-
Notifications
You must be signed in to change notification settings - Fork 77
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
1 changed file
with
116 additions
and
0 deletions.
There are no files selected for viewing
116 changes: 116 additions & 0 deletions
116
3001-3100/3016. Minimum Number of Pushes to Type Word II.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,116 @@ | ||
# 3016. Minimum Number of Pushes to Type Word II | ||
|
||
- Difficulty: Medium. | ||
- Related Topics: Hash Table, String, Greedy, Sorting, Counting. | ||
- Similar Questions: Letter Combinations of a Phone Number. | ||
|
||
## Problem | ||
|
||
You are given a string `word` containing lowercase English letters. | ||
|
||
Telephone keypads have keys mapped with **distinct** collections of lowercase English letters, which can be used to form words by pushing them. For example, the key `2` is mapped with `["a","b","c"]`, we need to push the key one time to type `"a"`, two times to type `"b"`, and three times to type `"c"` **.** | ||
|
||
It is allowed to remap the keys numbered `2` to `9` to **distinct** collections of letters. The keys can be remapped to **any** amount of letters, but each letter **must** be mapped to **exactly** one key. You need to find the **minimum** number of times the keys will be pushed to type the string `word`. | ||
|
||
Return **the **minimum** number of pushes needed to type **`word` **after remapping the keys**. | ||
|
||
An example mapping of letters to keys on a telephone keypad is given below. Note that `1`, `*`, `#`, and `0` do **not** map to any letters. | ||
|
||
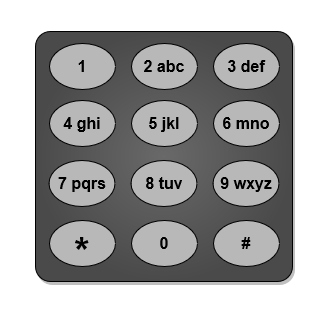 | ||
|
||
|
||
Example 1: | ||
|
||
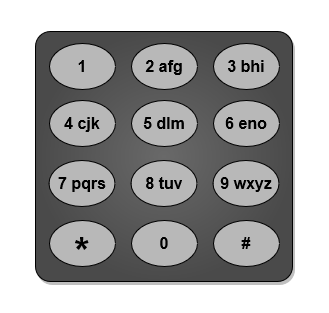 | ||
|
||
``` | ||
Input: word = "abcde" | ||
Output: 5 | ||
Explanation: The remapped keypad given in the image provides the minimum cost. | ||
"a" -> one push on key 2 | ||
"b" -> one push on key 3 | ||
"c" -> one push on key 4 | ||
"d" -> one push on key 5 | ||
"e" -> one push on key 6 | ||
Total cost is 1 + 1 + 1 + 1 + 1 = 5. | ||
It can be shown that no other mapping can provide a lower cost. | ||
``` | ||
|
||
Example 2: | ||
|
||
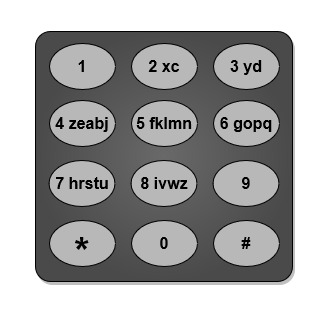 | ||
|
||
``` | ||
Input: word = "xyzxyzxyzxyz" | ||
Output: 12 | ||
Explanation: The remapped keypad given in the image provides the minimum cost. | ||
"x" -> one push on key 2 | ||
"y" -> one push on key 3 | ||
"z" -> one push on key 4 | ||
Total cost is 1 * 4 + 1 * 4 + 1 * 4 = 12 | ||
It can be shown that no other mapping can provide a lower cost. | ||
Note that the key 9 is not mapped to any letter: it is not necessary to map letters to every key, but to map all the letters. | ||
``` | ||
|
||
Example 3: | ||
|
||
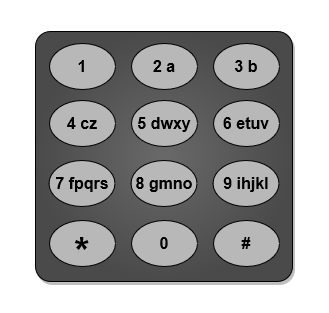 | ||
|
||
``` | ||
Input: word = "aabbccddeeffgghhiiiiii" | ||
Output: 24 | ||
Explanation: The remapped keypad given in the image provides the minimum cost. | ||
"a" -> one push on key 2 | ||
"b" -> one push on key 3 | ||
"c" -> one push on key 4 | ||
"d" -> one push on key 5 | ||
"e" -> one push on key 6 | ||
"f" -> one push on key 7 | ||
"g" -> one push on key 8 | ||
"h" -> two pushes on key 9 | ||
"i" -> one push on key 9 | ||
Total cost is 1 * 2 + 1 * 2 + 1 * 2 + 1 * 2 + 1 * 2 + 1 * 2 + 1 * 2 + 2 * 2 + 6 * 1 = 24. | ||
It can be shown that no other mapping can provide a lower cost. | ||
``` | ||
|
||
|
||
**Constraints:** | ||
|
||
|
||
|
||
- `1 <= word.length <= 105` | ||
|
||
- `word` consists of lowercase English letters. | ||
|
||
|
||
|
||
## Solution | ||
|
||
```javascript | ||
/** | ||
* @param {string} word | ||
* @return {number} | ||
*/ | ||
var minimumPushes = function(word) { | ||
var frequencyMap = Array(26).fill(0); | ||
var a = 'a'.charCodeAt(0); | ||
for (var i = 0; i < word.length; i++) { | ||
frequencyMap[word[i].charCodeAt(0) - a] += 1; | ||
} | ||
var arr = frequencyMap.sort((a, b) => b - a); | ||
var res = 0; | ||
for (var i = 0; i < arr.length; i++) { | ||
res += Math.ceil((i + 1) / 8) * arr[i]; | ||
} | ||
return res; | ||
}; | ||
``` | ||
|
||
**Explain:** | ||
|
||
nope. | ||
|
||
**Complexity:** | ||
|
||
* Time complexity : O(n). | ||
* Space complexity : O(1). |