ delay(2000); // wait additional time for the Menu load
+ }
+
+ for (uint_fast8_t i = 0; i < 4; ++i) {
+ IRSendWithDelay(0x61, 250); // Down arrow. For my Samsung, the high byte of the command is the inverse of the low byte
+ }
+
+ IRSendWithDelay(0x62, 400); // Right arrow
+ for (uint_fast8_t i = 0; i < 2; ++i) {
+ IRSendWithDelay(0x61, 250); // Down arrow
+ }
+
+ delay(250);
+ IRSendWithDelay(0x68, 1); // Enter for SmartHub
+
+ if (aDoSelect) {
+ Serial.println(F("Wait for SmartHub to show up, before entering current application"));
+ delay(10000); // Wait not longer than 12 seconds, because smarthub menu then disappears
+ IRSendWithDelay(0x68, 1); // Enter for last application (e.g. Netflix or Amazon)
+ }
+
+ sMacroWasCalledBefore = true;
+ Serial.println(F("Done"));
+
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/ReceiverTimingAnalysis/ReceiverTimingAnalysis.ino b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiverTimingAnalysis/ReceiverTimingAnalysis.ino
new file mode 100644
index 00000000..410b3fde
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/ReceiverTimingAnalysis/ReceiverTimingAnalysis.ino
@@ -0,0 +1,260 @@
+/*
+ * ReceiverTimingAnalysis.cpp
+ *
+ * This program enables the pin change interrupt at pin 3 and waits for NEC (or other Pulse-Distance-Coding) IR Signal.
+ * It measures the pulse and pause times of the incoming signal and computes some statistics for it.
+ *
+ * Observed values:
+ * Delta of each signal type is around 50 up to 100 and at low signals up to 200. TSOP is better, especially at low IR signal level.
+ * VS1838 Mark Excess -50 to +50 us
+ * TSOP31238 Mark Excess 0 to +50
+ *
+ *
+ * Copyright (C) 2019-2020 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRMP https://github.com/IRMP-org/IRMP.
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * IRMP is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+#include
+
+#define IR_RECEIVE_PIN 2
+//#define IR_RECEIVE_PIN 3
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+
+void measureTimingISR(void);
+
+void setup()
+{
+ pinMode(LED_BUILTIN, OUTPUT);
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__));
+
+#if defined(EICRA) && defined(EIFR) && defined(EIMSK)
+# if (IR_RECEIVE_PIN == 2)
+ EICRA |= _BV(ISC00); // interrupt on any logical change
+ EIFR |= _BV(INTF0); // clear interrupt bit
+ EIMSK |= _BV(INT0); // enable interrupt on next change
+# elif (IR_RECEIVE_PIN == 3)
+ EICRA |= _BV(ISC10); // enable interrupt on pin3 on both edges for ATmega328
+ EIFR |= _BV(INTF1); // clear interrupt bit
+ EIMSK |= _BV(INT1); // enable interrupt on next change
+# endif
+#else
+ attachInterrupt(digitalPinToInterrupt(IR_RECEIVE_PIN), measureTimingISR, CHANGE);
+#endif
+ Serial.println(F("Ready to analyze NEC IR signal at pin " STR(IR_RECEIVE_PIN)));
+ Serial.println();
+}
+
+uint8_t ISREdgeCounter = 0;
+volatile uint32_t LastMicros;
+struct timingStruct
+{
+ uint16_t minimum;
+ uint8_t indexOfMinimum;
+ uint16_t maximum;
+ uint8_t indexOfMaximum;
+ uint16_t average;
+
+ uint16_t SumForAverage;
+ uint8_t SampleCount;
+// uint8_t LastPrintedCount;
+};
+
+struct timingStruct Mark;
+struct timingStruct ShortSpace;
+struct timingStruct LongSpace;
+
+/*
+ * Compute minimum, maximum and average
+ */
+void processTmingValue(struct timingStruct *aTimingStruct, uint16_t aValue)
+{
+ if (aTimingStruct->SampleCount == 0)
+ {
+ // initialize values
+ aTimingStruct->minimum = UINT16_MAX;
+ aTimingStruct->maximum = 0;
+ aTimingStruct->SumForAverage = 0;
+ }
+
+ if (aTimingStruct->minimum > aValue)
+ {
+ aTimingStruct->minimum = aValue;
+ aTimingStruct->indexOfMinimum = aTimingStruct->SampleCount;
+ }
+ if (aTimingStruct->maximum < aValue)
+ {
+ aTimingStruct->maximum = aValue;
+ aTimingStruct->indexOfMaximum = aTimingStruct->SampleCount;
+ }
+
+ aTimingStruct->SampleCount++;
+ aTimingStruct->SumForAverage += aValue;
+ aTimingStruct->average = (aTimingStruct->SumForAverage + (aTimingStruct->SampleCount / 2)) / aTimingStruct->SampleCount;
+
+}
+
+void printTimingValues(struct timingStruct *aTimingStruct, const char *aCaption)
+{
+// if (aTimingStruct->LastPrintedCount != aTimingStruct->SampleCount)
+// {
+// aTimingStruct->LastPrintedCount = aTimingStruct->SampleCount;
+ Serial.print(aCaption);
+ Serial.print(F(": SampleCount="));
+ Serial.print(aTimingStruct->SampleCount);
+ Serial.print(F(" Minimum="));
+ Serial.print(aTimingStruct->minimum);
+ Serial.print(F(" @"));
+ Serial.print(aTimingStruct->indexOfMinimum);
+ Serial.print(F(" Maximum="));
+ Serial.print(aTimingStruct->maximum);
+ Serial.print(F(" @"));
+ Serial.print(aTimingStruct->indexOfMaximum);
+ Serial.print(F(" Delta="));
+ Serial.print(aTimingStruct->maximum - aTimingStruct->minimum);
+ Serial.print(F(" Average="));
+ Serial.print(aTimingStruct->average);
+
+ Serial.println();
+// }
+}
+
+void loop()
+{
+ if (Mark.SampleCount >= 32)
+ {
+ /*
+ * This check enables statistics for longer protocols like Kaseikyo/Panasonics
+ */
+#if !defined(ARDUINO_ARCH_MBED)
+ noInterrupts();
+#endif
+ uint32_t tLastMicros = LastMicros;
+#if !defined(ARDUINO_ARCH_MBED)
+ interrupts();
+#endif
+ uint32_t tMicrosDelta = micros() - tLastMicros;
+
+ if (tMicrosDelta > 10000)
+ {
+ // NEC signal ended just now
+ Serial.println();
+ printTimingValues(&Mark, "Mark ");
+ printTimingValues(&ShortSpace, "ShortSpace");
+ printTimingValues(&LongSpace, "LongSpace ");
+
+ /*
+ * Print analysis of mark and short spaces
+ */
+ Serial.println(F("Analysis :"));
+ Serial.print(F(" (Average of mark + short space)/2 = "));
+ int16_t MarkAndShortSpaceAverage = (Mark.average + ShortSpace.average) / 2;
+ Serial.print(MarkAndShortSpaceAverage);
+ Serial.print(F(" us\r\n Delta (to NEC standard 560) = "));
+ Serial.print(MarkAndShortSpaceAverage - 560);
+ Serial.print(F("us\r\n MARK_EXCESS_MICROS = (Average of mark - Average of mark and short space) = "));
+ Serial.print((int16_t) Mark.average - MarkAndShortSpaceAverage);
+ Serial.print(F("us"));
+ Serial.println();
+ Serial.println();
+
+ Mark.SampleCount = 0; // used as flag for not printing the results more than once
+ }
+ }
+}
+
+/*
+ * The interrupt handler.
+ * Just add to the appropriate timing structure.
+ */
+#if defined(ESP8266) || defined(ESP32)
+void IRAM_ATTR measureTimingISR()
+#else
+# if defined(EICRA) && defined(EIFR) && defined(EIMSK)
+# if (IR_RECEIVE_PIN == 2)
+ISR(INT0_vect)
+# elif (IR_RECEIVE_PIN == 3)
+ISR(INT1_vect)
+# endif
+# else
+void measureTimingISR()
+# endif
+#endif
+{
+ uint32_t tMicros = micros();
+ uint32_t tMicrosDelta = tMicros - LastMicros;
+ LastMicros = tMicros;
+ /*
+ * read level and give feedback
+ */
+ uint8_t tInputLevel = digitalRead(IR_RECEIVE_PIN);
+ digitalWrite(LED_BUILTIN, !tInputLevel);
+
+ if (tMicrosDelta > 10000)
+ {
+ // gap > 10 ms detected, reset counter to first detected edge and initialize timing structures
+ ISREdgeCounter = 1;
+ LongSpace.SampleCount = 0;
+ ShortSpace.SampleCount = 0;
+ Mark.SampleCount = 0;
+ }
+ else
+ {
+ ISREdgeCounter++;
+ }
+
+ /*
+ * Skip header mark and space and first bit mark and space
+ */
+ if (ISREdgeCounter > 4)
+ {
+ if (tInputLevel != LOW)
+ {
+ // Mark ended
+ processTmingValue(&Mark, tMicrosDelta);
+// Serial.print('M');
+ }
+ else
+ {
+ // Space ended
+ if (tMicrosDelta > 1000)
+ {
+ // long space - logical 1
+ processTmingValue(&LongSpace, tMicrosDelta);
+ Serial.print('1');
+ }
+ else
+ {
+ // short space - logical 0
+ processTmingValue(&ShortSpace, tMicrosDelta);
+ Serial.print('0');
+ }
+ }
+ }
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.ino
new file mode 100644
index 00000000..7303c1af
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.ino
@@ -0,0 +1,161 @@
+/*
+ * SendAndReceive.cpp
+ *
+ * Demonstrates sending IR codes and receiving it simultaneously
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2021-2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+#include
+
+// select only NEC and the universal decoder for pulse distance protocols
+#define DECODE_NEC // Includes Apple and Onkyo
+#define DECODE_DISTANCE_WIDTH // In case NEC is not received correctly. Universal decoder for pulse distance width protocols
+
+//#define EXCLUDE_UNIVERSAL_PROTOCOLS // Saves up to 1000 bytes program memory.
+//#define EXCLUDE_EXOTIC_PROTOCOLS // saves around 650 bytes program memory if all other protocols are active
+//#define NO_LED_FEEDBACK_CODE // saves 92 bytes program memory
+//#define RECORD_GAP_MICROS 12000 // Default is 5000. Activate it for some LG air conditioner protocols
+//#define SEND_PWM_BY_TIMER // Disable carrier PWM generation in software and use (restricted) hardware PWM.
+//#define USE_NO_SEND_PWM // Use no carrier PWM, just simulate an active low receiver signal. Overrides SEND_PWM_BY_TIMER definition
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+#define DELAY_AFTER_SEND 2000
+#define DELAY_AFTER_LOOP 5000
+
+void setup() {
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+ // Start the receiver and if not 3. parameter specified, take LED_BUILTIN pin from the internal boards definition as default feedback LED
+ IrReceiver.begin(IR_RECEIVE_PIN, ENABLE_LED_FEEDBACK);
+
+ Serial.print(F("Ready to receive IR signals of protocols: "));
+ printActiveIRProtocols(&Serial);
+ Serial.println(F("at pin " STR(IR_RECEIVE_PIN)));
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+
+#if FLASHEND >= 0x3FFF // For 16k flash or more, like ATtiny1604
+// For esp32 we use PWM generation by ledcWrite() for each pin.
+# if !defined(SEND_PWM_BY_TIMER) && !defined(USE_NO_SEND_PWM) && !defined(ESP32)
+ /*
+ * Print internal software PWM generation info
+ */
+ IrSender.enableIROut(38); // Call it with 38 kHz to initialize the values printed below
+ Serial.print(F("Send signal mark duration is "));
+ Serial.print(IrSender.periodOnTimeMicros);
+ Serial.print(F(" us, pulse correction is "));
+ Serial.print(IrSender.getPulseCorrectionNanos());
+ Serial.print(F(" ns, total period is "));
+ Serial.print(IrSender.periodTimeMicros);
+ Serial.println(F(" us"));
+# endif
+
+ // infos for receive
+ Serial.print(RECORD_GAP_MICROS);
+ Serial.println(F(" us is the (minimum) gap, after which the start of a new IR packet is assumed"));
+ Serial.print(MARK_EXCESS_MICROS);
+ Serial.println(F(" us are subtracted from all marks and added to all spaces for decoding"));
+#endif
+}
+
+uint16_t sAddress = 0x0102;
+uint8_t sCommand = 0x34;
+uint8_t sRepeats = 1;
+
+/*
+ * Send NEC IR protocol
+ */
+void send_ir_data() {
+ Serial.print(F("Sending: 0x"));
+ Serial.print(sAddress, HEX);
+ Serial.print(sCommand, HEX);
+ Serial.println(sRepeats, HEX);
+ Serial.flush(); // To avoid disturbing the software PWM generation by serial output interrupts
+
+ // clip repeats at 4
+ if (sRepeats > 4) {
+ sRepeats = 4;
+ }
+ // Results for the first loop to: Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 (32 bits)
+ IrSender.sendNEC(sAddress, sCommand, sRepeats);
+}
+
+void receive_ir_data() {
+ if (IrReceiver.decode()) {
+ Serial.print(F("Decoded protocol: "));
+ Serial.print(getProtocolString(IrReceiver.decodedIRData.protocol));
+ Serial.print(F(", decoded raw data: "));
+#if (__INT_WIDTH__ < 32)
+ Serial.print(IrReceiver.decodedIRData.decodedRawData, HEX);
+#else
+ PrintULL::print(&Serial, IrReceiver.decodedIRData.decodedRawData, HEX);
+#endif
+ Serial.print(F(", decoded address: "));
+ Serial.print(IrReceiver.decodedIRData.address, HEX);
+ Serial.print(F(", decoded command: "));
+ Serial.println(IrReceiver.decodedIRData.command, HEX);
+ IrReceiver.resume();
+ }
+}
+
+void loop() {
+ /*
+ * Print loop values
+ */
+ Serial.println();
+ Serial.print(F("address=0x"));
+ Serial.print(sAddress, HEX);
+ Serial.print(F(" command=0x"));
+ Serial.print(sCommand, HEX);
+ Serial.print(F(" repeats="));
+ Serial.println(sRepeats);
+ Serial.flush();
+
+ send_ir_data();
+ IrReceiver.restartAfterSend(); // Is a NOP if sending does not require a timer.
+
+ // wait for the receiver state machine to detect the end of a protocol
+ delay((RECORD_GAP_MICROS / 1000) + 5);
+ receive_ir_data();
+
+ // Prepare data for next loop
+ sAddress += 0x0101;
+ sCommand += 0x11;
+ sRepeats++;
+
+ delay(100); // Loop delay
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.log b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.log
new file mode 100644
index 00000000..ec6dc73b
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendAndReceive/SendAndReceive.log
@@ -0,0 +1,39 @@
+START ../src/SendAndReceive.cpp from Feb 24 2023
+Using library version 4.1.0
+Ready to receive IR signals of protocols: NEC/NEC2/Onkyo/Apple, Universal Pulse Distance Width, at pin 2
+Send IR signals at pin 3
+Send signal mark duration is 8 us, pulse correction is 3000 ns, total period is 26 us
+5000 us is the (minimum) gap, after which the start of a new IR packet is assumed
+20 us are subtracted from all marks and added to all spaces for decoding
+
+address=0x102 command=0x34 repeats=1
+Sending: 0x102341
+Decoded protocol: NEC2, decoded raw data: CB340102, decoded address: 102, decoded command: 34
+
+address=0x203 command=0x45 repeats=2
+Sending: 0x203452
+Decoded protocol: NEC, decoded raw data: BA450203, decoded address: 203, decoded command: 45
+
+address=0x304 command=0x56 repeats=3
+Sending: 0x304563
+Decoded protocol: NEC, decoded raw data: A9560304, decoded address: 304, decoded command: 56
+
+address=0x405 command=0x67 repeats=4
+Sending: 0x405674
+Decoded protocol: NEC, decoded raw data: 98670405, decoded address: 405, decoded command: 67
+
+address=0x506 command=0x78 repeats=5
+Sending: 0x506785
+Decoded protocol: NEC, decoded raw data: 87780506, decoded address: 506, decoded command: 78
+
+address=0x607 command=0x89 repeats=5
+Sending: 0x607895
+Decoded protocol: NEC, decoded raw data: 76890607, decoded address: 607, decoded command: 89
+
+address=0x708 command=0x9A repeats=5
+Sending: 0x7089A5
+Decoded protocol: NEC, decoded raw data: 659A0708, decoded address: 708, decoded command: 9A
+
+address=0x809 command=0xAB repeats=5
+Sending: 0x809AB5
+Decoded protocol: NEC, decoded raw data: 54AB0809, decoded address: 809, decoded command: AB
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/SendBoseWaveDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/SendBoseWaveDemo.ino
new file mode 100644
index 00000000..60197cc2
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendBoseWaveDemo/SendBoseWaveDemo.ino
@@ -0,0 +1,202 @@
+/*
+ * SendBoseWaveDemo.cpp
+ *
+ * Prompt user for a code to send. Make sure your 940-950nm IR LED is
+ * connected to the default digital output. Place your Bose Wave Radio
+ * CD in the line of sight of your LED, and send commands!
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020 Thomas Koch - 2022 AJ converted to inverted bits
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#define DISABLE_CODE_FOR_RECEIVER // Disables restarting receiver after each send. Saves 450 bytes program memory and 269 bytes RAM if receiving functions are not used.
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+//......................................................................
+//
+// Bose Wave Radio CD Remote Control
+// |-------------------------------------|
+// | On/Off Sleep VolUp |
+// | Play/Pause Stop VolDown |
+// | FM AM Aux |
+// | Tune Down Tune Up Mute |
+// | 1 2 3 |
+// | 4 5 6 |
+// |-------------------------------------|
+#define BOSE_CMD_ON_OFF 0x00
+#define BOSE_CMD_MUTE 0x01
+#define BOSE_CMD_VOL_UP 0x02
+#define BOSE_CMD_VOL_DOWN 0x03
+#define BOSE_CMD_PRESET_6 0x04
+#define BOSE_CMD_SLEEP 0x05
+#define BOSE_CMD_FM 0x06
+#define BOSE_CMD_AUX 0x07
+#define BOSE_CMD_AM 0x08
+#define BOSE_CMD_PLAY_PAUSE 0x09
+#define BOSE_CMD_STOP 0x0A
+#define BOSE_CMD_TUNE_UP 0x0B
+#define BOSE_CMD_TUNE_DOWN 0x0C
+#define BOSE_CMD_PRESET_1 0x0D
+#define BOSE_CMD_PRESET_2 0x0E
+#define BOSE_CMD_PRESET_3 0x0F
+#define BOSE_CMD_PRESET_4 0x10
+#define BOSE_CMD_PRESET_5 0x11
+
+// Codes for Wave Music System
+// https://github.com/Arduino-IRremote/Arduino-IRremote/blob/master/pictures/BoseWaveMusicSystem.jpg)
+//#define BOSE_CMD_ON_OFF 0x4C
+//#define BOSE_CMD_MUTE 0x01
+//#define BOSE_CMD_VOL_UP 0x03
+//#define BOSE_CMD_VOL_DOWN 0x02
+//#define BOSE_CMD_SLEEP 0x54
+//#define BOSE_CMD_FM_AM 0x06
+//#define BOSE_CMD_CD 0x53
+//#define BOSE_CMD_AUX 0x0F
+//#define BOSE_CMD_TRACK_BW 0x18
+//#define BOSE_CMD_TRACK_FW 0x19
+//#define BOSE_CMD_PLAY_PAUSE 0x1B
+//#define BOSE_CMD_STOP_EJECT 0x1A
+//#define BOSE_CMD_TUNE_UP 0x58
+//#define BOSE_CMD_TUNE_DOWN 0x57
+//#define BOSE_CMD_PRESET_1 0x07
+//#define BOSE_CMD_PRESET_2 0x08
+//#define BOSE_CMD_PRESET_3 0x09
+//#define BOSE_CMD_PRESET_4 0x0A
+//#define BOSE_CMD_PRESET_5 0x0B
+//#define BOSE_CMD_PRESET_6 0x0C
+//#define BOSE_CMD_TIME_MINUS 0x9E
+//#define BOSE_CMD_TIME_PLUS 0x24
+//#define BOSE_CMD_PLAY_MODE 0x21
+//#define BOSE_CMD_ALARM_ON_OFF 0x22
+//#define BOSE_CMD_ALARM_WAKE_TO 0x70
+//#define BOSE_CMD_ALARM_TIME 0x23
+
+bool sPrintMenu;
+void printMenu();
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+#if defined(IR_SEND_PIN)
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+#else
+ uint8_t tSendPin = 3;
+ IrSender.begin(tSendPin, ENABLE_LED_FEEDBACK, USE_DEFAULT_FEEDBACK_LED_PIN); // Specify send pin and enable feedback LED at default feedback LED pin
+ // You can change send pin later with IrSender.setSendPin();
+
+ Serial.print(F("Send IR signals at pin "));
+ Serial.println(tSendPin);
+#endif
+
+ sPrintMenu = true;
+}
+
+void loop() {
+ if (sPrintMenu) {
+ sPrintMenu = false;
+ printMenu();
+ }
+ int tSerialCommandCharacter;
+
+ if (Serial.available()) {
+ tSerialCommandCharacter = Serial.read();
+ sPrintMenu = true;
+ if (tSerialCommandCharacter == -1) {
+ Serial.print(F("available() was true, but no character read")); // should not happen
+ } else if (tSerialCommandCharacter == 48) { // 0
+ IrSender.sendBoseWave(BOSE_CMD_ON_OFF); // On/Off
+ } else if (tSerialCommandCharacter == 49) { // 1
+ IrSender.sendBoseWave(BOSE_CMD_VOL_UP); // Volume Up
+ } else if (tSerialCommandCharacter == 50) { // 2
+ IrSender.sendBoseWave(BOSE_CMD_VOL_DOWN); // Volume Down
+ } else if (tSerialCommandCharacter == 51) { // 3
+ IrSender.sendBoseWave(BOSE_CMD_TUNE_UP); // Tune Up
+ } else if (tSerialCommandCharacter == 52) { // 4
+ IrSender.sendBoseWave(BOSE_CMD_TUNE_DOWN); // Tune Down
+ } else if (tSerialCommandCharacter == 53) { // 5
+ IrSender.sendBoseWave(BOSE_CMD_AM); // AM
+ } else if (tSerialCommandCharacter == 54) { // 6
+ IrSender.sendBoseWave(BOSE_CMD_FM); // FM
+ } else if (tSerialCommandCharacter == 55) { // 7
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_1); // Preset 1
+ } else if (tSerialCommandCharacter == 56) { // 8
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_2); // Preset 2
+ } else if (tSerialCommandCharacter == 57) { // 9
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_3); // Preset 3
+ } else if (tSerialCommandCharacter == 97) { // a
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_4); // Preset 4
+ } else if (tSerialCommandCharacter == 98) { // b
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_5); // Preset 5
+ } else if (tSerialCommandCharacter == 99) { // c
+ IrSender.sendBoseWave(BOSE_CMD_PRESET_6); // Preset 6
+ } else if (tSerialCommandCharacter == 100) { // d
+ IrSender.sendBoseWave(BOSE_CMD_MUTE); // Mute
+ } else if (tSerialCommandCharacter == 101) { // e
+ IrSender.sendBoseWave(BOSE_CMD_PLAY_PAUSE); // Pause
+ } else if (tSerialCommandCharacter == 102) { // f
+ IrSender.sendBoseWave(BOSE_CMD_STOP); // Stop
+ } else if (tSerialCommandCharacter == 103) { // g
+ IrSender.sendBoseWave(BOSE_CMD_AUX); // Aux
+ } else if (tSerialCommandCharacter == 104) { // h
+ IrSender.sendBoseWave(BOSE_CMD_SLEEP); // Sleep
+ } else {
+ sPrintMenu = false;
+ }
+ delay(300);
+ }
+}
+
+void printMenu() {
+ Serial.println("0: On / Off");
+ Serial.println("1: Volume Up");
+ Serial.println("2: Volume Down");
+ Serial.println("3: Tune Up");
+ Serial.println("4: Tune Down");
+ Serial.println("5: AM");
+ Serial.println("6: FM");
+ Serial.println("7: Preset 1");
+ Serial.println("8: Preset 2");
+ Serial.println("9: Preset 3");
+ Serial.println("a: Preset 4");
+ Serial.println("b: Preset 5");
+ Serial.println("c: Preset 6");
+ Serial.println("d: Mute");
+ Serial.println("e: Play/Pause");
+ Serial.println("f: Stop");
+ Serial.println("g: Aux");
+ Serial.println("h: Sleep");
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/IRremote_SendDemo_ReceiveDemo.log b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/IRremote_SendDemo_ReceiveDemo.log
new file mode 100644
index 00000000..d56a9481
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/IRremote_SendDemo_ReceiveDemo.log
@@ -0,0 +1,178 @@
+START ../src/ReceiveDemo.cpp from Feb 24 2023
+Using library version 4.1.0
+Enabling IRin...
+Ready to receive IR signals of protocols: NEC/NEC2/Onkyo/Apple, Panasonic/Kaseikyo, Denon/Sharp, Sony, RC5, RC6, LG, JVC, Samsung, FAST, Whynter, Lego Power Functions, Bosewave , MagiQuest, Universal Pulse Distance Width, Hash at pin 2
+
+If you connect debug pin 5 to ground, raw data is always printed
+5000 us is the (minimum) gap, after which the start of a new IR packet is assumed
+20 us are subtracted from all marks and added to all spaces for decoding
+
+Protocol=NEC Address=0x2 Command=0x34 Raw-Data=0xCB34FD02 32 bits LSB first
+Send with: IrSender.sendNEC(0x2, 0x34, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=NEC Address=0x80 Command=0x45 Raw-Data=0xBA457F80 32 bits LSB first
+Send with: IrSender.sendNEC(0x80, 0x45, );
+
+Protocol=NEC Address=0x4 Command=0x8 Raw-Data=0xF708FB04 32 bits LSB first
+Send with: IrSender.sendNEC(0x4, 0x8, );
+
+Protocol=Onkyo Address=0x102 Command=0x304 Raw-Data=0x3040102 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x102, 0x304, );
+
+Protocol=NEC Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendNEC(0x102, 0x34, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=Panasonic Address=0xB Command=0x10 Raw-Data=0xA01000B0 48 bits LSB first
+Send with: IrSender.sendPanasonic(0xB, 0x10, );
+
+Protocol=PulseDistance Raw-Data=0x5A 72 bits LSB first
+Send with:
+ uint32_t tRawData[]={0x87654321, 0xAFEDCBA9, 0x5A};
+ IrSender.sendPulseDistanceWidthFromArray(38, 8850, 4400, 550, 1700, 550, 600, &tRawData[0], 72, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=PulseWidth Raw-Data=0xDCBA9 52 bits LSB first
+Send with:
+ uint32_t tRawData[]={0x87654321, 0xDCBA9};
+ IrSender.sendPulseDistanceWidthFromArray(38, 300, 600, 600, 300, 350, 600, &tRawData[0], 52, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=PulseWidth Raw-Data=0x87654321 32 bits LSB first
+Send with: IrSender.sendPulseDistanceWidth(38, 1000, 500, 600, 300, 350, 300, 0x87654321, 32, PROTOCOL_IS_LSB_FIRST, , );
+
+Protocol=Onkyo Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x102, 0x5634, );
+
+Protocol=Apple Address=0x2 Command=0x34 Raw-Data=0x23487EE 32 bits LSB first
+Send with: IrSender.sendApple(0x2, 0x34, );
+
+Protocol=Panasonic Address=0x102 Command=0x34 Raw-Data=0x4341020 48 bits LSB first
+Send with: IrSender.sendPanasonic(0x102, 0x34, );
+
+Protocol=Kaseikyo Address=0x102 Command=0x34 Extra=0x4711 Raw-Data=0x7341023 48 bits LSB first
+Send with: IrSender.sendKaseikyo(0x102, 0x34, , 0x4711);
+
+Protocol=Kaseikyo_Denon Address=0x102 Command=0x34 Raw-Data=0x4341020 48 bits LSB first
+Send with: IrSender.sendKaseikyo_Denon(0x102, 0x34, );
+
+Protocol=Denon Address=0x2 Command=0x34 Raw-Data=0x682 15 bits LSB first
+Send with: IrSender.sendDenon(0x2, 0x34, );
+
+Protocol=Denon Address=0x2 Command=0x34 Auto-Repeat gap=45650us Raw-Data=0x7962 15 bits LSB first
+
+Protocol=Sharp Address=0x2 Command=0x34 Raw-Data=0x4682 15 bits LSB first
+Send with: IrSender.sendSharp(0x2, 0x34, );
+
+Protocol=Sharp Address=0x2 Command=0x34 Auto-Repeat gap=46400us Raw-Data=0x3962 15 bits LSB first
+
+Protocol=Sony Address=0x2 Command=0x34 Raw-Data=0x134 12 bits LSB first
+Send with: IrSender.sendSony(0x2, 0x34, 2, 12);
+
+Protocol=Sony Address=0x2 Command=0x34 Raw-Data=0x134 15 bits LSB first
+Send with: IrSender.sendSony(0x2, 0x34, 2, 15);
+
+Protocol=Sony Address=0x102 Command=0x34 Raw-Data=0x8134 20 bits LSB first
+Send with: IrSender.sendSony(0x102, 0x34, 2, 20);
+
+Protocol=Samsung Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x5634, );
+
+Protocol=Samsung48 Address=0x102 Command=0x5634 Raw-Data=0xA956 48 bits LSB first
+Send with: IrSender.sendSamsung48(0x102, 0x5634, );
+
+Protocol=RC5 Address=0x2 Command=0x34 Raw-Data=0x10B4 13 bits MSB first
+Send with: IrSender.sendRC5(0x2, 0x34, );
+
+Protocol=RC5 Address=0x2 Command=0x74 Toggle=1 Raw-Data=0x8B4 13 bits MSB first
+Send with: IrSender.sendRC5(0x2, 0x74, );
+
+Protocol=RC6 Address=0x2 Command=0x34 Raw-Data=0x234 20 bits MSB first
+Send with: IrSender.sendRC6(0x2, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x34 Raw-Data=0xCB340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x34, );
+
+Protocol=JVC Address=0x2 Command=0x34 Raw-Data=0x3402 16 bits LSB first
+Send with: IrSender.sendJVC(0x2, 0x34, );
+
+Protocol=Samsung Address=0x102 Command=0x5634 Raw-Data=0x56340102 32 bits LSB first
+Send with: IrSender.sendSamsung(0x102, 0x5634, );
+
+Protocol=LG Address=0x2 Command=0x5634 Raw-Data=0x256342 28 bits MSB first
+Send with: IrSender.sendLG(0x2, 0x5634, );
+
+Protocol=MagiQuest Address=0x102 Command=0x34 Raw-Data=0x6BCD0102 56 bits MSB first
+Send with: IrSender.sendMagiQuest(0x6BCD0102, 0x34, );
+
+Protocol=BoseWave Address=0x0 Command=0x34 Raw-Data=0xCB34 16 bits LSB first
+Send with: IrSender.sendBoseWave(0x0, 0x34, );
+
+Protocol=FAST Address=0x0 Command=0x34 Raw-Data=0xCB34 16 bits LSB first
+Send with: IrSender.sendFAST(0x0, 0x34, );
+
+Protocol=Lego Address=0x2 Command=0x14 Raw-Data=0x2148 16 bits MSB first
+Send with: IrSender.sendLego(0x2, 0x14, );
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=180450us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179350us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179200us Raw-Data=0x2148 16 bits MSB first
+
+Protocol=Lego Address=0x2 Command=0x14 Auto-Repeat gap=179150us Raw-Data=0x2148 16 bits MSB first
+
+Overflow detected
+Try to increase the "RAW_BUFFER_LENGTH" value of 600 in ../src/ReceiveDemo.cpp
+
+Protocol=NEC Address=0x3 Command=0x45 Raw-Data=0xBA45FC03 32 bits LSB first
+Send with: IrSender.sendNEC(0x3, 0x45, );
+
+Protocol=NEC Address=0x3 Command=0x45 Repeat gap=43250us
+
+Protocol=NEC Address=0x203 Command=0x45 Raw-Data=0xBA450203 32 bits LSB first
+Send with: IrSender.sendNEC(0x203, 0x45, );
+
+Protocol=NEC Address=0x203 Command=0x45 Repeat gap=47550us
+
+Protocol=NEC Address=0x203 Command=0x45 Raw-Data=0xBA450203 32 bits LSB first
+Send with: IrSender.sendNEC(0x203, 0x45, );
+
+Protocol=NEC2 Address=0x203 Command=0x45 Repeat gap=46500us Raw-Data=0xBA450203 32 bits LSB first
+
+Protocol=Onkyo Address=0x203 Command=0x6745 Raw-Data=0x67450203 32 bits LSB first
+Send with: IrSender.sendOnkyo(0x203, 0x6745, );
+
+Protocol=Onkyo Address=0x203 Command=0x6745 Repeat gap=46550us
+
+Protocol=Apple Address=0x3 Command=0x45 Raw-Data=0x34587EE 32 bits LSB first
+Send with: IrSender.sendApple(0x3, 0x45, );
+
+Protocol=Apple Address=0x3 Command=0x45 Repeat gap=31550us
+
+Protocol=Panasonic Address=0x203 Command=0x45 Raw-Data=0x55452030 48 bits LSB first
+Send with: IrSender.sendPanasonic(0x203, 0x45, );
+
+Protocol=Panasonic Address=0x203 Command=0x45 Repeat gap=72550us Raw-Data=0x55452030 48 bits LSB first
+
+Protocol=Kaseikyo Address=0x203 Command=0x45 Extra=0x4711 Raw-Data=0x56452033 48 bits LSB first
+Send with: IrSender.sendKaseikyo(0x203, 0x45, , 0x4711);
+
+Protocol=Kaseikyo Address=0x203 Command=0x45 Extra=0x4711 Repeat gap=66750us Raw-Data=0x56452033 48 bits LSB first
+
+Protocol=Kaseikyo_Denon Address=0x203 Command=0x45 Raw-Data=0x55452030 48 bits LSB first
+Send with: IrSender.sendKaseikyo_Denon(0x203, 0x45, );
+
+Protocol=Kaseikyo_Denon Address=0x203 Command=0x45 Repeat gap=68300us Raw-Data=0x55452030 48 bits LSB first
\ No newline at end of file
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/SendDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/SendDemo.ino
new file mode 100644
index 00000000..27dc4696
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendDemo/SendDemo.ino
@@ -0,0 +1,436 @@
+/*
+ * SendDemo.cpp
+ *
+ * Demonstrates sending IR codes in standard format with address and command
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+#include
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+
+#define DISABLE_CODE_FOR_RECEIVER // Disables restarting receiver after each send. Saves 450 bytes program memory and 269 bytes RAM if receiving functions are not used.
+
+//#define EXCLUDE_EXOTIC_PROTOCOLS // Saves around 240 bytes program memory if IrSender.write is used
+//#define SEND_PWM_BY_TIMER // Disable carrier PWM generation in software and use (restricted) hardware PWM.
+//#define USE_NO_SEND_PWM // Use no carrier PWM, just simulate an active low receiver signal. Overrides SEND_PWM_BY_TIMER definition
+//#define NO_LED_FEEDBACK_CODE // Saves 566 bytes program memory
+//#define USE_OPEN_DRAIN_OUTPUT_FOR_SEND_PIN // Use or simulate open drain output mode at send pin. Attention, active state of open drain is LOW, so connect the send LED between positive supply and send pin!
+
+//#undef IR_SEND_PIN // enable this, if you need to set send pin programmatically using uint8_t tSendPin below
+#include
+
+#define DELAY_AFTER_SEND 2000
+#define DELAY_AFTER_LOOP 5000
+
+void setup() {
+ Serial.begin(115200);
+
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+
+#if defined(IR_SEND_PIN)
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+# if defined(IR_SEND_PIN_STRING)
+ Serial.println(F("Send IR signals at pin " IR_SEND_PIN_STRING));
+# else
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+# endif
+#else
+ // Here the macro IR_SEND_PIN is not defined or undefined above with #undef IR_SEND_PIN
+ uint8_t tSendPin = 3;
+ IrSender.begin(tSendPin, ENABLE_LED_FEEDBACK, USE_DEFAULT_FEEDBACK_LED_PIN); // Specify send pin and enable feedback LED at default feedback LED pin
+ // You can change send pin later with IrSender.setSendPin();
+
+ Serial.print(F("Send IR signals at pin "));
+ Serial.println(tSendPin);
+#endif
+
+#if !defined(SEND_PWM_BY_TIMER)
+ /*
+ * Print internal software PWM signal generation info
+ */
+ IrSender.enableIROut(38); // Call it with 38 kHz just to initialize the values printed below
+ Serial.print(F("Send signal mark duration is "));
+ Serial.print(IrSender.periodOnTimeMicros);
+ Serial.print(F(" us, pulse narrowing correction is "));
+ Serial.print(IrSender.getPulseCorrectionNanos());
+ Serial.print(F(" ns, total period is "));
+ Serial.print(IrSender.periodTimeMicros);
+ Serial.println(F(" us"));
+#endif
+}
+
+/*
+ * Set up the data to be sent.
+ * For most protocols, the data is build up with a constant 8 (or 16 byte) address
+ * and a variable 8 bit command.
+ * There are exceptions like Sony and Denon, which have 5 bit address.
+ */
+uint16_t sAddress = 0x0102;
+uint8_t sCommand = 0x34;
+uint16_t s16BitCommand = 0x5634;
+uint8_t sRepeats = 0;
+
+void loop() {
+ /*
+ * Print values
+ */
+ Serial.println();
+ Serial.print(F("address=0x"));
+ Serial.print(sAddress, HEX);
+ Serial.print(F(" command=0x"));
+ Serial.print(sCommand, HEX);
+ Serial.print(F(" repeats="));
+ Serial.println(sRepeats);
+ Serial.println();
+ Serial.println();
+ Serial.flush();
+
+ Serial.println(F("Send NEC with 8 bit address"));
+ Serial.flush();
+ IrSender.sendNEC(sAddress & 0xFF, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND); // delay must be greater than 5 ms (RECORD_GAP_MICROS), otherwise the receiver sees it as one long signal
+
+ Serial.println(F("Send NEC with 16 bit address"));
+ Serial.flush();
+ IrSender.sendNEC(sAddress, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send NEC2 with 16 bit address"));
+ Serial.flush();
+ IrSender.sendNEC2(sAddress, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ if (sRepeats == 0) {
+#if FLASHEND >= 0x3FFF && (RAMEND >= 0x4FF || RAMSIZE >= 0x4FF) // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ /*
+ * Send constant values only once in this demo
+ */
+ Serial.println(F("Sending NEC Pronto data with 8 bit address 0x80 and command 0x45 and no repeats"));
+ Serial.flush();
+ IrSender.sendPronto(F("0000 006D 0022 0000 015E 00AB " /* Pronto header + start bit */
+ "0017 0015 0017 0015 0017 0017 0015 0017 0017 0015 0017 0015 0017 0015 0017 003F " /* Lower address byte */
+ "0017 003F 0017 003E 0017 003F 0015 003F 0017 003E 0017 003F 0017 003E 0017 0015 " /* Upper address byte (inverted at 8 bit mode) */
+ "0017 003E 0017 0015 0017 003F 0017 0015 0017 0015 0017 0015 0017 003F 0017 0015 " /* command byte */
+ "0019 0013 0019 003C 0017 0015 0017 003F 0017 003E 0017 003F 0017 0015 0017 003E " /* inverted command byte */
+ "0017 0806"), 0); //stop bit, no repeat possible, because of missing repeat pattern
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * !!! The next data occupies 136 bytes RAM !!!
+ */
+ Serial.println(
+ F("Send NEC sendRaw data with 8 bit address=0xFB04 and command 0x08 and exact timing (16 bit array format)"));
+ Serial.flush();
+ const uint16_t irSignal[] = { 9000, 4500/*Start bit*/, 560, 560, 560, 560, 560, 1690, 560,
+ 560/*0010 0x4 of 16 bit address LSB first*/, 560, 560, 560, 560, 560, 560, 560, 560/*0000*/, 560, 1690, 560, 1690,
+ 560, 560, 560, 1690/*1101 0xB*/, 560, 1690, 560, 1690, 560, 1690, 560, 1690/*1111*/, 560, 560, 560, 560, 560, 560,
+ 560, 1690/*0001 0x08 of command LSB first*/, 560, 560, 560, 560, 560, 560, 560, 560/*0000 0x00*/, 560, 1690, 560,
+ 1690, 560, 1690, 560, 560/*1110 Inverted 8 of command*/, 560, 1690, 560, 1690, 560, 1690, 560,
+ 1690/*1111 inverted 0 of command*/, 560 /*stop bit*/}; // Using exact NEC timing
+ IrSender.sendRaw(irSignal, sizeof(irSignal) / sizeof(irSignal[0]), NEC_KHZ); // Note the approach used to automatically calculate the size of the array.
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * With sendNECRaw() you can send 32 bit combined codes
+ */
+ Serial.println(F("Send ONKYO with 16 bit address 0x0102 and 16 bit command 0x0304 with NECRaw(0x03040102)"));
+ Serial.flush();
+ IrSender.sendNECRaw(0x03040102, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * With Send sendNECMSB() you can send your old 32 bit codes.
+ * To convert one into the other, you must reverse the byte positions and then reverse all positions of each byte.
+ * Use bitreverse32Bit().
+ * Example:
+ * 0xCB340102 byte reverse -> 0x020134CB bit reverse-> 40802CD3
+ */
+ Serial.println(F("Send ONKYO with 16 bit address 0x0102 and command 0x34 with old 32 bit format MSB first (0x40802CD3)"));
+ Serial.flush();
+ IrSender.sendNECMSB(0x40802CD3, 32, false);
+ delay(DELAY_AFTER_SEND);
+#endif
+
+ Serial.println(F("Send Panasonic 0xB, 0x10 as 48 bit PulseDistance using ProtocolConstants"));
+ Serial.flush();
+#if __INT_WIDTH__ < 32
+ IRRawDataType tRawData[] = { 0xB02002, 0xA010 }; // LSB of tRawData[0] is sent first
+ IrSender.sendPulseDistanceWidthFromArray(&KaseikyoProtocolConstants, &tRawData[0], 48, NO_REPEATS); // Panasonic is a Kaseikyo variant
+#else
+ IrSender.sendPulseDistanceWidth(&KaseikyoProtocolConstants, 0xA010B02002, 48, NO_REPEATS); // Panasonic is a Kaseikyo variant
+#endif
+
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * Send 2 Panasonic 48 bit codes as generic Pulse Distance data, once with LSB and once with MSB first
+ */
+ Serial.println(F("Send Panasonic 0xB, 0x10 as generic 48 bit PulseDistance"));
+ Serial.println(F(" LSB first"));
+ Serial.flush();
+#if __INT_WIDTH__ < 32
+ IrSender.sendPulseDistanceWidthFromArray(38, 3450, 1700, 450, 1250, 450, 400, &tRawData[0], 48,
+ PROTOCOL_IS_LSB_FIRST, 0, NO_REPEATS);
+#else
+ IrSender.sendPulseDistanceWidth(38, 3450, 1700, 450, 1250, 450, 400, 0xA010B02002, 48, PROTOCOL_IS_LSB_FIRST,
+ 0, NO_REPEATS);
+#endif
+ delay(DELAY_AFTER_SEND);
+
+ // The same with MSB first. Use bit reversed raw data of LSB first part
+ Serial.println(F(" MSB first"));
+#if __INT_WIDTH__ < 32
+ tRawData[0] = 0x40040D00; // MSB of tRawData[0] is sent first
+ tRawData[1] = 0x805;
+ IrSender.sendPulseDistanceWidthFromArray(38, 3450, 1700, 450, 1250, 450, 400, &tRawData[0], 48,
+ PROTOCOL_IS_MSB_FIRST, 0, NO_REPEATS);
+#else
+ IrSender.sendPulseDistanceWidth(38, 3450, 1700, 450, 1250, 450, 400, 0x40040D000805, 48, PROTOCOL_IS_MSB_FIRST,
+ 0, NO_REPEATS);
+#endif
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send generic 72 bit PulseDistance 0x5A AFEDCBA9 87654321 LSB first"));
+ Serial.flush();
+# if __INT_WIDTH__ < 32
+ tRawData[0] = 0x87654321; // LSB of tRawData[0] is sent first
+ tRawData[1] = 0xAFEDCBA9;
+ tRawData[2] = 0x5A;
+ IrSender.sendPulseDistanceWidthFromArray(38, 8900, 4450, 550, 1700, 550, 600, &tRawData[0], 72, PROTOCOL_IS_LSB_FIRST, 0,
+ NO_REPEATS);
+# else
+ IRRawDataType tRawData[] = { 0xAFEDCBA987654321, 0x5A }; // LSB of tRawData[0] is sent first
+ IrSender.sendPulseDistanceWidthFromArray(38, 8900, 4450, 550, 1700, 550, 600, &tRawData[0], 72, PROTOCOL_IS_LSB_FIRST, 0, NO_REPEATS);
+# endif
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send generic 52 bit PulseDistanceWidth 0xDCBA9 87654321 LSB first"));
+ Serial.flush();
+ // Real PulseDistanceWidth (constant bit length) does not require a stop bit
+#if __INT_WIDTH__ < 32
+ IrSender.sendPulseDistanceWidthFromArray(38, 300, 600, 600, 300, 300, 600, &tRawData[0], 52,
+ PROTOCOL_IS_LSB_FIRST, 0, 0);
+#else
+ IrSender.sendPulseDistanceWidth(38, 300, 600, 600, 300, 300, 600, 0xDCBA987654321, 52, PROTOCOL_IS_LSB_FIRST,
+ 0, 0);
+#endif
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send generic 32 bit PulseWidth 0x87654321 LSB first"));
+ Serial.flush();
+ // Real PulseDistanceWidth (constant bit length) does not require a stop bit
+ IrSender.sendPulseDistanceWidth(38, 1000, 500, 600, 300, 300, 300, 0x87654321, 32, PROTOCOL_IS_LSB_FIRST, 0, 0);
+ delay(DELAY_AFTER_SEND);
+ }
+
+ Serial.println(F("Send Onkyo (NEC with 16 bit command)"));
+ Serial.flush();
+ IrSender.sendOnkyo(sAddress, s16BitCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Apple"));
+ Serial.flush();
+ IrSender.sendApple(sAddress & 0xFF, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Panasonic"));
+ Serial.flush();
+ IrSender.sendPanasonic(sAddress & 0xFFF, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Kaseikyo with 0x4711 as Vendor ID"));
+ Serial.flush();
+ IrSender.sendKaseikyo(sAddress & 0xFFF, sCommand, sRepeats, 0x4711);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Kaseikyo_Denon variant"));
+ Serial.flush();
+ IrSender.sendKaseikyo_Denon(sAddress & 0xFFF, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Denon"));
+ Serial.flush();
+ IrSender.sendDenon(sAddress & 0x1F, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Denon/Sharp variant"));
+ Serial.flush();
+ IrSender.sendSharp(sAddress & 0x1F, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Sony/SIRCS with 7 command and 5 address bits"));
+ Serial.flush();
+ IrSender.sendSony(sAddress & 0x1F, sCommand & 0x7F, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Sony/SIRCS with 7 command and 8 address bits"));
+ Serial.flush();
+ IrSender.sendSony(sAddress & 0xFF, sCommand, sRepeats, SIRCS_15_PROTOCOL);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Sony/SIRCS with 7 command and 13 address bits"));
+ Serial.flush();
+ IrSender.sendSony(sAddress & 0x1FFF, sCommand & 0x7F, sRepeats, SIRCS_20_PROTOCOL);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Samsung 8 bit command"));
+ Serial.flush();
+ IrSender.sendSamsung(sAddress, sCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Samsung 16 bit command"));
+ Serial.flush();
+ IrSender.sendSamsung(sAddress, s16BitCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send Samsung48 16 bit command"));
+ Serial.flush();
+ IrSender.sendSamsung48(sAddress, s16BitCommand, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send RC5"));
+ Serial.flush();
+ IrSender.sendRC5(sAddress & 0x1F, sCommand & 0x3F, sRepeats, true); // 5 address, 6 command bits
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send RC5X with 7.th MSB of command set"));
+ Serial.flush();
+ IrSender.sendRC5(sAddress & 0x1F, (sCommand & 0x3F) + 0x40, sRepeats, true); // 5 address, 7 command bits
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send RC6"));
+ Serial.flush();
+ IrSender.sendRC6(sAddress, sCommand, sRepeats, true);
+ delay(DELAY_AFTER_SEND);
+
+#if FLASHEND >= 0x3FFF && (RAMEND >= 0x4FF || RAMSIZE >= 0x4FF) // For 16k flash or more, like ATtiny1604. Code does not fit in program memory of ATtiny85 etc.
+ /*
+ * Next example how to use the IrSender.write function
+ */
+ IRData IRSendData;
+ // prepare data
+ IRSendData.address = sAddress;
+ IRSendData.command = sCommand;
+ IRSendData.flags = IRDATA_FLAGS_EMPTY;
+
+ IRSendData.protocol = SAMSUNG;
+ Serial.print(F("Send "));
+ Serial.println(getProtocolString(IRSendData.protocol));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ IRSendData.protocol = JVC; // switch protocol
+ Serial.print(F("Send "));
+ Serial.println(getProtocolString(IRSendData.protocol));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ IRSendData.command = s16BitCommand; // LG support more than 8 bit command
+
+ IRSendData.protocol = SAMSUNG;
+ Serial.print(F("Send "));
+ Serial.println(getProtocolString(IRSendData.protocol));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ IRSendData.protocol = LG;
+ Serial.print(F("Send "));
+ Serial.println(getProtocolString(IRSendData.protocol));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ Serial.println(F("Send MagiQuest"));
+ Serial.flush();
+ IrSender.sendMagiQuest(0x6BCD0000 | (uint32_t) sAddress, s16BitCommand); // we have 31 bit address
+ delay(DELAY_AFTER_SEND);
+
+ // Bang&Olufsen must be sent with 455 kHz
+// Serial.println(F("Send Bang&Olufsen"));
+// Serial.flush();
+// IrSender.sendBangOlufsen(sAddress, sCommand, sRepeats);
+// delay(DELAY_AFTER_SEND);
+
+ IRSendData.protocol = BOSEWAVE;
+ Serial.println(F("Send Bosewave with no address and 8 command bits"));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ IRSendData.protocol = FAST;
+ Serial.print(F("Send "));
+ Serial.println(getProtocolString(IRSendData.protocol));
+ Serial.flush();
+ IrSender.write(&IRSendData, sRepeats);
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * LEGO is difficult to receive because of its short marks and spaces
+ */
+ Serial.println(F("Send Lego with 2 channel and with 4 command bits"));
+ Serial.flush();
+ IrSender.sendLegoPowerFunctions(sAddress, sCommand, LEGO_MODE_COMBO, true);
+ delay(DELAY_AFTER_SEND);
+
+#endif // FLASHEND
+ /*
+ * Force buffer overflow
+ */
+ Serial.println(F("Force buffer overflow by sending 700 marks and spaces"));
+ for (unsigned int i = 0; i < 350; ++i) {
+ // 400 + 400 should be received as 8/8 and sometimes as 9/7 or 7/9 if compensation by MARK_EXCESS_MICROS is optimal.
+ // 210 + 540 = 750 should be received as 5/10 or 4/11 if compensation by MARK_EXCESS_MICROS is optimal.
+ IrSender.mark(210); // 8 pulses at 38 kHz
+ IrSender.space(540); // to fill up to 750 us
+ }
+ delay(DELAY_AFTER_SEND);
+
+ /*
+ * Increment values
+ * Also increment address just for demonstration, which normally makes no sense
+ */
+ sAddress += 0x0101;
+ sCommand += 0x11;
+ s16BitCommand += 0x1111;
+ sRepeats++;
+ // clip repeats at 4
+ if (sRepeats > 4) {
+ sRepeats = 4;
+ }
+
+ delay(DELAY_AFTER_LOOP); // additional delay at the end of each loop
+}
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/Readme.md b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/Readme.md
new file mode 100644
index 00000000..ad16367c
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/Readme.md
@@ -0,0 +1,93 @@
+=== decoding for LG A/C ====
+- 1) remote of LG AC has two type of HDR mark/space, 8000/4000 and 3100/10000
+- 2) HDR 8000/4000 is decoded using decodeLG(IRrecvDumpV2) without problem
+- 3) for HDR 3100/10000, use AnalysIR's code : http://www.analysir.com/blog/2014/03/19/air-conditioners-problems-recording-long-infrared-remote-control-signals-arduino/
+- 4) for bin output based on AnalysIR's code : https://gist.github.com/chaeplin/a3a4b4b6b887c663bfe8
+- 5) remove first two byte(11)
+- 6) sample rawcode with bin output : https://gist.github.com/chaeplin/134d232e0b8cfb898860
+
+
+=== *** ===
+- 1) Sample raw code : https://gist.github.com/chaeplin/ab2a7ad1533c41260f0d
+- 2) send raw code : https://gist.github.com/chaeplin/7c800d3166463bb51be4
+
+
+=== *** ===
+- (0) : Cooling or Heating
+- (1) : fixed address
+- (2) : fixed address
+- (3) : special(power, swing, air clean)
+- (4) : change air flow, temperature, cooling(0)/heating(4)
+- (5) : temperature ( 15 + (5) = )
+- (6) : air flow
+- (7) : checksum ( 3 + 4 + 5 + 6 ) & B00001111
+
+
+°F = °C × 1.8 + 32
+°C = (°F - 32) / 1.8
+
+
+=== *** ===
+* remote / Korea / without heating
+
+| status |(0)| (1)| (2)| (3)| (4)| (5)| (6)| (7)
+|----------------|---|----|----|----|----|----|----|----
+| on / 25 / mid | C |1000|1000|0000|0000|1010|0010|1100
+| on / 26 / mid | C |1000|1000|0000|0000|1011|0010|1101
+| on / 27 / mid | C |1000|1000|0000|0000|1100|0010|1110
+| on / 28 / mid | C |1000|1000|0000|0000|1101|0010|1111
+| on / 25 / high | C |1000|1000|0000|0000|1010|0100|1110
+| on / 26 / high | C |1000|1000|0000|0000|1011|0100|1111
+| on / 27 / high | C |1000|1000|0000|0000|1100|0100|0000
+| on / 28 / high | C |1000|1000|0000|0000|1101|0100|0001
+|----------------|---|----|----|----|----|----|----|----
+| 1 up | C |1000|1000|0000|1000|1101|0100|1001
+|----------------|---|----|----|----|----|----|----|----
+| Cool power | C |1000|1000|0001|0000|0000|1100|1101
+| energy saving | C |1000|1000|0001|0000|0000|0100|0101
+| power | C |1000|1000|0001|0000|0000|1000|1001
+| flow/up/down | C |1000|1000|0001|0011|0001|0100|1001
+| up/down off | C |1000|1000|0001|0011|0001|0101|1010
+| flow/left/right| C |1000|1000|0001|0011|0001|0110|1011
+| left/right off | C |1000|1000|0001|0011|0001|0111|1100
+|----------------|---|----|----|----|----|----|----|----
+| Air clean | C |1000|1000|1100|0000|0000|0000|1100
+|----------------|---|----|----|----|----|----|----|----
+| off | C |1000|1000|1100|0000|0000|0101|0001
+
+
+
+* remote / with heating
+* converted using raw code at https://github.com/chaeplin/RaspAC/blob/master/lircd.conf
+
+| status |(0)| (1)| (2)| (3)| (4)| (5)| (6)| (7)
+|----------------|---|----|----|----|----|----|----|----
+| on | C |1000|1000|0000|0000|1011|0010|1101
+|----------------|---|----|----|----|----|----|----|----
+| off | C |1000|1000|1100|0000|0000|0101|0001
+|----------------|---|----|----|----|----|----|----|----
+| 64 / 18 | C |1000|1000|0000|0000|0011|0100|0111
+| 66 / 19 | C |1000|1000|0000|0000|0100|0100|1000
+| 68 / 20 | C |1000|1000|0000|0000|0101|0100|1001
+| 70 / 21 | C |1000|1000|0000|0000|0110|0100|1010
+| 72 / 22 | C |1000|1000|0000|0000|0111|0100|1011
+| 74 / 23 | C |1000|1000|0000|0000|1000|0100|1100
+| 76 / 25 | C |1000|1000|0000|0000|1010|0100|1110
+| 78 / 26 | C |1000|1000|0000|0000|1011|0100|1111
+| 80 / 27 | C |1000|1000|0000|0000|1100|0100|0000
+| 82 / 28 | C |1000|1000|0000|0000|1101|0100|0001
+| 84 / 29 | C |1000|1000|0000|0000|1110|0100|0010
+| 86 / 30 | C |1000|1000|0000|0000|1111|0100|0011
+|----------------|---|----|----|----|----|----|----|----
+| heat64 | H |1000|1000|0000|0100|0011|0100|1011
+| heat66 | H |1000|1000|0000|0100|0100|0100|1100
+| heat68 | H |1000|1000|0000|0100|0101|0100|1101
+| heat70 | H |1000|1000|0000|0100|0110|0100|1110
+| heat72 | H |1000|1000|0000|0100|0111|0100|1111
+| heat74 | H |1000|1000|0000|0100|1000|0100|0000
+| heat76 | H |1000|1000|0000|0100|1001|0100|0001
+| heat78 | H |1000|1000|0000|0100|1011|0100|0011
+| heat80 | H |1000|1000|0000|0100|1100|0100|0100
+| heat82 | H |1000|1000|0000|0100|1101|0100|0101
+| heat84 | H |1000|1000|0000|0100|1110|0100|0110
+| heat86 | H |1000|1000|0000|0100|1111|0100|0111
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/SendLGAirConditionerDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/SendLGAirConditionerDemo.ino
new file mode 100644
index 00000000..9697745e
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendLGAirConditionerDemo/SendLGAirConditionerDemo.ino
@@ -0,0 +1,149 @@
+/*
+ * SendLGAirConditionerDemo.cpp
+ *
+ * Sending LG air conditioner IR codes controlled by Serial input
+ * Based on he old IRremote source from https://github.com/chaeplin
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+/*
+ * LG2 has different header timing and a shorter bit time
+ * Known LG remote controls, which uses LG2 protocol are:
+ * AKB75215403
+ * AKB74955603
+ * AKB73757604:
+ */
+//#define USE_LG2_PROTOCOL // Try it if you do not have success with the default LG protocol
+#define NUMBER_OF_COMMANDS_BETWEEN_PRINT_OF_MENU 5
+
+#define DISABLE_CODE_FOR_RECEIVER // Disables restarting receiver after each send. Saves 450 bytes program memory and 269 bytes RAM if receiving functions are not used.
+
+#define INFO // Deactivate this to save program memory and suppress info output from the LG-AC driver.
+//#define DEBUG // Activate this for more output from the LG-AC driver.
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) || defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__)
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+#endif
+
+#include "ac_LG.hpp"
+
+#define SIZE_OF_RECEIVE_BUFFER 10
+char sRequestString[SIZE_OF_RECEIVE_BUFFER];
+
+Aircondition_LG MyLG_Aircondition;
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+// Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+
+ /*
+ * The IR library setup. That's all!
+ */
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+
+
+ Serial.println();
+ MyLG_Aircondition.setType(LG_IS_WALL_TYPE);
+ MyLG_Aircondition.printMenu(&Serial);
+
+ delay(1000);
+
+// test
+// MyLG_Aircondition.sendCommandAndParameter('j', 1);
+// delay(5000);
+// MyLG_Aircondition.sendCommandAndParameter('f', 3);
+// delay(5000);
+
+}
+
+void loop() {
+ static uint8_t sShowmenuConter = 0;
+
+ if (Serial.available()) {
+ /*
+ * Get parameters from serial
+ */
+ uint8_t tNumberOfBytesReceived = Serial.readBytesUntil('\n', sRequestString, SIZE_OF_RECEIVE_BUFFER - 1);
+ // handle CR LF
+ if (sRequestString[tNumberOfBytesReceived - 1] == '\r') {
+ tNumberOfBytesReceived--;
+ }
+ sRequestString[tNumberOfBytesReceived] = '\0'; // terminate as string
+ char tCommand = sRequestString[0];
+
+ /*
+ * Handle parameter numbers which can be greater 9
+ */
+ int tParameter = 0;
+ if (tNumberOfBytesReceived >= 2) {
+ tParameter = sRequestString[1] - '0';
+ if (tCommand == LG_COMMAND_TEMPERATURE || tCommand == LG_COMMAND_SWING || tCommand == LG_COMMAND_SLEEP
+ || tCommand == LG_COMMAND_TIMER_ON || tCommand == LG_COMMAND_TIMER_OFF) {
+ tParameter = atoi(&sRequestString[1]);
+ }
+ }
+
+ /*
+ * Print command to send
+ */
+ Serial.println();
+ Serial.print(F("Command="));
+ Serial.print(tCommand);
+ if (tParameter != 0) {
+ Serial.print(F(" Parameter="));
+ Serial.print(tParameter);
+ }
+ Serial.println();
+
+ if (!MyLG_Aircondition.sendCommandAndParameter(tCommand, tParameter)) {
+ Serial.print(F("Error: unknown command or invalid parameter in \""));
+ Serial.print(sRequestString);
+ Serial.println('\"');
+ }
+
+ if (sShowmenuConter == 0) {
+ MyLG_Aircondition.printMenu(&Serial);
+ sShowmenuConter = NUMBER_OF_COMMANDS_BETWEEN_PRINT_OF_MENU;
+ } else {
+ sShowmenuConter--;
+ }
+ }
+ delay(100);
+}
+
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/SendProntoDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/SendProntoDemo.ino
new file mode 100644
index 00000000..ba92eff2
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendProntoDemo/SendProntoDemo.ino
@@ -0,0 +1,102 @@
+/*
+ * SendProntoDemo.cpp
+ *
+ * Example for sending pronto codes with the IRremote library.
+ * The code used here, sends NEC protocol data.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#define DISABLE_CODE_FOR_RECEIVER // Disables restarting receiver after each send. Saves 450 bytes program memory and 269 bytes RAM if receiving functions are not used.
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+#define NUMBER_OF_REPEATS 3U
+
+// The first number, here 0000, denotes the type of the signal. 0000 denotes a raw IR signal with modulation.
+// The second number, here 006C, denotes a frequency code. 006C corresponds to 1000000/(0x006c * 0.241246) = 38381 Hertz.
+// The third and the forth number denote the number of pairs (= half the number of durations) in the start- and the repeat sequence respectively.
+const char yamahaVolDown[]
+#if defined(__AVR__)
+PROGMEM
+#endif
+= "0000 006C 0022 0002 015B 00AD " /* Pronto header + start bit */
+ "0016 0016 0016 0041 0016 0016 0016 0041 0016 0041 0016 0041 0016 0041 0016 0016 " /* Lower address byte */
+ "0016 0041 0016 0016 0016 0041 0016 0016 0016 0016 0016 0016 0016 0016 0016 0041 " /* Upper address byte (inverted at 8 bit mode) */
+ "0016 0041 0016 0041 0016 0016 0016 0041 0016 0041 0016 0016 0016 0016 0016 0016 " /* command byte */
+ "0016 0016 0016 0016 0016 0041 0016 0016 0016 0016 0016 0041 0016 0041 0016 0041 0016 05F7 " /* inverted command byte + stop bit */
+ "015B 0057 0016 0E6C"; /* NEC repeat pattern*/
+
+IRsend irsend;
+
+void setup() {
+ Serial.begin(115200);
+ while (!Serial)
+ ;
+
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+}
+
+void loop() {
+
+#if defined(__AVR__)
+ Serial.println(F("Sending NEC from PROGMEM: address 0x85, data 0x1B"));
+ irsend.sendPronto_P(yamahaVolDown, NUMBER_OF_REPEATS);
+#else
+ Serial.println(F("Sending from normal memory"));
+ irsend.sendPronto(yamahaVolDown, NUMBER_OF_REPEATS);
+#endif
+
+ delay(2000);
+ Serial.println(F("Sending the NEC from PROGMEM using the F()-form: address 0x5, data 0x1A"));
+ irsend.sendPronto(F("0000 006C 0022 0002 015B 00AD " /* Pronto header + start bit */
+ "0016 0016 0016 0041 0016 0016 0016 0041 0016 0041 0016 0041 0016 0041 0016 0041 " /* Lower address byte */
+ "0016 0041 0016 0016 0016 0041 0016 0016 0016 0016 0016 0016 0016 0016 0016 0016 " /* Upper address byte (inverted at 8 bit mode) */
+ "0016 0016 0016 0041 0016 0016 0016 0041 0016 0041 0016 0016 0016 0016 0016 0016 " /* command byte */
+ "0016 0041 0016 0016 0016 0041 0016 0016 0016 0016 0016 0041 0016 0041 0016 0041 0016 05F7 " /* inverted command byte + stop bit */
+ "015B 0057 0016 0E6C"), /* NEC repeat pattern*/
+ NUMBER_OF_REPEATS);
+ delay(2000);
+
+ // send Nec code acquired by IRreceiveDump.cpp
+ Serial.println(F("Sending NEC from RAM: address 0xFF00, data 0x15"));
+ // 006D -> 38029 Hz
+ irsend.sendPronto("0000 006D 0022 0000 015C 00AB " /* Pronto header + start bit */
+ "0017 0015 0017 0015 0017 0015 0017 0015 0017 0015 0017 0015 0017 0015 0017 0015 " /* Lower address byte */
+ "0017 003F 0017 003E 0017 003F 0017 003E 0017 003F 0015 003F 0017 003F 0015 003F " /* Upper address byte (inverted at 8 bit mode) */
+ "0017 003E 0017 0015 0017 003F 0017 0015 0017 003E 0017 0015 0017 0017 0015 0017 " /* command byte */
+ "0017 0015 0017 003E 0017 0015 0017 003F 0015 0017 0017 003E 0017 003F 0015 003F 0017 0806" /* inverted command byte + stop bit */
+ , 0); // No repeat possible, because of missing repeat pattern
+
+ delay(5000);
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/SendRawDemo.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/SendRawDemo.ino
new file mode 100644
index 00000000..351148ad
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SendRawDemo/SendRawDemo.ino
@@ -0,0 +1,120 @@
+/*
+ * SendRawDemo.cpp - demonstrates sending IR codes with sendRaw
+ *
+ * This example shows how to send a RAW signal using the IRremote library.
+ * The example signal is actually a 32 bit NEC signal.
+ * Remote Control button: LGTV Power On/Off.
+ * Hex Value: 0x20DF10EF, 32 bits
+ *
+ * If it is a supported protocol, it is more efficient to use the protocol send function
+ * (here sendNEC) to send the signal.
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2022 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+#include
+
+#define DISABLE_CODE_FOR_RECEIVER // Disables restarting receiver after each send. Saves 450 bytes program memory and 269 bytes RAM if receiving functions are not used.
+
+//#define SEND_PWM_BY_TIMER // Disable carrier PWM generation in software and use (restricted) hardware PWM.
+//#define USE_NO_SEND_PWM // Use no carrier PWM, just simulate an active low receiver signal. Overrides SEND_PWM_BY_TIMER definition
+//#define NO_LED_FEEDBACK_CODE // Saves 566 bytes program memory
+//#define USE_OPEN_DRAIN_OUTPUT_FOR_SEND_PIN // Use or simulate open drain output mode at send pin. Attention, active state of open drain is LOW, so connect the send LED between positive supply and send pin!
+
+#include "PinDefinitionsAndMore.h" // Define macros for input and output pin etc.
+#include
+
+void setup() {
+ pinMode(LED_BUILTIN, OUTPUT);
+
+ Serial.begin(115200);
+#if defined(__AVR_ATmega32U4__) || defined(SERIAL_PORT_USBVIRTUAL) || defined(SERIAL_USB) /*stm32duino*/|| defined(USBCON) /*STM32_stm32*/|| defined(SERIALUSB_PID) || defined(ARDUINO_attiny3217)
+ delay(4000); // To be able to connect Serial monitor after reset or power up and before first print out. Do not wait for an attached Serial Monitor!
+#endif
+ // Just to know which program is running on my Arduino
+ Serial.println(F("START " __FILE__ " from " __DATE__ "\r\nUsing library version " VERSION_IRREMOTE));
+ Serial.println(F("Send IR signals at pin " STR(IR_SEND_PIN)));
+
+ IrSender.begin(); // Start with IR_SEND_PIN as send pin and enable feedback LED at default feedback LED pin
+}
+
+/*
+ * NEC address=0xFB0C, command=0x18
+ *
+ * This is data in byte format.
+ * The uint8_t/byte elements contain the number of ticks in 50 us.
+ * The uint16_t format contains the (number of ticks * 50) if generated by IRremote,
+ * so the uint16_t format has exact the same resolution but requires double space.
+ * With the uint16_t format, you are able to modify the timings to meet the standards,
+ * e.g. use 560 (instead of 11 * 50) for NEC or use 432 for Panasonic. But in this cases,
+ * you better use the timing generation functions e.g. sendNEC() directly.
+ */
+const uint8_t rawDataP[]
+#if defined(__AVR__)
+PROGMEM
+#endif
+= { 180, 90 /*Start bit*/, 11, 11, 11, 11, 11, 34, 11, 34/*0011 0xC of 16 bit address LSB first*/, 11, 11, 11, 11, 11, 11, 11,
+ 11/*0000*/, 11, 34, 11, 34, 11, 11, 11, 34/*1101 0xB*/, 11, 34, 11, 34, 11, 34, 11, 34/*1111*/, 11, 11, 11, 11, 11, 11, 11,
+ 34/*0001 0x08 of command LSB first*/, 11, 34, 11, 11, 11, 11, 11, 11/*1000 0x01*/, 11, 34, 11, 34, 11, 34, 11,
+ 11/*1110 Inverted 8 of command*/, 11, 11, 11, 34, 11, 34, 11, 34/*0111 inverted 1 of command*/, 11 /*stop bit*/};
+
+void loop() {
+
+#if !(defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__))
+ /*
+ * Send hand crafted data from RAM
+ * The values are NOT multiple of 50, but are taken from the NEC timing definitions
+ */
+ Serial.println(F("Send NEC 16 bit address=0xFB04 and command 0x08 with exact timing (16 bit array format)"));
+ Serial.flush();
+
+ const uint16_t rawData[] = { 9000, 4500/*Start bit*/, 560, 560, 560, 560, 560, 1690, 560,
+ 560/*0010 0x4 of 16 bit address LSB first*/, 560, 560, 560, 560, 560, 560, 560, 560/*0000*/, 560, 1690, 560, 1690, 560,
+ 560, 560, 1690/*1101 0xB*/, 560, 1690, 560, 1690, 560, 1690, 560, 1690/*1111*/, 560, 560, 560, 560, 560, 560, 560,
+ 1690/*0001 0x08 of command LSB first*/, 560, 560, 560, 560, 560, 560, 560, 560/*0000 0x00*/, 560, 1690, 560, 1690, 560,
+ 1690, 560, 560/*1110 Inverted 8 of command*/, 560, 1690, 560, 1690, 560, 1690, 560, 1690/*1111 inverted 0 of command*/,
+ 560 /*stop bit*/}; // Using exact NEC timing
+ IrSender.sendRaw(rawData, sizeof(rawData) / sizeof(rawData[0]), NEC_KHZ); // Note the approach used to automatically calculate the size of the array.
+
+ delay(1000); // delay must be greater than 5 ms (RECORD_GAP_MICROS), otherwise the receiver sees it as one long signal
+#endif
+
+ /*
+ * Send byte data direct from FLASH
+ * Note the approach used to automatically calculate the size of the array.
+ */
+ Serial.println(F("Send NEC 16 bit address 0xFB0C and data 0x18 with (50 us) tick resolution timing (8 bit array format) "));
+ Serial.flush();
+ IrSender.sendRaw_P(rawDataP, sizeof(rawDataP) / sizeof(rawDataP[0]), NEC_KHZ);
+
+ delay(1000); // delay must be greater than 5 ms (RECORD_GAP_MICROS), otherwise the receiver sees it as one long signal
+
+ Serial.println(F("Send NEC 16 bit address 0x0102, 8 bit data 0x34 with generated timing"));
+ Serial.flush();
+ IrSender.sendNEC(0x0102, 0x34, 0);
+
+ delay(3000);
+}
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/PinDefinitionsAndMore.h b/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/PinDefinitionsAndMore.h
new file mode 100644
index 00000000..54395f68
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/PinDefinitionsAndMore.h
@@ -0,0 +1,345 @@
+/*
+ * PinDefinitionsAndMore.h
+ *
+ * Contains pin definitions for IRremote examples for various platforms
+ * as well as definitions for feedback LED and tone() and includes
+ *
+ * Copyright (C) 2021-2023 Armin Joachimsmeyer
+ * armin.joachimsmeyer@gmail.com
+ *
+ * This file is part of IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ * Arduino-IRremote is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
+ * See the GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ *
+ */
+
+/*
+ * Pin mapping table for different platforms
+ *
+ * Platform IR input IR output Tone Core/Pin schema
+ * --------------------------------------------------------------
+ * DEFAULT/AVR 2 3 4 Arduino
+ * ATtinyX5 0|PB0 4|PB4 3|PB3 ATTinyCore
+ * ATtiny167 3|PA3 2|PA2 7|PA7 ATTinyCore
+ * ATtiny167 9|PA3 8|PA2 5|PA7 Digispark original core
+ * ATtiny84 |PB2 |PA4 |PA3 ATTinyCore
+ * ATtiny88 3|PD3 4|PD4 9|PB1 ATTinyCore
+ * ATtiny3217 18|PA1 19|PA2 20|PA3 MegaTinyCore
+ * ATtiny1604 2 3|PA5 %
+ * ATtiny816 14|PA1 16|PA3 1|PA5 MegaTinyCore
+ * ATtiny1614 8|PA1 10|PA3 1|PA5 MegaTinyCore
+ * SAMD21 3 4 5
+ * ESP8266 14|D5 12|D6 %
+ * ESP32 15 4 27
+ * BluePill PA6 PA7 PA3
+ * APOLLO3 11 12 5
+ * RP2040 3|GPIO15 4|GPIO16 5|GPIO17
+ */
+//#define _IR_MEASURE_TIMING // For debugging purposes.
+
+#if defined(__AVR__)
+#if defined(__AVR_ATtiny25__) || defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__) // Digispark board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // TX is at pin 2 - Available as Arduino library "ATtinySerialOut". Saves 700 bytes program memory and 70 bytes RAM for ATtinyCore.
+#define IR_RECEIVE_PIN PIN_PB0
+#define IR_SEND_PIN PIN_PB4 // Pin 2 is serial output with ATtinySerialOut. Pin 1 is internal LED and Pin3 is USB+ with pullup on Digispark board.
+#define TONE_PIN PIN_PB3
+#define _IR_TIMING_TEST_PIN PIN_PB3
+
+# elif defined(__AVR_ATtiny87__) || defined(__AVR_ATtiny167__) // Digispark pro board
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut"
+// For ATtiny167 Pins PB6 and PA3 are usable as interrupt source.
+# if defined(ARDUINO_AVR_DIGISPARKPRO)
+// For use with Digispark original core
+#define IR_RECEIVE_PIN 9 // PA3 - on Digispark board labeled as pin 9
+//#define IR_RECEIVE_PIN 14 // PB6 / INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN 8 // PA2 - on Digispark board labeled as pin 8
+#define TONE_PIN 5 // PA7 - on Digispark board labeled as pin 5
+#define _IR_TIMING_TEST_PIN 10 // PA4
+# else
+// For use with ATTinyCore
+#define IR_RECEIVE_PIN PIN_PA3 // On Digispark board labeled as pin 9 - INT0 is connected to USB+ on DigisparkPro boards
+#define IR_SEND_PIN PIN_PA2 // On Digispark board labeled as pin 8
+#define TONE_PIN PIN_PA7 // On Digispark board labeled as pin 5
+# endif
+
+# elif defined(__AVR_ATtiny84__) // For use with ATTinyCore
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+#define IR_RECEIVE_PIN PIN_PB2 // INT0
+#define IR_SEND_PIN PIN_PA4
+#define TONE_PIN PIN_PA3
+#define _IR_TIMING_TEST_PIN PIN_PA5
+
+# elif defined(__AVR_ATtiny88__) // MH-ET Tiny88 board. For use with ATTinyCore.
+#include "ATtinySerialOut.hpp" // Available as Arduino library "ATtinySerialOut". Saves 128 bytes program memory.
+// Pin 6 is TX, pin 7 is RX
+#define IR_RECEIVE_PIN PIN_PD3 // 3 - INT1
+#define IR_SEND_PIN PIN_PD4 // 4
+#define TONE_PIN PIN_PB1 // 9
+#define _IR_TIMING_TEST_PIN PIN_PB0 // 8
+
+# elif defined(__AVR_ATtiny1616__) || defined(__AVR_ATtiny3216__) || defined(__AVR_ATtiny3217__) // For use with megaTinyCore
+// Tiny Core Dev board
+// https://www.tindie.com/products/xkimi/tiny-core-16-dev-board-attiny1616/ - Out of Stock
+// https://www.tindie.com/products/xkimi/tiny-core-32-dev-board-attiny3217/ - Out of Stock
+#define IR_RECEIVE_PIN PIN_PA1 // use 18 instead of PIN_PA1 for TinyCore32
+#define IR_SEND_PIN PIN_PA2 // 19
+#define TONE_PIN PIN_PA3 // 20
+#define APPLICATION_PIN PIN_PA0 // 0
+#undef LED_BUILTIN // No LED available on the TinyCore 32 board, take the one on the programming board which is connected to the DAC output
+#define LED_BUILTIN PIN_PA6 // use 2 instead of PIN_PA6 for TinyCore32
+
+# elif defined(__AVR_ATtiny816__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 14
+#define IR_SEND_PIN PIN_PA1 // 16
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+#undef LED_BUILTIN // No LED available, take the one which is connected to the DAC output
+#define LED_BUILTIN PIN_PB5 // 4
+
+# elif defined(__AVR_ATtiny1614__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA1 // 8
+#define IR_SEND_PIN PIN_PA3 // 10
+#define TONE_PIN PIN_PA5 // 1
+#define APPLICATION_PIN PIN_PA4 // 0
+
+# elif defined(__AVR_ATtiny1604__) // For use with megaTinyCore
+#define IR_RECEIVE_PIN PIN_PA6 // 2 - To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN PIN_PA7 // 3
+#define APPLICATION_PIN PIN_PB2 // 5
+
+#define tone(...) void() // Define as void, since TCB0_INT_vect is also used by tone()
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+# elif defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__) \
+|| defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) \
+|| defined(__AVR_ATmega324P__) || defined(__AVR_ATmega324A__) \
+|| defined(__AVR_ATmega324PA__) || defined(__AVR_ATmega164A__) \
+|| defined(__AVR_ATmega164P__) || defined(__AVR_ATmega32__) \
+|| defined(__AVR_ATmega16__) || defined(__AVR_ATmega8535__) \
+|| defined(__AVR_ATmega64__) || defined(__AVR_ATmega128__) \
+|| defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2561__) \
+|| defined(__AVR_ATmega8515__) || defined(__AVR_ATmega162__)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 13
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# else // Default as for ATmega328 like on Uno, Nano, Leonardo, Teensy 2.0 etc.
+#define IR_RECEIVE_PIN 2 // To be compatible with interrupt example, pin 2 is chosen here.
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+# if defined(ARDUINO_AVR_PROMICRO) // Sparkfun Pro Micro is __AVR_ATmega32U4__ but has different external circuit
+// We have no built in LED at pin 13 -> reuse RX LED
+#undef LED_BUILTIN
+#define LED_BUILTIN LED_BUILTIN_RX
+# endif
+# endif // defined(__AVR_ATtiny25__)...
+
+#elif defined(ARDUINO_ARCH_RENESAS_UNO) // Uno R4
+// To be compatible with Uno R3.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ESP8266)
+#define FEEDBACK_LED_IS_ACTIVE_LOW // The LED on my board (D4) is active LOW
+#define IR_RECEIVE_PIN 14 // D5
+#define IR_SEND_PIN 12 // D6 - D4/pin 2 is internal LED
+#define _IR_TIMING_TEST_PIN 2 // D4
+#define APPLICATION_PIN 13 // D7
+
+#define tone(...) void() // tone() inhibits receive timer
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#elif defined(CONFIG_IDF_TARGET_ESP32C3)
+#define IR_RECEIVE_PIN 8
+#define IR_SEND_PIN 9
+#define TONE_PIN 10 // ADC2_0
+#define APPLICATION_PIN 11
+
+#elif defined(ESP32)
+#include
+
+// tone() is included in ESP32 core since 2.0.2
+#if !defined(ESP_ARDUINO_VERSION_VAL)
+#define ESP_ARDUINO_VERSION_VAL(major, minor, patch) 12345678
+#endif
+#if ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+#define TONE_LEDC_CHANNEL 1 // Using channel 1 makes tone() independent of receiving timer -> No need to stop receiving timer.
+void tone(uint8_t aPinNumber, unsigned int aFrequency){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+}
+void tone(uint8_t aPinNumber, unsigned int aFrequency, unsigned long aDuration){
+ ledcAttachPin(aPinNumber, TONE_LEDC_CHANNEL);
+ ledcWriteTone(TONE_LEDC_CHANNEL, aFrequency);
+ delay(aDuration);
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+void noTone(uint8_t aPinNumber){
+ ledcWriteTone(TONE_LEDC_CHANNEL, 0);
+}
+#endif // ESP_ARDUINO_VERSION <= ESP_ARDUINO_VERSION_VAL(2, 0, 2)
+
+#define IR_RECEIVE_PIN 15 // D15
+#define IR_SEND_PIN 4 // D4
+#define TONE_PIN 27 // D27 25 & 26 are DAC0 and 1
+#define APPLICATION_PIN 16 // RX2 pin
+
+#elif defined(ARDUINO_ARCH_STM32) || defined(ARDUINO_ARCH_STM32F1) // BluePill
+// Timer 3 blocks PA6, PA7, PB0, PB1 for use by Servo or tone()
+#define IR_RECEIVE_PIN PA6
+#define IR_RECEIVE_PIN_STRING "PA6"
+#define IR_SEND_PIN PA7
+#define IR_SEND_PIN_STRING "PA7"
+#define TONE_PIN PA3
+#define _IR_TIMING_TEST_PIN PA5
+#define APPLICATION_PIN PA2
+#define APPLICATION_PIN_STRING "PA2"
+# if defined(ARDUINO_GENERIC_STM32F103C) || defined(ARDUINO_BLUEPILL_F103C8)
+// BluePill LED is active low
+#define FEEDBACK_LED_IS_ACTIVE_LOW
+# endif
+
+#elif defined(ARDUINO_ARCH_APOLLO3) // Sparkfun Apollo boards
+#define IR_RECEIVE_PIN 11
+#define IR_SEND_PIN 12
+#define TONE_PIN 5
+
+#elif defined(ARDUINO_ARCH_MBED) && defined(ARDUINO_ARCH_MBED_NANO) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 3 // GPIO15 Start with pin 3 since pin 2|GPIO25 is connected to LED on Pi pico
+#define IR_SEND_PIN 4 // GPIO16
+#define TONE_PIN 5
+#define APPLICATION_PIN 6
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 7 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 8
+
+#elif defined(ARDUINO_ARCH_RP2040) // Arduino Nano Connect, Pi Pico with arduino-pico core https://github.com/earlephilhower/arduino-pico
+#define IR_RECEIVE_PIN 15 // GPIO15 to be compatible with the Arduino Nano RP2040 Connect (pin3)
+#define IR_SEND_PIN 16 // GPIO16
+#define TONE_PIN 17
+#define APPLICATION_PIN 18
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 19 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 20
+
+// If you program the Nano RP2040 Connect with this core, then you must redefine LED_BUILTIN
+// and use the external reset with 1 kOhm to ground to enter UF2 mode
+#undef LED_BUILTIN
+#define LED_BUILTIN 6
+
+#elif defined(PARTICLE) // !!!UNTESTED!!!
+#define IR_RECEIVE_PIN A4
+#define IR_SEND_PIN A5 // Particle supports multiple pins
+
+#define LED_BUILTIN D7
+
+/*
+ * 4 times the same (default) layout for easy adaption in the future
+ */
+#elif defined(TEENSYDUINO) // Teensy 2.0 is handled at default for ATmega328 like on Uno, Nano, Leonardo etc.
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_MBED) // Arduino Nano 33 BLE
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#elif defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_SAM)
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+
+#if !defined(ARDUINO_SAMD_ADAFRUIT) && !defined(ARDUINO_SEEED_XIAO_M0)
+// On the Zero and others we switch explicitly to SerialUSB
+#define Serial SerialUSB
+#endif
+
+// Definitions for the Chinese SAMD21 M0-Mini clone, which has no led connected to D13/PA17.
+// Attention!!! D2 and D4 are swapped on these boards!!!
+// If you connect the LED, it is on pin 24/PB11. In this case activate the next two lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 24 // PB11
+// As an alternative you can choose pin 25, it is the RX-LED pin (PB03), but active low.In this case activate the next 3 lines.
+//#undef LED_BUILTIN
+//#define LED_BUILTIN 25 // PB03
+//#define FEEDBACK_LED_IS_ACTIVE_LOW // The RX LED on the M0-Mini is active LOW
+
+#elif defined (NRF51) // BBC micro:bit
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define APPLICATION_PIN 1
+#define _IR_TIMING_TEST_PIN 4
+
+#define tone(...) void() // no tone() available
+#define noTone(a) void()
+#define TONE_PIN 42 // Dummy for examples using it
+
+#else
+#warning Board / CPU is not detected using pre-processor symbols -> using default values, which may not fit. Please extend PinDefinitionsAndMore.h.
+// Default valued for unidentified boards
+#define IR_RECEIVE_PIN 2
+#define IR_SEND_PIN 3
+#define TONE_PIN 4
+#define APPLICATION_PIN 5
+#define ALTERNATIVE_IR_FEEDBACK_LED_PIN 6 // E.g. used for examples which use LED_BUILDIN for example output.
+#define _IR_TIMING_TEST_PIN 7
+#endif // defined(ESP8266)
+
+#if defined(ESP32) || defined(ARDUINO_ARCH_RP2040) || defined(PARTICLE) || defined(ARDUINO_ARCH_MBED)
+#define SEND_PWM_BY_TIMER // We do not have pin restrictions for this CPU's, so lets use the hardware PWM for send carrier signal generation
+#else
+# if defined(SEND_PWM_BY_TIMER)
+#undef IR_SEND_PIN // SendPin is determined by timer! This avoids warnings in IRremote.hpp and IRTimer.hpp
+# endif
+#endif
+
+#if !defined (FLASHEND)
+#define FLASHEND 0xFFFF // Dummy value for platforms where FLASHEND is not defined
+#endif
+#if !defined (RAMEND)
+#define RAMEND 0xFFFF // Dummy value for platforms where RAMEND is not defined
+#endif
+#if !defined (RAMSIZE)
+#define RAMSIZE 0xFFFF // Dummy value for platforms where RAMSIZE is not defined
+#endif
+
+/*
+ * Helper macro for getting a macro definition as string
+ */
+#if !defined(STR_HELPER)
+#define STR_HELPER(x) #x
+#define STR(x) STR_HELPER(x)
+#endif
diff --git a/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/SimpleReceiver.ino b/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/SimpleReceiver.ino
new file mode 100644
index 00000000..12b950b2
--- /dev/null
+++ b/libraries/_07_m_Sensor_IR_Remote/examples/SimpleReceiver/SimpleReceiver.ino
@@ -0,0 +1,123 @@
+/*
+ * SimpleReceiver.cpp
+ *
+ * Demonstrates receiving NEC IR codes with IRremote
+ *
+ * This file is part of Arduino-IRremote https://github.com/Arduino-IRremote/Arduino-IRremote.
+ *
+ ************************************************************************************
+ * MIT License
+ *
+ * Copyright (c) 2020-2023 Armin Joachimsmeyer
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to deal
+ * in the Software without restriction, including without limitation the rights
+ * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ * copies of the Software, and to permit persons to whom the Software is furnished
+ * to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in all
+ * copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
+ * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
+ * PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+ * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
+ * CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE
+ * OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+ *
+ ************************************************************************************
+ */
+
+/*
+ * Specify which protocol(s) should be used for decoding.
+ * If no protocol is defined, all protocols (except Bang&Olufsen) are active.
+ * This must be done before the #include
+ */
+//#define DECODE_DENON // Includes Sharp
+//#define DECODE_JVC
+//#define DECODE_KASEIKYO
+//#define DECODE_PANASONIC // alias for DECODE_KASEIKYO
+//#define DECODE_LG
+#define DECODE_NEC // Includes Apple and Onkyo
+//#define DECODE_SAMSUNG
+//#define DECODE_SONY
+//#define DECODE_RC5
+//#define DECODE_RC6
+
+//#define DECODE_BOSEWAVE
+//#define DECODE_LEGO_PF
+//#define DECODE_MAGIQUEST
+//#define DECODE_WHYNTER
+//#define DECODE_FAST
+
+//#define DECODE_DISTANCE_WIDTH // Universal decoder for pulse distance width protocols
+//#define DECODE_HASH // special decoder for all protocols
+
+//#define DECODE_BEO // This protocol must always be enabled manually, i.e. it is NOT enabled if no protocol is defined. It prevents decoding of SONY!
+
+//#define DEBUG // Activate this for lots of lovely debug output from the decoders.
+
+//#define RAW_BUFFER_LENGTH 180 // Default is 112 if DECODE_MAGIQUEST is enabled, otherwise 100.
+
+#include
+
+/*
+ * This include defines the actual pin number for pins like IR_RECEIVE_PIN, IR_SEND_PIN for many different boards and architectures
+ */
+#include "PinDefinitionsAndMore.h"
+#include